-
Notifications
You must be signed in to change notification settings - Fork 3
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
0fe3ce0
commit 90ff53c
Showing
6 changed files
with
140 additions
and
40 deletions.
There are no files selected for viewing
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
# 实现数组map方法 | ||
|
||
原文 <https://juejin.cn/post/6844903986479251464#heading-25> | ||
|
||
依照 [ecma262 草案](https://tc39.es/ecma262/#sec-array.prototype.map),实现的map的规范如下: | ||
|
||
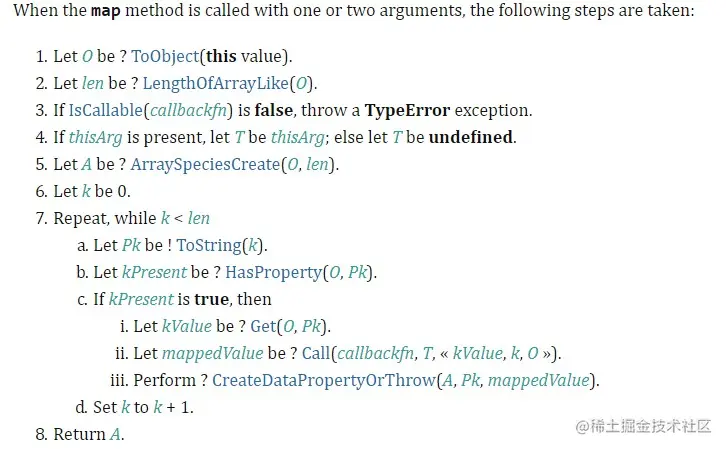 | ||
|
||
```js | ||
Array.prototype.map = function(callbackFn, thisArg) { | ||
// 处理数组类型异常 | ||
if (this === null || this === undefined) { | ||
throw new TypeError("Cannot read property 'map' of null or undefined"); | ||
} | ||
// 处理回调类型异常 | ||
if (Object.prototype.toString.call(callbackfn) != "[object Function]") { | ||
throw new TypeError(callbackfn + ' is not a function') | ||
} | ||
// 草案中提到要先转换为对象 | ||
let O = Object(this); | ||
let T = thisArg; | ||
|
||
let len = O.length >>> 0; // 字面意思是指"右移 0 位",但实际上是把前面的空位用0填充,这里的作用是保证len为数字且为整数。 | ||
let A = new Array(len); | ||
for(let k = 0; k < len; k++) { | ||
// 还记得原型链那一节提到的 in 吗?in 表示在原型链查找 | ||
// 如果用 hasOwnProperty 是有问题的,它只能找私有属性 | ||
// 如果没有找到就不处理,能有效处理稀疏数组的情况。 | ||
if (k in O) { | ||
let kValue = O[k]; | ||
// 依次传入this, 当前项,当前索引,整个数组 | ||
let mappedValue = callbackfn.call(T, KValue, k, O); | ||
A[k] = mappedValue; | ||
} | ||
} | ||
return A; | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
# 实现数组 push、pop 方法 | ||
|
||
原文 <https://juejin.cn/post/6844903986479251464#heading-27> | ||
|
||
参照 ecma262 草案的规定,关于 push 和 pop 的规范如下图所示: | ||
|
||
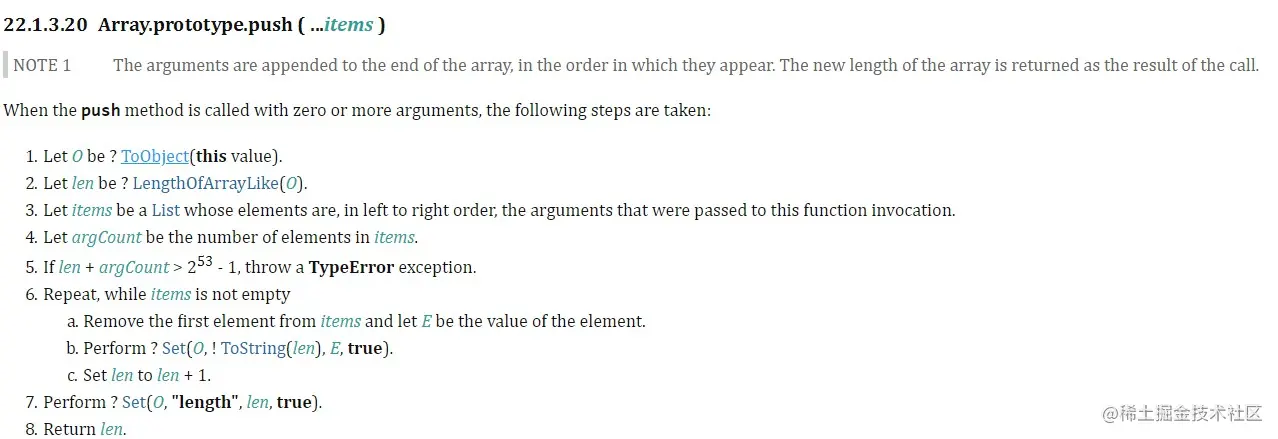 | ||
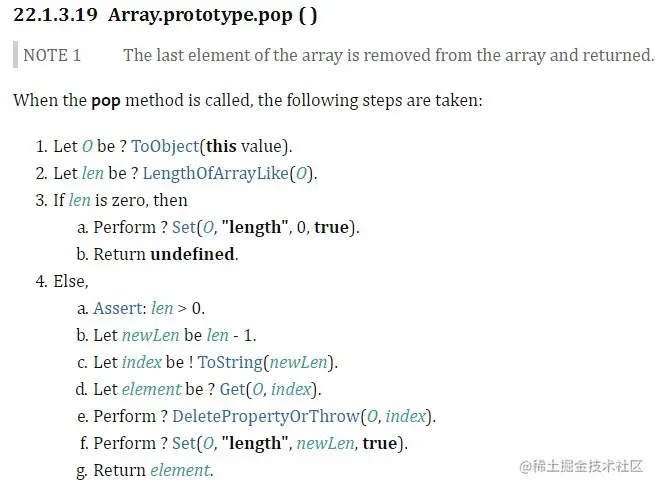 | ||
|
||
```js | ||
Array.prototype.push = function(...items) { | ||
let O = Object(this); | ||
let len = this.length >>> 0; | ||
let argCount = items.length >>> 0; | ||
// 2 ** 53 - 1 为JS能表示的最大正整数 | ||
if (len + argCount > 2 ** 53 - 1) { | ||
