-
Notifications
You must be signed in to change notification settings - Fork 0
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
added support for audio and captions #1
Conversation
added support for burning captions for both audio and video. Added support to upload video with animated wave form
For context, this was made with the aim to use it in this autoEdit integration OpenNewsLabs/autoEdit_2#74 |
Trying wrapping new Promise((resolve, reject) => {
ffmpeg('...')
.on('progress', (progress) => {
log.verbose(`[ffmpeg] ${JSON.stringify(progress)}`);
})
.on('error', (err) => {
log.info(`[ffmpeg] error: ${err.message}`);
reject(err);
})
.on('end', () => {
log.verbose('[ffmpeg] finished');
resolve();
})
.save('...');
}); example - /**
* Trims video and match to the specs from the Twitter video documentation.
* See Twitter Docs for for more info
* https://developer.twitter.com/en/docs/media/upload-media/uploading-media/media-best-practices
*/
const ffmpeg = require('fluent-ffmpeg');
function trimVideo(opts, callback) {
if(opts.ffmpegPath !== undefined){
ffmpeg.setFfmpegPath(opts.ffmpegPath)
}
return new Promise((resolve, reject)=>{
ffmpeg(opts.inputFile)
.seekInput(opts.inputSeconds)
.setDuration(opts.durationSeconds)
.output(opts.outputFile)
.audioCodec('aac')
.videoCodec('libx264')
.size('1280x720')
.aspect('16:9')
.format('mp4')
.on('end', ()=>{
resolve(opts.outputFile);
}
)
.on('error',(error)=>{
reject(error);
})
.run();
})
}
module.exports = trimVideo; example usage const trimVideo = require('./index.js');
const path = require('path');
// The `time` argument may be a number (in seconds) or a timestamp string (with format `[[hh:]mm:]ss[.xxx]`).
let opts = {
inputFile: path.join(__dirname, '../../assets/test.mp4'),
inputSeconds: 10,
durationSeconds: 20,
outputFile: path.join(__dirname, '../../assets/test_clipped.mp4')
}
trimVideo(opts)
.then((res)=>{
console.log('in example usage')
console.log(res);
})
.catch(error => console.log(error)); Next, need to look at how changing all the modules like that effects the refactor of main |
both to individual module and to main index.js - still needs a bit more refactor clean up, intermeidate commit
Still a bit of refactoring to wrap up, but had a first go at adding promises to both individual modules and main |
moved tweet video funciton out main index.js and into TweetWithVideo module
and bumbed up version to 2 as changing to promises is a breaking change from previous npm version
Added promises to both individual modules and refactored main Also updated |
See example below
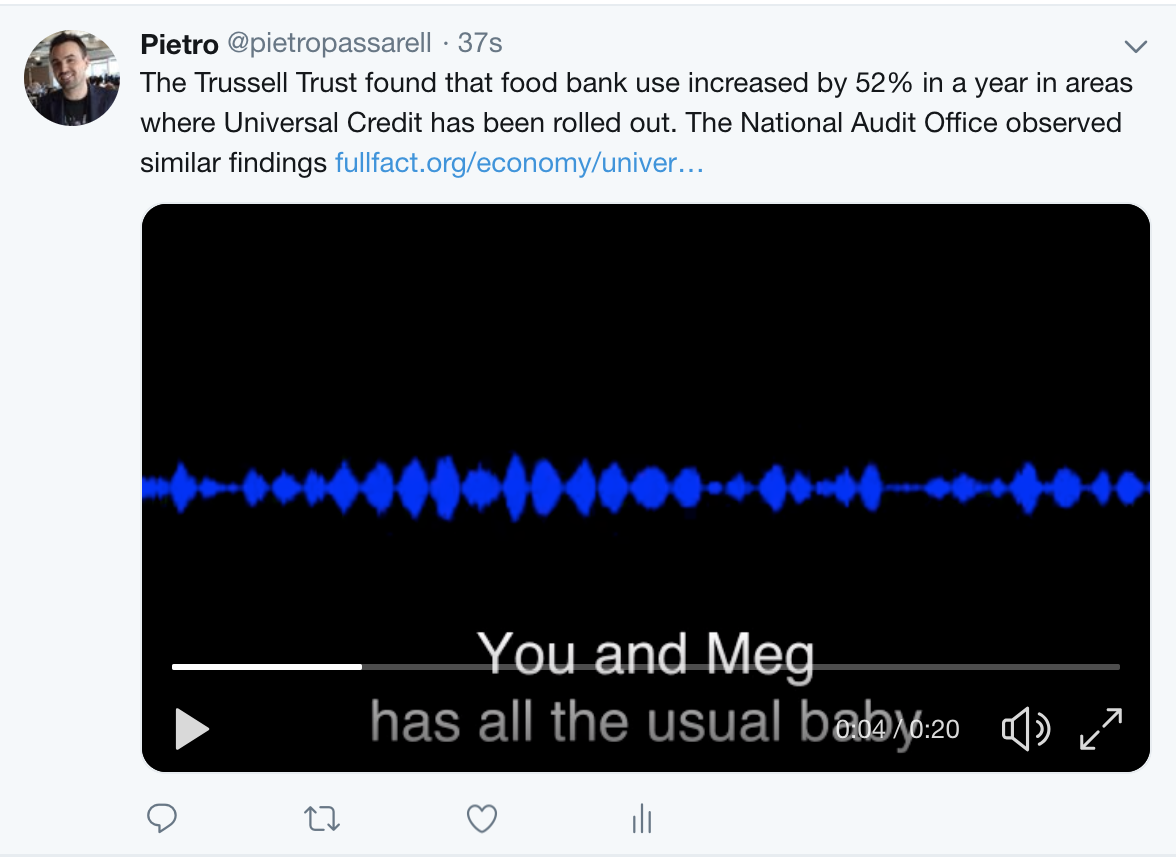
Note that, text of tweet, captions, and media content are randomly paired, for the purpose of testing only might come up with a better example use case later if needed. For now only focusing on functionality.
The code base is quite modular, but here are some things I'd like to improve before merging, with some refactoring
/lib
, (egaudio-to-video-waveform
,burn-captions
,trim
,convert-to-twitter-video-specs
etc..) that usefluent-ffmpeg
, there has to be a better way to wrap apipe
into a function (?) perhaps with promises instead of using callbacks?index.js
could be considerably simplified, to aid readability and future proofing.Welcome, Ideas, suggestions, PRs etc...