throw new TypeError("The number of array is over the max value restricted!") | ||
} | ||
for(let i = 0; i < argCount; i++) { | ||
O[len + i] = items[i]; | ||
} | ||
let newLength = len + argCount; | ||
O.length = newLength; | ||
return newLength; | ||
} | ||
``` | ||
|
||
```js | ||
Array.prototype.pop = function() { | ||
let O = Object(this); | ||
let len = this.length >>> 0; | ||
if (len === 0) { | ||
O.length = 0; | ||
return undefined; | ||
} | ||
len --; | ||
let value = O[len]; | ||
delete O[len]; | ||
O.length = len; | ||
return value; | ||
} | ||
``` |
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,49 @@ | ||
# 实现数组reduce方法 | ||
|
||
原文 <https://juejin.cn/post/6844903986479251464#heading-26> | ||
|
||
依照 [ecma262 草案](https://p1-jj.byteimg.com/tos-cn-i-t2oaga2asx/gold-user-assets/2019/11/3/16e311ed2bfa8fad~tplv-t2oaga2asx-zoom-in-crop-mark:3024:0:0:0.awebp),实现的reduce的规范如下: | ||
|
||
其中有几个核心要点: | ||
|
||
1、初始值不传怎么处理 | ||
2、回调函数的参数有哪些,返回值如何处理。 | ||
|
||
```js | ||
Array.prototype.reduce = function(callbackfn, initialValue) { | ||
// 异常处理,和 map 一样 | ||
// 处理数组类型异常 | ||
if (this === null || this === undefined) { | ||
throw new TypeError("Cannot read property 'reduce' of null or undefined"); | ||
} | ||
// 处理回调类型异常 | ||
if (Object.prototype.toString.call(callbackfn) != "[object Function]") { | ||
throw new TypeError(callbackfn + ' is not a function') | ||
} | ||
let O = Object(this); | ||
let len = O.length >>> 0; | ||
let k = 0; | ||
let accumulator = initialValue; | ||
if (accumulator === undefined) { | ||
for(; k < len ; k++) { | ||
// 查找原型链 | ||
if (k in O) { | ||
accumulator = O[k]; | ||
k++; | ||
break; | ||
} | ||
} | ||
} | ||
// 表示数组全为空 | ||
if(k === len && accumulator === undefined) | ||
throw new Error('Each element of the array is empty'); | ||
console.log(k) | ||
for(;k < len; k++) { | ||
if (k in O) { | ||
// 注意,核心! | ||
accumulator = callbackfn.call(undefined, accumulator, O[k], k, O); | ||
} | ||
} | ||
return accumulator; | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters