diff --git a/Podfile b/Podfile
new file mode 100644
index 0000000..cf258c9
--- /dev/null
+++ b/Podfile
@@ -0,0 +1,21 @@
+platform :ios, '11.0'
+
+target 'iOS-Depth-Sampler' do
+ use_frameworks!
+
+ pod 'SwiftAssetsPickerController', :git => 'https://github.com/shu223/SwiftAssetsPickerController', :branch => 'temp/depth'
+ pod 'Vivid'
+
+end
+
+post_install do |installer|
+ installer.pods_project.targets.each do |target|
+ target.build_configurations.each do |config|
+ config.build_settings['GCC_WARN_INHIBIT_ALL_WARNINGS'] = 'YES'
+ if target.name == 'SwiftAssetsPickerController'
+ config.build_settings['SWIFT_VERSION'] = '4.0'
+ config.build_settings['IPHONEOS_DEPLOYMENT_TARGET'] = '11.0'
+ end
+ end
+ end
+end
diff --git a/Podfile.lock b/Podfile.lock
new file mode 100644
index 0000000..d1cbda1
--- /dev/null
+++ b/Podfile.lock
@@ -0,0 +1,33 @@
+PODS:
+ - CheckMarkView (0.4.2)
+ - SwiftAssetsPickerController (0.4.0):
+ - CheckMarkView (~> 0.4.2)
+ - Vivid (0.9)
+
+DEPENDENCIES:
+ - SwiftAssetsPickerController (from `https://github.com/shu223/SwiftAssetsPickerController`, branch `temp/depth`)
+ - Vivid
+
+SPEC REPOS:
+ https://github.com/cocoapods/specs.git:
+ - CheckMarkView
+ - Vivid
+
+EXTERNAL SOURCES:
+ SwiftAssetsPickerController:
+ :branch: temp/depth
+ :git: https://github.com/shu223/SwiftAssetsPickerController
+
+CHECKOUT OPTIONS:
+ SwiftAssetsPickerController:
+ :commit: 08fc8f3482f143bce3b42a3cf7e7095c2c908377
+ :git: https://github.com/shu223/SwiftAssetsPickerController
+
+SPEC CHECKSUMS:
+ CheckMarkView: 1f911464cf552fa2dea5abc111894468f5f805b8
+ SwiftAssetsPickerController: e8a3b2aac2e57d054a89e91c1bcd244af8141376
+ Vivid: 0fde7409beac71224deb151dd78f98a5bb860497
+
+PODFILE CHECKSUM: ba6f97967707a961ba6dc83c6824573bfda89295
+
+COCOAPODS: 1.5.3
diff --git a/Pods/CheckMarkView/CheckMarkView/Sources/CheckMarkView.swift b/Pods/CheckMarkView/CheckMarkView/Sources/CheckMarkView.swift
new file mode 100644
index 0000000..b3810b2
--- /dev/null
+++ b/Pods/CheckMarkView/CheckMarkView/Sources/CheckMarkView.swift
@@ -0,0 +1,193 @@
+//
+// CheckMarkView.swift
+// CheckMarkView
+//
+// Created by Maxim on 7/18/15.
+// Copyright (c) 2015 Maxim. All rights reserved.
+//
+
+import UIKit
+
+public class CheckMarkView: UIView {
+
+ // MARK: - Enumerations
+
+ public enum Style: Int {
+ case nothing
+ case openCircle
+ case grayedOut
+ }
+
+ // MARK: - Public Properties
+
+ public var checked: Bool {
+ get {
+ return _checked
+ }
+ set(newValue) {
+ _checked = newValue
+ setNeedsDisplay()
+ }
+ }
+
+ public var style: Style {
+ get {
+ return _style
+ }
+ set(newValue) {
+ _style = newValue
+ setNeedsDisplay()
+ }
+ }
+
+ // MARK: - Private Properties
+
+ private var _checked: Bool = false
+ private var _style: Style = .nothing
+
+ // MARK: - Drawing
+
+ override public func draw(_ rect: CGRect) {
+ super.draw(rect)
+
+ if _checked {
+ drawRectChecked(rect: rect)
+ }
+ else {
+ if _style == .openCircle {
+ drawRectOpenCircle(rect: rect)
+ }
+ else if _style == .grayedOut {
+ drawRectGrayedOut(rect: rect)
+ }
+ }
+ }
+
+ func drawRectChecked(rect: CGRect) {
+ guard let context = UIGraphicsGetCurrentContext() else {
+ return
+ }
+
+ let bounds = self.bounds
+
+ let checkmarkBlue = UIColor(red: 0.078, green: 0.435, blue: 0.875, alpha: 1)
+ let shadow = UIColor.black
+ let shadowOffset = CGSize(width: 0.1, height: -0.1)
+ let shadowBlurRadius: CGFloat = 2.5
+
+ let group = CGRect(x: bounds.minX + 3,
+ y: bounds.minY + 3,
+ width: bounds.width - 6,
+ height: bounds.height - 6)
+
+ let checkedOvalPath = UIBezierPath(ovalIn: CGRect(x: group.minX + floor(group.width * 0.00000 + 0.5),
+ y: group.minY + floor(group.height * 0.00000 + 0.5),
+ width: floor(group.width * 1.00000 + 0.5) - floor(group.width * 0.00000 + 0.5),
+ height: floor(group.height * 1.00000 + 0.5) - floor(group.height * 0.00000 + 0.5)))
+
+ context.saveGState()
+ context.setShadow(offset: shadowOffset, blur: shadowBlurRadius, color: shadow.cgColor)
+ checkmarkBlue.setFill()
+ checkedOvalPath.fill()
+ context.restoreGState()
+
+ UIColor.white.setStroke()
+ checkedOvalPath.lineWidth = 1
+ checkedOvalPath.stroke()
+
+ let bezierPath = UIBezierPath()
+ bezierPath.move(to: CGPoint(x: group.minX + 0.27083 * group.width,
+ y: group.minY + 0.54167 * group.height))
+ bezierPath.addLine(to: CGPoint(x: group.minX + 0.41667 * group.width,
+ y: group.minY + 0.68750 * group.height))
+ bezierPath.addLine(to: CGPoint(x: group.minX + 0.75000 * group.width,
+ y: group.minY + 0.35417 * group.height))
+ bezierPath.lineCapStyle = CGLineCap.square
+
+ UIColor.white.setStroke()
+ bezierPath.lineWidth = 1.3
+ bezierPath.stroke()
+ }
+
+ func drawRectOpenCircle(rect: CGRect) {
+ guard let context = UIGraphicsGetCurrentContext() else {
+ return
+ }
+
+ let bounds = self.bounds
+
+ let shadow = UIColor.black
+ let shadowOffset = CGSize(width: 0.1, height: -0.1)
+ let shadowBlurRadius: CGFloat = 0.5
+ let shadow2 = UIColor.black
+ let shadow2Offset = CGSize(width: 0.1, height: -0.1)
+ let shadow2BlurRadius: CGFloat = 2.5
+
+ let group = CGRect(x: bounds.minX + 3,
+ y: bounds.minY + 3,
+ width: bounds.width - 6,
+ height: bounds.height - 6)
+ let emptyOvalPath = UIBezierPath(ovalIn: CGRect(x: group.minX + floor(group.width * 0.00000 + 0.5),
+ y: group.minY + floor(group.height * 0.00000 + 0.5),
+ width: floor(group.width * 1.00000 + 0.5) - floor(group.width * 0.00000 + 0.5),
+ height: floor(group.height * 1.00000 + 0.5) - floor(group.height * 0.00000 + 0.5)))
+
+ context.saveGState()
+ context.setShadow(offset: shadow2Offset, blur: shadow2BlurRadius, color: shadow2.cgColor)
+ context.restoreGState()
+
+ context.saveGState()
+ context.setShadow(offset: shadowOffset, blur: shadowBlurRadius, color: shadow.cgColor)
+ UIColor.white.setStroke()
+ emptyOvalPath.lineWidth = 1
+ emptyOvalPath.stroke()
+ context.restoreGState()
+ }
+
+ func drawRectGrayedOut(rect: CGRect) {
+ guard let context = UIGraphicsGetCurrentContext() else {
+ return
+ }
+
+ let bounds = self.bounds
+
+ let grayTranslucent = UIColor(red: 1, green: 1, blue: 1, alpha: 0.6)
+ let shadow = UIColor.black
+ let shadowOffset = CGSize(width: 0.1, height: -0.1)
+ let shadowBlurRadius: CGFloat = 2.5
+
+ let group = CGRect(x: bounds.minX + 3,
+ y: bounds.minY + 3,
+ width: bounds.width - 6,
+ height: bounds.height - 6)
+
+ let uncheckedOvalPath = UIBezierPath(ovalIn: CGRect(x: group.minX + floor(group.width * 0.00000 + 0.5),
+ y: group.minY + floor(group.height * 0.00000 + 0.5),
+ width: floor(group.width * 1.00000 + 0.5) - floor(group.width * 0.00000 + 0.5),
+ height: floor(group.height * 1.00000 + 0.5) - floor(group.height * 0.00000 + 0.5)))
+
+ context.saveGState()
+ context.setShadow(offset: shadowOffset, blur: shadowBlurRadius, color: shadow.cgColor)
+ grayTranslucent.setFill()
+ uncheckedOvalPath.fill()
+ context.restoreGState()
+
+ UIColor.white.setStroke()
+ uncheckedOvalPath.lineWidth = 1
+ uncheckedOvalPath.stroke()
+
+ let bezierPath = UIBezierPath()
+ bezierPath.move(to: CGPoint(x: group.minX + 0.27083 * group.width,
+ y: group.minY + 0.54167 * group.height))
+ bezierPath.addLine(to: CGPoint(x: group.minX + 0.41667 * group.width,
+ y: group.minY + 0.68750 * group.height))
+ bezierPath.addLine(to: CGPoint(x: group.minX + 0.75000 * group.width,
+ y: group.minY + 0.35417 * group.height))
+ bezierPath.lineCapStyle = CGLineCap.square
+
+ UIColor.white.setStroke()
+ bezierPath.lineWidth = 1.3
+ bezierPath.stroke()
+ }
+
+}
diff --git a/Pods/CheckMarkView/LICENSE b/Pods/CheckMarkView/LICENSE
new file mode 100644
index 0000000..6d5f3b3
--- /dev/null
+++ b/Pods/CheckMarkView/LICENSE
@@ -0,0 +1,22 @@
+The MIT License (MIT)
+
+Copyright (c) 2015 Maxim Bilan
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
diff --git a/Pods/CheckMarkView/README.md b/Pods/CheckMarkView/README.md
new file mode 100644
index 0000000..5ee88a6
--- /dev/null
+++ b/Pods/CheckMarkView/README.md
@@ -0,0 +1,49 @@
+# CheckMarkView
+
+[](http://cocoadocs.org/docsets/CheckMarkView)
+[](http://cocoadocs.org/docsets/CheckMarkView)
+[](http://cocoadocs.org/docsets/CheckMarkView)
+
+Unfortunately Apple doesn't provide accessory type property for UICollectionViewCell, such as for UITableViewCell, so I provide custom way to create checkmark.
+Just simple view which draws programmatically checkmark with some styles.
+
+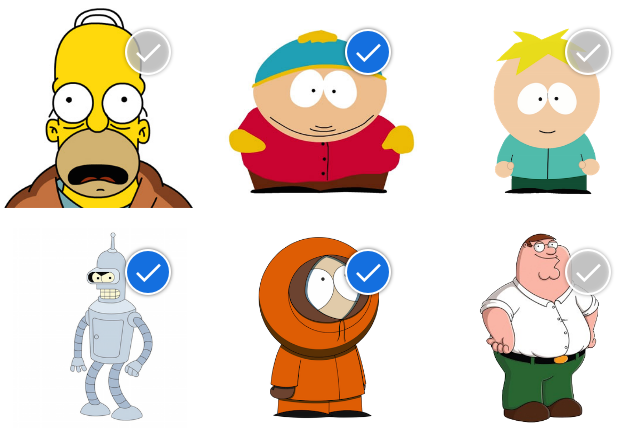
+
+# Installation
+
+CocoaPods:
+
+Swift 3.0:
+pod 'CheckMarkView', '~> 0.3.0'
+
+Swift 4.0:
+pod 'CheckMarkView', '~> 0.4.0'
+
+
+Manual:
+
+Copy CheckMarkView.swift to your project.
+
+
+## Using
+
+You can create from code or setup view in the Storyboard, XIB.
+
+
+let checkMarkView = CheckMarkView()
+
+
+For controlling you have checked property.
+And style property for unchecked view. There are some styles:
+
+
+enum CheckMarkStyle: Int {
+ case Nothing
+ case OpenCircle
+ case GrayedOut
+}
+
+
+## License
+
+CheckMarkView is available under the MIT license. See the LICENSE file for more info.
diff --git a/Pods/Local Podspecs/SwiftAssetsPickerController.podspec.json b/Pods/Local Podspecs/SwiftAssetsPickerController.podspec.json
new file mode 100644
index 0000000..72fba53
--- /dev/null
+++ b/Pods/Local Podspecs/SwiftAssetsPickerController.podspec.json
@@ -0,0 +1,31 @@
+{
+ "name": "SwiftAssetsPickerController",
+ "version": "0.4.0",
+ "summary": "Assets Picker Controller",
+ "description": "Simple assets picker controller based on iOS 8 Photos framework. Supports iCloud photos and videos. It's written in Swift.",
+ "homepage": "https://github.com/maximbilan/SwiftAssetsPickerController",
+ "license": {
+ "type": "MIT"
+ },
+ "authors": {
+ "Maxim Bilan": "maximb.mail@gmail.com"
+ },
+ "platforms": {
+ "ios": "8.0"
+ },
+ "source": {
+ "git": "https://github.com/maximbilan/SwiftAssetsPickerController.git",
+ "tag": "0.4.0"
+ },
+ "source_files": [
+ "Classes",
+ "SwiftAssetsPickerController/Sources/**/*.{swift}"
+ ],
+ "resources": "SwiftAssetsPickerController/Resources/*.*",
+ "requires_arc": true,
+ "dependencies": {
+ "CheckMarkView": [
+ "~> 0.4.2"
+ ]
+ }
+}
diff --git a/Pods/Manifest.lock b/Pods/Manifest.lock
new file mode 100644
index 0000000..d1cbda1
--- /dev/null
+++ b/Pods/Manifest.lock
@@ -0,0 +1,33 @@
+PODS:
+ - CheckMarkView (0.4.2)
+ - SwiftAssetsPickerController (0.4.0):
+ - CheckMarkView (~> 0.4.2)
+ - Vivid (0.9)
+
+DEPENDENCIES:
+ - SwiftAssetsPickerController (from `https://github.com/shu223/SwiftAssetsPickerController`, branch `temp/depth`)
+ - Vivid
+
+SPEC REPOS:
+ https://github.com/cocoapods/specs.git:
+ - CheckMarkView
+ - Vivid
+
+EXTERNAL SOURCES:
+ SwiftAssetsPickerController:
+ :branch: temp/depth
+ :git: https://github.com/shu223/SwiftAssetsPickerController
+
+CHECKOUT OPTIONS:
+ SwiftAssetsPickerController:
+ :commit: 08fc8f3482f143bce3b42a3cf7e7095c2c908377
+ :git: https://github.com/shu223/SwiftAssetsPickerController
+
+SPEC CHECKSUMS:
+ CheckMarkView: 1f911464cf552fa2dea5abc111894468f5f805b8
+ SwiftAssetsPickerController: e8a3b2aac2e57d054a89e91c1bcd244af8141376
+ Vivid: 0fde7409beac71224deb151dd78f98a5bb860497
+
+PODFILE CHECKSUM: ba6f97967707a961ba6dc83c6824573bfda89295
+
+COCOAPODS: 1.5.3
diff --git a/Pods/Pods.xcodeproj/project.pbxproj b/Pods/Pods.xcodeproj/project.pbxproj
new file mode 100644
index 0000000..c9a667b
--- /dev/null
+++ b/Pods/Pods.xcodeproj/project.pbxproj
@@ -0,0 +1,1217 @@
+// !$*UTF8*$!
+{
+ archiveVersion = 1;
+ classes = {
+ };
+ objectVersion = 50;
+ objects = {
+
+/* Begin PBXBuildFile section */
+ 0355812D88010D31EA944A6F9A0BD23D /* YUCIFilterPreviewGenerator.m in Sources */ = {isa = PBXBuildFile; fileRef = EE4EB7B7F3131461600CA97A09A2F195 /* YUCIFilterPreviewGenerator.m */; };
+ 045549B2370B900DF9C79DFC7EDB2F16 /* YUCIBlobsGenerator.m in Sources */ = {isa = PBXBuildFile; fileRef = A961086A2D853AAABAE0969AE4FF96F2 /* YUCIBlobsGenerator.m */; };
+ 0479FF77B6FDF5FE425E53F967D4CAA2 /* YUCIRGBToHSL.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = E97BAF7EA8198E2DE74E988CB392F2B1 /* YUCIRGBToHSL.cikernel */; };
+ 0761DD9351B67040466C822544A722B8 /* YUCICrossZoomTransition.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 8826F8507844D22EE964120F6EBCB32E /* YUCICrossZoomTransition.cikernel */; };
+ 0B758CA0028C2DBD873AFB539A71F966 /* YUCIColorLookup.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = A6F95414BBAC0D224A0AD5865E59CA2A /* YUCIColorLookup.cikernel */; };
+ 0C58CBE231244C713F17D2C9AD8B2BE8 /* YUCIFlashTransition.m in Sources */ = {isa = PBXBuildFile; fileRef = 10C277C48E7BF34DBBD3F973BDEB1B45 /* YUCIFlashTransition.m */; };
+ 164EDDE6A4F3ADEC3417700785D7A602 /* Foundation.framework in Frameworks */ = {isa = PBXBuildFile; fileRef = ED732F3295868CB9E54D81DD4BEA0416 /* Foundation.framework */; };
+ 1771A562ECE0978985CCF404E0A60266 /* YUCITriangularPixellate.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 94532DF461095742FAB154DBE3B9AF7F /* YUCITriangularPixellate.cikernel */; };
+ 17BCDEEA1E963D735018E6D26A6E02AC /* YUCIBlobsGenerator.h in Headers */ = {isa = PBXBuildFile; fileRef = 9802258AC56C9CBB5C03B64E391041B9 /* YUCIBlobsGenerator.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 17F5F64AA0D1EEA04A6264D7AD5F5704 /* YUCIFXAA.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 449B7B73E80D65D0C99D45E14A00882B /* YUCIFXAA.cikernel */; };
+ 1C7A917D84B93F188C4EABEB8F4E3672 /* YUCIColorLookup.m in Sources */ = {isa = PBXBuildFile; fileRef = 38F6B9D7C7C3EEC6E0405BF06731BAE1 /* YUCIColorLookup.m */; };
+ 1F191A2DF59A79B190FB67C5C8B35160 /* Base.lproj in Resources */ = {isa = PBXBuildFile; fileRef = 41B033F114CC6969815ADA124A416DC7 /* Base.lproj */; };
+ 1F2407534E73794E3B4CBEE906FEDD51 /* Foundation.framework in Frameworks */ = {isa = PBXBuildFile; fileRef = ED732F3295868CB9E54D81DD4BEA0416 /* Foundation.framework */; };
+ 2458DA96FF9D4605911108C6E895C79F /* YUCIFXAA.h in Headers */ = {isa = PBXBuildFile; fileRef = 244537953EDEEC1F93BD61195BD37B56 /* YUCIFXAA.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 26902991AEB59C46C0D22E26988FC018 /* SwiftAssetsPickerController-dummy.m in Sources */ = {isa = PBXBuildFile; fileRef = 9158281357180EE87C0417FA4C82E576 /* SwiftAssetsPickerController-dummy.m */; };
+ 26FA3BEE0508B6254AE2A0FF476C5673 /* YUCIRGBToneCurve.h in Headers */ = {isa = PBXBuildFile; fileRef = F8689E2EEFCC224A60430C86C342E810 /* YUCIRGBToneCurve.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 29765801E1ED611F918ED33F6AE1A614 /* SwiftAssetsPickerController-umbrella.h in Headers */ = {isa = PBXBuildFile; fileRef = 366F1A407304DF280930486E74F79339 /* SwiftAssetsPickerController-umbrella.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 2BCA4E5BFFD81A7E3DE3044016371E75 /* Pods-iOS-Depth-Sampler-dummy.m in Sources */ = {isa = PBXBuildFile; fileRef = EA16086EEFCA95F8DC1D5025114ACFEC /* Pods-iOS-Depth-Sampler-dummy.m */; };
+ 2EB1A09A7FFAEE79062C092227C61934 /* Foundation.framework in Frameworks */ = {isa = PBXBuildFile; fileRef = ED732F3295868CB9E54D81DD4BEA0416 /* Foundation.framework */; };
+ 32510A826DF6F93EC480DC86E57D7692 /* CheckMarkView.framework in Frameworks */ = {isa = PBXBuildFile; fileRef = C0D33443CE113045A81E2829995AB4EE /* CheckMarkView.framework */; };
+ 3B661BFC2BBA16C23A64BA240154154B /* panorama-icon.png in Resources */ = {isa = PBXBuildFile; fileRef = CAF95A24A88B23F107B067B47A98A110 /* panorama-icon.png */; };
+ 405ABAD50EFE43A95F4D7C539E83A484 /* CheckMarkView.swift in Sources */ = {isa = PBXBuildFile; fileRef = 2EC0AA1A310EAB3B011D89118B3AEE87 /* CheckMarkView.swift */; };
+ 4249F341CCA4BC81C0645952BE8C05BE /* Vivid-dummy.m in Sources */ = {isa = PBXBuildFile; fileRef = 668806A6D1A8B202899CC9C9DDDC8462 /* Vivid-dummy.m */; };
+ 46F5FEFE52A547C0BFFA5341E306D8E3 /* YUCIColorLookup.h in Headers */ = {isa = PBXBuildFile; fileRef = 9A138AA83F58162999640F5ECCCC0D0A /* YUCIColorLookup.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 47490C0B0C23C789C6C32044ECE538DF /* YUCIReflectedTile.h in Headers */ = {isa = PBXBuildFile; fileRef = D76DE83C0C697134099239F1AD32518D /* YUCIReflectedTile.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 477D8D88E31AFAFDC45CD5FD3A1A843D /* YUCIRGBToneCurve.m in Sources */ = {isa = PBXBuildFile; fileRef = F93A691AAB3331EDAFC7D71F83BF515C /* YUCIRGBToneCurve.m */; };
+ 4BBC648BF928D281CDC20D393CFBC4D8 /* YUCITriangularPixellate.h in Headers */ = {isa = PBXBuildFile; fileRef = 89888371A9263467382CFD11062BF139 /* YUCITriangularPixellate.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 4D2E78996E28048DB565D1128A8CD2D6 /* YUCICLAHE.m in Sources */ = {isa = PBXBuildFile; fileRef = 312B5F85831E0B0D432259F336C05F6C /* YUCICLAHE.m */; };
+ 4E6D0EF4079EF8FED003C886367300E1 /* YUCIBlobsGenerator.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 940EFBD431C3409783CD8BD103D67143 /* YUCIBlobsGenerator.cikernel */; };
+ 514B3824FA0FC3304145AA53227DBE4F /* YUCISkyGenerator.m in Sources */ = {isa = PBXBuildFile; fileRef = 9AE27C4A814DFED8B0024AA120434F75 /* YUCISkyGenerator.m */; };
+ 53E2600F9B0BF30A6F402C54A1E17D31 /* YUCISkyGenerator.h in Headers */ = {isa = PBXBuildFile; fileRef = 1BBC2B86EDC64F126D5754748473E312 /* YUCISkyGenerator.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 5A3E86B081429654AB36B138562DD948 /* YUCICLAHE.h in Headers */ = {isa = PBXBuildFile; fileRef = F4ACBD776FB5C68A387D96A3A3A2F6CA /* YUCICLAHE.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 5A98A69CC444C88FEB53EB8174161001 /* Vivid-umbrella.h in Headers */ = {isa = PBXBuildFile; fileRef = F97AEAEACB475786EC1D6F07931077F2 /* Vivid-umbrella.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 5D081D4E7AF4627B8FF83B96F05644FB /* YUCICrossZoomTransition.h in Headers */ = {isa = PBXBuildFile; fileRef = AC20559DECA12496794CFAF42AE0968D /* YUCICrossZoomTransition.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 5E750B65566190CF568D54B73DEEC8D7 /* YUCISurfaceBlur.m in Sources */ = {isa = PBXBuildFile; fileRef = 33E50F90084387CD9A885E6C5E4E57C1 /* YUCISurfaceBlur.m */; };
+ 6795A500C325EE3FCD8DCA18F736D0F1 /* Foundation.framework in Frameworks */ = {isa = PBXBuildFile; fileRef = ED732F3295868CB9E54D81DD4BEA0416 /* Foundation.framework */; };
+ 6A0E0EB8E99E50A0EA84D5107F6FB1A4 /* YUCIHistogramEqualization.h in Headers */ = {isa = PBXBuildFile; fileRef = 9635A6FA16F1069FD93439D613EC0E71 /* YUCIHistogramEqualization.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 70CB28C344834038845C13CF11D53C5D /* video-icon.png in Resources */ = {isa = PBXBuildFile; fileRef = DCC36527D5450E61F26EE75CC2AAEB65 /* video-icon.png */; };
+ 73F7B1278F66B4CCDE890C6ED684191C /* YUCIStarfieldGenerator.m in Sources */ = {isa = PBXBuildFile; fileRef = 642759CFB3814D884280D08012AE799E /* YUCIStarfieldGenerator.m */; };
+ 76A953617889CBDA32BBCDB6D824B006 /* YUCIBilateralFilter.m in Sources */ = {isa = PBXBuildFile; fileRef = A8B342C638D95F2CE5F30573DE9D9850 /* YUCIBilateralFilter.m */; };
+ 7806869FDC91A58AF4DC7843086E9406 /* YUCIUtilities.m in Sources */ = {isa = PBXBuildFile; fileRef = C8EEEC1D9C1FCB69CC5C314136998932 /* YUCIUtilities.m */; };
+ 7DC85D898923159D6F7D26D68B2ECD39 /* YUCIFilterPreviewGenerator.h in Headers */ = {isa = PBXBuildFile; fileRef = 0FE5AF3664593EEE00FEE2B9C0BB0F1B /* YUCIFilterPreviewGenerator.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 7F11D0062BE9AB63DCE199F4746D4021 /* AssetsPickerController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 79B1C3D16B0FCA67D0E65DF6831F5525 /* AssetsPickerController.swift */; };
+ 822345453F20B92B38CE0E2000DD0E5A /* YUCISurfaceBlur.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 9C036D6FC915976237D525778988312C /* YUCISurfaceBlur.cikernel */; };
+ 83DC64D64615AFF26113225B5591F9A3 /* YUCIFilmBurnTransition.m in Sources */ = {isa = PBXBuildFile; fileRef = 5E6A1B9155ECE0C195151F3A14CE8619 /* YUCIFilmBurnTransition.m */; };
+ 845229C9E99B5631F77A1ABCF1E6A8E9 /* CheckMarkView-umbrella.h in Headers */ = {isa = PBXBuildFile; fileRef = 93B14ACE50B7EB88BA120EDA0478E810 /* CheckMarkView-umbrella.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 88E3DB0292BD4D5D34E01623D853C4BE /* en.lproj in Resources */ = {isa = PBXBuildFile; fileRef = 63366F374B08EB9F3AEB9E9CB7CF400E /* en.lproj */; };
+ 8CE3921A3EF71DDF8B8494DDB8EBDB29 /* YUCIReflectedTileROICalculator.m in Sources */ = {isa = PBXBuildFile; fileRef = A39B015A87997300464F5816900164E3 /* YUCIReflectedTileROICalculator.m */; };
+ 95CEB3201F16C2ADD836697EBF3AE1A5 /* Pods-iOS-Depth-Sampler-umbrella.h in Headers */ = {isa = PBXBuildFile; fileRef = 7997301B37B2965B2E5E09DA15A7D245 /* Pods-iOS-Depth-Sampler-umbrella.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 98D1259F3D35FBA57A019FA388FD9E76 /* YUCIUtilities.h in Headers */ = {isa = PBXBuildFile; fileRef = E696645C46630CD5CB05D647F4E70845 /* YUCIUtilities.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ 9AD2B83C7EBAFFE603C91B3B51D30C61 /* YUCIColorLookupTableDefault.png in Resources */ = {isa = PBXBuildFile; fileRef = D7189BC6D41CD6B913C43250029A1980 /* YUCIColorLookupTableDefault.png */; };
+ A1C476D302EC446B3A184A914AF8F287 /* YUCICLAHE.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 4BB0D588EC40E67161708E0220961F03 /* YUCICLAHE.cikernel */; };
+ A20558191B7838AE17C82E6FEEB453FF /* YUCIHSLToRGB.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 17A0B067F5EC6A9EEC8995F6CA1AE623 /* YUCIHSLToRGB.cikernel */; };
+ A2E91F4151C435FAB58C2D3A885561DC /* YUCIStarfieldGenerator.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = A6DAC65535D5D28FF9BBFFF09E0AE979 /* YUCIStarfieldGenerator.cikernel */; };
+ A613029ACDA1625938F91201A29AFA4F /* YUCIFilterUtilities.m in Sources */ = {isa = PBXBuildFile; fileRef = 1AE9D43986A950FDEBBD6D4BEF2CA28F /* YUCIFilterUtilities.m */; };
+ A975451B6A268051262C6C1F5F87B9A1 /* YUCISurfaceBlur.h in Headers */ = {isa = PBXBuildFile; fileRef = A7933F95E98B31B4191737CACD7C6A52 /* YUCISurfaceBlur.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ AEA696946694746981FEE23D7BA47CA7 /* YUCIReflectedTile.m in Sources */ = {isa = PBXBuildFile; fileRef = D8F72D5652E81DAF98513144E0AF2C1B /* YUCIReflectedTile.m */; };
+ B36D734C8AACE4BAE4114665B79BDE63 /* YUCIFilmBurnTransition.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 415045B65D9AA5BC6040DE7D54098950 /* YUCIFilmBurnTransition.cikernel */; };
+ B77DA20ADBC535A42D6B7EC3258CDFCA /* YUCIReflectedTile.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = A7564A33FE162D13E9F2B44EF29ED8CB /* YUCIReflectedTile.cikernel */; };
+ B8D46C7BD96170222A6835C9E029F413 /* YUCICrossZoomTransition.m in Sources */ = {isa = PBXBuildFile; fileRef = 6B14B48D10991CCD3A81E204A476123D /* YUCICrossZoomTransition.m */; };
+ BA61546A10659B82B1C67DD974E479BF /* YUCIBilateralFilter.h in Headers */ = {isa = PBXBuildFile; fileRef = 589A54E7266DC122062700F2FE6782B2 /* YUCIBilateralFilter.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ C1FA563F2FB5E5B88F22EFEC020EE904 /* YUCIReflectedTileROICalculator.h in Headers */ = {isa = PBXBuildFile; fileRef = 770CF9682893A01D6DEEAFF5FE9112CA /* YUCIReflectedTileROICalculator.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ C35A633C028E7C7897B2BE7F4CA589B7 /* YUCIFilmBurnTransition.h in Headers */ = {isa = PBXBuildFile; fileRef = 0684A61C5B4F378A427692014BAD98B1 /* YUCIFilmBurnTransition.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ CA227A879FE95A2A9ECB2F3F92521668 /* YUCISkyGenerator.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 20F4742A040A965108614F171DBE6DDE /* YUCISkyGenerator.cikernel */; };
+ CE1110E7CDDB94CA51965F55B053ECD8 /* YUCIFilterConstructor.h in Headers */ = {isa = PBXBuildFile; fileRef = 02D57BE434D8A681FF60CFA7519BB16C /* YUCIFilterConstructor.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ CF61B5A2CB426462FA859C8BEB98B8E7 /* YUCIFilterUtilities.h in Headers */ = {isa = PBXBuildFile; fileRef = CDF8416C6CF98EC3B551FAAC7AFDCFE2 /* YUCIFilterUtilities.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ D18D393EFE3195C164EE5F7744273CD7 /* YUCIFXAA.m in Sources */ = {isa = PBXBuildFile; fileRef = 7AA3A2C5AD81375442D5A6EA842A91C9 /* YUCIFXAA.m */; };
+ D7E9D928E7FA4B35A2F720D7FEF061B8 /* YUCIFlashTransition.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 58D2911208E3CBDDFC3E936EEEC515A7 /* YUCIFlashTransition.cikernel */; };
+ D8C982C0E7054E1A42DC2230E557F9C9 /* YUCIFilterConstructor.m in Sources */ = {isa = PBXBuildFile; fileRef = 1D280949BCE17802D6FCCB182560ABB4 /* YUCIFilterConstructor.m */; };
+ D9B210B5D427474F0F2BFDC1A10F0235 /* YUCIFlashTransition.h in Headers */ = {isa = PBXBuildFile; fileRef = CD52B1570045FFF28F1B9742D8115F03 /* YUCIFlashTransition.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ DA486502D6F21CB13761315972D9B131 /* YUCIRGBToneCurve.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = D6363F052E9DF7DE3C37EABA06EF62E9 /* YUCIRGBToneCurve.cikernel */; };
+ DB36FC5CAD296FEA5F9A80B9933A25CA /* YUCIStarfieldGenerator.h in Headers */ = {isa = PBXBuildFile; fileRef = 82608716946753A1F5CA63E265D2309A /* YUCIStarfieldGenerator.h */; settings = {ATTRIBUTES = (Public, ); }; };
+ DF3B29DFEB7E2ABE269E18B306BA40B5 /* AssetsPickerGridController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 5D700CF5170120B5EB2BA6DBFB7A121C /* AssetsPickerGridController.swift */; };
+ E69128BB562E4EC3954B3E80E80E0794 /* YUCIBilateralFilter.cikernel in Resources */ = {isa = PBXBuildFile; fileRef = 5CC3CBDC4476E9C005BA695876A481C6 /* YUCIBilateralFilter.cikernel */; };
+ EEEDB220E8731680DA51EC442E1CA617 /* CheckMarkView-dummy.m in Sources */ = {isa = PBXBuildFile; fileRef = 0885A8FA5F9CA0C27887355B2751C12A /* CheckMarkView-dummy.m */; };
+ F3DE85D541B7FC01AC2859D28AA91EE0 /* YUCITriangularPixellate.m in Sources */ = {isa = PBXBuildFile; fileRef = 7606F8EBFF6EC7590E53ACACC7A38777 /* YUCITriangularPixellate.m */; };
+ F7CE4A0869F9C98B9223B6481DFEB13C /* YUCIHistogramEqualization.m in Sources */ = {isa = PBXBuildFile; fileRef = 1AE20B508D248C0F62A5A0E26D649061 /* YUCIHistogramEqualization.m */; };
+ F8A3A5E5A8B2A125E3B7A46BC4EE4374 /* timelapse-icon.png in Resources */ = {isa = PBXBuildFile; fileRef = E58F4583C97CF9AA2CBAE0FC3B7D36F2 /* timelapse-icon.png */; };
+/* End PBXBuildFile section */
+
+/* Begin PBXContainerItemProxy section */
+ 05FBE67461EE27C3E255D8E5B5D068F7 /* PBXContainerItemProxy */ = {
+ isa = PBXContainerItemProxy;
+ containerPortal = D41D8CD98F00B204E9800998ECF8427E /* Project object */;
+ proxyType = 1;
+ remoteGlobalIDString = C6446C484B26560A27A42983580052E9;
+ remoteInfo = CheckMarkView;
+ };
+ 08B0B3F88FF77C920651EE528714ED7F /* PBXContainerItemProxy */ = {
+ isa = PBXContainerItemProxy;
+ containerPortal = D41D8CD98F00B204E9800998ECF8427E /* Project object */;
+ proxyType = 1;
+ remoteGlobalIDString = 73C842E482B6B3C2CA32BFD8F26FF4F6;
+ remoteInfo = Vivid;
+ };
+ 2DA5FACCE9E3A139A3A97A714CFCA799 /* PBXContainerItemProxy */ = {
+ isa = PBXContainerItemProxy;
+ containerPortal = D41D8CD98F00B204E9800998ECF8427E /* Project object */;
+ proxyType = 1;
+ remoteGlobalIDString = C6446C484B26560A27A42983580052E9;
+ remoteInfo = CheckMarkView;
+ };
+ 824D927267E5BA2812677F0CC30E7C2F /* PBXContainerItemProxy */ = {
+ isa = PBXContainerItemProxy;
+ containerPortal = D41D8CD98F00B204E9800998ECF8427E /* Project object */;
+ proxyType = 1;
+ remoteGlobalIDString = FE4A9D1B324616BC82D0D8D73BE4D382;
+ remoteInfo = SwiftAssetsPickerController;
+ };
+/* End PBXContainerItemProxy section */
+
+/* Begin PBXFileReference section */
+ 02D57BE434D8A681FF60CFA7519BB16C /* YUCIFilterConstructor.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIFilterConstructor.h; path = Sources/YUCIFilterConstructor.h; sourceTree = ""; };
+ 0684A61C5B4F378A427692014BAD98B1 /* YUCIFilmBurnTransition.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIFilmBurnTransition.h; path = Sources/YUCIFilmBurnTransition.h; sourceTree = ""; };
+ 0885A8FA5F9CA0C27887355B2751C12A /* CheckMarkView-dummy.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; path = "CheckMarkView-dummy.m"; sourceTree = ""; };
+ 0E118BB3EEC8B7F8DF2D68409B2284BE /* SwiftAssetsPickerController.framework */ = {isa = PBXFileReference; explicitFileType = wrapper.framework; includeInIndex = 0; name = SwiftAssetsPickerController.framework; path = SwiftAssetsPickerController.framework; sourceTree = BUILT_PRODUCTS_DIR; };
+ 0FE5AF3664593EEE00FEE2B9C0BB0F1B /* YUCIFilterPreviewGenerator.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIFilterPreviewGenerator.h; path = Sources/YUCIFilterPreviewGenerator.h; sourceTree = ""; };
+ 10C277C48E7BF34DBBD3F973BDEB1B45 /* YUCIFlashTransition.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIFlashTransition.m; path = Sources/YUCIFlashTransition.m; sourceTree = ""; };
+ 17A0B067F5EC6A9EEC8995F6CA1AE623 /* YUCIHSLToRGB.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIHSLToRGB.cikernel; path = Sources/YUCIHSLToRGB.cikernel; sourceTree = ""; };
+ 19CD51DFB0B70AE9BBB2568AAD47895D /* Info.plist */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = ""; };
+ 1AE20B508D248C0F62A5A0E26D649061 /* YUCIHistogramEqualization.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIHistogramEqualization.m; path = Sources/YUCIHistogramEqualization.m; sourceTree = ""; };
+ 1AE9D43986A950FDEBBD6D4BEF2CA28F /* YUCIFilterUtilities.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIFilterUtilities.m; path = Sources/YUCIFilterUtilities.m; sourceTree = ""; };
+ 1BBC2B86EDC64F126D5754748473E312 /* YUCISkyGenerator.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCISkyGenerator.h; path = Sources/YUCISkyGenerator.h; sourceTree = ""; };
+ 1D280949BCE17802D6FCCB182560ABB4 /* YUCIFilterConstructor.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIFilterConstructor.m; path = Sources/YUCIFilterConstructor.m; sourceTree = ""; };
+ 20F4742A040A965108614F171DBE6DDE /* YUCISkyGenerator.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCISkyGenerator.cikernel; path = Sources/YUCISkyGenerator.cikernel; sourceTree = ""; };
+ 244537953EDEEC1F93BD61195BD37B56 /* YUCIFXAA.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIFXAA.h; path = Sources/YUCIFXAA.h; sourceTree = ""; };
+ 2AF0E47048AC80725F4AE0120B763D31 /* CheckMarkView-prefix.pch */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "CheckMarkView-prefix.pch"; sourceTree = ""; };
+ 2C86F32573F3F6AE2D95E6CE94B326DA /* Pods-iOS-Depth-Sampler-resources.sh */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.script.sh; path = "Pods-iOS-Depth-Sampler-resources.sh"; sourceTree = ""; };
+ 2EC0AA1A310EAB3B011D89118B3AEE87 /* CheckMarkView.swift */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.swift; name = CheckMarkView.swift; path = CheckMarkView/Sources/CheckMarkView.swift; sourceTree = ""; };
+ 312B5F85831E0B0D432259F336C05F6C /* YUCICLAHE.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCICLAHE.m; path = Sources/YUCICLAHE.m; sourceTree = ""; };
+ 33E50F90084387CD9A885E6C5E4E57C1 /* YUCISurfaceBlur.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCISurfaceBlur.m; path = Sources/YUCISurfaceBlur.m; sourceTree = ""; };
+ 366F1A407304DF280930486E74F79339 /* SwiftAssetsPickerController-umbrella.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "SwiftAssetsPickerController-umbrella.h"; sourceTree = ""; };
+ 385C4B854706867C6A919A9656B3A195 /* Vivid.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; path = Vivid.xcconfig; sourceTree = ""; };
+ 38F6B9D7C7C3EEC6E0405BF06731BAE1 /* YUCIColorLookup.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIColorLookup.m; path = Sources/YUCIColorLookup.m; sourceTree = ""; };
+ 415045B65D9AA5BC6040DE7D54098950 /* YUCIFilmBurnTransition.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIFilmBurnTransition.cikernel; path = Sources/YUCIFilmBurnTransition.cikernel; sourceTree = ""; };
+ 41B033F114CC6969815ADA124A416DC7 /* Base.lproj */ = {isa = PBXFileReference; includeInIndex = 1; name = Base.lproj; path = SwiftAssetsPickerController/Resources/Base.lproj; sourceTree = ""; };
+ 449B7B73E80D65D0C99D45E14A00882B /* YUCIFXAA.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIFXAA.cikernel; path = Sources/YUCIFXAA.cikernel; sourceTree = ""; };
+ 4BB0D588EC40E67161708E0220961F03 /* YUCICLAHE.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCICLAHE.cikernel; path = Sources/YUCICLAHE.cikernel; sourceTree = ""; };
+ 589A54E7266DC122062700F2FE6782B2 /* YUCIBilateralFilter.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIBilateralFilter.h; path = Sources/YUCIBilateralFilter.h; sourceTree = ""; };
+ 58D2911208E3CBDDFC3E936EEEC515A7 /* YUCIFlashTransition.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIFlashTransition.cikernel; path = Sources/YUCIFlashTransition.cikernel; sourceTree = ""; };
+ 5988358DFE4EA6054E360BE5A29D6EF7 /* Info.plist */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = ""; };
+ 5CC3CBDC4476E9C005BA695876A481C6 /* YUCIBilateralFilter.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIBilateralFilter.cikernel; path = Sources/YUCIBilateralFilter.cikernel; sourceTree = ""; };
+ 5D700CF5170120B5EB2BA6DBFB7A121C /* AssetsPickerGridController.swift */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.swift; name = AssetsPickerGridController.swift; path = SwiftAssetsPickerController/Sources/AssetsPickerGridController.swift; sourceTree = ""; };
+ 5E6A1B9155ECE0C195151F3A14CE8619 /* YUCIFilmBurnTransition.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIFilmBurnTransition.m; path = Sources/YUCIFilmBurnTransition.m; sourceTree = ""; };
+ 6104F06D28DD44441A578F94815C2B84 /* Info.plist */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = ""; };
+ 63366F374B08EB9F3AEB9E9CB7CF400E /* en.lproj */ = {isa = PBXFileReference; includeInIndex = 1; name = en.lproj; path = SwiftAssetsPickerController/Resources/en.lproj; sourceTree = ""; };
+ 642759CFB3814D884280D08012AE799E /* YUCIStarfieldGenerator.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIStarfieldGenerator.m; path = Sources/YUCIStarfieldGenerator.m; sourceTree = ""; };
+ 668806A6D1A8B202899CC9C9DDDC8462 /* Vivid-dummy.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; path = "Vivid-dummy.m"; sourceTree = ""; };
+ 6B14B48D10991CCD3A81E204A476123D /* YUCICrossZoomTransition.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCICrossZoomTransition.m; path = Sources/YUCICrossZoomTransition.m; sourceTree = ""; };
+ 7606F8EBFF6EC7590E53ACACC7A38777 /* YUCITriangularPixellate.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCITriangularPixellate.m; path = Sources/YUCITriangularPixellate.m; sourceTree = ""; };
+ 770CF9682893A01D6DEEAFF5FE9112CA /* YUCIReflectedTileROICalculator.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIReflectedTileROICalculator.h; path = Sources/YUCIReflectedTileROICalculator.h; sourceTree = ""; };
+ 7997301B37B2965B2E5E09DA15A7D245 /* Pods-iOS-Depth-Sampler-umbrella.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "Pods-iOS-Depth-Sampler-umbrella.h"; sourceTree = ""; };
+ 79B1C3D16B0FCA67D0E65DF6831F5525 /* AssetsPickerController.swift */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.swift; name = AssetsPickerController.swift; path = SwiftAssetsPickerController/Sources/AssetsPickerController.swift; sourceTree = ""; };
+ 7AA3A2C5AD81375442D5A6EA842A91C9 /* YUCIFXAA.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIFXAA.m; path = Sources/YUCIFXAA.m; sourceTree = ""; };
+ 7AE89405C7A239E69E2FAE62D135EB7E /* Vivid.framework */ = {isa = PBXFileReference; explicitFileType = wrapper.framework; includeInIndex = 0; name = Vivid.framework; path = Vivid.framework; sourceTree = BUILT_PRODUCTS_DIR; };
+ 82608716946753A1F5CA63E265D2309A /* YUCIStarfieldGenerator.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIStarfieldGenerator.h; path = Sources/YUCIStarfieldGenerator.h; sourceTree = ""; };
+ 85F7AA12070D33E641E4042EC2BAE8FD /* Pods-iOS-Depth-Sampler.debug.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; path = "Pods-iOS-Depth-Sampler.debug.xcconfig"; sourceTree = ""; };
+ 86C1AC38A9095AC21B04C96840448DE3 /* Info.plist */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = ""; };
+ 8826F8507844D22EE964120F6EBCB32E /* YUCICrossZoomTransition.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCICrossZoomTransition.cikernel; path = Sources/YUCICrossZoomTransition.cikernel; sourceTree = ""; };
+ 89888371A9263467382CFD11062BF139 /* YUCITriangularPixellate.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCITriangularPixellate.h; path = Sources/YUCITriangularPixellate.h; sourceTree = ""; };
+ 9158281357180EE87C0417FA4C82E576 /* SwiftAssetsPickerController-dummy.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; path = "SwiftAssetsPickerController-dummy.m"; sourceTree = ""; };
+ 93A4A3777CF96A4AAC1D13BA6DCCEA73 /* Podfile */ = {isa = PBXFileReference; explicitFileType = text.script.ruby; includeInIndex = 1; lastKnownFileType = text; name = Podfile; path = ../Podfile; sourceTree = SOURCE_ROOT; xcLanguageSpecificationIdentifier = xcode.lang.ruby; };
+ 93B14ACE50B7EB88BA120EDA0478E810 /* CheckMarkView-umbrella.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "CheckMarkView-umbrella.h"; sourceTree = ""; };
+ 940EFBD431C3409783CD8BD103D67143 /* YUCIBlobsGenerator.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIBlobsGenerator.cikernel; path = Sources/YUCIBlobsGenerator.cikernel; sourceTree = ""; };
+ 94532DF461095742FAB154DBE3B9AF7F /* YUCITriangularPixellate.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCITriangularPixellate.cikernel; path = Sources/YUCITriangularPixellate.cikernel; sourceTree = ""; };
+ 9635A6FA16F1069FD93439D613EC0E71 /* YUCIHistogramEqualization.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIHistogramEqualization.h; path = Sources/YUCIHistogramEqualization.h; sourceTree = ""; };
+ 9802258AC56C9CBB5C03B64E391041B9 /* YUCIBlobsGenerator.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIBlobsGenerator.h; path = Sources/YUCIBlobsGenerator.h; sourceTree = ""; };
+ 9A138AA83F58162999640F5ECCCC0D0A /* YUCIColorLookup.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIColorLookup.h; path = Sources/YUCIColorLookup.h; sourceTree = ""; };
+ 9AE27C4A814DFED8B0024AA120434F75 /* YUCISkyGenerator.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCISkyGenerator.m; path = Sources/YUCISkyGenerator.m; sourceTree = ""; };
+ 9AF3D626E5DF8DB7996AA1967A9FEED6 /* SwiftAssetsPickerController.modulemap */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.module; path = SwiftAssetsPickerController.modulemap; sourceTree = ""; };
+ 9C036D6FC915976237D525778988312C /* YUCISurfaceBlur.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCISurfaceBlur.cikernel; path = Sources/YUCISurfaceBlur.cikernel; sourceTree = ""; };
+ A39B015A87997300464F5816900164E3 /* YUCIReflectedTileROICalculator.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIReflectedTileROICalculator.m; path = Sources/YUCIReflectedTileROICalculator.m; sourceTree = ""; };
+ A54AE47AD786377058FCD36676B805F1 /* SwiftAssetsPickerController-prefix.pch */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "SwiftAssetsPickerController-prefix.pch"; sourceTree = ""; };
+ A6DAC65535D5D28FF9BBFFF09E0AE979 /* YUCIStarfieldGenerator.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIStarfieldGenerator.cikernel; path = Sources/YUCIStarfieldGenerator.cikernel; sourceTree = ""; };
+ A6F95414BBAC0D224A0AD5865E59CA2A /* YUCIColorLookup.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIColorLookup.cikernel; path = Sources/YUCIColorLookup.cikernel; sourceTree = ""; };
+ A7564A33FE162D13E9F2B44EF29ED8CB /* YUCIReflectedTile.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIReflectedTile.cikernel; path = Sources/YUCIReflectedTile.cikernel; sourceTree = ""; };
+ A7933F95E98B31B4191737CACD7C6A52 /* YUCISurfaceBlur.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCISurfaceBlur.h; path = Sources/YUCISurfaceBlur.h; sourceTree = ""; };
+ A8B342C638D95F2CE5F30573DE9D9850 /* YUCIBilateralFilter.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIBilateralFilter.m; path = Sources/YUCIBilateralFilter.m; sourceTree = ""; };
+ A961086A2D853AAABAE0969AE4FF96F2 /* YUCIBlobsGenerator.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIBlobsGenerator.m; path = Sources/YUCIBlobsGenerator.m; sourceTree = ""; };
+ AC20559DECA12496794CFAF42AE0968D /* YUCICrossZoomTransition.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCICrossZoomTransition.h; path = Sources/YUCICrossZoomTransition.h; sourceTree = ""; };
+ B13645DF60C7B8FFBD233CE0ECE918F4 /* Pods-iOS-Depth-Sampler-acknowledgements.markdown */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text; path = "Pods-iOS-Depth-Sampler-acknowledgements.markdown"; sourceTree = ""; };
+ B783A94CD15B197C81B14E9B682F7B01 /* Pods-iOS-Depth-Sampler.modulemap */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.module; path = "Pods-iOS-Depth-Sampler.modulemap"; sourceTree = ""; };
+ BA8D1258EA69F184F48352D8F2B1D305 /* SwiftAssetsPickerController.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; path = SwiftAssetsPickerController.xcconfig; sourceTree = ""; };
+ C0D33443CE113045A81E2829995AB4EE /* CheckMarkView.framework */ = {isa = PBXFileReference; explicitFileType = wrapper.framework; includeInIndex = 0; path = CheckMarkView.framework; sourceTree = BUILT_PRODUCTS_DIR; };
+ C8EEEC1D9C1FCB69CC5C314136998932 /* YUCIUtilities.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIUtilities.m; path = Sources/YUCIUtilities.m; sourceTree = ""; };
+ CAF95A24A88B23F107B067B47A98A110 /* panorama-icon.png */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = image.png; name = "panorama-icon.png"; path = "SwiftAssetsPickerController/Resources/panorama-icon.png"; sourceTree = ""; };
+ CB9F548A7C4201899FF508311CE2B101 /* Pods-iOS-Depth-Sampler-acknowledgements.plist */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.plist.xml; path = "Pods-iOS-Depth-Sampler-acknowledgements.plist"; sourceTree = ""; };
+ CCB23A7F6876E95245FAA85FEECC109E /* Pods-iOS-Depth-Sampler.release.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; path = "Pods-iOS-Depth-Sampler.release.xcconfig"; sourceTree = ""; };
+ CD52B1570045FFF28F1B9742D8115F03 /* YUCIFlashTransition.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIFlashTransition.h; path = Sources/YUCIFlashTransition.h; sourceTree = ""; };
+ CDF8416C6CF98EC3B551FAAC7AFDCFE2 /* YUCIFilterUtilities.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIFilterUtilities.h; path = Sources/YUCIFilterUtilities.h; sourceTree = ""; };
+ D3E28829404FDB70E1D686A92FE0DC99 /* CheckMarkView.framework */ = {isa = PBXFileReference; explicitFileType = wrapper.framework; includeInIndex = 0; name = CheckMarkView.framework; path = CheckMarkView.framework; sourceTree = BUILT_PRODUCTS_DIR; };
+ D6363F052E9DF7DE3C37EABA06EF62E9 /* YUCIRGBToneCurve.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIRGBToneCurve.cikernel; path = Sources/YUCIRGBToneCurve.cikernel; sourceTree = ""; };
+ D7189BC6D41CD6B913C43250029A1980 /* YUCIColorLookupTableDefault.png */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = image.png; name = YUCIColorLookupTableDefault.png; path = Sources/YUCIColorLookupTableDefault.png; sourceTree = ""; };
+ D76DE83C0C697134099239F1AD32518D /* YUCIReflectedTile.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIReflectedTile.h; path = Sources/YUCIReflectedTile.h; sourceTree = ""; };
+ D8F72D5652E81DAF98513144E0AF2C1B /* YUCIReflectedTile.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIReflectedTile.m; path = Sources/YUCIReflectedTile.m; sourceTree = ""; };
+ D95E86CEBA5D64C4F82AB5E249120223 /* Pods-iOS-Depth-Sampler-frameworks.sh */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.script.sh; path = "Pods-iOS-Depth-Sampler-frameworks.sh"; sourceTree = ""; };
+ DC9ABFE7072488A803F34370C879181E /* Vivid.modulemap */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.module; path = Vivid.modulemap; sourceTree = ""; };
+ DCC36527D5450E61F26EE75CC2AAEB65 /* video-icon.png */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = image.png; name = "video-icon.png"; path = "SwiftAssetsPickerController/Resources/video-icon.png"; sourceTree = ""; };
+ DF3C6EF09C7770D88173CFA66BDF1A64 /* CheckMarkView.modulemap */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.module; path = CheckMarkView.modulemap; sourceTree = ""; };
+ DFDB68A78607519C63571C68344A9044 /* Pods_iOS_Depth_Sampler.framework */ = {isa = PBXFileReference; explicitFileType = wrapper.framework; includeInIndex = 0; name = Pods_iOS_Depth_Sampler.framework; path = "Pods-iOS-Depth-Sampler.framework"; sourceTree = BUILT_PRODUCTS_DIR; };
+ E58F4583C97CF9AA2CBAE0FC3B7D36F2 /* timelapse-icon.png */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = image.png; name = "timelapse-icon.png"; path = "SwiftAssetsPickerController/Resources/timelapse-icon.png"; sourceTree = ""; };
+ E696645C46630CD5CB05D647F4E70845 /* YUCIUtilities.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIUtilities.h; path = Sources/YUCIUtilities.h; sourceTree = ""; };
+ E919F2773E4565BE5DB4338B5EB193FC /* Vivid-prefix.pch */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "Vivid-prefix.pch"; sourceTree = ""; };
+ E97BAF7EA8198E2DE74E988CB392F2B1 /* YUCIRGBToHSL.cikernel */ = {isa = PBXFileReference; includeInIndex = 1; name = YUCIRGBToHSL.cikernel; path = Sources/YUCIRGBToHSL.cikernel; sourceTree = ""; };
+ EA16086EEFCA95F8DC1D5025114ACFEC /* Pods-iOS-Depth-Sampler-dummy.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; path = "Pods-iOS-Depth-Sampler-dummy.m"; sourceTree = ""; };
+ ED732F3295868CB9E54D81DD4BEA0416 /* Foundation.framework */ = {isa = PBXFileReference; lastKnownFileType = wrapper.framework; name = Foundation.framework; path = Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS10.3.sdk/System/Library/Frameworks/Foundation.framework; sourceTree = DEVELOPER_DIR; };
+ EE4EB7B7F3131461600CA97A09A2F195 /* YUCIFilterPreviewGenerator.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIFilterPreviewGenerator.m; path = Sources/YUCIFilterPreviewGenerator.m; sourceTree = ""; };
+ F4ACBD776FB5C68A387D96A3A3A2F6CA /* YUCICLAHE.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCICLAHE.h; path = Sources/YUCICLAHE.h; sourceTree = ""; };
+ F7536114BC001F60ECFF230D1F13B1B3 /* CheckMarkView.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; path = CheckMarkView.xcconfig; sourceTree = ""; };
+ F8689E2EEFCC224A60430C86C342E810 /* YUCIRGBToneCurve.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; name = YUCIRGBToneCurve.h; path = Sources/YUCIRGBToneCurve.h; sourceTree = ""; };
+ F93A691AAB3331EDAFC7D71F83BF515C /* YUCIRGBToneCurve.m */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.objc; name = YUCIRGBToneCurve.m; path = Sources/YUCIRGBToneCurve.m; sourceTree = ""; };
+ F97AEAEACB475786EC1D6F07931077F2 /* Vivid-umbrella.h */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = sourcecode.c.h; path = "Vivid-umbrella.h"; sourceTree = ""; };
+/* End PBXFileReference section */
+
+/* Begin PBXFrameworksBuildPhase section */
+ 08A96BBEB293CEA462DFE2FF4FA0889A /* Frameworks */ = {
+ isa = PBXFrameworksBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 164EDDE6A4F3ADEC3417700785D7A602 /* Foundation.framework in Frameworks */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 773512B4CB9D2728B3638F75BCED6314 /* Frameworks */ = {
+ isa = PBXFrameworksBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 1F2407534E73794E3B4CBEE906FEDD51 /* Foundation.framework in Frameworks */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 9073D1FA65879FCF4AAB1C6324857281 /* Frameworks */ = {
+ isa = PBXFrameworksBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 6795A500C325EE3FCD8DCA18F736D0F1 /* Foundation.framework in Frameworks */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ D83877FFF1E5CCD34DB8270AA4DFE80A /* Frameworks */ = {
+ isa = PBXFrameworksBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 32510A826DF6F93EC480DC86E57D7692 /* CheckMarkView.framework in Frameworks */,
+ 2EB1A09A7FFAEE79062C092227C61934 /* Foundation.framework in Frameworks */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXFrameworksBuildPhase section */
+
+/* Begin PBXGroup section */
+ 4234A69510CF6AC3FB616D2C8278F993 /* Products */ = {
+ isa = PBXGroup;
+ children = (
+ D3E28829404FDB70E1D686A92FE0DC99 /* CheckMarkView.framework */,
+ DFDB68A78607519C63571C68344A9044 /* Pods_iOS_Depth_Sampler.framework */,
+ 0E118BB3EEC8B7F8DF2D68409B2284BE /* SwiftAssetsPickerController.framework */,
+ 7AE89405C7A239E69E2FAE62D135EB7E /* Vivid.framework */,
+ );
+ name = Products;
+ sourceTree = "";
+ };
+ 4E19CDE254C3E58916CA30157DEDE5BB /* iOS */ = {
+ isa = PBXGroup;
+ children = (
+ ED732F3295868CB9E54D81DD4BEA0416 /* Foundation.framework */,
+ );
+ name = iOS;
+ sourceTree = "";
+ };
+ 5AF23CAE32B0C6D42B2B3015A0027837 /* CheckMarkView */ = {
+ isa = PBXGroup;
+ children = (
+ 2EC0AA1A310EAB3B011D89118B3AEE87 /* CheckMarkView.swift */,
+ 6C7DFC688DCE66967234E6083DD4B918 /* Support Files */,
+ );
+ name = CheckMarkView;
+ path = CheckMarkView;
+ sourceTree = "";
+ };
+ 5C4DF8B1C8192444E4FDB1A7365641AE /* Support Files */ = {
+ isa = PBXGroup;
+ children = (
+ 86C1AC38A9095AC21B04C96840448DE3 /* Info.plist */,
+ DC9ABFE7072488A803F34370C879181E /* Vivid.modulemap */,
+ 385C4B854706867C6A919A9656B3A195 /* Vivid.xcconfig */,
+ 668806A6D1A8B202899CC9C9DDDC8462 /* Vivid-dummy.m */,
+ E919F2773E4565BE5DB4338B5EB193FC /* Vivid-prefix.pch */,
+ F97AEAEACB475786EC1D6F07931077F2 /* Vivid-umbrella.h */,
+ );
+ name = "Support Files";
+ path = "../Target Support Files/Vivid";
+ sourceTree = "";
+ };
+ 6B333FCF48F08B8F7AE7FC988B73B111 /* Targets Support Files */ = {
+ isa = PBXGroup;
+ children = (
+ B5E4F35A13079F47B0DB9B56C21AA9DA /* Pods-iOS-Depth-Sampler */,
+ );
+ name = "Targets Support Files";
+ sourceTree = "";
+ };
+ 6C7DFC688DCE66967234E6083DD4B918 /* Support Files */ = {
+ isa = PBXGroup;
+ children = (
+ DF3C6EF09C7770D88173CFA66BDF1A64 /* CheckMarkView.modulemap */,
+ F7536114BC001F60ECFF230D1F13B1B3 /* CheckMarkView.xcconfig */,
+ 0885A8FA5F9CA0C27887355B2751C12A /* CheckMarkView-dummy.m */,
+ 2AF0E47048AC80725F4AE0120B763D31 /* CheckMarkView-prefix.pch */,
+ 93B14ACE50B7EB88BA120EDA0478E810 /* CheckMarkView-umbrella.h */,
+ 5988358DFE4EA6054E360BE5A29D6EF7 /* Info.plist */,
+ );
+ name = "Support Files";
+ path = "../Target Support Files/CheckMarkView";
+ sourceTree = "";
+ };
+ 7371D5E1290B9586B2A167512DF59FC3 /* Support Files */ = {
+ isa = PBXGroup;
+ children = (
+ 6104F06D28DD44441A578F94815C2B84 /* Info.plist */,
+ 9AF3D626E5DF8DB7996AA1967A9FEED6 /* SwiftAssetsPickerController.modulemap */,
+ BA8D1258EA69F184F48352D8F2B1D305 /* SwiftAssetsPickerController.xcconfig */,
+ 9158281357180EE87C0417FA4C82E576 /* SwiftAssetsPickerController-dummy.m */,
+ A54AE47AD786377058FCD36676B805F1 /* SwiftAssetsPickerController-prefix.pch */,
+ 366F1A407304DF280930486E74F79339 /* SwiftAssetsPickerController-umbrella.h */,
+ );
+ name = "Support Files";
+ path = "../Target Support Files/SwiftAssetsPickerController";
+ sourceTree = "";
+ };
+ 7DB346D0F39D3F0E887471402A8071AB = {
+ isa = PBXGroup;
+ children = (
+ 93A4A3777CF96A4AAC1D13BA6DCCEA73 /* Podfile */,
+ 9457170DA9D3F951DFAFAFAE051557E3 /* Frameworks */,
+ ACDA2798CCA9ED23D5122BB25A0F76E8 /* Pods */,
+ 4234A69510CF6AC3FB616D2C8278F993 /* Products */,
+ 6B333FCF48F08B8F7AE7FC988B73B111 /* Targets Support Files */,
+ );
+ sourceTree = "";
+ };
+ 8BE05D1D2E122F4E73173DAE96E779EB /* SwiftAssetsPickerController */ = {
+ isa = PBXGroup;
+ children = (
+ 79B1C3D16B0FCA67D0E65DF6831F5525 /* AssetsPickerController.swift */,
+ 5D700CF5170120B5EB2BA6DBFB7A121C /* AssetsPickerGridController.swift */,
+ C67E0D64482D0C0288B4D3A23EAD75CF /* Resources */,
+ 7371D5E1290B9586B2A167512DF59FC3 /* Support Files */,
+ );
+ name = SwiftAssetsPickerController;
+ path = SwiftAssetsPickerController;
+ sourceTree = "";
+ };
+ 9457170DA9D3F951DFAFAFAE051557E3 /* Frameworks */ = {
+ isa = PBXGroup;
+ children = (
+ C0D33443CE113045A81E2829995AB4EE /* CheckMarkView.framework */,
+ 4E19CDE254C3E58916CA30157DEDE5BB /* iOS */,
+ );
+ name = Frameworks;
+ sourceTree = "";
+ };
+ 96255374ED7237FDDBD75145F359047E /* Resources */ = {
+ isa = PBXGroup;
+ children = (
+ 5CC3CBDC4476E9C005BA695876A481C6 /* YUCIBilateralFilter.cikernel */,
+ 940EFBD431C3409783CD8BD103D67143 /* YUCIBlobsGenerator.cikernel */,
+ 4BB0D588EC40E67161708E0220961F03 /* YUCICLAHE.cikernel */,
+ A6F95414BBAC0D224A0AD5865E59CA2A /* YUCIColorLookup.cikernel */,
+ D7189BC6D41CD6B913C43250029A1980 /* YUCIColorLookupTableDefault.png */,
+ 8826F8507844D22EE964120F6EBCB32E /* YUCICrossZoomTransition.cikernel */,
+ 415045B65D9AA5BC6040DE7D54098950 /* YUCIFilmBurnTransition.cikernel */,
+ 58D2911208E3CBDDFC3E936EEEC515A7 /* YUCIFlashTransition.cikernel */,
+ 449B7B73E80D65D0C99D45E14A00882B /* YUCIFXAA.cikernel */,
+ 17A0B067F5EC6A9EEC8995F6CA1AE623 /* YUCIHSLToRGB.cikernel */,
+ A7564A33FE162D13E9F2B44EF29ED8CB /* YUCIReflectedTile.cikernel */,
+ E97BAF7EA8198E2DE74E988CB392F2B1 /* YUCIRGBToHSL.cikernel */,
+ D6363F052E9DF7DE3C37EABA06EF62E9 /* YUCIRGBToneCurve.cikernel */,
+ 20F4742A040A965108614F171DBE6DDE /* YUCISkyGenerator.cikernel */,
+ A6DAC65535D5D28FF9BBFFF09E0AE979 /* YUCIStarfieldGenerator.cikernel */,
+ 9C036D6FC915976237D525778988312C /* YUCISurfaceBlur.cikernel */,
+ 94532DF461095742FAB154DBE3B9AF7F /* YUCITriangularPixellate.cikernel */,
+ );
+ name = Resources;
+ sourceTree = "";
+ };
+ ACDA2798CCA9ED23D5122BB25A0F76E8 /* Pods */ = {
+ isa = PBXGroup;
+ children = (
+ 5AF23CAE32B0C6D42B2B3015A0027837 /* CheckMarkView */,
+ 8BE05D1D2E122F4E73173DAE96E779EB /* SwiftAssetsPickerController */,
+ B13ECB66AF219CD3CF0459D83270A011 /* Vivid */,
+ );
+ name = Pods;
+ sourceTree = "";
+ };
+ B13ECB66AF219CD3CF0459D83270A011 /* Vivid */ = {
+ isa = PBXGroup;
+ children = (
+ 589A54E7266DC122062700F2FE6782B2 /* YUCIBilateralFilter.h */,
+ A8B342C638D95F2CE5F30573DE9D9850 /* YUCIBilateralFilter.m */,
+ 9802258AC56C9CBB5C03B64E391041B9 /* YUCIBlobsGenerator.h */,
+ A961086A2D853AAABAE0969AE4FF96F2 /* YUCIBlobsGenerator.m */,
+ F4ACBD776FB5C68A387D96A3A3A2F6CA /* YUCICLAHE.h */,
+ 312B5F85831E0B0D432259F336C05F6C /* YUCICLAHE.m */,
+ 9A138AA83F58162999640F5ECCCC0D0A /* YUCIColorLookup.h */,
+ 38F6B9D7C7C3EEC6E0405BF06731BAE1 /* YUCIColorLookup.m */,
+ AC20559DECA12496794CFAF42AE0968D /* YUCICrossZoomTransition.h */,
+ 6B14B48D10991CCD3A81E204A476123D /* YUCICrossZoomTransition.m */,
+ 0684A61C5B4F378A427692014BAD98B1 /* YUCIFilmBurnTransition.h */,
+ 5E6A1B9155ECE0C195151F3A14CE8619 /* YUCIFilmBurnTransition.m */,
+ 02D57BE434D8A681FF60CFA7519BB16C /* YUCIFilterConstructor.h */,
+ 1D280949BCE17802D6FCCB182560ABB4 /* YUCIFilterConstructor.m */,
+ 0FE5AF3664593EEE00FEE2B9C0BB0F1B /* YUCIFilterPreviewGenerator.h */,
+ EE4EB7B7F3131461600CA97A09A2F195 /* YUCIFilterPreviewGenerator.m */,
+ CDF8416C6CF98EC3B551FAAC7AFDCFE2 /* YUCIFilterUtilities.h */,
+ 1AE9D43986A950FDEBBD6D4BEF2CA28F /* YUCIFilterUtilities.m */,
+ CD52B1570045FFF28F1B9742D8115F03 /* YUCIFlashTransition.h */,
+ 10C277C48E7BF34DBBD3F973BDEB1B45 /* YUCIFlashTransition.m */,
+ 244537953EDEEC1F93BD61195BD37B56 /* YUCIFXAA.h */,
+ 7AA3A2C5AD81375442D5A6EA842A91C9 /* YUCIFXAA.m */,
+ 9635A6FA16F1069FD93439D613EC0E71 /* YUCIHistogramEqualization.h */,
+ 1AE20B508D248C0F62A5A0E26D649061 /* YUCIHistogramEqualization.m */,
+ D76DE83C0C697134099239F1AD32518D /* YUCIReflectedTile.h */,
+ D8F72D5652E81DAF98513144E0AF2C1B /* YUCIReflectedTile.m */,
+ 770CF9682893A01D6DEEAFF5FE9112CA /* YUCIReflectedTileROICalculator.h */,
+ A39B015A87997300464F5816900164E3 /* YUCIReflectedTileROICalculator.m */,
+ F8689E2EEFCC224A60430C86C342E810 /* YUCIRGBToneCurve.h */,
+ F93A691AAB3331EDAFC7D71F83BF515C /* YUCIRGBToneCurve.m */,
+ 1BBC2B86EDC64F126D5754748473E312 /* YUCISkyGenerator.h */,
+ 9AE27C4A814DFED8B0024AA120434F75 /* YUCISkyGenerator.m */,
+ 82608716946753A1F5CA63E265D2309A /* YUCIStarfieldGenerator.h */,
+ 642759CFB3814D884280D08012AE799E /* YUCIStarfieldGenerator.m */,
+ A7933F95E98B31B4191737CACD7C6A52 /* YUCISurfaceBlur.h */,
+ 33E50F90084387CD9A885E6C5E4E57C1 /* YUCISurfaceBlur.m */,
+ 89888371A9263467382CFD11062BF139 /* YUCITriangularPixellate.h */,
+ 7606F8EBFF6EC7590E53ACACC7A38777 /* YUCITriangularPixellate.m */,
+ E696645C46630CD5CB05D647F4E70845 /* YUCIUtilities.h */,
+ C8EEEC1D9C1FCB69CC5C314136998932 /* YUCIUtilities.m */,
+ 96255374ED7237FDDBD75145F359047E /* Resources */,
+ 5C4DF8B1C8192444E4FDB1A7365641AE /* Support Files */,
+ );
+ name = Vivid;
+ path = Vivid;
+ sourceTree = "";
+ };
+ B5E4F35A13079F47B0DB9B56C21AA9DA /* Pods-iOS-Depth-Sampler */ = {
+ isa = PBXGroup;
+ children = (
+ 19CD51DFB0B70AE9BBB2568AAD47895D /* Info.plist */,
+ B783A94CD15B197C81B14E9B682F7B01 /* Pods-iOS-Depth-Sampler.modulemap */,
+ B13645DF60C7B8FFBD233CE0ECE918F4 /* Pods-iOS-Depth-Sampler-acknowledgements.markdown */,
+ CB9F548A7C4201899FF508311CE2B101 /* Pods-iOS-Depth-Sampler-acknowledgements.plist */,
+ EA16086EEFCA95F8DC1D5025114ACFEC /* Pods-iOS-Depth-Sampler-dummy.m */,
+ D95E86CEBA5D64C4F82AB5E249120223 /* Pods-iOS-Depth-Sampler-frameworks.sh */,
+ 2C86F32573F3F6AE2D95E6CE94B326DA /* Pods-iOS-Depth-Sampler-resources.sh */,
+ 7997301B37B2965B2E5E09DA15A7D245 /* Pods-iOS-Depth-Sampler-umbrella.h */,
+ 85F7AA12070D33E641E4042EC2BAE8FD /* Pods-iOS-Depth-Sampler.debug.xcconfig */,
+ CCB23A7F6876E95245FAA85FEECC109E /* Pods-iOS-Depth-Sampler.release.xcconfig */,
+ );
+ name = "Pods-iOS-Depth-Sampler";
+ path = "Target Support Files/Pods-iOS-Depth-Sampler";
+ sourceTree = "";
+ };
+ C67E0D64482D0C0288B4D3A23EAD75CF /* Resources */ = {
+ isa = PBXGroup;
+ children = (
+ 41B033F114CC6969815ADA124A416DC7 /* Base.lproj */,
+ 63366F374B08EB9F3AEB9E9CB7CF400E /* en.lproj */,
+ CAF95A24A88B23F107B067B47A98A110 /* panorama-icon.png */,
+ E58F4583C97CF9AA2CBAE0FC3B7D36F2 /* timelapse-icon.png */,
+ DCC36527D5450E61F26EE75CC2AAEB65 /* video-icon.png */,
+ );
+ name = Resources;
+ sourceTree = "";
+ };
+/* End PBXGroup section */
+
+/* Begin PBXHeadersBuildPhase section */
+ 0ED16D8493F363FAA70A95D1229631A8 /* Headers */ = {
+ isa = PBXHeadersBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 845229C9E99B5631F77A1ABCF1E6A8E9 /* CheckMarkView-umbrella.h in Headers */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 45E946D303D73EAEDC6DDE6D98329CF2 /* Headers */ = {
+ isa = PBXHeadersBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 5A98A69CC444C88FEB53EB8174161001 /* Vivid-umbrella.h in Headers */,
+ BA61546A10659B82B1C67DD974E479BF /* YUCIBilateralFilter.h in Headers */,
+ 17BCDEEA1E963D735018E6D26A6E02AC /* YUCIBlobsGenerator.h in Headers */,
+ 5A3E86B081429654AB36B138562DD948 /* YUCICLAHE.h in Headers */,
+ 46F5FEFE52A547C0BFFA5341E306D8E3 /* YUCIColorLookup.h in Headers */,
+ 5D081D4E7AF4627B8FF83B96F05644FB /* YUCICrossZoomTransition.h in Headers */,
+ C35A633C028E7C7897B2BE7F4CA589B7 /* YUCIFilmBurnTransition.h in Headers */,
+ CE1110E7CDDB94CA51965F55B053ECD8 /* YUCIFilterConstructor.h in Headers */,
+ 7DC85D898923159D6F7D26D68B2ECD39 /* YUCIFilterPreviewGenerator.h in Headers */,
+ CF61B5A2CB426462FA859C8BEB98B8E7 /* YUCIFilterUtilities.h in Headers */,
+ D9B210B5D427474F0F2BFDC1A10F0235 /* YUCIFlashTransition.h in Headers */,
+ 2458DA96FF9D4605911108C6E895C79F /* YUCIFXAA.h in Headers */,
+ 6A0E0EB8E99E50A0EA84D5107F6FB1A4 /* YUCIHistogramEqualization.h in Headers */,
+ 47490C0B0C23C789C6C32044ECE538DF /* YUCIReflectedTile.h in Headers */,
+ C1FA563F2FB5E5B88F22EFEC020EE904 /* YUCIReflectedTileROICalculator.h in Headers */,
+ 26FA3BEE0508B6254AE2A0FF476C5673 /* YUCIRGBToneCurve.h in Headers */,
+ 53E2600F9B0BF30A6F402C54A1E17D31 /* YUCISkyGenerator.h in Headers */,
+ DB36FC5CAD296FEA5F9A80B9933A25CA /* YUCIStarfieldGenerator.h in Headers */,
+ A975451B6A268051262C6C1F5F87B9A1 /* YUCISurfaceBlur.h in Headers */,
+ 4BBC648BF928D281CDC20D393CFBC4D8 /* YUCITriangularPixellate.h in Headers */,
+ 98D1259F3D35FBA57A019FA388FD9E76 /* YUCIUtilities.h in Headers */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 89D564C50621CAEFFFBF0DC100D22468 /* Headers */ = {
+ isa = PBXHeadersBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 29765801E1ED611F918ED33F6AE1A614 /* SwiftAssetsPickerController-umbrella.h in Headers */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ FFD1E7062E5DC74A1E18FD3344444D0A /* Headers */ = {
+ isa = PBXHeadersBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 95CEB3201F16C2ADD836697EBF3AE1A5 /* Pods-iOS-Depth-Sampler-umbrella.h in Headers */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXHeadersBuildPhase section */
+
+/* Begin PBXNativeTarget section */
+ 73C842E482B6B3C2CA32BFD8F26FF4F6 /* Vivid */ = {
+ isa = PBXNativeTarget;
+ buildConfigurationList = 0D38F66B70CF8063F6E8EF32E3BBEEC0 /* Build configuration list for PBXNativeTarget "Vivid" */;
+ buildPhases = (
+ 76AFD7A627A9765C682A571875854708 /* Sources */,
+ 9073D1FA65879FCF4AAB1C6324857281 /* Frameworks */,
+ 45E946D303D73EAEDC6DDE6D98329CF2 /* Headers */,
+ DA51BD13F3645877F8F28F06B8F0DD76 /* Resources */,
+ );
+ buildRules = (
+ );
+ dependencies = (
+ );
+ name = Vivid;
+ productName = Vivid;
+ productReference = 7AE89405C7A239E69E2FAE62D135EB7E /* Vivid.framework */;
+ productType = "com.apple.product-type.framework";
+ };
+ C03D2E625C313D133C7FDFAAD794F2EB /* Pods-iOS-Depth-Sampler */ = {
+ isa = PBXNativeTarget;
+ buildConfigurationList = 75C0BB3898C3E2A171785B9CD2D3CD19 /* Build configuration list for PBXNativeTarget "Pods-iOS-Depth-Sampler" */;
+ buildPhases = (
+ 843C5BB6D45174BAC9A96DA1FE39D488 /* Sources */,
+ 773512B4CB9D2728B3638F75BCED6314 /* Frameworks */,
+ FFD1E7062E5DC74A1E18FD3344444D0A /* Headers */,
+ );
+ buildRules = (
+ );
+ dependencies = (
+ 23AEC1DA9831F9CF50B1B1F0E1B6F9F9 /* PBXTargetDependency */,
+ C03D8EFF40DDB1EACDE29B9F4EB4E338 /* PBXTargetDependency */,
+ 035335880A61BD88A1BA325E4A837B26 /* PBXTargetDependency */,
+ );
+ name = "Pods-iOS-Depth-Sampler";
+ productName = "Pods-iOS-Depth-Sampler";
+ productReference = DFDB68A78607519C63571C68344A9044 /* Pods_iOS_Depth_Sampler.framework */;
+ productType = "com.apple.product-type.framework";
+ };
+ C6446C484B26560A27A42983580052E9 /* CheckMarkView */ = {
+ isa = PBXNativeTarget;
+ buildConfigurationList = 5B724D6802587D991C0376784658CA09 /* Build configuration list for PBXNativeTarget "CheckMarkView" */;
+ buildPhases = (
+ 9D85D19EAC16347B8BAD51847DEC5A94 /* Sources */,
+ 08A96BBEB293CEA462DFE2FF4FA0889A /* Frameworks */,
+ 0ED16D8493F363FAA70A95D1229631A8 /* Headers */,
+ );
+ buildRules = (
+ );
+ dependencies = (
+ );
+ name = CheckMarkView;
+ productName = CheckMarkView;
+ productReference = D3E28829404FDB70E1D686A92FE0DC99 /* CheckMarkView.framework */;
+ productType = "com.apple.product-type.framework";
+ };
+ FE4A9D1B324616BC82D0D8D73BE4D382 /* SwiftAssetsPickerController */ = {
+ isa = PBXNativeTarget;
+ buildConfigurationList = 79F2F23897EFF8BE3B815B886E082C78 /* Build configuration list for PBXNativeTarget "SwiftAssetsPickerController" */;
+ buildPhases = (
+ 3B8366E0E0A1925CE908BF6023E09B09 /* Sources */,
+ D83877FFF1E5CCD34DB8270AA4DFE80A /* Frameworks */,
+ 682ABC1C4CC2BE0E11FD1F1237213761 /* Resources */,
+ 89D564C50621CAEFFFBF0DC100D22468 /* Headers */,
+ );
+ buildRules = (
+ );
+ dependencies = (
+ F79DCCD694A23F3B0071A02666BF0449 /* PBXTargetDependency */,
+ );
+ name = SwiftAssetsPickerController;
+ productName = SwiftAssetsPickerController;
+ productReference = 0E118BB3EEC8B7F8DF2D68409B2284BE /* SwiftAssetsPickerController.framework */;
+ productType = "com.apple.product-type.framework";
+ };
+/* End PBXNativeTarget section */
+
+/* Begin PBXProject section */
+ D41D8CD98F00B204E9800998ECF8427E /* Project object */ = {
+ isa = PBXProject;
+ attributes = {
+ LastSwiftUpdateCheck = 0930;
+ LastUpgradeCheck = 0930;
+ };
+ buildConfigurationList = 2D8E8EC45A3A1A1D94AE762CB5028504 /* Build configuration list for PBXProject "Pods" */;
+ compatibilityVersion = "Xcode 3.2";
+ developmentRegion = English;
+ hasScannedForEncodings = 0;
+ knownRegions = (
+ en,
+ );
+ mainGroup = 7DB346D0F39D3F0E887471402A8071AB;
+ productRefGroup = 4234A69510CF6AC3FB616D2C8278F993 /* Products */;
+ projectDirPath = "";
+ projectRoot = "";
+ targets = (
+ C6446C484B26560A27A42983580052E9 /* CheckMarkView */,
+ C03D2E625C313D133C7FDFAAD794F2EB /* Pods-iOS-Depth-Sampler */,
+ FE4A9D1B324616BC82D0D8D73BE4D382 /* SwiftAssetsPickerController */,
+ 73C842E482B6B3C2CA32BFD8F26FF4F6 /* Vivid */,
+ );
+ };
+/* End PBXProject section */
+
+/* Begin PBXResourcesBuildPhase section */
+ 682ABC1C4CC2BE0E11FD1F1237213761 /* Resources */ = {
+ isa = PBXResourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 1F191A2DF59A79B190FB67C5C8B35160 /* Base.lproj in Resources */,
+ 88E3DB0292BD4D5D34E01623D853C4BE /* en.lproj in Resources */,
+ 3B661BFC2BBA16C23A64BA240154154B /* panorama-icon.png in Resources */,
+ F8A3A5E5A8B2A125E3B7A46BC4EE4374 /* timelapse-icon.png in Resources */,
+ 70CB28C344834038845C13CF11D53C5D /* video-icon.png in Resources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ DA51BD13F3645877F8F28F06B8F0DD76 /* Resources */ = {
+ isa = PBXResourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ E69128BB562E4EC3954B3E80E80E0794 /* YUCIBilateralFilter.cikernel in Resources */,
+ 4E6D0EF4079EF8FED003C886367300E1 /* YUCIBlobsGenerator.cikernel in Resources */,
+ A1C476D302EC446B3A184A914AF8F287 /* YUCICLAHE.cikernel in Resources */,
+ 0B758CA0028C2DBD873AFB539A71F966 /* YUCIColorLookup.cikernel in Resources */,
+ 9AD2B83C7EBAFFE603C91B3B51D30C61 /* YUCIColorLookupTableDefault.png in Resources */,
+ 0761DD9351B67040466C822544A722B8 /* YUCICrossZoomTransition.cikernel in Resources */,
+ B36D734C8AACE4BAE4114665B79BDE63 /* YUCIFilmBurnTransition.cikernel in Resources */,
+ D7E9D928E7FA4B35A2F720D7FEF061B8 /* YUCIFlashTransition.cikernel in Resources */,
+ 17F5F64AA0D1EEA04A6264D7AD5F5704 /* YUCIFXAA.cikernel in Resources */,
+ A20558191B7838AE17C82E6FEEB453FF /* YUCIHSLToRGB.cikernel in Resources */,
+ B77DA20ADBC535A42D6B7EC3258CDFCA /* YUCIReflectedTile.cikernel in Resources */,
+ 0479FF77B6FDF5FE425E53F967D4CAA2 /* YUCIRGBToHSL.cikernel in Resources */,
+ DA486502D6F21CB13761315972D9B131 /* YUCIRGBToneCurve.cikernel in Resources */,
+ CA227A879FE95A2A9ECB2F3F92521668 /* YUCISkyGenerator.cikernel in Resources */,
+ A2E91F4151C435FAB58C2D3A885561DC /* YUCIStarfieldGenerator.cikernel in Resources */,
+ 822345453F20B92B38CE0E2000DD0E5A /* YUCISurfaceBlur.cikernel in Resources */,
+ 1771A562ECE0978985CCF404E0A60266 /* YUCITriangularPixellate.cikernel in Resources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXResourcesBuildPhase section */
+
+/* Begin PBXSourcesBuildPhase section */
+ 3B8366E0E0A1925CE908BF6023E09B09 /* Sources */ = {
+ isa = PBXSourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 7F11D0062BE9AB63DCE199F4746D4021 /* AssetsPickerController.swift in Sources */,
+ DF3B29DFEB7E2ABE269E18B306BA40B5 /* AssetsPickerGridController.swift in Sources */,
+ 26902991AEB59C46C0D22E26988FC018 /* SwiftAssetsPickerController-dummy.m in Sources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 76AFD7A627A9765C682A571875854708 /* Sources */ = {
+ isa = PBXSourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 4249F341CCA4BC81C0645952BE8C05BE /* Vivid-dummy.m in Sources */,
+ 76A953617889CBDA32BBCDB6D824B006 /* YUCIBilateralFilter.m in Sources */,
+ 045549B2370B900DF9C79DFC7EDB2F16 /* YUCIBlobsGenerator.m in Sources */,
+ 4D2E78996E28048DB565D1128A8CD2D6 /* YUCICLAHE.m in Sources */,
+ 1C7A917D84B93F188C4EABEB8F4E3672 /* YUCIColorLookup.m in Sources */,
+ B8D46C7BD96170222A6835C9E029F413 /* YUCICrossZoomTransition.m in Sources */,
+ 83DC64D64615AFF26113225B5591F9A3 /* YUCIFilmBurnTransition.m in Sources */,
+ D8C982C0E7054E1A42DC2230E557F9C9 /* YUCIFilterConstructor.m in Sources */,
+ 0355812D88010D31EA944A6F9A0BD23D /* YUCIFilterPreviewGenerator.m in Sources */,
+ A613029ACDA1625938F91201A29AFA4F /* YUCIFilterUtilities.m in Sources */,
+ 0C58CBE231244C713F17D2C9AD8B2BE8 /* YUCIFlashTransition.m in Sources */,
+ D18D393EFE3195C164EE5F7744273CD7 /* YUCIFXAA.m in Sources */,
+ F7CE4A0869F9C98B9223B6481DFEB13C /* YUCIHistogramEqualization.m in Sources */,
+ AEA696946694746981FEE23D7BA47CA7 /* YUCIReflectedTile.m in Sources */,
+ 8CE3921A3EF71DDF8B8494DDB8EBDB29 /* YUCIReflectedTileROICalculator.m in Sources */,
+ 477D8D88E31AFAFDC45CD5FD3A1A843D /* YUCIRGBToneCurve.m in Sources */,
+ 514B3824FA0FC3304145AA53227DBE4F /* YUCISkyGenerator.m in Sources */,
+ 73F7B1278F66B4CCDE890C6ED684191C /* YUCIStarfieldGenerator.m in Sources */,
+ 5E750B65566190CF568D54B73DEEC8D7 /* YUCISurfaceBlur.m in Sources */,
+ F3DE85D541B7FC01AC2859D28AA91EE0 /* YUCITriangularPixellate.m in Sources */,
+ 7806869FDC91A58AF4DC7843086E9406 /* YUCIUtilities.m in Sources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 843C5BB6D45174BAC9A96DA1FE39D488 /* Sources */ = {
+ isa = PBXSourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 2BCA4E5BFFD81A7E3DE3044016371E75 /* Pods-iOS-Depth-Sampler-dummy.m in Sources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+ 9D85D19EAC16347B8BAD51847DEC5A94 /* Sources */ = {
+ isa = PBXSourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ EEEDB220E8731680DA51EC442E1CA617 /* CheckMarkView-dummy.m in Sources */,
+ 405ABAD50EFE43A95F4D7C539E83A484 /* CheckMarkView.swift in Sources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXSourcesBuildPhase section */
+
+/* Begin PBXTargetDependency section */
+ 035335880A61BD88A1BA325E4A837B26 /* PBXTargetDependency */ = {
+ isa = PBXTargetDependency;
+ name = Vivid;
+ target = 73C842E482B6B3C2CA32BFD8F26FF4F6 /* Vivid */;
+ targetProxy = 08B0B3F88FF77C920651EE528714ED7F /* PBXContainerItemProxy */;
+ };
+ 23AEC1DA9831F9CF50B1B1F0E1B6F9F9 /* PBXTargetDependency */ = {
+ isa = PBXTargetDependency;
+ name = CheckMarkView;
+ target = C6446C484B26560A27A42983580052E9 /* CheckMarkView */;
+ targetProxy = 2DA5FACCE9E3A139A3A97A714CFCA799 /* PBXContainerItemProxy */;
+ };
+ C03D8EFF40DDB1EACDE29B9F4EB4E338 /* PBXTargetDependency */ = {
+ isa = PBXTargetDependency;
+ name = SwiftAssetsPickerController;
+ target = FE4A9D1B324616BC82D0D8D73BE4D382 /* SwiftAssetsPickerController */;
+ targetProxy = 824D927267E5BA2812677F0CC30E7C2F /* PBXContainerItemProxy */;
+ };
+ F79DCCD694A23F3B0071A02666BF0449 /* PBXTargetDependency */ = {
+ isa = PBXTargetDependency;
+ name = CheckMarkView;
+ target = C6446C484B26560A27A42983580052E9 /* CheckMarkView */;
+ targetProxy = 05FBE67461EE27C3E255D8E5B5D068F7 /* PBXContainerItemProxy */;
+ };
+/* End PBXTargetDependency section */
+
+/* Begin XCBuildConfiguration section */
+ 08638A96A528B1EC32DE62F8A728389D /* Release */ = {
+ isa = XCBuildConfiguration;
+ buildSettings = {
+ ALWAYS_SEARCH_USER_PATHS = NO;
+ CLANG_ANALYZER_NONNULL = YES;
+ CLANG_ANALYZER_NUMBER_OBJECT_CONVERSION = YES_AGGRESSIVE;
+ CLANG_CXX_LANGUAGE_STANDARD = "gnu++14";
+ CLANG_CXX_LIBRARY = "libc++";
+ CLANG_ENABLE_MODULES = YES;
+ CLANG_ENABLE_OBJC_ARC = YES;
+ CLANG_ENABLE_OBJC_WEAK = YES;
+ CLANG_WARN_BLOCK_CAPTURE_AUTORELEASING = YES;
+ CLANG_WARN_BOOL_CONVERSION = YES;
+ CLANG_WARN_COMMA = YES;
+ CLANG_WARN_CONSTANT_CONVERSION = YES;
+ CLANG_WARN_DEPRECATED_OBJC_IMPLEMENTATIONS = YES;
+ CLANG_WARN_DIRECT_OBJC_ISA_USAGE = YES_ERROR;
+ CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
+ CLANG_WARN_EMPTY_BODY = YES;
+ CLANG_WARN_ENUM_CONVERSION = YES;
+ CLANG_WARN_INFINITE_RECURSION = YES;
+ CLANG_WARN_INT_CONVERSION = YES;
+ CLANG_WARN_NON_LITERAL_NULL_CONVERSION = YES;
+ CLANG_WARN_OBJC_IMPLICIT_RETAIN_SELF = YES;
+ CLANG_WARN_OBJC_LITERAL_CONVERSION = YES;
+ CLANG_WARN_OBJC_ROOT_CLASS = YES_ERROR;
+ CLANG_WARN_RANGE_LOOP_ANALYSIS = YES;
+ CLANG_WARN_STRICT_PROTOTYPES = YES;
+ CLANG_WARN_SUSPICIOUS_MOVE = YES;
+ CLANG_WARN_UNGUARDED_AVAILABILITY = YES_AGGRESSIVE;
+ CLANG_WARN_UNREACHABLE_CODE = YES;
+ CLANG_WARN__DUPLICATE_METHOD_MATCH = YES;
+ CODE_SIGNING_ALLOWED = NO;
+ CODE_SIGNING_REQUIRED = NO;
+ COPY_PHASE_STRIP = NO;
+ DEBUG_INFORMATION_FORMAT = "dwarf-with-dsym";
+ ENABLE_NS_ASSERTIONS = NO;
+ ENABLE_STRICT_OBJC_MSGSEND = YES;
+ GCC_C_LANGUAGE_STANDARD = gnu11;
+ GCC_NO_COMMON_BLOCKS = YES;
+ GCC_PREPROCESSOR_DEFINITIONS = (
+ "POD_CONFIGURATION_RELEASE=1",
+ "$(inherited)",
+ );
+ GCC_WARN_64_TO_32_BIT_CONVERSION = YES;
+ GCC_WARN_ABOUT_RETURN_TYPE = YES_ERROR;
+ GCC_WARN_UNDECLARED_SELECTOR = YES;
+ GCC_WARN_UNINITIALIZED_AUTOS = YES_AGGRESSIVE;
+ GCC_WARN_UNUSED_FUNCTION = YES;
+ GCC_WARN_UNUSED_VARIABLE = YES;
+ IPHONEOS_DEPLOYMENT_TARGET = 11.0;
+ MTL_ENABLE_DEBUG_INFO = NO;
+ PRODUCT_NAME = "$(TARGET_NAME)";
+ STRIP_INSTALLED_PRODUCT = NO;
+ SYMROOT = "${SRCROOT}/../build";
+ };
+ name = Release;
+ };
+ 0C921C73872DA6F13EFD3F45BF4AAD8F /* Release */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = BA8D1258EA69F184F48352D8F2B1D305 /* SwiftAssetsPickerController.xcconfig */;
+ buildSettings = {
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_PREFIX_HEADER = "Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-prefix.pch";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/SwiftAssetsPickerController/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 11.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MODULEMAP_FILE = "Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.modulemap";
+ PRODUCT_MODULE_NAME = SwiftAssetsPickerController;
+ PRODUCT_NAME = SwiftAssetsPickerController;
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = "$(inherited) ";
+ SWIFT_OPTIMIZATION_LEVEL = "-Owholemodule";
+ SWIFT_VERSION = 4.0;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VALIDATE_PRODUCT = YES;
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Release;
+ };
+ 4E0C55C15BDB168ED47E0BE1949794A6 /* Debug */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = F7536114BC001F60ECFF230D1F13B1B3 /* CheckMarkView.xcconfig */;
+ buildSettings = {
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_PREFIX_HEADER = "Target Support Files/CheckMarkView/CheckMarkView-prefix.pch";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/CheckMarkView/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 8.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MODULEMAP_FILE = "Target Support Files/CheckMarkView/CheckMarkView.modulemap";
+ PRODUCT_MODULE_NAME = CheckMarkView;
+ PRODUCT_NAME = CheckMarkView;
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = "$(inherited) ";
+ SWIFT_OPTIMIZATION_LEVEL = "-Onone";
+ SWIFT_VERSION = 4.2;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Debug;
+ };
+ 5112851510D968B33CAEE873E9A21AA2 /* Debug */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = 385C4B854706867C6A919A9656B3A195 /* Vivid.xcconfig */;
+ buildSettings = {
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_PREFIX_HEADER = "Target Support Files/Vivid/Vivid-prefix.pch";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/Vivid/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 8.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MODULEMAP_FILE = "Target Support Files/Vivid/Vivid.modulemap";
+ PRODUCT_MODULE_NAME = Vivid;
+ PRODUCT_NAME = Vivid;
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = "$(inherited) ";
+ SWIFT_VERSION = 4.2;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Debug;
+ };
+ 6D2AD94BCAE6FFB292AFD7EE443B2381 /* Release */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = F7536114BC001F60ECFF230D1F13B1B3 /* CheckMarkView.xcconfig */;
+ buildSettings = {
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_PREFIX_HEADER = "Target Support Files/CheckMarkView/CheckMarkView-prefix.pch";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/CheckMarkView/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 8.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MODULEMAP_FILE = "Target Support Files/CheckMarkView/CheckMarkView.modulemap";
+ PRODUCT_MODULE_NAME = CheckMarkView;
+ PRODUCT_NAME = CheckMarkView;
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = "$(inherited) ";
+ SWIFT_OPTIMIZATION_LEVEL = "-Owholemodule";
+ SWIFT_VERSION = 4.2;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VALIDATE_PRODUCT = YES;
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Release;
+ };
+ 7BC1F1491993CCED4097068D607BD105 /* Debug */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = 85F7AA12070D33E641E4042EC2BAE8FD /* Pods-iOS-Depth-Sampler.debug.xcconfig */;
+ buildSettings = {
+ ALWAYS_EMBED_SWIFT_STANDARD_LIBRARIES = NO;
+ CLANG_ENABLE_OBJC_WEAK = NO;
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/Pods-iOS-Depth-Sampler/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 11.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MACH_O_TYPE = staticlib;
+ MODULEMAP_FILE = "Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.modulemap";
+ OTHER_LDFLAGS = "";
+ OTHER_LIBTOOLFLAGS = "";
+ PODS_ROOT = "$(SRCROOT)";
+ PRODUCT_BUNDLE_IDENTIFIER = "org.cocoapods.${PRODUCT_NAME:rfc1034identifier}";
+ PRODUCT_NAME = "$(TARGET_NAME:c99extidentifier)";
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = DEBUG;
+ SWIFT_OPTIMIZATION_LEVEL = "-Onone";
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Debug;
+ };
+ 8B2E0E5F0116537FD53FB31BB337A483 /* Release */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = CCB23A7F6876E95245FAA85FEECC109E /* Pods-iOS-Depth-Sampler.release.xcconfig */;
+ buildSettings = {
+ ALWAYS_EMBED_SWIFT_STANDARD_LIBRARIES = NO;
+ CLANG_ENABLE_OBJC_WEAK = NO;
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/Pods-iOS-Depth-Sampler/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 11.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MACH_O_TYPE = staticlib;
+ MODULEMAP_FILE = "Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.modulemap";
+ OTHER_LDFLAGS = "";
+ OTHER_LIBTOOLFLAGS = "";
+ PODS_ROOT = "$(SRCROOT)";
+ PRODUCT_BUNDLE_IDENTIFIER = "org.cocoapods.${PRODUCT_NAME:rfc1034identifier}";
+ PRODUCT_NAME = "$(TARGET_NAME:c99extidentifier)";
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_OPTIMIZATION_LEVEL = "-Owholemodule";
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VALIDATE_PRODUCT = YES;
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Release;
+ };
+ 9DAE5233986C01ADB6E67169C53A3FD2 /* Debug */ = {
+ isa = XCBuildConfiguration;
+ buildSettings = {
+ ALWAYS_SEARCH_USER_PATHS = NO;
+ CLANG_ANALYZER_NONNULL = YES;
+ CLANG_ANALYZER_NUMBER_OBJECT_CONVERSION = YES_AGGRESSIVE;
+ CLANG_CXX_LANGUAGE_STANDARD = "gnu++14";
+ CLANG_CXX_LIBRARY = "libc++";
+ CLANG_ENABLE_MODULES = YES;
+ CLANG_ENABLE_OBJC_ARC = YES;
+ CLANG_ENABLE_OBJC_WEAK = YES;
+ CLANG_WARN_BLOCK_CAPTURE_AUTORELEASING = YES;
+ CLANG_WARN_BOOL_CONVERSION = YES;
+ CLANG_WARN_COMMA = YES;
+ CLANG_WARN_CONSTANT_CONVERSION = YES;
+ CLANG_WARN_DEPRECATED_OBJC_IMPLEMENTATIONS = YES;
+ CLANG_WARN_DIRECT_OBJC_ISA_USAGE = YES_ERROR;
+ CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
+ CLANG_WARN_EMPTY_BODY = YES;
+ CLANG_WARN_ENUM_CONVERSION = YES;
+ CLANG_WARN_INFINITE_RECURSION = YES;
+ CLANG_WARN_INT_CONVERSION = YES;
+ CLANG_WARN_NON_LITERAL_NULL_CONVERSION = YES;
+ CLANG_WARN_OBJC_IMPLICIT_RETAIN_SELF = YES;
+ CLANG_WARN_OBJC_LITERAL_CONVERSION = YES;
+ CLANG_WARN_OBJC_ROOT_CLASS = YES_ERROR;
+ CLANG_WARN_RANGE_LOOP_ANALYSIS = YES;
+ CLANG_WARN_STRICT_PROTOTYPES = YES;
+ CLANG_WARN_SUSPICIOUS_MOVE = YES;
+ CLANG_WARN_UNGUARDED_AVAILABILITY = YES_AGGRESSIVE;
+ CLANG_WARN_UNREACHABLE_CODE = YES;
+ CLANG_WARN__DUPLICATE_METHOD_MATCH = YES;
+ CODE_SIGNING_ALLOWED = NO;
+ CODE_SIGNING_REQUIRED = NO;
+ COPY_PHASE_STRIP = NO;
+ DEBUG_INFORMATION_FORMAT = dwarf;
+ ENABLE_STRICT_OBJC_MSGSEND = YES;
+ ENABLE_TESTABILITY = YES;
+ GCC_C_LANGUAGE_STANDARD = gnu11;
+ GCC_DYNAMIC_NO_PIC = NO;
+ GCC_NO_COMMON_BLOCKS = YES;
+ GCC_OPTIMIZATION_LEVEL = 0;
+ GCC_PREPROCESSOR_DEFINITIONS = (
+ "POD_CONFIGURATION_DEBUG=1",
+ "DEBUG=1",
+ "$(inherited)",
+ );
+ GCC_WARN_64_TO_32_BIT_CONVERSION = YES;
+ GCC_WARN_ABOUT_RETURN_TYPE = YES_ERROR;
+ GCC_WARN_UNDECLARED_SELECTOR = YES;
+ GCC_WARN_UNINITIALIZED_AUTOS = YES_AGGRESSIVE;
+ GCC_WARN_UNUSED_FUNCTION = YES;
+ GCC_WARN_UNUSED_VARIABLE = YES;
+ IPHONEOS_DEPLOYMENT_TARGET = 11.0;
+ MTL_ENABLE_DEBUG_INFO = YES;
+ ONLY_ACTIVE_ARCH = YES;
+ PRODUCT_NAME = "$(TARGET_NAME)";
+ STRIP_INSTALLED_PRODUCT = NO;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = DEBUG;
+ SYMROOT = "${SRCROOT}/../build";
+ };
+ name = Debug;
+ };
+ A2DCF9DEE081E0FCDE9D5C67A3A51FFF /* Release */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = 385C4B854706867C6A919A9656B3A195 /* Vivid.xcconfig */;
+ buildSettings = {
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_PREFIX_HEADER = "Target Support Files/Vivid/Vivid-prefix.pch";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/Vivid/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 8.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MODULEMAP_FILE = "Target Support Files/Vivid/Vivid.modulemap";
+ PRODUCT_MODULE_NAME = Vivid;
+ PRODUCT_NAME = Vivid;
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = "$(inherited) ";
+ SWIFT_VERSION = 4.2;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VALIDATE_PRODUCT = YES;
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Release;
+ };
+ F02A9FC009331D107E6759BB8A12CF0D /* Debug */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = BA8D1258EA69F184F48352D8F2B1D305 /* SwiftAssetsPickerController.xcconfig */;
+ buildSettings = {
+ CODE_SIGN_IDENTITY = "";
+ "CODE_SIGN_IDENTITY[sdk=appletvos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=iphoneos*]" = "";
+ "CODE_SIGN_IDENTITY[sdk=watchos*]" = "";
+ CURRENT_PROJECT_VERSION = 1;
+ DEFINES_MODULE = YES;
+ DYLIB_COMPATIBILITY_VERSION = 1;
+ DYLIB_CURRENT_VERSION = 1;
+ DYLIB_INSTALL_NAME_BASE = "@rpath";
+ GCC_PREFIX_HEADER = "Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-prefix.pch";
+ GCC_WARN_INHIBIT_ALL_WARNINGS = YES;
+ INFOPLIST_FILE = "Target Support Files/SwiftAssetsPickerController/Info.plist";
+ INSTALL_PATH = "$(LOCAL_LIBRARY_DIR)/Frameworks";
+ IPHONEOS_DEPLOYMENT_TARGET = 11.0;
+ LD_RUNPATH_SEARCH_PATHS = "$(inherited) @executable_path/Frameworks @loader_path/Frameworks";
+ MODULEMAP_FILE = "Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.modulemap";
+ PRODUCT_MODULE_NAME = SwiftAssetsPickerController;
+ PRODUCT_NAME = SwiftAssetsPickerController;
+ SDKROOT = iphoneos;
+ SKIP_INSTALL = YES;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = "$(inherited) ";
+ SWIFT_OPTIMIZATION_LEVEL = "-Onone";
+ SWIFT_VERSION = 4.0;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ VERSIONING_SYSTEM = "apple-generic";
+ VERSION_INFO_PREFIX = "";
+ };
+ name = Debug;
+ };
+/* End XCBuildConfiguration section */
+
+/* Begin XCConfigurationList section */
+ 0D38F66B70CF8063F6E8EF32E3BBEEC0 /* Build configuration list for PBXNativeTarget "Vivid" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ 5112851510D968B33CAEE873E9A21AA2 /* Debug */,
+ A2DCF9DEE081E0FCDE9D5C67A3A51FFF /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+ 2D8E8EC45A3A1A1D94AE762CB5028504 /* Build configuration list for PBXProject "Pods" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ 9DAE5233986C01ADB6E67169C53A3FD2 /* Debug */,
+ 08638A96A528B1EC32DE62F8A728389D /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+ 5B724D6802587D991C0376784658CA09 /* Build configuration list for PBXNativeTarget "CheckMarkView" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ 4E0C55C15BDB168ED47E0BE1949794A6 /* Debug */,
+ 6D2AD94BCAE6FFB292AFD7EE443B2381 /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+ 75C0BB3898C3E2A171785B9CD2D3CD19 /* Build configuration list for PBXNativeTarget "Pods-iOS-Depth-Sampler" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ 7BC1F1491993CCED4097068D607BD105 /* Debug */,
+ 8B2E0E5F0116537FD53FB31BB337A483 /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+ 79F2F23897EFF8BE3B815B886E082C78 /* Build configuration list for PBXNativeTarget "SwiftAssetsPickerController" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ F02A9FC009331D107E6759BB8A12CF0D /* Debug */,
+ 0C921C73872DA6F13EFD3F45BF4AAD8F /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+/* End XCConfigurationList section */
+ };
+ rootObject = D41D8CD98F00B204E9800998ECF8427E /* Project object */;
+}
diff --git a/Pods/SwiftAssetsPickerController/LICENSE b/Pods/SwiftAssetsPickerController/LICENSE
new file mode 100644
index 0000000..6d5f3b3
--- /dev/null
+++ b/Pods/SwiftAssetsPickerController/LICENSE
@@ -0,0 +1,22 @@
+The MIT License (MIT)
+
+Copyright (c) 2015 Maxim Bilan
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
diff --git a/Pods/SwiftAssetsPickerController/README.md b/Pods/SwiftAssetsPickerController/README.md
new file mode 100644
index 0000000..9daadf6
--- /dev/null
+++ b/Pods/SwiftAssetsPickerController/README.md
@@ -0,0 +1,46 @@
+# SwiftAssetsPickerController
+
+[](http://cocoadocs.org/docsets/SwiftAssetsPickerController)
+[](http://cocoadocs.org/docsets/SwiftAssetsPickerController)
+[](http://cocoadocs.org/docsets/SwiftAssetsPickerController)
+[](https://cocoapods.org/pods/SwiftAssetsPickerController)
+[](https://cocoapods.org/pods/SwiftAssetsPickerController)
+
+Simple assets picker controller based on Photos framework. Supports iCloud photos and videos.
+
+
+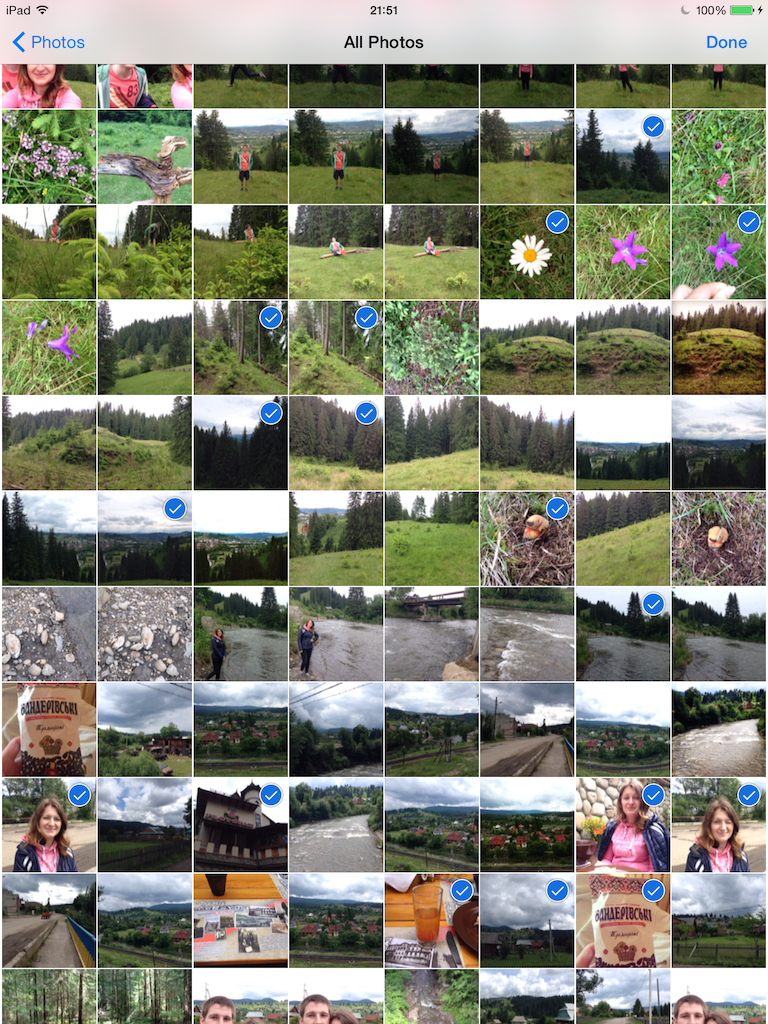
+
+# Installation
+
+CocoaPods:
+
+Swift 3.0:
+pod 'SwiftAssetsPickerController', '0.3.2'
+
+Swift 4.0:
+pod 'SwiftAssetsPickerController', '~> 0.4.0'
+
+
+Manual:
+
+Copy AssetsPickerController.swift and AssetsPickerGridController.swift to your project.
+Also framework uses CheckMarkView, you can found here.
+
+
+## Using
+
+It's really simple. Just see the example:
+
+
+let assetsPickerController = AssetsPickerController()
+assetsPickerController.didSelectAssets = {(assets: Array) -> () in
+ println(assets)
+}
+let navigationController = UINavigationController(rootViewController: rootListAssets)
+presentViewController(navigationController, animated: true, completion: nil)
+
+
+## License
+
+SwiftAssetsPickerController is available under the MIT license. See the LICENSE file for more info.
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/Base.lproj/Localizable.strings b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/Base.lproj/Localizable.strings
new file mode 100644
index 0000000..f11359d
--- /dev/null
+++ b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/Base.lproj/Localizable.strings
@@ -0,0 +1,18 @@
+/*
+ Localizable.strings
+ SwiftAssetsPickerController
+
+ Created by Maxim on 7/22/15.
+ Copyright (c) 2015 Maxim Bilan. All rights reserved.
+*/
+
+"All Photos" = "All Photos";
+"Favorites" = "Favorites";
+"Panoramas" = "Panoramas";
+"Videos" = "Videos";
+"Time Lapse" = "Time Lapse";
+"Recently Deleted" = "Recently Deleted";
+"User Album" = "User Album";
+"Photos" = "Photos";
+"Cancel" = "Cancel";
+"Done" = "Done";
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/en.lproj/Localizable.strings b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/en.lproj/Localizable.strings
new file mode 100644
index 0000000..f11359d
--- /dev/null
+++ b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/en.lproj/Localizable.strings
@@ -0,0 +1,18 @@
+/*
+ Localizable.strings
+ SwiftAssetsPickerController
+
+ Created by Maxim on 7/22/15.
+ Copyright (c) 2015 Maxim Bilan. All rights reserved.
+*/
+
+"All Photos" = "All Photos";
+"Favorites" = "Favorites";
+"Panoramas" = "Panoramas";
+"Videos" = "Videos";
+"Time Lapse" = "Time Lapse";
+"Recently Deleted" = "Recently Deleted";
+"User Album" = "User Album";
+"Photos" = "Photos";
+"Cancel" = "Cancel";
+"Done" = "Done";
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/panorama-icon.png b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/panorama-icon.png
new file mode 100644
index 0000000..d357fbb
Binary files /dev/null and b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/panorama-icon.png differ
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/timelapse-icon.png b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/timelapse-icon.png
new file mode 100644
index 0000000..c0aaf57
Binary files /dev/null and b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/timelapse-icon.png differ
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/video-icon.png b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/video-icon.png
new file mode 100644
index 0000000..4dd9b50
Binary files /dev/null and b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Resources/video-icon.png differ
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Sources/AssetsPickerController.swift b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Sources/AssetsPickerController.swift
new file mode 100644
index 0000000..8d651ae
--- /dev/null
+++ b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Sources/AssetsPickerController.swift
@@ -0,0 +1,225 @@
+//
+// RootListAssetsViewController.swift
+// SwiftAssetsPickerController
+//
+// Created by Maxim Bilan on 6/5/15.
+// Copyright (c) 2015 Maxim Bilan. All rights reserved.
+//
+
+import UIKit
+import Photos
+
+open class AssetsPickerController: UITableViewController, PHPhotoLibraryChangeObserver {
+
+ enum AlbumType: Int {
+// case allPhotos
+// case favorites
+// case panoramas
+// case videos
+// case timeLapse
+// case recentlyDeleted
+// case userAlbum
+ case depthEffect
+
+// static let titles = ["All Photos", "Favorites", "Panoramas", "Videos", "Time Lapse", "Recently Deleted", "User Album", "Depth Effect"]
+ static let titles = ["Depth Effect"]
+ }
+
+ struct RootListItem {
+ var title: String!
+ var albumType: AlbumType
+ var image: UIImage!
+ var collection: PHAssetCollection?
+ }
+
+ fileprivate var items: Array!
+ fileprivate var activityIndicator: UIActivityIndicatorView!
+ fileprivate let thumbnailSize = CGSize(width: 64, height: 64)
+ fileprivate let reuseIdentifier = "RootListAssetsCell"
+
+ open var didSelectAssets: ((Array) -> ())?
+
+ // MARK: View controllers methods
+
+ override open func viewDidLoad() {
+ super.viewDidLoad()
+
+ // Navigation bar
+ navigationItem.title = NSLocalizedString("Photos", comment: "")
+ navigationItem.leftBarButtonItem = UIBarButtonItem(title: NSLocalizedString("Cancel", comment: ""), style: UIBarButtonItemStyle.plain, target: self, action: #selector(AssetsPickerController.cancelAction))
+ navigationItem.rightBarButtonItem = UIBarButtonItem(title: NSLocalizedString("Done", comment: ""), style: UIBarButtonItemStyle.done, target: self, action: #selector(AssetsPickerController.doneAction))
+ navigationItem.rightBarButtonItem?.isEnabled = false
+
+ // Activity indicator
+ activityIndicator = UIActivityIndicatorView(activityIndicatorStyle: UIActivityIndicatorViewStyle.gray)
+ activityIndicator.hidesWhenStopped = true
+ activityIndicator.center = self.view.center
+ self.view.addSubview(activityIndicator)
+
+ // Data
+ items = Array()
+
+ // Notifications
+ PHPhotoLibrary.shared().register(self)
+
+ // Load photo library
+ loadData()
+ }
+
+ deinit {
+ PHPhotoLibrary.shared().unregisterChangeObserver(self)
+ }
+
+ // MARK: Data loading
+
+ func loadData() {
+ tableView.isUserInteractionEnabled = false
+ activityIndicator.startAnimating()
+
+ DispatchQueue.global(qos: .default).async {
+
+ self.items.removeAll(keepingCapacity: false)
+
+// let allPhotosItem = RootListItem(title: AlbumType.titles[AlbumType.allPhotos.rawValue], albumType: AlbumType.allPhotos, image: self.lastImageFromCollection(nil), collection: nil)
+// let assetsCount = self.assetsCountFromCollection(nil)
+// if assetsCount > 0 {
+// self.items.append(allPhotosItem)
+// }
+
+ let smartAlbums = PHAssetCollection.fetchAssetCollections(with: PHAssetCollectionType.smartAlbum, subtype: PHAssetCollectionSubtype.smartAlbumDepthEffect, options: nil)
+ for i: Int in 0 ..< smartAlbums.count {
+ let smartAlbum = smartAlbums[i]
+ var item: RootListItem? = nil
+
+ let assetsCount = self.assetsCountFromCollection(smartAlbum)
+ if assetsCount == 0 {
+ continue
+ }
+
+ switch smartAlbum.assetCollectionSubtype {
+ case .smartAlbumDepthEffect:
+ item = RootListItem(title: AlbumType.titles[AlbumType.depthEffect.rawValue], albumType: AlbumType.depthEffect, image: self.lastImageFromCollection(smartAlbum), collection: smartAlbum)
+ break
+// case .smartAlbumFavorites:
+// item = RootListItem(title: AlbumType.titles[AlbumType.favorites.rawValue], albumType: AlbumType.favorites, image: self.lastImageFromCollection(smartAlbum), collection: smartAlbum)
+// break
+// case .smartAlbumPanoramas:
+// item = RootListItem(title: AlbumType.titles[AlbumType.panoramas.rawValue], albumType: AlbumType.panoramas, image: self.lastImageFromCollection(smartAlbum), collection: smartAlbum)
+// break
+// case .smartAlbumVideos:
+// item = RootListItem(title: AlbumType.titles[AlbumType.videos.rawValue], albumType: AlbumType.videos, image: self.lastImageFromCollection(smartAlbum), collection: smartAlbum)
+// break
+// case .smartAlbumTimelapses:
+// item = RootListItem(title: AlbumType.titles[AlbumType.timeLapse.rawValue], albumType: AlbumType.timeLapse, image: self.lastImageFromCollection(smartAlbum), collection: smartAlbum)
+// break
+
+ default:
+ break
+ }
+
+ if item != nil {
+ self.items.append(item!)
+ }
+ }
+
+// let topLevelUserCollections = PHCollectionList.fetchTopLevelUserCollections(with: nil)
+// for i: Int in 0 ..< topLevelUserCollections.count {
+// if let userCollection = topLevelUserCollections[i] as? PHAssetCollection {
+// let assetsCount = self.assetsCountFromCollection(userCollection)
+// if assetsCount == 0 {
+// continue
+// }
+// let item = RootListItem(title: userCollection.localizedTitle, albumType: AlbumType.userAlbum, image: self.lastImageFromCollection(userCollection), collection: userCollection)
+// self.items.append(item)
+// }
+// }
+
+ DispatchQueue.main.async {
+ self.tableView.reloadData()
+ self.activityIndicator.stopAnimating()
+ self.tableView.isUserInteractionEnabled = true
+ }
+ }
+ }
+
+ // MARK: UITableViewDataSource
+
+ override open func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
+ return items.count
+ }
+
+ override open func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
+ let cell: UITableViewCell = UITableViewCell(style: UITableViewCellStyle.subtitle, reuseIdentifier: reuseIdentifier)
+
+ cell.imageView?.image = items[(indexPath as NSIndexPath).row].image
+ cell.textLabel?.text = NSLocalizedString(items[(indexPath as NSIndexPath).row].title, comment: "")
+
+ return cell
+ }
+
+ // MARK: UITableViewDelegate
+
+ override open func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
+ let assetsGrid = AssetsPickerGridController(collectionViewLayout: UICollectionViewLayout())
+ assetsGrid.collection = items[(indexPath as NSIndexPath).row].collection
+ assetsGrid.didSelectAssets = didSelectAssets
+ assetsGrid.title = items[(indexPath as NSIndexPath).row].title
+ navigationController?.pushViewController(assetsGrid, animated: true)
+ }
+
+ // MARK: Navigation bar actions
+
+ @objc func cancelAction() {
+ dismiss(animated: true, completion: nil)
+ }
+
+ @objc func doneAction() {
+
+ }
+
+ // MARK: PHPhotoLibraryChangeObserver
+
+ open func photoLibraryDidChange(_ changeInstance: PHChange) {
+ loadData()
+ }
+
+ // MARK: Other
+
+ func assetsCountFromCollection(_ collection: PHAssetCollection?) -> Int {
+ let fetchResult = (collection == nil) ? PHAsset.fetchAssets(with: .image, options: nil) : PHAsset.fetchAssets(in: collection!, options: nil)
+ return fetchResult.count
+ }
+
+ func lastImageFromCollection(_ collection: PHAssetCollection?) -> UIImage? {
+
+ var returnImage: UIImage? = nil
+
+ let fetchOptions = PHFetchOptions()
+ fetchOptions.sortDescriptors = [NSSortDescriptor(key: "creationDate", ascending: true)]
+
+ let fetchResult = (collection == nil) ? PHAsset.fetchAssets(with: .image, options: fetchOptions) : PHAsset.fetchAssets(in: collection!, options: fetchOptions)
+ if let lastAsset = fetchResult.lastObject {
+
+ let imageRequestOptions = PHImageRequestOptions()
+ imageRequestOptions.deliveryMode = PHImageRequestOptionsDeliveryMode.fastFormat
+ imageRequestOptions.resizeMode = PHImageRequestOptionsResizeMode.exact
+ imageRequestOptions.isSynchronous = true
+
+ let retinaScale = UIScreen.main.scale
+ let retinaSquare = CGSize(width: thumbnailSize.width * retinaScale, height: thumbnailSize.height * retinaScale)
+
+ let cropSideLength = min(lastAsset.pixelWidth, lastAsset.pixelHeight)
+ let square = CGRect(x: CGFloat(0), y: CGFloat(0), width: CGFloat(cropSideLength), height: CGFloat(cropSideLength))
+ let cropRect = square.applying(CGAffineTransform(scaleX: 1.0 / CGFloat(lastAsset.pixelWidth), y: 1.0 / CGFloat(lastAsset.pixelHeight)))
+
+ imageRequestOptions.normalizedCropRect = cropRect
+
+ PHImageManager.default().requestImage(for: lastAsset, targetSize: retinaSquare, contentMode: PHImageContentMode.aspectFit, options: imageRequestOptions, resultHandler: { (image: UIImage?, info :[AnyHashable: Any]?) -> Void in
+ returnImage = image
+ })
+ }
+
+ return returnImage
+ }
+
+}
diff --git a/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Sources/AssetsPickerGridController.swift b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Sources/AssetsPickerGridController.swift
new file mode 100644
index 0000000..da0a0fb
--- /dev/null
+++ b/Pods/SwiftAssetsPickerController/SwiftAssetsPickerController/Sources/AssetsPickerGridController.swift
@@ -0,0 +1,185 @@
+//
+// AssetsGridViewController.swift
+// SwiftAssetsPickerController
+//
+// Created by Maxim Bilan on 6/5/15.
+// Copyright (c) 2015 Maxim Bilan. All rights reserved.
+//
+
+import UIKit
+import Photos
+import CheckMarkView
+
+class AssetsPickerGridController: UICollectionViewController, UICollectionViewDelegateFlowLayout {
+
+ fileprivate var assetGridThumbnailSize: CGSize = CGSize(width: 0, height: 0)
+ fileprivate let reuseIdentifier = "AssetsGridCell"
+ fileprivate let typeIconSize = CGSize(width: 20, height: 20)
+ fileprivate let checkMarkSize = CGSize(width: 28, height: 28)
+ fileprivate let iconOffset: CGFloat = 3
+ fileprivate let collectionViewEdgeInset: CGFloat = 2
+ fileprivate let assetsInRow: CGFloat = UIDevice.current.userInterfaceIdiom == .phone ? 4 : 8
+
+ let cachingImageManager = PHCachingImageManager()
+ var collection: PHAssetCollection?
+ var selectedIndexes: Set = Set()
+ var didSelectAssets: ((Array) -> ())?
+ fileprivate var assets: [PHAsset]! {
+ willSet {
+ cachingImageManager.stopCachingImagesForAllAssets()
+ }
+
+ didSet {
+ cachingImageManager.startCachingImages(for: self.assets, targetSize: PHImageManagerMaximumSize, contentMode: PHImageContentMode.aspectFill, options: nil)
+ }
+ }
+
+ // MARK: - Initialization
+
+ override init(collectionViewLayout layout: UICollectionViewLayout) {
+ super.init(collectionViewLayout: layout)
+ }
+
+ required init?(coder aDecoder: NSCoder) {
+ fatalError("init(coder:) has not been implemented")
+ }
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+
+ let flowLayout = UICollectionViewFlowLayout()
+ flowLayout.scrollDirection = UICollectionViewScrollDirection.vertical
+
+ collectionView?.collectionViewLayout = flowLayout
+ collectionView?.backgroundColor = UIColor.white
+ collectionView?.register(UICollectionViewCell.classForCoder(), forCellWithReuseIdentifier: reuseIdentifier)
+
+ navigationItem.rightBarButtonItem = UIBarButtonItem(title: NSLocalizedString("Done", comment: ""), style: UIBarButtonItemStyle.done, target: self, action: #selector(AssetsPickerGridController.doneAction))
+ navigationItem.rightBarButtonItem?.isEnabled = false
+
+ let scale = UIScreen.main.scale
+ let cellSize = flowLayout.itemSize
+ assetGridThumbnailSize = CGSize(width: cellSize.width * scale, height: cellSize.height * scale)
+
+ let assetsFetchResult = (collection == nil) ? PHAsset.fetchAssets(with: .image, options: nil) : PHAsset.fetchAssets(in: collection!, options: nil)
+ assets = assetsFetchResult.objects(at: IndexSet(integersIn: Range(NSMakeRange(0, assetsFetchResult.count))!))
+ }
+
+ // MARK: - UICollectionViewDataSource
+
+ override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
+ return assets.count
+ }
+
+ override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
+ let cell = collectionView.dequeueReusableCell(withReuseIdentifier: reuseIdentifier, for: indexPath)
+ cell.backgroundColor = UIColor.black
+
+ let currentTag = cell.tag + 1
+ cell.tag = currentTag
+
+ var thumbnail: UIImageView!
+ var typeIcon: UIImageView!
+ var checkMarkView: CheckMarkView!
+
+ if cell.contentView.subviews.count == 0 {
+ thumbnail = UIImageView(frame: cell.contentView.frame)
+ thumbnail.contentMode = .scaleAspectFill
+ thumbnail.clipsToBounds = true
+ cell.contentView.addSubview(thumbnail)
+
+ typeIcon = UIImageView(frame: CGRect(x: iconOffset, y: cell.contentView.frame.size.height - iconOffset - typeIconSize.height, width: typeIconSize.width, height: typeIconSize.height))
+ typeIcon.contentMode = .scaleAspectFill
+ typeIcon.clipsToBounds = true
+ cell.contentView.addSubview(typeIcon)
+
+ checkMarkView = CheckMarkView(frame: CGRect(x: cell.contentView.frame.size.width - iconOffset - checkMarkSize.width, y: iconOffset, width: checkMarkSize.width, height: checkMarkSize.height))
+ checkMarkView.backgroundColor = UIColor.clear
+ checkMarkView.style = CheckMarkView.Style.nothing
+ cell.contentView.addSubview(checkMarkView)
+ }
+ else {
+ thumbnail = cell.contentView.subviews[0] as! UIImageView
+ typeIcon = cell.contentView.subviews[1] as! UIImageView
+ checkMarkView = cell.contentView.subviews[2] as! CheckMarkView
+ }
+
+ let asset = assets[(indexPath as NSIndexPath).row]
+
+ typeIcon.image = nil
+ if asset.mediaType == .video {
+ if asset.mediaSubtypes == .videoTimelapse {
+ typeIcon.image = UIImage(named: "timelapse-icon.png")
+ }
+ else {
+ typeIcon.image = UIImage(named: "video-icon.png")
+ }
+ }
+ else if asset.mediaType == .image {
+ if asset.mediaSubtypes == .photoPanorama {
+ typeIcon.image = UIImage(named: "panorama-icon.png")
+ }
+ }
+
+ checkMarkView.checked = selectedIndexes.contains(indexPath.row)
+
+ cachingImageManager.requestImage(for: asset, targetSize: assetGridThumbnailSize, contentMode: PHImageContentMode.aspectFill, options: nil, resultHandler: { (image: UIImage?, info :[AnyHashable: Any]?) -> Void in
+ if cell.tag == currentTag {
+ thumbnail.image = image
+ }
+ })
+
+ return cell
+ }
+
+ // MARK: - UICollectionViewDelegate
+
+ override func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) {
+ if selectedIndexes.contains((indexPath as NSIndexPath).row) {
+ selectedIndexes.remove((indexPath as NSIndexPath).row)
+ navigationItem.rightBarButtonItem?.isEnabled = selectedIndexes.count > 0 ? true : false
+ }
+ else {
+ navigationItem.rightBarButtonItem?.isEnabled = true
+ selectedIndexes.insert((indexPath as NSIndexPath).row)
+ }
+ collectionView.reloadItems(at: [indexPath])
+ }
+
+ // MARK: - UICollectionViewDelegateFlowLayout
+
+ func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
+ let a = (self.view.frame.size.width - assetsInRow * 1 - 2 * collectionViewEdgeInset) / assetsInRow
+ return CGSize(width: a, height: a)
+ }
+
+ func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, insetForSectionAt section: Int) -> UIEdgeInsets {
+ return UIEdgeInsetsMake(collectionViewEdgeInset, collectionViewEdgeInset, collectionViewEdgeInset, collectionViewEdgeInset)
+ }
+
+ func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumLineSpacingForSectionAt section: Int) -> CGFloat {
+ return 1
+ }
+
+ func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumInteritemSpacingForSectionAt section: Int) -> CGFloat {
+ return 1
+ }
+
+ // MARK: - Navigation bar actions
+
+ @objc func doneAction() {
+
+ var selectedAssets: Array = Array()
+ for index in selectedIndexes {
+ let asset = assets[index]
+ selectedAssets.append(asset)
+ }
+
+ if didSelectAssets != nil {
+ didSelectAssets!(selectedAssets)
+ }
+
+ navigationController!.dismiss(animated: true, completion: nil)
+ }
+
+}
diff --git a/Pods/Target Support Files/CheckMarkView/CheckMarkView-dummy.m b/Pods/Target Support Files/CheckMarkView/CheckMarkView-dummy.m
new file mode 100644
index 0000000..89f61d5
--- /dev/null
+++ b/Pods/Target Support Files/CheckMarkView/CheckMarkView-dummy.m
@@ -0,0 +1,5 @@
+#import
+@interface PodsDummy_CheckMarkView : NSObject
+@end
+@implementation PodsDummy_CheckMarkView
+@end
diff --git a/Pods/Target Support Files/CheckMarkView/CheckMarkView-prefix.pch b/Pods/Target Support Files/CheckMarkView/CheckMarkView-prefix.pch
new file mode 100644
index 0000000..beb2a24
--- /dev/null
+++ b/Pods/Target Support Files/CheckMarkView/CheckMarkView-prefix.pch
@@ -0,0 +1,12 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
diff --git a/Pods/Target Support Files/CheckMarkView/CheckMarkView-umbrella.h b/Pods/Target Support Files/CheckMarkView/CheckMarkView-umbrella.h
new file mode 100644
index 0000000..4c29997
--- /dev/null
+++ b/Pods/Target Support Files/CheckMarkView/CheckMarkView-umbrella.h
@@ -0,0 +1,16 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
+
+FOUNDATION_EXPORT double CheckMarkViewVersionNumber;
+FOUNDATION_EXPORT const unsigned char CheckMarkViewVersionString[];
+
diff --git a/Pods/Target Support Files/CheckMarkView/CheckMarkView.modulemap b/Pods/Target Support Files/CheckMarkView/CheckMarkView.modulemap
new file mode 100644
index 0000000..fa6d701
--- /dev/null
+++ b/Pods/Target Support Files/CheckMarkView/CheckMarkView.modulemap
@@ -0,0 +1,6 @@
+framework module CheckMarkView {
+ umbrella header "CheckMarkView-umbrella.h"
+
+ export *
+ module * { export * }
+}
diff --git a/Pods/Target Support Files/CheckMarkView/CheckMarkView.xcconfig b/Pods/Target Support Files/CheckMarkView/CheckMarkView.xcconfig
new file mode 100644
index 0000000..23a61c2
--- /dev/null
+++ b/Pods/Target Support Files/CheckMarkView/CheckMarkView.xcconfig
@@ -0,0 +1,9 @@
+CONFIGURATION_BUILD_DIR = ${PODS_CONFIGURATION_BUILD_DIR}/CheckMarkView
+GCC_PREPROCESSOR_DEFINITIONS = $(inherited) COCOAPODS=1
+OTHER_SWIFT_FLAGS = $(inherited) "-D" "COCOAPODS"
+PODS_BUILD_DIR = ${BUILD_DIR}
+PODS_CONFIGURATION_BUILD_DIR = ${PODS_BUILD_DIR}/$(CONFIGURATION)$(EFFECTIVE_PLATFORM_NAME)
+PODS_ROOT = ${SRCROOT}
+PODS_TARGET_SRCROOT = ${PODS_ROOT}/CheckMarkView
+PRODUCT_BUNDLE_IDENTIFIER = org.cocoapods.${PRODUCT_NAME:rfc1034identifier}
+SKIP_INSTALL = YES
diff --git a/Pods/Target Support Files/CheckMarkView/Info.plist b/Pods/Target Support Files/CheckMarkView/Info.plist
new file mode 100644
index 0000000..a71f7a0
--- /dev/null
+++ b/Pods/Target Support Files/CheckMarkView/Info.plist
@@ -0,0 +1,26 @@
+
+
+
+
+ CFBundleDevelopmentRegion
+ en
+ CFBundleExecutable
+ ${EXECUTABLE_NAME}
+ CFBundleIdentifier
+ ${PRODUCT_BUNDLE_IDENTIFIER}
+ CFBundleInfoDictionaryVersion
+ 6.0
+ CFBundleName
+ ${PRODUCT_NAME}
+ CFBundlePackageType
+ FMWK
+ CFBundleShortVersionString
+ 0.4.2
+ CFBundleSignature
+ ????
+ CFBundleVersion
+ ${CURRENT_PROJECT_VERSION}
+ NSPrincipalClass
+
+
+
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Info.plist b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Info.plist
new file mode 100644
index 0000000..2243fe6
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Info.plist
@@ -0,0 +1,26 @@
+
+
+
+
+ CFBundleDevelopmentRegion
+ en
+ CFBundleExecutable
+ ${EXECUTABLE_NAME}
+ CFBundleIdentifier
+ ${PRODUCT_BUNDLE_IDENTIFIER}
+ CFBundleInfoDictionaryVersion
+ 6.0
+ CFBundleName
+ ${PRODUCT_NAME}
+ CFBundlePackageType
+ FMWK
+ CFBundleShortVersionString
+ 1.0.0
+ CFBundleSignature
+ ????
+ CFBundleVersion
+ ${CURRENT_PROJECT_VERSION}
+ NSPrincipalClass
+
+
+
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-acknowledgements.markdown b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-acknowledgements.markdown
new file mode 100644
index 0000000..e2591bc
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-acknowledgements.markdown
@@ -0,0 +1,80 @@
+# Acknowledgements
+This application makes use of the following third party libraries:
+
+## CheckMarkView
+
+The MIT License (MIT)
+
+Copyright (c) 2015 Maxim Bilan
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+
+
+## SwiftAssetsPickerController
+
+The MIT License (MIT)
+
+Copyright (c) 2015 Maxim Bilan
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+
+
+## Vivid
+
+The MIT License (MIT)
+
+Copyright (c) 2016 Yu Ao
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+Generated by CocoaPods - https://cocoapods.org
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-acknowledgements.plist b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-acknowledgements.plist
new file mode 100644
index 0000000..8e108a6
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-acknowledgements.plist
@@ -0,0 +1,124 @@
+
+
+
+
+ PreferenceSpecifiers
+
+
+ FooterText
+ This application makes use of the following third party libraries:
+ Title
+ Acknowledgements
+ Type
+ PSGroupSpecifier
+
+
+ FooterText
+ The MIT License (MIT)
+
+Copyright (c) 2015 Maxim Bilan
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+
+ License
+ MIT
+ Title
+ CheckMarkView
+ Type
+ PSGroupSpecifier
+
+
+ FooterText
+ The MIT License (MIT)
+
+Copyright (c) 2015 Maxim Bilan
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+
+ License
+ MIT
+ Title
+ SwiftAssetsPickerController
+ Type
+ PSGroupSpecifier
+
+
+ FooterText
+ The MIT License (MIT)
+
+Copyright (c) 2016 Yu Ao
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+ License
+ MIT
+ Title
+ Vivid
+ Type
+ PSGroupSpecifier
+
+
+ FooterText
+ Generated by CocoaPods - https://cocoapods.org
+ Title
+
+ Type
+ PSGroupSpecifier
+
+
+ StringsTable
+ Acknowledgements
+ Title
+ Acknowledgements
+
+
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-dummy.m b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-dummy.m
new file mode 100644
index 0000000..74b9814
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-dummy.m
@@ -0,0 +1,5 @@
+#import
+@interface PodsDummy_Pods_iOS_Depth_Sampler : NSObject
+@end
+@implementation PodsDummy_Pods_iOS_Depth_Sampler
+@end
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-frameworks.sh b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-frameworks.sh
new file mode 100755
index 0000000..02224a2
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-frameworks.sh
@@ -0,0 +1,157 @@
+#!/bin/sh
+set -e
+set -u
+set -o pipefail
+
+if [ -z ${FRAMEWORKS_FOLDER_PATH+x} ]; then
+ # If FRAMEWORKS_FOLDER_PATH is not set, then there's nowhere for us to copy
+ # frameworks to, so exit 0 (signalling the script phase was successful).
+ exit 0
+fi
+
+echo "mkdir -p ${CONFIGURATION_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}"
+mkdir -p "${CONFIGURATION_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}"
+
+COCOAPODS_PARALLEL_CODE_SIGN="${COCOAPODS_PARALLEL_CODE_SIGN:-false}"
+SWIFT_STDLIB_PATH="${DT_TOOLCHAIN_DIR}/usr/lib/swift/${PLATFORM_NAME}"
+
+# Used as a return value for each invocation of `strip_invalid_archs` function.
+STRIP_BINARY_RETVAL=0
+
+# This protects against multiple targets copying the same framework dependency at the same time. The solution
+# was originally proposed here: https://lists.samba.org/archive/rsync/2008-February/020158.html
+RSYNC_PROTECT_TMP_FILES=(--filter "P .*.??????")
+
+# Copies and strips a vendored framework
+install_framework()
+{
+ if [ -r "${BUILT_PRODUCTS_DIR}/$1" ]; then
+ local source="${BUILT_PRODUCTS_DIR}/$1"
+ elif [ -r "${BUILT_PRODUCTS_DIR}/$(basename "$1")" ]; then
+ local source="${BUILT_PRODUCTS_DIR}/$(basename "$1")"
+ elif [ -r "$1" ]; then
+ local source="$1"
+ fi
+
+ local destination="${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}"
+
+ if [ -L "${source}" ]; then
+ echo "Symlinked..."
+ source="$(readlink "${source}")"
+ fi
+
+ # Use filter instead of exclude so missing patterns don't throw errors.
+ echo "rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" --filter \"- CVS/\" --filter \"- .svn/\" --filter \"- .git/\" --filter \"- .hg/\" --filter \"- Headers\" --filter \"- PrivateHeaders\" --filter \"- Modules\" \"${source}\" \"${destination}\""
+ rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" --filter "- CVS/" --filter "- .svn/" --filter "- .git/" --filter "- .hg/" --filter "- Headers" --filter "- PrivateHeaders" --filter "- Modules" "${source}" "${destination}"
+
+ local basename
+ basename="$(basename -s .framework "$1")"
+ binary="${destination}/${basename}.framework/${basename}"
+ if ! [ -r "$binary" ]; then
+ binary="${destination}/${basename}"
+ fi
+
+ # Strip invalid architectures so "fat" simulator / device frameworks work on device
+ if [[ "$(file "$binary")" == *"dynamically linked shared library"* ]]; then
+ strip_invalid_archs "$binary"
+ fi
+
+ # Resign the code if required by the build settings to avoid unstable apps
+ code_sign_if_enabled "${destination}/$(basename "$1")"
+
+ # Embed linked Swift runtime libraries. No longer necessary as of Xcode 7.
+ if [ "${XCODE_VERSION_MAJOR}" -lt 7 ]; then
+ local swift_runtime_libs
+ swift_runtime_libs=$(xcrun otool -LX "$binary" | grep --color=never @rpath/libswift | sed -E s/@rpath\\/\(.+dylib\).*/\\1/g | uniq -u && exit ${PIPESTATUS[0]})
+ for lib in $swift_runtime_libs; do
+ echo "rsync -auv \"${SWIFT_STDLIB_PATH}/${lib}\" \"${destination}\""
+ rsync -auv "${SWIFT_STDLIB_PATH}/${lib}" "${destination}"
+ code_sign_if_enabled "${destination}/${lib}"
+ done
+ fi
+}
+
+# Copies and strips a vendored dSYM
+install_dsym() {
+ local source="$1"
+ if [ -r "$source" ]; then
+ # Copy the dSYM into a the targets temp dir.
+ echo "rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" --filter \"- CVS/\" --filter \"- .svn/\" --filter \"- .git/\" --filter \"- .hg/\" --filter \"- Headers\" --filter \"- PrivateHeaders\" --filter \"- Modules\" \"${source}\" \"${DERIVED_FILES_DIR}\""
+ rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" --filter "- CVS/" --filter "- .svn/" --filter "- .git/" --filter "- .hg/" --filter "- Headers" --filter "- PrivateHeaders" --filter "- Modules" "${source}" "${DERIVED_FILES_DIR}"
+
+ local basename
+ basename="$(basename -s .framework.dSYM "$source")"
+ binary="${DERIVED_FILES_DIR}/${basename}.framework.dSYM/Contents/Resources/DWARF/${basename}"
+
+ # Strip invalid architectures so "fat" simulator / device frameworks work on device
+ if [[ "$(file "$binary")" == *"Mach-O dSYM companion"* ]]; then
+ strip_invalid_archs "$binary"
+ fi
+
+ if [[ $STRIP_BINARY_RETVAL == 1 ]]; then
+ # Move the stripped file into its final destination.
+ echo "rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" --filter \"- CVS/\" --filter \"- .svn/\" --filter \"- .git/\" --filter \"- .hg/\" --filter \"- Headers\" --filter \"- PrivateHeaders\" --filter \"- Modules\" \"${DERIVED_FILES_DIR}/${basename}.framework.dSYM\" \"${DWARF_DSYM_FOLDER_PATH}\""
+ rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" --filter "- CVS/" --filter "- .svn/" --filter "- .git/" --filter "- .hg/" --filter "- Headers" --filter "- PrivateHeaders" --filter "- Modules" "${DERIVED_FILES_DIR}/${basename}.framework.dSYM" "${DWARF_DSYM_FOLDER_PATH}"
+ else
+ # The dSYM was not stripped at all, in this case touch a fake folder so the input/output paths from Xcode do not reexecute this script because the file is missing.
+ touch "${DWARF_DSYM_FOLDER_PATH}/${basename}.framework.dSYM"
+ fi
+ fi
+}
+
+# Signs a framework with the provided identity
+code_sign_if_enabled() {
+ if [ -n "${EXPANDED_CODE_SIGN_IDENTITY}" -a "${CODE_SIGNING_REQUIRED:-}" != "NO" -a "${CODE_SIGNING_ALLOWED}" != "NO" ]; then
+ # Use the current code_sign_identitiy
+ echo "Code Signing $1 with Identity ${EXPANDED_CODE_SIGN_IDENTITY_NAME}"
+ local code_sign_cmd="/usr/bin/codesign --force --sign ${EXPANDED_CODE_SIGN_IDENTITY} ${OTHER_CODE_SIGN_FLAGS:-} --preserve-metadata=identifier,entitlements '$1'"
+
+ if [ "${COCOAPODS_PARALLEL_CODE_SIGN}" == "true" ]; then
+ code_sign_cmd="$code_sign_cmd &"
+ fi
+ echo "$code_sign_cmd"
+ eval "$code_sign_cmd"
+ fi
+}
+
+# Strip invalid architectures
+strip_invalid_archs() {
+ binary="$1"
+ # Get architectures for current target binary
+ binary_archs="$(lipo -info "$binary" | rev | cut -d ':' -f1 | awk '{$1=$1;print}' | rev)"
+ # Intersect them with the architectures we are building for
+ intersected_archs="$(echo ${ARCHS[@]} ${binary_archs[@]} | tr ' ' '\n' | sort | uniq -d)"
+ # If there are no archs supported by this binary then warn the user
+ if [[ -z "$intersected_archs" ]]; then
+ echo "warning: [CP] Vendored binary '$binary' contains architectures ($binary_archs) none of which match the current build architectures ($ARCHS)."
+ STRIP_BINARY_RETVAL=0
+ return
+ fi
+ stripped=""
+ for arch in $binary_archs; do
+ if ! [[ "${ARCHS}" == *"$arch"* ]]; then
+ # Strip non-valid architectures in-place
+ lipo -remove "$arch" -output "$binary" "$binary" || exit 1
+ stripped="$stripped $arch"
+ fi
+ done
+ if [[ "$stripped" ]]; then
+ echo "Stripped $binary of architectures:$stripped"
+ fi
+ STRIP_BINARY_RETVAL=1
+}
+
+
+if [[ "$CONFIGURATION" == "Debug" ]]; then
+ install_framework "${BUILT_PRODUCTS_DIR}/CheckMarkView/CheckMarkView.framework"
+ install_framework "${BUILT_PRODUCTS_DIR}/SwiftAssetsPickerController/SwiftAssetsPickerController.framework"
+ install_framework "${BUILT_PRODUCTS_DIR}/Vivid/Vivid.framework"
+fi
+if [[ "$CONFIGURATION" == "Release" ]]; then
+ install_framework "${BUILT_PRODUCTS_DIR}/CheckMarkView/CheckMarkView.framework"
+ install_framework "${BUILT_PRODUCTS_DIR}/SwiftAssetsPickerController/SwiftAssetsPickerController.framework"
+ install_framework "${BUILT_PRODUCTS_DIR}/Vivid/Vivid.framework"
+fi
+if [ "${COCOAPODS_PARALLEL_CODE_SIGN}" == "true" ]; then
+ wait
+fi
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-resources.sh b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-resources.sh
new file mode 100755
index 0000000..345301f
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-resources.sh
@@ -0,0 +1,118 @@
+#!/bin/sh
+set -e
+set -u
+set -o pipefail
+
+if [ -z ${UNLOCALIZED_RESOURCES_FOLDER_PATH+x} ]; then
+ # If UNLOCALIZED_RESOURCES_FOLDER_PATH is not set, then there's nowhere for us to copy
+ # resources to, so exit 0 (signalling the script phase was successful).
+ exit 0
+fi
+
+mkdir -p "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}"
+
+RESOURCES_TO_COPY=${PODS_ROOT}/resources-to-copy-${TARGETNAME}.txt
+> "$RESOURCES_TO_COPY"
+
+XCASSET_FILES=()
+
+# This protects against multiple targets copying the same framework dependency at the same time. The solution
+# was originally proposed here: https://lists.samba.org/archive/rsync/2008-February/020158.html
+RSYNC_PROTECT_TMP_FILES=(--filter "P .*.??????")
+
+case "${TARGETED_DEVICE_FAMILY:-}" in
+ 1,2)
+ TARGET_DEVICE_ARGS="--target-device ipad --target-device iphone"
+ ;;
+ 1)
+ TARGET_DEVICE_ARGS="--target-device iphone"
+ ;;
+ 2)
+ TARGET_DEVICE_ARGS="--target-device ipad"
+ ;;
+ 3)
+ TARGET_DEVICE_ARGS="--target-device tv"
+ ;;
+ 4)
+ TARGET_DEVICE_ARGS="--target-device watch"
+ ;;
+ *)
+ TARGET_DEVICE_ARGS="--target-device mac"
+ ;;
+esac
+
+install_resource()
+{
+ if [[ "$1" = /* ]] ; then
+ RESOURCE_PATH="$1"
+ else
+ RESOURCE_PATH="${PODS_ROOT}/$1"
+ fi
+ if [[ ! -e "$RESOURCE_PATH" ]] ; then
+ cat << EOM
+error: Resource "$RESOURCE_PATH" not found. Run 'pod install' to update the copy resources script.
+EOM
+ exit 1
+ fi
+ case $RESOURCE_PATH in
+ *.storyboard)
+ echo "ibtool --reference-external-strings-file --errors --warnings --notices --minimum-deployment-target ${!DEPLOYMENT_TARGET_SETTING_NAME} --output-format human-readable-text --compile ${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename \"$RESOURCE_PATH\" .storyboard`.storyboardc $RESOURCE_PATH --sdk ${SDKROOT} ${TARGET_DEVICE_ARGS}" || true
+ ibtool --reference-external-strings-file --errors --warnings --notices --minimum-deployment-target ${!DEPLOYMENT_TARGET_SETTING_NAME} --output-format human-readable-text --compile "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename \"$RESOURCE_PATH\" .storyboard`.storyboardc" "$RESOURCE_PATH" --sdk "${SDKROOT}" ${TARGET_DEVICE_ARGS}
+ ;;
+ *.xib)
+ echo "ibtool --reference-external-strings-file --errors --warnings --notices --minimum-deployment-target ${!DEPLOYMENT_TARGET_SETTING_NAME} --output-format human-readable-text --compile ${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename \"$RESOURCE_PATH\" .xib`.nib $RESOURCE_PATH --sdk ${SDKROOT} ${TARGET_DEVICE_ARGS}" || true
+ ibtool --reference-external-strings-file --errors --warnings --notices --minimum-deployment-target ${!DEPLOYMENT_TARGET_SETTING_NAME} --output-format human-readable-text --compile "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename \"$RESOURCE_PATH\" .xib`.nib" "$RESOURCE_PATH" --sdk "${SDKROOT}" ${TARGET_DEVICE_ARGS}
+ ;;
+ *.framework)
+ echo "mkdir -p ${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}" || true
+ mkdir -p "${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}"
+ echo "rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" $RESOURCE_PATH ${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}" || true
+ rsync --delete -av "${RSYNC_PROTECT_TMP_FILES[@]}" "$RESOURCE_PATH" "${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}"
+ ;;
+ *.xcdatamodel)
+ echo "xcrun momc \"$RESOURCE_PATH\" \"${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename "$RESOURCE_PATH"`.mom\"" || true
+ xcrun momc "$RESOURCE_PATH" "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename "$RESOURCE_PATH" .xcdatamodel`.mom"
+ ;;
+ *.xcdatamodeld)
+ echo "xcrun momc \"$RESOURCE_PATH\" \"${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename "$RESOURCE_PATH" .xcdatamodeld`.momd\"" || true
+ xcrun momc "$RESOURCE_PATH" "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename "$RESOURCE_PATH" .xcdatamodeld`.momd"
+ ;;
+ *.xcmappingmodel)
+ echo "xcrun mapc \"$RESOURCE_PATH\" \"${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename "$RESOURCE_PATH" .xcmappingmodel`.cdm\"" || true
+ xcrun mapc "$RESOURCE_PATH" "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}/`basename "$RESOURCE_PATH" .xcmappingmodel`.cdm"
+ ;;
+ *.xcassets)
+ ABSOLUTE_XCASSET_FILE="$RESOURCE_PATH"
+ XCASSET_FILES+=("$ABSOLUTE_XCASSET_FILE")
+ ;;
+ *)
+ echo "$RESOURCE_PATH" || true
+ echo "$RESOURCE_PATH" >> "$RESOURCES_TO_COPY"
+ ;;
+ esac
+}
+
+mkdir -p "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}"
+rsync -avr --copy-links --no-relative --exclude '*/.svn/*' --files-from="$RESOURCES_TO_COPY" / "${TARGET_BUILD_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}"
+if [[ "${ACTION}" == "install" ]] && [[ "${SKIP_INSTALL}" == "NO" ]]; then
+ mkdir -p "${INSTALL_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}"
+ rsync -avr --copy-links --no-relative --exclude '*/.svn/*' --files-from="$RESOURCES_TO_COPY" / "${INSTALL_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}"
+fi
+rm -f "$RESOURCES_TO_COPY"
+
+if [[ -n "${WRAPPER_EXTENSION}" ]] && [ "`xcrun --find actool`" ] && [ -n "${XCASSET_FILES:-}" ]
+then
+ # Find all other xcassets (this unfortunately includes those of path pods and other targets).
+ OTHER_XCASSETS=$(find "$PWD" -iname "*.xcassets" -type d)
+ while read line; do
+ if [[ $line != "${PODS_ROOT}*" ]]; then
+ XCASSET_FILES+=("$line")
+ fi
+ done <<<"$OTHER_XCASSETS"
+
+ if [ -z ${ASSETCATALOG_COMPILER_APPICON_NAME+x} ]; then
+ printf "%s\0" "${XCASSET_FILES[@]}" | xargs -0 xcrun actool --output-format human-readable-text --notices --warnings --platform "${PLATFORM_NAME}" --minimum-deployment-target "${!DEPLOYMENT_TARGET_SETTING_NAME}" ${TARGET_DEVICE_ARGS} --compress-pngs --compile "${BUILT_PRODUCTS_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}"
+ else
+ printf "%s\0" "${XCASSET_FILES[@]}" | xargs -0 xcrun actool --output-format human-readable-text --notices --warnings --platform "${PLATFORM_NAME}" --minimum-deployment-target "${!DEPLOYMENT_TARGET_SETTING_NAME}" ${TARGET_DEVICE_ARGS} --compress-pngs --compile "${BUILT_PRODUCTS_DIR}/${UNLOCALIZED_RESOURCES_FOLDER_PATH}" --app-icon "${ASSETCATALOG_COMPILER_APPICON_NAME}" --output-partial-info-plist "${TARGET_TEMP_DIR}/assetcatalog_generated_info_cocoapods.plist"
+ fi
+fi
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-umbrella.h b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-umbrella.h
new file mode 100644
index 0000000..8d9d29d
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-umbrella.h
@@ -0,0 +1,16 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
+
+FOUNDATION_EXPORT double Pods_iOS_Depth_SamplerVersionNumber;
+FOUNDATION_EXPORT const unsigned char Pods_iOS_Depth_SamplerVersionString[];
+
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.debug.xcconfig b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.debug.xcconfig
new file mode 100644
index 0000000..1f6e613
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.debug.xcconfig
@@ -0,0 +1,11 @@
+ALWAYS_EMBED_SWIFT_STANDARD_LIBRARIES = YES
+FRAMEWORK_SEARCH_PATHS = $(inherited) "${PODS_CONFIGURATION_BUILD_DIR}/CheckMarkView" "${PODS_CONFIGURATION_BUILD_DIR}/SwiftAssetsPickerController" "${PODS_CONFIGURATION_BUILD_DIR}/Vivid"
+GCC_PREPROCESSOR_DEFINITIONS = $(inherited) COCOAPODS=1
+LD_RUNPATH_SEARCH_PATHS = $(inherited) '@executable_path/Frameworks' '@loader_path/Frameworks'
+OTHER_CFLAGS = $(inherited) -iquote "${PODS_CONFIGURATION_BUILD_DIR}/CheckMarkView/CheckMarkView.framework/Headers" -iquote "${PODS_CONFIGURATION_BUILD_DIR}/SwiftAssetsPickerController/SwiftAssetsPickerController.framework/Headers" -iquote "${PODS_CONFIGURATION_BUILD_DIR}/Vivid/Vivid.framework/Headers"
+OTHER_LDFLAGS = $(inherited) -framework "CheckMarkView" -framework "SwiftAssetsPickerController" -framework "Vivid"
+OTHER_SWIFT_FLAGS = $(inherited) "-D" "COCOAPODS"
+PODS_BUILD_DIR = ${BUILD_DIR}
+PODS_CONFIGURATION_BUILD_DIR = ${PODS_BUILD_DIR}/$(CONFIGURATION)$(EFFECTIVE_PLATFORM_NAME)
+PODS_PODFILE_DIR_PATH = ${SRCROOT}/.
+PODS_ROOT = ${SRCROOT}/Pods
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.modulemap b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.modulemap
new file mode 100644
index 0000000..01ae8ee
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.modulemap
@@ -0,0 +1,6 @@
+framework module Pods_iOS_Depth_Sampler {
+ umbrella header "Pods-iOS-Depth-Sampler-umbrella.h"
+
+ export *
+ module * { export * }
+}
diff --git a/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.release.xcconfig b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.release.xcconfig
new file mode 100644
index 0000000..1f6e613
--- /dev/null
+++ b/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.release.xcconfig
@@ -0,0 +1,11 @@
+ALWAYS_EMBED_SWIFT_STANDARD_LIBRARIES = YES
+FRAMEWORK_SEARCH_PATHS = $(inherited) "${PODS_CONFIGURATION_BUILD_DIR}/CheckMarkView" "${PODS_CONFIGURATION_BUILD_DIR}/SwiftAssetsPickerController" "${PODS_CONFIGURATION_BUILD_DIR}/Vivid"
+GCC_PREPROCESSOR_DEFINITIONS = $(inherited) COCOAPODS=1
+LD_RUNPATH_SEARCH_PATHS = $(inherited) '@executable_path/Frameworks' '@loader_path/Frameworks'
+OTHER_CFLAGS = $(inherited) -iquote "${PODS_CONFIGURATION_BUILD_DIR}/CheckMarkView/CheckMarkView.framework/Headers" -iquote "${PODS_CONFIGURATION_BUILD_DIR}/SwiftAssetsPickerController/SwiftAssetsPickerController.framework/Headers" -iquote "${PODS_CONFIGURATION_BUILD_DIR}/Vivid/Vivid.framework/Headers"
+OTHER_LDFLAGS = $(inherited) -framework "CheckMarkView" -framework "SwiftAssetsPickerController" -framework "Vivid"
+OTHER_SWIFT_FLAGS = $(inherited) "-D" "COCOAPODS"
+PODS_BUILD_DIR = ${BUILD_DIR}
+PODS_CONFIGURATION_BUILD_DIR = ${PODS_BUILD_DIR}/$(CONFIGURATION)$(EFFECTIVE_PLATFORM_NAME)
+PODS_PODFILE_DIR_PATH = ${SRCROOT}/.
+PODS_ROOT = ${SRCROOT}/Pods
diff --git a/Pods/Target Support Files/SwiftAssetsPickerController/Info.plist b/Pods/Target Support Files/SwiftAssetsPickerController/Info.plist
new file mode 100644
index 0000000..53e4061
--- /dev/null
+++ b/Pods/Target Support Files/SwiftAssetsPickerController/Info.plist
@@ -0,0 +1,26 @@
+
+
+
+
+ CFBundleDevelopmentRegion
+ en
+ CFBundleExecutable
+ ${EXECUTABLE_NAME}
+ CFBundleIdentifier
+ ${PRODUCT_BUNDLE_IDENTIFIER}
+ CFBundleInfoDictionaryVersion
+ 6.0
+ CFBundleName
+ ${PRODUCT_NAME}
+ CFBundlePackageType
+ FMWK
+ CFBundleShortVersionString
+ 0.4.0
+ CFBundleSignature
+ ????
+ CFBundleVersion
+ ${CURRENT_PROJECT_VERSION}
+ NSPrincipalClass
+
+
+
diff --git a/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-dummy.m b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-dummy.m
new file mode 100644
index 0000000..b3a99ef
--- /dev/null
+++ b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-dummy.m
@@ -0,0 +1,5 @@
+#import
+@interface PodsDummy_SwiftAssetsPickerController : NSObject
+@end
+@implementation PodsDummy_SwiftAssetsPickerController
+@end
diff --git a/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-prefix.pch b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-prefix.pch
new file mode 100644
index 0000000..beb2a24
--- /dev/null
+++ b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-prefix.pch
@@ -0,0 +1,12 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
diff --git a/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-umbrella.h b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-umbrella.h
new file mode 100644
index 0000000..1d2d671
--- /dev/null
+++ b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController-umbrella.h
@@ -0,0 +1,16 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
+
+FOUNDATION_EXPORT double SwiftAssetsPickerControllerVersionNumber;
+FOUNDATION_EXPORT const unsigned char SwiftAssetsPickerControllerVersionString[];
+
diff --git a/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.modulemap b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.modulemap
new file mode 100644
index 0000000..1fa4d97
--- /dev/null
+++ b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.modulemap
@@ -0,0 +1,6 @@
+framework module SwiftAssetsPickerController {
+ umbrella header "SwiftAssetsPickerController-umbrella.h"
+
+ export *
+ module * { export * }
+}
diff --git a/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.xcconfig b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.xcconfig
new file mode 100644
index 0000000..f8eaef9
--- /dev/null
+++ b/Pods/Target Support Files/SwiftAssetsPickerController/SwiftAssetsPickerController.xcconfig
@@ -0,0 +1,10 @@
+CONFIGURATION_BUILD_DIR = ${PODS_CONFIGURATION_BUILD_DIR}/SwiftAssetsPickerController
+FRAMEWORK_SEARCH_PATHS = $(inherited) "${PODS_CONFIGURATION_BUILD_DIR}/CheckMarkView"
+GCC_PREPROCESSOR_DEFINITIONS = $(inherited) COCOAPODS=1
+OTHER_SWIFT_FLAGS = $(inherited) "-D" "COCOAPODS"
+PODS_BUILD_DIR = ${BUILD_DIR}
+PODS_CONFIGURATION_BUILD_DIR = ${PODS_BUILD_DIR}/$(CONFIGURATION)$(EFFECTIVE_PLATFORM_NAME)
+PODS_ROOT = ${SRCROOT}
+PODS_TARGET_SRCROOT = ${PODS_ROOT}/SwiftAssetsPickerController
+PRODUCT_BUNDLE_IDENTIFIER = org.cocoapods.${PRODUCT_NAME:rfc1034identifier}
+SKIP_INSTALL = YES
diff --git a/Pods/Target Support Files/Vivid/Info.plist b/Pods/Target Support Files/Vivid/Info.plist
new file mode 100644
index 0000000..b07fa9b
--- /dev/null
+++ b/Pods/Target Support Files/Vivid/Info.plist
@@ -0,0 +1,26 @@
+
+
+
+
+ CFBundleDevelopmentRegion
+ en
+ CFBundleExecutable
+ ${EXECUTABLE_NAME}
+ CFBundleIdentifier
+ ${PRODUCT_BUNDLE_IDENTIFIER}
+ CFBundleInfoDictionaryVersion
+ 6.0
+ CFBundleName
+ ${PRODUCT_NAME}
+ CFBundlePackageType
+ FMWK
+ CFBundleShortVersionString
+ 0.9.0
+ CFBundleSignature
+ ????
+ CFBundleVersion
+ ${CURRENT_PROJECT_VERSION}
+ NSPrincipalClass
+
+
+
diff --git a/Pods/Target Support Files/Vivid/Vivid-dummy.m b/Pods/Target Support Files/Vivid/Vivid-dummy.m
new file mode 100644
index 0000000..7cd88d7
--- /dev/null
+++ b/Pods/Target Support Files/Vivid/Vivid-dummy.m
@@ -0,0 +1,5 @@
+#import
+@interface PodsDummy_Vivid : NSObject
+@end
+@implementation PodsDummy_Vivid
+@end
diff --git a/Pods/Target Support Files/Vivid/Vivid-prefix.pch b/Pods/Target Support Files/Vivid/Vivid-prefix.pch
new file mode 100644
index 0000000..beb2a24
--- /dev/null
+++ b/Pods/Target Support Files/Vivid/Vivid-prefix.pch
@@ -0,0 +1,12 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
diff --git a/Pods/Target Support Files/Vivid/Vivid-umbrella.h b/Pods/Target Support Files/Vivid/Vivid-umbrella.h
new file mode 100644
index 0000000..461132d
--- /dev/null
+++ b/Pods/Target Support Files/Vivid/Vivid-umbrella.h
@@ -0,0 +1,36 @@
+#ifdef __OBJC__
+#import
+#else
+#ifndef FOUNDATION_EXPORT
+#if defined(__cplusplus)
+#define FOUNDATION_EXPORT extern "C"
+#else
+#define FOUNDATION_EXPORT extern
+#endif
+#endif
+#endif
+
+#import "YUCIBilateralFilter.h"
+#import "YUCIBlobsGenerator.h"
+#import "YUCICLAHE.h"
+#import "YUCIColorLookup.h"
+#import "YUCICrossZoomTransition.h"
+#import "YUCIFilmBurnTransition.h"
+#import "YUCIFilterConstructor.h"
+#import "YUCIFilterPreviewGenerator.h"
+#import "YUCIFilterUtilities.h"
+#import "YUCIFlashTransition.h"
+#import "YUCIFXAA.h"
+#import "YUCIHistogramEqualization.h"
+#import "YUCIReflectedTile.h"
+#import "YUCIReflectedTileROICalculator.h"
+#import "YUCIRGBToneCurve.h"
+#import "YUCISkyGenerator.h"
+#import "YUCIStarfieldGenerator.h"
+#import "YUCISurfaceBlur.h"
+#import "YUCITriangularPixellate.h"
+#import "YUCIUtilities.h"
+
+FOUNDATION_EXPORT double VividVersionNumber;
+FOUNDATION_EXPORT const unsigned char VividVersionString[];
+
diff --git a/Pods/Target Support Files/Vivid/Vivid.modulemap b/Pods/Target Support Files/Vivid/Vivid.modulemap
new file mode 100644
index 0000000..4fa740b
--- /dev/null
+++ b/Pods/Target Support Files/Vivid/Vivid.modulemap
@@ -0,0 +1,6 @@
+framework module Vivid {
+ umbrella header "Vivid-umbrella.h"
+
+ export *
+ module * { export * }
+}
diff --git a/Pods/Target Support Files/Vivid/Vivid.xcconfig b/Pods/Target Support Files/Vivid/Vivid.xcconfig
new file mode 100644
index 0000000..dffbfc2
--- /dev/null
+++ b/Pods/Target Support Files/Vivid/Vivid.xcconfig
@@ -0,0 +1,8 @@
+CONFIGURATION_BUILD_DIR = ${PODS_CONFIGURATION_BUILD_DIR}/Vivid
+GCC_PREPROCESSOR_DEFINITIONS = $(inherited) COCOAPODS=1
+PODS_BUILD_DIR = ${BUILD_DIR}
+PODS_CONFIGURATION_BUILD_DIR = ${PODS_BUILD_DIR}/$(CONFIGURATION)$(EFFECTIVE_PLATFORM_NAME)
+PODS_ROOT = ${SRCROOT}
+PODS_TARGET_SRCROOT = ${PODS_ROOT}/Vivid
+PRODUCT_BUNDLE_IDENTIFIER = org.cocoapods.${PRODUCT_NAME:rfc1034identifier}
+SKIP_INSTALL = YES
diff --git a/Pods/Vivid/LICENSE b/Pods/Vivid/LICENSE
new file mode 100644
index 0000000..fbe50e7
--- /dev/null
+++ b/Pods/Vivid/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2016 Yu Ao
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/Pods/Vivid/README.md b/Pods/Vivid/README.md
new file mode 100644
index 0000000..c022b39
--- /dev/null
+++ b/Pods/Vivid/README.md
@@ -0,0 +1,131 @@
+
+
+
+
+
+
+A set of filters and utilities for Apple's [Core Image](https://developer.apple.com/library/mac/documentation/GraphicsImaging/Conceptual/CoreImaging/ci_intro/ci_intro.html) framework.
+
+Available on both OS X and iOS.
+
+Involving...
+
+##Core Image Filters
+
+###Filters
+
+####YUCIRGBToneCurve
+
+Adjusts tone response of the R, G, and B channels of an image.
+
+The filter takes in an array of control points that define the spline curve for each color component, or for all three in the composite.
+
+These are stored as `CIVector`s in an `NSArray`, with normalized X and Y coordinates from `0` to `1`.
+
+The defaults are `[(0,0), (0.5,0.5), (1,1)]`
+
+
+
+####YUCIColorLookup
+
+Uses a color lookup table (LUT) to remap the colors in an image. The default LUT can be found at `Sources/YUCIColorLookupTableDefault.png`
+
+*This filter may not work well in the default light-linear working color space. Use `kCIContextWorkingColorSpace` key to specify a working color space when creating the `CIContext` object.*
+
+
+
+####YUCISurfaceBlur
+
+A bilateral filter. Blurs an image while preserving edges. This filter is almost identical to Photoshop's "Surface Blur" filter.
+
+Useful for creating special effects and for removing noise or graininess. Slow on large `inputRadius`.
+
+
+
+####YUCITriangularPixellate
+
+Maps an image to colored triangles.
+
+
+
+####YUCIFXAA
+
+A basic implementation of FXAA (Fast Approximate Anti-Aliasing).
+
+
+
+####YUCIHistogramEqualization
+
+Perform a [Histogram Equalization](https://en.wikipedia.org/wiki/Histogram_equalization) on the input image. Internally uses `Accelerate.framework`.
+
+
+
+####YUCIReflectedTile
+
+Produces a tiled image from a source image by reflecting pixels over the edges.
+
+
+
+####YUCICLAHE
+
+Perform a [Contrast Limited Adaptive Histogram Equalization](https://en.wikipedia.org/wiki/Adaptive_histogram_equalization#Contrast_Limited_AHE) on the lightness channel of the input image.
+
+
+
+###Transitions
+
+####YUCICrossZoomTransition
+
+A transition that pushes the `inputImage` toward the viewer and then snaps back with the `inputTargetImage`.
+
+
+
+####YUCIFlashTransition
+
+Transitions from one image to another by creating a flash effect.
+
+
+
+###Generators
+
+####YUCIStarfieldGenerator
+
+Generate a starfield image. Animatable by changing the `inputTime` parameter. Based on [Star Nest](https://www.shadertoy.com/view/XlfGRj) by Pablo Román Andrioli
+
+
+
+####YUCIBlobsGenerator
+
+Generate a image with colorful blobs. Animatable by changing the `inputTime` parameter. Based on [Blobs](https://www.shadertoy.com/view/lsfGzr) by [@paulofalcao](https://twitter.com/paulofalcao)
+
+
+
+##Utilities
+
+####YUCIFilterConstructor
+
+A singleton that conforms to `CIFilterConstructor` protocol.
+
+Can be used in `+[CIFilter registerFilterName:constructor:classAttributes:]` to register a `CIFilter`. This filter constructor simply assume that the `filterName` is the class name of the custom `CIFilter` and calls `[[FilterClass alloc] init]` to construct a filter.
+
+####YUCIFilterPreviewGenerator
+
+Can be used to generate a preview image/gif for a filter. All the preview images/gifs on this page are generated by this utility. __For demonstration/testing purposes only, do not use it in your production code.__
+
+##Next
+
+- [x] Add filter previews to readme.
+- [x] AA for triangular pixellate filter.
+- [x] CLAHE
+- [ ] Write a paper on the implementation of CLAHE.
+- [ ] Kuwahara filter
+
+##Related Projects
+
+####[YUCIHighPassSkinSmoothing](https://github.com/YuAo/YUCIHighPassSkinSmoothing)
+
+An implementation of High Pass Skin Smoothing.
+
+####[YUCIImageView](https://github.com/YuAo/YUCIImageView)
+
+An image view for rendering CIImage with Metal/OpenGL/CoreGraphics.
diff --git a/Pods/Vivid/Sources/YUCIBilateralFilter.cikernel b/Pods/Vivid/Sources/YUCIBilateralFilter.cikernel
new file mode 100644
index 0000000..585b98c
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIBilateralFilter.cikernel
@@ -0,0 +1,49 @@
+const int GAUSSIAN_SAMPLES = MACRO_GAUSSIAN_SAMPLES;
+
+kernel vec4 filterKernel(sampler inputImage, vec2 texelOffset, float distanceNormalizationFactor) {
+
+ float GAUSSIAN_WEIGHTS[(GAUSSIAN_SAMPLES + 1)/2];
+
+ MACRO_SETUP_GAUSSIAN_WEIGHTS
+
+ vec2 blurCoordinates[GAUSSIAN_SAMPLES];
+
+ for (int i = 0; i < GAUSSIAN_SAMPLES; i++) {
+ int multiplier = (i - ((GAUSSIAN_SAMPLES - 1) / 2));
+ vec2 blurStep = float(multiplier) * texelOffset;
+ blurCoordinates[i] = destCoord() + blurStep;
+ }
+
+ int centralIndex = (GAUSSIAN_SAMPLES - 1)/2;
+
+ vec4 centralColor;
+ float gaussianWeightTotal;
+ vec4 sum;
+ vec4 sampleColor;
+ float distanceFromCentralColor;
+ float gaussianWeight;
+
+ centralColor = sample(inputImage,samplerTransform(inputImage, blurCoordinates[centralIndex]));
+ gaussianWeightTotal = GAUSSIAN_WEIGHTS[0];
+ sum = centralColor * GAUSSIAN_WEIGHTS[0];
+
+ for (int i = 0; i < centralIndex; i++) {
+ sampleColor = sample(inputImage,samplerTransform(inputImage, blurCoordinates[i]));
+ distanceFromCentralColor = min(distance(centralColor, sampleColor) * distanceNormalizationFactor, 1.0);
+ gaussianWeight = GAUSSIAN_WEIGHTS[centralIndex - i] * (1.0 - distanceFromCentralColor);
+ gaussianWeightTotal += gaussianWeight;
+ sum += sampleColor * gaussianWeight;
+ }
+
+ for (int i = centralIndex + 1; i < GAUSSIAN_SAMPLES; i++) {
+ sampleColor = sample(inputImage,samplerTransform(inputImage, blurCoordinates[i]));
+ distanceFromCentralColor = min(distance(centralColor, sampleColor) * distanceNormalizationFactor, 1.0);
+ gaussianWeight = GAUSSIAN_WEIGHTS[i-centralIndex] * (1.0 - distanceFromCentralColor);
+ gaussianWeightTotal += gaussianWeight;
+ sum += sampleColor * gaussianWeight;
+ }
+
+ vec4 result = sum / gaussianWeightTotal;
+
+ return result;
+}
diff --git a/Pods/Vivid/Sources/YUCIBilateralFilter.h b/Pods/Vivid/Sources/YUCIBilateralFilter.h
new file mode 100644
index 0000000..bd23a9a
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIBilateralFilter.h
@@ -0,0 +1,19 @@
+//
+// YUCIBilateralFilter.h
+// Pods
+//
+// Created by YuAo on 2/2/16.
+//
+//
+
+#import
+
+@interface YUCIBilateralFilter : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputRadius; //default 10
+@property (nonatomic, copy, null_resettable) NSNumber *inputDistanceNormalizationFactor; //default 6.0
+@property (nonatomic, copy, null_resettable) NSNumber *inputTexelSpacingMultiplier; //default 1.0
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIBilateralFilter.m b/Pods/Vivid/Sources/YUCIBilateralFilter.m
new file mode 100644
index 0000000..62887d4
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIBilateralFilter.m
@@ -0,0 +1,118 @@
+//
+// YUCIBilateralFilter.m
+// Pods
+//
+// Created by YuAo on 2/2/16.
+//
+//
+
+#import "YUCIBilateralFilter.h"
+#import "YUCIFilterUtilities.h"
+
+@implementation YUCIBilateralFilter
+
+static NSDictionary *YUCIBilateralFilterKernels;
+
++ (NSInteger)sampleCountForRadius:(NSInteger)radius {
+ CGFloat minimumWeightToFindEdgeOfSamplingArea = 1.0/256.0;
+ radius = floor(sqrt(-2.0 * pow(radius, 2.0) * log(minimumWeightToFindEdgeOfSamplingArea * sqrt(2.0 * M_PI * pow(radius, 2.0))) ));
+ if (radius % 2 == 0) {
+ radius = radius - 1;
+ }
+ if (radius <= 0) {
+ radius = 1;
+ }
+ return radius;
+}
+
++ (CIKernel *)filterKernelForRadius:(NSInteger)radius {
+ NSInteger sampleCount = [self sampleCountForRadius:radius];
+
+ CIKernel *kernel = YUCIBilateralFilterKernels[@(sampleCount)];
+
+ if (kernel) {
+ return kernel;
+ } else {
+ double sigma = radius;
+
+ double sum = 0;
+ NSMutableArray *wights = [NSMutableArray array];
+ for (NSInteger i = 0; i < (sampleCount + 1)/2; ++i) {
+ double wight = YUCIGaussianDistributionPDF(i, sigma);
+ if (i == 0) {
+ sum += wight;
+ } else {
+ sum += wight * 2;
+ }
+ [wights addObject:@(wight)];
+ }
+ double scale = 1.0/sum;
+
+ NSString *setupString = @"";
+ for (NSInteger i = 0; i < wights.count; ++i) {
+ setupString = [setupString stringByAppendingFormat:@"GAUSSIAN_WEIGHTS[%@] = %@; \n",@(i),@([wights[i] doubleValue] * scale)];
+ }
+
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIBilateralFilter class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernelString = [kernelString stringByReplacingOccurrencesOfString:@"MACRO_GAUSSIAN_SAMPLES" withString:@(sampleCount).stringValue];
+ kernelString = [kernelString stringByReplacingOccurrencesOfString:@"MACRO_SETUP_GAUSSIAN_WEIGHTS" withString:setupString];
+ kernel = [CIKernel kernelWithString:kernelString];
+
+ NSMutableDictionary *newKernels = [NSMutableDictionary dictionaryWithDictionary:YUCIBilateralFilterKernels];
+ [newKernels setObject:kernel forKey:@(sampleCount)];
+ YUCIBilateralFilterKernels = newKernels.copy;
+
+ return kernel;
+ }
+}
+
+- (NSNumber *)inputRadius {
+ if (!_inputRadius) {
+ _inputRadius = @(10);
+ }
+ return _inputRadius;
+}
+
+- (NSNumber *)inputTexelSpacingMultiplier {
+ if (!_inputTexelSpacingMultiplier) {
+ _inputTexelSpacingMultiplier = @(1.0);
+ }
+ return _inputTexelSpacingMultiplier;
+}
+
+- (NSNumber *)inputDistanceNormalizationFactor {
+ if (!_inputDistanceNormalizationFactor) {
+ _inputDistanceNormalizationFactor = @(5.0);
+ }
+ return _inputDistanceNormalizationFactor;
+}
+
+- (void)setDefaults {
+ self.inputRadius = nil;
+ self.inputTexelSpacingMultiplier = nil;
+ self.inputDistanceNormalizationFactor = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ CIKernel *kernel = [YUCIBilateralFilter filterKernelForRadius:[self.inputRadius integerValue]];
+ CGFloat inset = -([self.class sampleCountForRadius:self.inputRadius.integerValue] * self.inputTexelSpacingMultiplier.doubleValue)/2.0;
+ CIImage *horizontalPassResult = [kernel applyWithExtent:self.inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return CGRectInset(destRect, inset, 0);
+ } arguments:@[self.inputImage,
+ [CIVector vectorWithX:[self.inputTexelSpacingMultiplier doubleValue] Y:0],
+ self.inputDistanceNormalizationFactor]];
+ CIImage *verticalPassResult = [kernel applyWithExtent:horizontalPassResult.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return CGRectInset(destRect, 0, inset);
+ } arguments:@[horizontalPassResult,
+ [CIVector vectorWithX:0 Y:[self.inputTexelSpacingMultiplier doubleValue]],
+ self.inputDistanceNormalizationFactor]];
+ return verticalPassResult;
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIBlobsGenerator.cikernel b/Pods/Vivid/Sources/YUCIBlobsGenerator.cikernel
new file mode 100644
index 0000000..6f3d7fa
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIBlobsGenerator.cikernel
@@ -0,0 +1,55 @@
+// Blobs by @paulofalcao
+// https://www.shadertoy.com/view/lsfGzr
+
+float makePoint(float x,float y,float fx,float fy,float sx,float sy,float t){
+ float xx=x+sin(t*fx)*sx;
+ float yy=y+cos(t*fy)*sy;
+ return 1.0/sqrt(xx*xx+yy*yy);
+}
+
+kernel vec4 coreImageKernel(vec4 inputExtent, float inputTime) {
+ vec2 fragCoord = destCoord();
+ vec2 iResolution = inputExtent.zw;
+ float time = inputTime;
+
+ vec2 p=(fragCoord.xy/iResolution.x)*2.0-vec2(1.0,iResolution.y/iResolution.x);
+
+ p=p*2.0;
+
+ float x=p.x;
+ float y=p.y;
+
+ float a=makePoint(x,y,3.3,2.9,0.3,0.3,time);
+ a=a+makePoint(x,y,1.9,2.0,0.4,0.4,time);
+ a=a+makePoint(x,y,0.8,0.7,0.4,0.5,time);
+ a=a+makePoint(x,y,2.3,0.1,0.6,0.3,time);
+ a=a+makePoint(x,y,0.8,1.7,0.5,0.4,time);
+ a=a+makePoint(x,y,0.3,1.0,0.4,0.4,time);
+ a=a+makePoint(x,y,1.4,1.7,0.4,0.5,time);
+ a=a+makePoint(x,y,1.3,2.1,0.6,0.3,time);
+ a=a+makePoint(x,y,1.8,1.7,0.5,0.4,time);
+
+ float b=makePoint(x,y,1.2,1.9,0.3,0.3,time);
+ b=b+makePoint(x,y,0.7,2.7,0.4,0.4,time);
+ b=b+makePoint(x,y,1.4,0.6,0.4,0.5,time);
+ b=b+makePoint(x,y,2.6,0.4,0.6,0.3,time);
+ b=b+makePoint(x,y,0.7,1.4,0.5,0.4,time);
+ b=b+makePoint(x,y,0.7,1.7,0.4,0.4,time);
+ b=b+makePoint(x,y,0.8,0.5,0.4,0.5,time);
+ b=b+makePoint(x,y,1.4,0.9,0.6,0.3,time);
+ b=b+makePoint(x,y,0.7,1.3,0.5,0.4,time);
+
+ float c=makePoint(x,y,3.7,0.3,0.3,0.3,time);
+ c=c+makePoint(x,y,1.9,1.3,0.4,0.4,time);
+ c=c+makePoint(x,y,0.8,0.9,0.4,0.5,time);
+ c=c+makePoint(x,y,1.2,1.7,0.6,0.3,time);
+ c=c+makePoint(x,y,0.3,0.6,0.5,0.4,time);
+ c=c+makePoint(x,y,0.3,0.3,0.4,0.4,time);
+ c=c+makePoint(x,y,1.4,0.8,0.4,0.5,time);
+ c=c+makePoint(x,y,0.2,0.6,0.6,0.3,time);
+ c=c+makePoint(x,y,1.3,0.5,0.5,0.4,time);
+
+ vec3 d=vec3(a,b,c)/32.0;
+
+ return vec4(d.x,d.y,d.z,1.0);
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIBlobsGenerator.h b/Pods/Vivid/Sources/YUCIBlobsGenerator.h
new file mode 100644
index 0000000..c9eeedf
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIBlobsGenerator.h
@@ -0,0 +1,18 @@
+//
+// YUCIBlobsGenerator.h
+// Pods
+//
+// Created by YuAo on 2/6/16.
+//
+//
+
+#import
+
+@interface YUCIBlobsGenerator : CIFilter
+
+@property (nonatomic, copy, null_resettable) CIVector *inputExtent; //default 640x800
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputTime; //default 0
+
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIBlobsGenerator.m b/Pods/Vivid/Sources/YUCIBlobsGenerator.m
new file mode 100644
index 0000000..8358ead
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIBlobsGenerator.m
@@ -0,0 +1,62 @@
+//
+// YUCIBlobsGenerator.m
+// Pods
+//
+// Created by YuAo on 2/6/16.
+//
+//
+
+#import "YUCIBlobsGenerator.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIBlobsGenerator
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIBlobsGenerator class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryGenerator],
+ kCIAttributeFilterDisplayName: @"Blobs Generator"}];
+ }
+ }
+ });
+}
+
++ (CIColorKernel *)filterKernel {
+ static CIColorKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIBlobsGenerator class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIColorKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (CIVector *)inputExtent {
+ if (!_inputExtent) {
+ _inputExtent = [CIVector vectorWithCGRect:CGRectMake(0, 0, 640, 480)];
+ }
+ return _inputExtent;
+}
+
+- (NSNumber *)inputTime {
+ if (!_inputTime) {
+ _inputTime = @(0);
+ }
+ return _inputTime;
+}
+
+- (void)setDefaults {
+ self.inputExtent = nil;
+ self.inputTime = nil;
+}
+
+- (CIImage *)outputImage {
+ return [[YUCIBlobsGenerator filterKernel] applyWithExtent:self.inputExtent.CGRectValue
+ arguments:@[self.inputExtent,self.inputTime]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCICLAHE.cikernel b/Pods/Vivid/Sources/YUCICLAHE.cikernel
new file mode 100644
index 0000000..f764f41
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCICLAHE.cikernel
@@ -0,0 +1,42 @@
+
+#define YUCICLAHE_LOOKUP(LUTs, LUTIndex, value) \
+(sample(LUTs,samplerTransform(LUTs,vec2(value * 255.0 + 0.5 + samplerExtent(LUTs).x, LUTIndex + 0.5 + samplerExtent(LUTs).y))).r)
+
+kernel vec4 filterKernel(sampler inputImage, sampler LUTs, vec2 tileGridSize, vec2 tileSize) {
+ vec4 textureColor = sample(inputImage,samplerCoord(inputImage)); /* HSL Color */
+
+ vec2 coord = destCoord() - samplerExtent(inputImage).xy;
+
+ float txf = coord.x / tileSize.x - 0.5;
+
+ float tx1 = floor(txf);
+ float tx2 = tx1 + 1.0;
+
+ float xa_p = txf - tx1;
+ float xa1_p = 1.0 - xa_p;
+
+ tx1 = max(tx1, 0.0);
+ tx2 = min(tx2, tileGridSize.x - 1.0);
+
+ float tyf = coord.y / tileSize.y - 0.5;
+
+ float ty1 = floor(tyf);
+ float ty2 = ty1 + 1.0;
+
+ float ya = tyf - ty1;
+ float ya1 = 1.0 - ya;
+
+ ty1 = max(ty1, 0.0);
+ ty2 = min(ty2, tileGridSize.y - 1.0);
+
+ float srcVal = textureColor.b;
+
+ float lutPlane1_ind1 = YUCICLAHE_LOOKUP(LUTs, ty1 * tileGridSize.x + tx1, srcVal);
+ float lutPlane1_ind2 = YUCICLAHE_LOOKUP(LUTs, ty1 * tileGridSize.x + tx2, srcVal);
+ float lutPlane2_ind1 = YUCICLAHE_LOOKUP(LUTs, ty2 * tileGridSize.x + tx1, srcVal);
+ float lutPlane2_ind2 = YUCICLAHE_LOOKUP(LUTs, ty2 * tileGridSize.x + tx2, srcVal);
+
+ float res = (lutPlane1_ind1 * xa1_p + lutPlane1_ind2 * xa_p) * ya1 + (lutPlane2_ind1 * xa1_p + lutPlane2_ind2 * xa_p) * ya;
+
+ return vec4(vec3(textureColor.r, textureColor.g, clamp(res,0.0,1.0)),textureColor.a);
+}
diff --git a/Pods/Vivid/Sources/YUCICLAHE.h b/Pods/Vivid/Sources/YUCICLAHE.h
new file mode 100644
index 0000000..3f24ef8
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCICLAHE.h
@@ -0,0 +1,19 @@
+//
+// YUCICLAHE.h
+// Pods
+//
+// Created by YuAo on 2/16/16.
+//
+//
+
+#import
+
+@interface YUCICLAHE : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputClipLimit; //default 1.0;
+
+@property (nonatomic, copy, null_resettable) CIVector *inputTileGridSize; //default (x:8 y:8)
+
+@end
diff --git a/Pods/Vivid/Sources/YUCICLAHE.m b/Pods/Vivid/Sources/YUCICLAHE.m
new file mode 100644
index 0000000..6ac675b
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCICLAHE.m
@@ -0,0 +1,246 @@
+//
+// YUCICLAHE.m
+// Pods
+//
+// Created by YuAo on 2/16/16.
+//
+//
+
+#import "YUCICLAHE.h"
+#import "YUCIFilterConstructor.h"
+#import
+#import "YUCIReflectedTile.h"
+#import "YUCIUtilities.h"
+
+NSInteger const YUCICLAHEHistogramBinCount = 256;
+
+static NSData * YUCICLAHETransformLUTForContrastLimitedHistogram(vImagePixelCount histogram[YUCICLAHEHistogramBinCount], vImagePixelCount totalPixelCount) {
+ vImagePixelCount sum = 0;
+ uint8_t equalizationFunction[YUCICLAHEHistogramBinCount];
+ for (NSInteger index = 0; index < YUCICLAHEHistogramBinCount; ++index) {
+ sum += histogram[index];
+ equalizationFunction[index] = round(sum * (YUCICLAHEHistogramBinCount - 1) / (double)totalPixelCount);
+ }
+ NSData *data = [NSData dataWithBytes:equalizationFunction length:YUCICLAHEHistogramBinCount * sizeof(u_int8_t)];
+ return data;
+}
+
+@interface YUCICLAHE ()
+
+@property (nonatomic,strong) CIContext *context;
+
+@end
+
+@implementation YUCICLAHE
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCICLAHE class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryColorAdjustment],
+ kCIAttributeFilterDisplayName: @"Contrast Limited Adaptive Histogram Equalization"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCICLAHE class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
++ (CIColorKernel *)RGBToHSLKernel {
+ static CIColorKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:@"YUCIRGBToHSL" withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIColorKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
++ (CIColorKernel *)HSLToRGBKernel {
+ static CIColorKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:@"YUCIHSLToRGB" withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIColorKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (CIContext *)context {
+ if (!_context) {
+ _context = [CIContext contextWithOptions:@{kCIContextWorkingColorSpace: CFBridgingRelease(CGColorSpaceCreateWithName(kCGColorSpaceSRGB))}];
+ }
+ return _context;
+}
+
+- (NSNumber *)inputClipLimit {
+ if (!_inputClipLimit) {
+ _inputClipLimit = @(1.0);
+ }
+ return _inputClipLimit;
+}
+
+- (CIVector *)inputTileGridSize {
+ if (!_inputTileGridSize) {
+ _inputTileGridSize = [CIVector vectorWithX:8 Y:8];
+ }
+ return _inputTileGridSize;
+}
+
+- (void)setDefaults {
+ self.inputClipLimit = nil;
+ self.inputTileGridSize = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ /* Convert to HSL */
+ CIImage *inputImage = [[YUCICLAHE RGBToHSLKernel] applyWithExtent:self.inputImage.extent arguments:@[self.inputImage]];
+
+ /* Prepare */
+ NSInteger tileGridSizeX = self.inputTileGridSize.X;
+ NSInteger tileGridSizeY = self.inputTileGridSize.Y;
+
+ CIImage *inputImageForLUT;
+
+ if ((NSInteger)inputImage.extent.size.width % tileGridSizeX == 0 &&
+ (NSInteger)inputImage.extent.size.height % tileGridSizeY == 0) {
+ inputImageForLUT = inputImage;
+ } else {
+ NSInteger dY = (tileGridSizeY - ((NSInteger)inputImage.extent.size.height % tileGridSizeY)) % tileGridSizeY;
+ NSInteger dX = (tileGridSizeX - ((NSInteger)inputImage.extent.size.width % tileGridSizeX)) % tileGridSizeX;
+
+ inputImageForLUT = [inputImage imageByApplyingFilter:NSStringFromClass([YUCIReflectedTile class]) withInputParameters:@{@"inputMode": @(YUCIReflectedTileModeReflectWithoutBorder)}];
+ inputImageForLUT = [inputImageForLUT imageByCroppingToRect:CGRectMake(inputImage.extent.origin.x, inputImage.extent.origin.y, inputImage.extent.size.width + dX, inputImage.extent.size.height + dY)];
+ }
+
+ CGSize tileSize = CGSizeMake(inputImageForLUT.extent.size.width/tileGridSizeX, inputImageForLUT.extent.size.height/tileGridSizeY);
+
+ NSInteger clipLimit = 0;
+ if (self.inputClipLimit.doubleValue > 0.0) {
+ clipLimit = (NSInteger)(self.inputClipLimit.doubleValue * tileSize.width * tileSize.height / YUCICLAHEHistogramBinCount);
+ clipLimit = MAX(clipLimit, 1);
+ }
+
+ vImagePixelCount totalPixelCountPerTile = (vImagePixelCount)tileSize.width * (vImagePixelCount)tileSize.height;
+
+ /* Create LUTs */
+ NSUInteger numberOfLUTs = tileGridSizeX * tileGridSizeY;
+ NSUInteger byteCountPerLUT = YUCICLAHEHistogramBinCount * sizeof(u_int8_t);
+
+ NSMutableData *LUTsData = [NSMutableData dataWithLength:numberOfLUTs * byteCountPerLUT];
+ if (!LUTsData) {
+ NSLog(@"%@: failed to allocates memory for LUTs.",self);
+ return nil;
+ }
+
+ {
+ ptrdiff_t LUTRowBytes = tileSize.width * 4; // ARGB has 4 components
+ uint8_t *tileImageDataBuffer = calloc(LUTRowBytes * tileSize.height, sizeof(uint8_t)); // Buffer to render into
+ @YUCIDefer {
+ //defer free operation
+ if (tileImageDataBuffer) {
+ free(tileImageDataBuffer);
+ }
+ };
+
+ if (!tileImageDataBuffer) {
+ NSLog(@"%@: failed to allocates memory for tile buffer.",self);
+ return nil;
+ }
+
+ for (NSInteger tileIndex = 0; tileIndex < tileGridSizeX * tileGridSizeY; ++tileIndex) {
+ NSInteger colum = tileIndex % tileGridSizeX;
+ NSInteger row = tileIndex / tileGridSizeX;
+
+ CIImage *tile = [inputImageForLUT imageByCroppingToRect:CGRectMake(inputImageForLUT.extent.origin.x + colum * tileSize.width,
+ inputImageForLUT.extent.origin.y + row * tileSize.height,
+ tileSize.width,
+ tileSize.height)];
+
+ [self.context render:tile
+ toBitmap:tileImageDataBuffer
+ rowBytes:LUTRowBytes
+ bounds:tile.extent
+ format:kCIFormatARGB8
+ colorSpace:self.context.workingColorSpace];
+
+ vImage_Buffer vImageBuffer;
+ vImageBuffer.data = tileImageDataBuffer;
+ vImageBuffer.width = tile.extent.size.width;
+ vImageBuffer.height = tile.extent.size.height;
+ vImageBuffer.rowBytes = LUTRowBytes;
+
+ vImagePixelCount h[YUCICLAHEHistogramBinCount];
+ vImagePixelCount s[YUCICLAHEHistogramBinCount];
+ vImagePixelCount l[YUCICLAHEHistogramBinCount];
+ vImagePixelCount alpha[YUCICLAHEHistogramBinCount];
+ vImagePixelCount *histogram[4] = {alpha, h, s, l};
+
+ vImage_Error error = vImageHistogramCalculation_ARGB8888(&vImageBuffer, histogram, kvImageNoFlags);
+ if (error != kvImageNoError) {
+ NSLog(@"%@: failed to generate histogram. (vImage error: %@)",self,@(error));
+ return nil;
+ }
+
+ NSInteger histSize = YUCICLAHEHistogramBinCount;
+ vImagePixelCount clipped = 0;
+ for (NSInteger i = 0; i < histSize; ++i) {
+ if(l[i] > clipLimit) {
+ clipped += (l[i] - clipLimit);
+ l[i] = clipLimit;
+ };
+ }
+
+ vImagePixelCount redistBatch = clipped / histSize;
+ vImagePixelCount residual = clipped - redistBatch * histSize;
+
+ for (NSInteger i = 0; i < histSize; ++i) {
+ l[i] += redistBatch;
+ }
+
+ for (NSInteger i = 0; i < residual; ++i) {
+ l[i]++;
+ }
+
+ NSData *tileLUTData = YUCICLAHETransformLUTForContrastLimitedHistogram(l, totalPixelCountPerTile);
+
+ //using (numberOfLUTs - tileIndex - 1) * byteCountPerLUT as insert location for core image's y-inverted coordinate system.
+ [LUTsData replaceBytesInRange:NSMakeRange((numberOfLUTs - tileIndex - 1) * byteCountPerLUT, byteCountPerLUT) withBytes:tileLUTData.bytes];
+ }
+ }
+
+ CIImage *LUTs = [CIImage imageWithBitmapData:LUTsData
+ bytesPerRow:YUCICLAHEHistogramBinCount * sizeof(uint8_t)
+ size:CGSizeMake(YUCICLAHEHistogramBinCount, numberOfLUTs)
+ format:kCIFormatR8
+ colorSpace:self.context.workingColorSpace];
+
+ /* Apply & Interpolation */
+ CIImage *equalizedImage = [[YUCICLAHE filterKernel] applyWithExtent:inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ if (index == 1) {
+ return LUTs.extent;
+ } else {
+ return destRect;
+ }
+ } arguments:@[inputImage,LUTs,[CIVector vectorWithX:tileGridSizeX Y:tileGridSizeY],[CIVector vectorWithX:tileSize.width Y:tileSize.height]]];
+ /* Back to RGB */
+ return [[YUCICLAHE HSLToRGBKernel] applyWithExtent:equalizedImage.extent arguments:@[equalizedImage]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIColorLookup.cikernel b/Pods/Vivid/Sources/YUCIColorLookup.cikernel
new file mode 100644
index 0000000..5656c4d
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIColorLookup.cikernel
@@ -0,0 +1,34 @@
+
+kernel vec4 filterKernel(sampler inputImage, sampler inputLUT, float intensity) {
+ vec4 textureColor = sample(inputImage,samplerCoord(inputImage));
+ textureColor = clamp(textureColor, vec4(0.0), vec4(1.0));
+
+ float blueColor = textureColor.b * 63.0;
+
+ vec2 quad1;
+ quad1.y = floor(floor(blueColor) / 8.0);
+ quad1.x = floor(blueColor) - (quad1.y * 8.0);
+
+ vec2 quad2;
+ quad2.y = floor(ceil(blueColor) / 8.0);
+ quad2.x = ceil(blueColor) - (quad2.y * 8.0);
+
+ vec2 texPos1;
+ texPos1.x = (quad1.x * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.r);
+ texPos1.y = (quad1.y * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.g);
+
+ vec2 texPos2;
+ texPos2.x = (quad2.x * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.r);
+ texPos2.y = (quad2.y * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.g);
+
+ texPos1.y = 1.0 - texPos1.y;
+ texPos2.y = 1.0 - texPos2.y;
+
+ vec4 inputLUTExtent = samplerExtent(inputLUT);
+
+ vec4 newColor1 = sample(inputLUT, samplerTransform(inputLUT, texPos1 * vec2(512.0) + inputLUTExtent.xy));
+ vec4 newColor2 = sample(inputLUT, samplerTransform(inputLUT, texPos2 * vec2(512.0) + inputLUTExtent.xy));
+
+ vec4 newColor = mix(newColor1, newColor2, fract(blueColor));
+ return mix(textureColor, vec4(newColor.rgb, textureColor.a), intensity);
+}
diff --git a/Pods/Vivid/Sources/YUCIColorLookup.h b/Pods/Vivid/Sources/YUCIColorLookup.h
new file mode 100644
index 0000000..49392ba
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIColorLookup.h
@@ -0,0 +1,26 @@
+//
+// YUCIColorLookupFilter.h
+// CoreImageSkinEnhancementFilter
+//
+// Created by YuAo on 1/20/16.
+// Copyright © 2016 YuAo. All rights reserved.
+//
+
+#import
+
+/*
+ Note:
+
+ Requires CIContext object with a sRGB working color space instead of the default light-linear color space.
+
+ */
+
+@interface YUCIColorLookup : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, strong, null_resettable) CIImage *inputColorLookupTable;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputIntensity;
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIColorLookup.m b/Pods/Vivid/Sources/YUCIColorLookup.m
new file mode 100644
index 0000000..d9fb46a
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIColorLookup.m
@@ -0,0 +1,73 @@
+//
+// YUCIColorLookupFilter.m
+// CoreImageSkinEnhancementFilter
+//
+// Created by YuAo on 1/20/16.
+// Copyright © 2016 YuAo. All rights reserved.
+//
+
+#import "YUCIColorLookup.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIColorLookup
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIColorLookup class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryColorEffect,kCICategoryInterlaced,kCICategoryNonSquarePixels],
+ kCIAttributeFilterDisplayName: @"Color Lookup"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIColorLookup class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputIntensity {
+ if (!_inputIntensity) {
+ _inputIntensity = @(1.0);
+ }
+ return _inputIntensity;
+}
+
+- (CIImage *)inputColorLookupTable {
+ if (!_inputColorLookupTable) {
+ _inputColorLookupTable = [CIImage imageWithContentsOfURL:[[NSBundle bundleForClass:self.class] URLForResource:@"YUCIColorLookupTableDefault" withExtension:@"png"]];
+ }
+ return _inputColorLookupTable;
+}
+
+- (void)setDefaults {
+ self.inputIntensity = nil;
+ self.inputColorLookupTable = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ return [[YUCIColorLookup filterKernel] applyWithExtent:self.inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ if (index == 0) {
+ return destRect;
+ } else {
+ return self.inputColorLookupTable.extent;
+ }
+ }
+ arguments:@[self.inputImage,self.inputColorLookupTable,self.inputIntensity]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIColorLookupTableDefault.png b/Pods/Vivid/Sources/YUCIColorLookupTableDefault.png
new file mode 100644
index 0000000..ed814df
Binary files /dev/null and b/Pods/Vivid/Sources/YUCIColorLookupTableDefault.png differ
diff --git a/Pods/Vivid/Sources/YUCICrossZoomTransition.cikernel b/Pods/Vivid/Sources/YUCICrossZoomTransition.cikernel
new file mode 100644
index 0000000..2e11d7c
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCICrossZoomTransition.cikernel
@@ -0,0 +1,58 @@
+
+const float PI = 3.141592653589793;
+
+float linearEase(float begin, float change, float duration, float time) {
+ return change * time / duration + begin;
+}
+
+float exponentialEaseInOut(float begin, float change, float duration, float time) {
+ if (time == 0.0) {
+ return begin;
+ } else if (time == duration) {
+ return begin + change;
+ }
+ time = time / (duration / 2.0);
+ if (time < 1.0) {
+ return change / 2.0 * pow(2.0, 10.0 * (time - 1.0)) + begin;
+ } else {
+ return change / 2.0 * (-pow(2.0, -10.0 * (time - 1.0)) + 2.0) + begin;
+ }
+ }
+
+float sinusoidalEaseInOut(float begin, float change, float duration, float time) {
+ return -change / 2.0 * (cos(PI * time / duration) - 1.0) + begin;
+}
+
+/* random number between 0 and 1 */
+float random(vec3 scale, float seed) {
+ /* use the fragment position for randomness */
+ return fract(sin(dot(gl_FragCoord.xyz + seed, scale)) * 43758.5453 + seed);
+}
+
+kernel vec4 filterKernel(sampler inputImage, sampler inputTargetImage, float inputStrength, vec4 inputExtent, float progress) {
+ // Linear interpolate center across center half of the image
+ vec2 center = vec2(linearEase(0.25, 0.5, 1.0, progress), 0.5);
+ float dissolve = exponentialEaseInOut(0.0, 1.0, 1.0, progress);
+
+ // Mirrored sinusoidal loop. 0->strength then strength->0
+ float strength = sinusoidalEaseInOut(0.0, inputStrength, 0.5, progress);
+
+ vec4 color = vec4(0.0);
+ float total = 0.0;
+ vec2 textureCoordinate = ((destCoord() - inputExtent.xy)/inputExtent.zw);
+ vec2 toCenter = center - textureCoordinate;
+
+ /* randomize the lookup values to hide the fixed number of samples */
+ float offset = random(vec3(12.9898, 78.233, 151.7182), 0.0);
+
+ for (float t = 0.0; t <= 10.0; t++) {
+ float percent = (t + offset) / 10.0;
+ float weight = 4.0 * (percent - percent * percent);
+
+ vec2 uv = (textureCoordinate + toCenter * percent * strength) * inputExtent.zw + inputExtent.xy;
+ vec4 crossFade = mix(sample(inputImage, samplerTransform(inputImage,uv)), sample(inputTargetImage, samplerTransform(inputTargetImage,uv)), dissolve);
+ color += crossFade * weight;
+ total += weight;
+ }
+ return color/total;
+}
diff --git a/Pods/Vivid/Sources/YUCICrossZoomTransition.h b/Pods/Vivid/Sources/YUCICrossZoomTransition.h
new file mode 100644
index 0000000..aa67347
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCICrossZoomTransition.h
@@ -0,0 +1,22 @@
+//
+// YUCICrossZoomTransition.h
+// Pods
+//
+// Created by YuAo on 2/4/16.
+//
+//
+
+#import
+
+@interface YUCICrossZoomTransition : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+@property (nonatomic, strong, nullable) CIImage *inputTargetImage;
+
+@property (nonatomic, copy, nullable) CIVector *inputExtent;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputStrength; //default 0.3
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputTime; /* 0 to 1 */
+
+@end
diff --git a/Pods/Vivid/Sources/YUCICrossZoomTransition.m b/Pods/Vivid/Sources/YUCICrossZoomTransition.m
new file mode 100644
index 0000000..f2ee2df
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCICrossZoomTransition.m
@@ -0,0 +1,71 @@
+//
+// YUCICrossZoomTransition.m
+// Pods
+//
+// Created by YuAo on 2/4/16.
+//
+//
+
+#import "YUCICrossZoomTransition.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCICrossZoomTransition
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCICrossZoomTransition class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryTransition],
+ kCIAttributeFilterDisplayName: @"Cross Zoom Transition"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCICrossZoomTransition class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputStrength {
+ if (!_inputStrength) {
+ _inputStrength = @(0.3);
+ }
+ return _inputStrength;
+}
+
+- (NSNumber *)inputTime {
+ if (!_inputTime) {
+ _inputTime = @(0.0);
+ }
+ return _inputTime;
+}
+
+- (void)setDefaults {
+ self.inputStrength = nil;
+ self.inputTime = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage || !self.inputTargetImage) {
+ return nil;
+ }
+
+ CIVector *defaultInputExtent = [CIVector vectorWithCGRect:CGRectUnion(self.inputImage.extent, self.inputTargetImage.extent)];
+ CIVector *extent = self.inputExtent?:defaultInputExtent;
+ return [[YUCICrossZoomTransition filterKernel] applyWithExtent:extent.CGRectValue
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return extent.CGRectValue;
+ }
+ arguments:@[self.inputImage,self.inputTargetImage,self.inputStrength,extent,self.inputTime]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFXAA.cikernel b/Pods/Vivid/Sources/YUCIFXAA.cikernel
new file mode 100644
index 0000000..91211c2
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFXAA.cikernel
@@ -0,0 +1,55 @@
+
+#define YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, coord) \
+(sample(tex, samplerTransform(tex, coord)))
+
+kernel vec4 filterKernel(sampler tex) {
+ vec2 fragCoord = destCoord();
+ vec2 v_rgbNW = (fragCoord + vec2(-1.0, -1.0));
+ vec2 v_rgbNE = (fragCoord + vec2(1.0, -1.0));
+ vec2 v_rgbSW = (fragCoord + vec2(-1.0, 1.0));
+ vec2 v_rgbSE = (fragCoord + vec2(1.0, 1.0));
+ vec2 v_rgbM = fragCoord;
+
+ const float FXAA_REDUCE_MIN = (1.0/ 128.0);
+ const float FXAA_REDUCE_MUL = (1.0 / 8.0);
+ const float FXAA_SPAN_MAX = 8.0;
+
+ vec4 color;
+ vec3 rgbNW = YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, v_rgbNW).xyz;
+ vec3 rgbNE = YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, v_rgbNE).xyz;
+ vec3 rgbSW = YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, v_rgbSW).xyz;
+ vec3 rgbSE = YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, v_rgbSE).xyz;
+ vec4 texColor = YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, v_rgbM);
+ vec3 rgbM = texColor.xyz;
+ vec3 luma = vec3(0.299, 0.587, 0.114);
+ float lumaNW = dot(rgbNW, luma);
+ float lumaNE = dot(rgbNE, luma);
+ float lumaSW = dot(rgbSW, luma);
+ float lumaSE = dot(rgbSE, luma);
+ float lumaM = dot(rgbM, luma);
+ float lumaMin = min(lumaM, min(min(lumaNW, lumaNE), min(lumaSW, lumaSE)));
+ float lumaMax = max(lumaM, max(max(lumaNW, lumaNE), max(lumaSW, lumaSE)));
+
+ vec2 dir;
+ dir.x = -((lumaNW + lumaNE) - (lumaSW + lumaSE));
+ dir.y = ((lumaNW + lumaSW) - (lumaNE + lumaSE));
+
+ float dirReduce = max((lumaNW + lumaNE + lumaSW + lumaSE) *
+ (0.25 * FXAA_REDUCE_MUL), FXAA_REDUCE_MIN);
+
+ float rcpDirMin = 1.0 / (min(abs(dir.x), abs(dir.y)) + dirReduce);
+ dir = min(vec2(FXAA_SPAN_MAX, FXAA_SPAN_MAX),
+ max(vec2(-FXAA_SPAN_MAX, -FXAA_SPAN_MAX),
+ dir * rcpDirMin));
+
+ vec3 rgbA = 0.5 * (YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, fragCoord + dir * (1.0 / 3.0 - 0.5)).xyz + YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, fragCoord + dir * (2.0 / 3.0 - 0.5)).xyz);
+
+ vec3 rgbB = rgbA * 0.5 + 0.25 * (YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, fragCoord + dir * -0.5).xyz + YUCIFXAA_SAMPLE_TEXTURE_AT_LOCATION(tex, fragCoord + dir * 0.5).xyz);
+
+ float lumaB = dot(rgbB, luma);
+ if ((lumaB < lumaMin) || (lumaB > lumaMax))
+ color = vec4(rgbA, texColor.a);
+ else
+ color = vec4(rgbB, texColor.a);
+ return color;
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIFXAA.h b/Pods/Vivid/Sources/YUCIFXAA.h
new file mode 100644
index 0000000..1ffcafc
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFXAA.h
@@ -0,0 +1,15 @@
+//
+// YUCIFXAA.h
+// Pods
+//
+// Created by YuAo on 2/14/16.
+//
+//
+
+#import
+
+@interface YUCIFXAA : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFXAA.m b/Pods/Vivid/Sources/YUCIFXAA.m
new file mode 100644
index 0000000..09ed892
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFXAA.m
@@ -0,0 +1,49 @@
+//
+// YUCIFXAA.m
+// Pods
+//
+// Created by YuAo on 2/14/16.
+//
+//
+
+#import "YUCIFXAA.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIFXAA
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIFXAA class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo],
+ kCIAttributeFilterDisplayName: @"Fast Approximate Anti-Aliasing"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIFXAA class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ return [[YUCIFXAA filterKernel] applyWithExtent:self.inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return CGRectInset(destRect, -8, -8); //FXAA_SPAN_MAX
+ } arguments:@[self.inputImage.imageByClampingToExtent]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFilmBurnTransition.cikernel b/Pods/Vivid/Sources/YUCIFilmBurnTransition.cikernel
new file mode 100644
index 0000000..8969bd5
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilmBurnTransition.cikernel
@@ -0,0 +1,81 @@
+
+const float pi = 3.141592653589793;
+
+const float Seed = 2.31;
+
+const float FPS = 40.0;
+
+float sigmoid(float x, float a) {
+ float b = pow(x*2.,a)/2.;
+ if (x > .5) {
+ b = 1.-pow(2.-(x*2.),a)/2.;
+ }
+ return b;
+}
+
+float rand(float co){
+ return fract(sin((co*24.9898)+Seed)*43758.5453);
+}
+
+float rand(vec2 co){
+ return fract(sin(dot(co.xy ,vec2(12.9898,78.233))) * 43758.5453);
+}
+
+float apow(float a,float b) {
+ return pow(abs(a),b)*sign(b);
+}
+
+vec3 pow3(vec3 a,vec3 b) {
+ return vec3(apow(a.r,b.r),apow(a.g,b.g),apow(a.b,b.b));
+}
+
+float smooth_mix(float a,float b,float c) {
+ return mix(a,b,sigmoid(c,2.));
+}
+
+float random(vec2 co, float shft){
+ co += 10.;
+ return smooth_mix(fract(sin(dot(co.xy ,vec2(12.9898+(floor(shft)*.5),78.233+Seed))) * 43758.5453),fract(sin(dot(co.xy ,vec2(12.9898+(floor(shft+1.)*.5),78.233+Seed))) * 43758.5453),fract(shft));
+}
+
+float smooth_random(vec2 co, float shft) {
+ return smooth_mix(smooth_mix(random(floor(co),shft),random(floor(co+vec2(1.,0.)),shft),fract(co.x)),smooth_mix(random(floor(co+vec2(0.,1.)),shft),random(floor(co+vec2(1.,1.)),shft),fract(co.x)),fract(co.y));
+}
+
+vec4 sampleTexture(vec2 p, sampler from, sampler to, float progress) {
+ return mix(sample(from, samplerTransform(from,p)), sample(to, samplerTransform(to,p)), sigmoid(progress,10.));
+}
+
+#define clamps(x) clamp(x,0.,1.)
+
+kernel vec4 filterKernel(sampler inputImage, sampler inputTargetImage, vec4 inputExtent, float progress) {
+ vec2 textureCoordinate = ((destCoord() - inputExtent.xy)/inputExtent.zw);
+ vec2 p = textureCoordinate;
+ vec3 f = vec3(0.);
+ for (float i = 0.; i < 13.; i++) {
+ f += sin(((p.x*rand(i)*6.)+(progress*8.))+rand(i+1.43))*sin(((p.y*rand(i+4.4)*6.)+(progress*6.))+rand(i+2.4));
+ f += 1.-clamps(length(p-vec2(smooth_random(vec2(progress*1.3),i+1.),smooth_random(vec2(progress*.5),i+6.25)))*mix(20.,70.,rand(i)));
+ }
+ f += 4.;
+ f /= 11.;
+ f = pow3(f*vec3(1.,0.7,0.6),vec3(1.,2.-sin(progress*pi),1.3));
+ f *= sin(progress*pi);
+
+ p -= .5;
+ p *= 1.+(smooth_random(vec2(progress*5.),6.3)*sin(progress*pi)*.05);
+ p += .5;
+
+ vec4 blurred_image = vec4(0.);
+ float bluramount = sin(progress*pi)*.03;
+
+#define repeats 30.
+ for (float i = 0.; i < repeats; i++) {
+ vec2 q = vec2(cos(degrees((i/repeats)*360.)),sin(degrees((i/repeats)*360.))) * (rand(vec2(i,p.x+p.y))+bluramount);
+ vec2 uv2 = p+(q*bluramount);
+ uv2 = uv2 * inputExtent.zw + inputExtent.xy;
+ blurred_image += sampleTexture(uv2, inputImage, inputTargetImage, progress);
+ }
+ blurred_image /= repeats;
+
+ return blurred_image+vec4(f,0.);
+}
diff --git a/Pods/Vivid/Sources/YUCIFilmBurnTransition.h b/Pods/Vivid/Sources/YUCIFilmBurnTransition.h
new file mode 100644
index 0000000..3c31d30
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilmBurnTransition.h
@@ -0,0 +1,20 @@
+//
+// YUCIFilmBurnTransition.h
+// Pods
+//
+// Created by YuAo on 22/05/2017.
+//
+//
+
+#import
+
+@interface YUCIFilmBurnTransition : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+@property (nonatomic, strong, nullable) CIImage *inputTargetImage;
+
+@property (nonatomic, copy, nullable) CIVector *inputExtent;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputTime; /* 0 to 1 */
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFilmBurnTransition.m b/Pods/Vivid/Sources/YUCIFilmBurnTransition.m
new file mode 100644
index 0000000..5c771eb
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilmBurnTransition.m
@@ -0,0 +1,64 @@
+//
+// YUCIFilmBurnTransition.m
+// Pods
+//
+// Created by YuAo on 22/05/2017.
+//
+//
+
+#import "YUCIFilmBurnTransition.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIFilmBurnTransition
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIFilmBurnTransition class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryTransition],
+ kCIAttributeFilterDisplayName: @"Film Burn Transition"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIFilmBurnTransition class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputTime {
+ if (!_inputTime) {
+ _inputTime = @(0.0);
+ }
+ return _inputTime;
+}
+
+- (void)setDefaults {
+ self.inputExtent = nil;
+ self.inputTime = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage || !self.inputTargetImage) {
+ return nil;
+ }
+
+ CIVector *defaultInputExtent = [CIVector vectorWithCGRect:CGRectUnion(self.inputImage.extent, self.inputTargetImage.extent)];
+ CIVector *extent = self.inputExtent?:defaultInputExtent;
+ return [[YUCIFilmBurnTransition filterKernel] applyWithExtent:extent.CGRectValue
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return extent.CGRectValue;
+ }
+ arguments:@[self.inputImage,self.inputTargetImage,extent,self.inputTime]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFilterConstructor.h b/Pods/Vivid/Sources/YUCIFilterConstructor.h
new file mode 100644
index 0000000..f155ac6
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilterConstructor.h
@@ -0,0 +1,25 @@
+//
+// YUCIFilterConstructor.h
+// Pods
+//
+// Created by YuAo on 1/23/16.
+//
+//
+
+#import
+#import
+
+NS_ASSUME_NONNULL_BEGIN
+/*
+ Using class with name `filterName` to construct a filter object.
+ */
+
+@interface YUCIFilterConstructor : NSObject
+
++ (instancetype)constructor;
+
+- (instancetype)init NS_UNAVAILABLE;
+
+@end
+
+NS_ASSUME_NONNULL_END
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIFilterConstructor.m b/Pods/Vivid/Sources/YUCIFilterConstructor.m
new file mode 100644
index 0000000..c7274f3
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilterConstructor.m
@@ -0,0 +1,33 @@
+//
+// YUCIFilterConstructor.m
+// Pods
+//
+// Created by YuAo on 1/23/16.
+//
+//
+
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIFilterConstructor
+
++ (instancetype)constructor {
+ static YUCIFilterConstructor *constructor;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ constructor = [[YUCIFilterConstructor alloc] initForSharedConstructor];
+ });
+ return constructor;
+}
+
+- (instancetype)initForSharedConstructor {
+ if (self = [super init]) {
+
+ }
+ return self;
+}
+
+- (CIFilter *)filterWithName:(NSString *)name {
+ return [[NSClassFromString(name) alloc] init];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFilterPreviewGenerator.h b/Pods/Vivid/Sources/YUCIFilterPreviewGenerator.h
new file mode 100644
index 0000000..43f8f7f
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilterPreviewGenerator.h
@@ -0,0 +1,22 @@
+//
+// YUCIFilterPreviewGenerator.h
+// Pods
+//
+// Created by YuAo on 2/14/16.
+//
+//
+
+#import
+#import
+
+NS_ASSUME_NONNULL_BEGIN
+
+@interface YUCIFilterPreviewGenerator : NSObject
+
++ (void)generatePreviewForFilter:(CIFilter *)filter
+ context:(nullable CIContext *)context
+ completion:(void (^)(NSData *previewData, NSString *preferredFilename))completion;
+
+@end
+
+NS_ASSUME_NONNULL_END
diff --git a/Pods/Vivid/Sources/YUCIFilterPreviewGenerator.m b/Pods/Vivid/Sources/YUCIFilterPreviewGenerator.m
new file mode 100644
index 0000000..6e9e3e6
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilterPreviewGenerator.m
@@ -0,0 +1,205 @@
+//
+// YUCIFilterPreviewGenerator.m
+// Pods
+//
+// Created by YuAo on 2/14/16.
+//
+//
+
+#import "YUCIFilterPreviewGenerator.h"
+#import
+#if __has_include()
+ #import
+#endif
+
+CGSize const YUCIFilterPreviewImageSize = (CGSize){280,210};
+CGFloat const YUCIFilterPreviewImageSpacing = 60;
+CGFloat const YUCIFilterPreviewAreaEdgeInset = 0;
+
+CGRect YUCIMakeRectWithAspectRatioFillRect(CGSize aspectRatio, CGRect boundingRect) {
+ if (CGRectIsInfinite(boundingRect)) {
+ boundingRect = CGRectMake(0, 0, 1600, 1200);
+ }
+ CGFloat horizontalRatio = boundingRect.size.width / aspectRatio.width;
+ CGFloat verticalRatio = boundingRect.size.height / aspectRatio.height;
+ CGFloat ratio;
+
+ ratio = MAX(horizontalRatio, verticalRatio);
+ //ratio = MIN(horizontalRatio, verticalRatio);
+
+ CGSize newSize = CGSizeMake(floor(aspectRatio.width * ratio), floor(aspectRatio.height * ratio));
+ CGRect rect = CGRectMake(boundingRect.origin.x + (boundingRect.size.width - newSize.width)/2, boundingRect.origin.y + (boundingRect.size.height - newSize.height)/2, newSize.width, newSize.height);
+ return rect;
+}
+
+@implementation YUCIFilterPreviewGenerator
+
++ (void)generatePreviewForFilter:(CIFilter *)filter context:(CIContext *)inputContext completion:(void (^)(NSData *, NSString *))completion {
+
+ CIContext *context = inputContext;
+ if (!context) {
+ context = [CIContext contextWithOptions:@{kCIContextWorkingColorSpace: CFBridgingRelease(CGColorSpaceCreateWithName(kCGColorSpaceSRGB))}];
+ }
+
+ NSArray *categories = filter.attributes[kCIAttributeFilterCategories];
+ if([categories containsObject:kCICategoryTransition]) {
+ //transition
+ NSData *data = [self generateGIFPreviewForFilter:filter context:context duration:2.0 frameCallback:^(NSTimeInterval frameTime) {
+ [filter setValue:@(frameTime/2.0) forKey:@"inputTime"];
+ }];
+ completion(data,[filter.name stringByAppendingPathExtension:@"gif"]);
+ } else if ([categories containsObject:kCICategoryGenerator] && [filter.inputKeys containsObject:@"inputTime"]) {
+ //animatable generator
+ NSData *data = [self generateGIFPreviewForFilter:filter context:context duration:5.0 frameCallback:^(NSTimeInterval frameTime) {
+ [filter setValue:@(frameTime) forKey:@"inputTime"];
+ }];
+ completion(data,[filter.name stringByAppendingPathExtension:@"gif"]);
+ } else {
+ NSMutableArray *inputImageKeys = [NSMutableArray array];
+ for (NSString *inputKey in filter.inputKeys) {
+ id value = [filter valueForKey:inputKey];
+ if ([value isKindOfClass:[CIImage class]]) {
+ if ([inputKey isEqualToString:kCIInputImageKey]) {
+ [inputImageKeys insertObject:inputKey atIndex:0];
+ } else {
+ [inputImageKeys addObject:inputKey];
+ }
+ }
+ }
+ NSMutableData *data = [NSMutableData data];
+ CGImageDestinationRef destination = CGImageDestinationCreateWithData((CFMutableDataRef)data, kUTTypePNG, 1, NULL);
+ //NSDictionary *properties = @{(NSString *)kCGImageDestinationLossyCompressionQuality: @(0.75)};
+ //CGImageDestinationSetProperties(destination,(CFDictionaryRef)properties);
+ CGImageRef image = [self generatePreviewForFilter:filter context:context inputImageKeys:inputImageKeys];
+ CGImageDestinationAddImage(destination, image, NULL);
+ CGImageDestinationFinalize(destination);
+ CFRelease(destination);
+ completion(data,[filter.name stringByAppendingPathExtension:@"png"]);
+ }
+}
+
++ (NSData *)generateGIFPreviewForFilter:(CIFilter *)filter context:(CIContext *)context duration:(NSTimeInterval)duration frameCallback:(void (^)(NSTimeInterval frameTime))frameCallback {
+ NSInteger framePerSecond = 30;
+ NSMutableData *data = [NSMutableData data];
+ CGImageDestinationRef destination = CGImageDestinationCreateWithData((CFMutableDataRef)data, kUTTypeGIF, (size_t)floor(framePerSecond * duration), NULL);
+ for (NSInteger frame = 0; frame < framePerSecond * duration; ++frame) {
+ frameCallback(frame/(double)framePerSecond);
+ CGImageRef image = [self generatePreviewForFilter:filter context:context inputImageKeys:nil];
+ NSDictionary *frameProperties = @{(NSString *)kCGImagePropertyGIFDictionary:
+ @{(NSString *)kCGImagePropertyGIFDelayTime: @(1.0/framePerSecond)}
+ };
+ CGImageDestinationAddImage(destination, image, (CFDictionaryRef)frameProperties);
+ }
+ NSDictionary *gifProperties = @{(NSString *)kCGImagePropertyGIFDictionary:
+ @{(NSString *)kCGImagePropertyGIFLoopCount: @(0)}
+ };
+ CGImageDestinationSetProperties(destination, (CFDictionaryRef)gifProperties);
+ CGImageDestinationFinalize(destination);
+ CFRelease(destination);
+ return data.copy;
+}
+
++ (void)drawPlusSignInRect:(CGRect)rect context:(CGContextRef)context {
+ CGFloat size = MIN(CGRectGetWidth(rect), CGRectGetHeight(rect));
+ CGPoint center = CGPointMake(CGRectGetMidX(rect), CGRectGetMidY(rect));
+ CGMutablePathRef path = CGPathCreateMutable();
+ CGPathMoveToPoint(path, NULL, center.x - size/2.0, center.y);
+ CGPathAddLineToPoint(path, NULL, center.x + size/2.0, center.y);
+ CGPathMoveToPoint(path, NULL, center.x, center.y - size/2.0);
+ CGPathAddLineToPoint(path, NULL, center.x, center.y + size/2.0);
+ CGContextAddPath(context, path);
+ CGContextStrokePath(context);
+ CGPathRelease(path);
+}
+
++ (void)drawArrowSignInRect:(CGRect)rect context:(CGContextRef)context {
+ CGFloat size = MIN(CGRectGetWidth(rect), CGRectGetHeight(rect))/2.0;
+ CGPoint center = CGPointMake(CGRectGetMidX(rect), CGRectGetMidY(rect));
+ CGMutablePathRef path = CGPathCreateMutable();
+ CGPathMoveToPoint(path, NULL, center.x - size/2.0, center.y - size);
+ CGPathAddLineToPoint(path, NULL, center.x + size/2.0, center.y);
+ CGPathAddLineToPoint(path, NULL, center.x - size/2.0, center.y + size);
+ CGContextAddPath(context, path);
+ CGContextStrokePath(context);
+ CGPathRelease(path);
+}
+
++ (CGImageRef)generatePreviewForFilter:(CIFilter *)filter context:(CIContext *)context inputImageKeys:(NSArray *)inputImageKeys {
+ //filter
+ NSMutableArray *inputImages = [NSMutableArray array];
+ for (NSString *inputKey in inputImageKeys) {
+ id value = [filter valueForKey:inputKey];
+ if ([value isKindOfClass:[CIImage class]]) {
+ [inputImages addObject:value];
+ }
+ }
+
+ CGRect previewRect = CGRectMake(0, 0, (inputImages.count + 1) * YUCIFilterPreviewImageSize.width + inputImages.count * YUCIFilterPreviewImageSpacing + YUCIFilterPreviewAreaEdgeInset * 2.0, YUCIFilterPreviewImageSize.height + YUCIFilterPreviewAreaEdgeInset * 2.0);
+
+ CGImageRef image = [self imageByPerformingDrawing:^(CGContextRef cgContext) {
+ CGContextSetLineWidth(cgContext, 5);
+ CGFloat color[4] = {0.7,0.7,0.7,1.0};
+ CGContextSetStrokeColor(cgContext, color);
+ CGContextSetLineJoin(cgContext, kCGLineJoinRound);
+
+ CIImage *backgroundImage = [CIImage imageWithColor:[CIColor colorWithRed:0 green:0 blue:0 alpha:0.05]];
+ CGFloat x = YUCIFilterPreviewAreaEdgeInset;
+ for (CIImage *inputImage in inputImages) {
+ CGImageRef inputCGImage = [context createCGImage:[inputImage imageByCompositingOverImage:backgroundImage] fromRect:YUCIMakeRectWithAspectRatioFillRect(YUCIFilterPreviewImageSize, inputImage.extent)];
+ CGContextDrawImage(cgContext, (CGRect){{x,YUCIFilterPreviewAreaEdgeInset},YUCIFilterPreviewImageSize}, inputCGImage);
+ CGImageRelease(inputCGImage);
+
+ x += YUCIFilterPreviewImageSize.width;
+
+ CGRect spacingRect = CGRectInset(CGRectMake(x, YUCIFilterPreviewAreaEdgeInset, YUCIFilterPreviewImageSpacing, YUCIFilterPreviewImageSize.height), 15, 15);
+ if (inputImage == inputImages.lastObject) {
+ //>
+ [self drawArrowSignInRect:spacingRect context:cgContext];
+ } else {
+ //+
+ [self drawPlusSignInRect:spacingRect context:cgContext];
+ }
+
+ x += YUCIFilterPreviewImageSpacing;
+ }
+
+ {
+ //render output image
+ CGImageRef inputCGImage = [context createCGImage:[filter.outputImage imageByCompositingOverImage:backgroundImage] fromRect:YUCIMakeRectWithAspectRatioFillRect(YUCIFilterPreviewImageSize, filter.outputImage.extent)];
+ CGContextDrawImage(cgContext, (CGRect){{x,YUCIFilterPreviewAreaEdgeInset},YUCIFilterPreviewImageSize}, inputCGImage);
+ CGImageRelease(inputCGImage);
+ }
+ } inRect:previewRect];
+
+ return image;
+}
+
++ (CGImageRef)imageByPerformingDrawing:(void (^)(CGContextRef context))drawing inRect:(CGRect)rect {
+ // Build a context that's the same dimensions as the new size
+ CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB();
+ CGBitmapInfo bitmapInfo = kCGImageAlphaPremultipliedFirst | kCGBitmapByteOrder32Host;
+
+ CGContextRef bitmap = CGBitmapContextCreate(NULL,
+ rect.size.width,
+ rect.size.height,
+ 8,
+ 0,
+ colorSpace,
+ bitmapInfo);
+ // Set the quality level to use when rescaling
+ CGContextSetInterpolationQuality(bitmap, kCGInterpolationMedium);
+
+ drawing(bitmap);
+
+ // Get the resized image from the context and a UIImage
+ CGImageRef newImageRef = CGBitmapContextCreateImage(bitmap);
+
+ // Clean up
+ CFAutorelease(newImageRef);
+ CGColorSpaceRelease(colorSpace);
+ CGContextRelease(bitmap);
+
+ return newImageRef;
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFilterUtilities.h b/Pods/Vivid/Sources/YUCIFilterUtilities.h
new file mode 100644
index 0000000..1f62046
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilterUtilities.h
@@ -0,0 +1,11 @@
+//
+// YUCIFilterUtilities.h
+// Pods
+//
+// Created by YuAo on 2/3/16.
+//
+//
+
+#import
+
+FOUNDATION_EXPORT double YUCIGaussianDistributionPDF(double x, double sigma);
diff --git a/Pods/Vivid/Sources/YUCIFilterUtilities.m b/Pods/Vivid/Sources/YUCIFilterUtilities.m
new file mode 100644
index 0000000..001d0bd
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFilterUtilities.m
@@ -0,0 +1,13 @@
+//
+// YUCIFilterUtilities.m
+// Pods
+//
+// Created by YuAo on 2/3/16.
+//
+//
+
+#import "YUCIFilterUtilities.h"
+
+double YUCIGaussianDistributionPDF(double x, double sigma) {
+ return 1.0/sqrt(2 * M_PI * sigma * sigma) * exp((- x * x) / (2 * sigma * sigma));
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIFlashTransition.cikernel b/Pods/Vivid/Sources/YUCIFlashTransition.cikernel
new file mode 100644
index 0000000..c7ccb64
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFlashTransition.cikernel
@@ -0,0 +1,15 @@
+const vec3 flashColor = vec3(1.0, 0.8, 0.3);
+const float flashVelocity = 3.0;
+
+kernel vec4 filterKernel(__sample inputImage, __sample inputTargetImage,
+float flashPhase,
+float flashIntensity,
+float flashZoomEffect,
+vec4 inputExtent, float progress)
+{
+ vec2 p = (destCoord() - inputExtent.xy)/inputExtent.zw;
+ float intensity = mix(1.0, 2.0*distance(p, vec2(0.5, 0.5)), flashZoomEffect) * flashIntensity * pow(smoothstep(flashPhase, 0.0, distance(0.5, progress)), flashVelocity);
+ vec4 c = mix(inputImage, inputTargetImage, smoothstep(0.5*(1.0-flashPhase), 0.5*(1.0+flashPhase), progress));
+ c += intensity * vec4(flashColor, 1.0);
+ return c;
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIFlashTransition.h b/Pods/Vivid/Sources/YUCIFlashTransition.h
new file mode 100644
index 0000000..39db7c3
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFlashTransition.h
@@ -0,0 +1,24 @@
+//
+// YUCIFlashTransition.h
+// Pods
+//
+// Created by YuAo on 2/4/16.
+//
+//
+
+#import
+
+@interface YUCIFlashTransition : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+@property (nonatomic, strong, nullable) CIImage *inputTargetImage;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputFlashPhase;
+@property (nonatomic, copy, null_resettable) NSNumber *inputFlashIntensity;
+@property (nonatomic, copy, null_resettable) NSNumber *inputFlashZoom;
+
+@property (nonatomic, copy, nullable) CIVector *inputExtent;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputTime; /* 0 to 1 */
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIFlashTransition.m b/Pods/Vivid/Sources/YUCIFlashTransition.m
new file mode 100644
index 0000000..f6205c3
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIFlashTransition.m
@@ -0,0 +1,84 @@
+//
+// YUCIFlashTransition.m
+// Pods
+//
+// Created by YuAo on 2/4/16.
+//
+//
+
+#import "YUCIFlashTransition.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIFlashTransition
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIFlashTransition class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryTransition],
+ kCIAttributeFilterDisplayName: @"Flash Transition"}];
+ }
+ }
+ });
+}
+
++ (CIColorKernel *)filterKernel {
+ static CIColorKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIFlashTransition class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIColorKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputFlashPhase {
+ if (!_inputFlashPhase) {
+ _inputFlashPhase = @(0.6);
+ }
+ return _inputFlashPhase;
+}
+
+- (NSNumber *)inputFlashIntensity {
+ if (!_inputFlashIntensity) {
+ _inputFlashIntensity = @(3.0);
+ }
+ return _inputFlashIntensity;
+}
+
+- (NSNumber *)inputFlashZoom {
+ if (!_inputFlashZoom) {
+ _inputFlashZoom = @(0.5);
+ }
+ return _inputFlashZoom;
+}
+
+- (NSNumber *)inputTime {
+ if (!_inputTime) {
+ _inputTime = @(0);
+ }
+ return _inputTime;
+}
+
+- (void)setDefaults {
+ self.inputFlashPhase = nil;
+ self.inputFlashIntensity = nil;
+ self.inputFlashZoom = nil;
+ self.inputTime = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage || !self.inputTargetImage) {
+ return nil;
+ }
+
+ CIVector *defaultInputExtent = [CIVector vectorWithCGRect:CGRectUnion(self.inputImage.extent, self.inputTargetImage.extent)];
+ CIVector *extent = self.inputExtent?:defaultInputExtent;
+ return [[YUCIFlashTransition filterKernel] applyWithExtent:extent.CGRectValue
+ arguments:@[self.inputImage,self.inputTargetImage,self.inputFlashPhase,self.inputFlashIntensity,self.inputFlashZoom,extent,self.inputTime]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIHSLToRGB.cikernel b/Pods/Vivid/Sources/YUCIHSLToRGB.cikernel
new file mode 100644
index 0000000..43dbf16
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIHSLToRGB.cikernel
@@ -0,0 +1,29 @@
+float hue2rgb(float p, float q, float t){
+ if(t < 0.0) t += 1.0;
+ if(t > 1.0) t -= 1.0;
+ if(t < 1.0/6.0) return p + (q - p) * 6.0 * t;
+ if(t < 1.0/2.0) return q;
+ if(t < 2.0/3.0) return p + (q - p) * (2.0/3.0 - t) * 6.0;
+ return p;
+}
+
+kernel vec4 hsl2rgb(__sample inputColor)
+{
+ vec4 color = clamp(inputColor,vec4(0.0),vec4(1.0));
+
+ float h = color.r;
+ float s = color.g;
+ float l = color.b;
+
+ float r,g,b;
+ if(s <= 0.0){
+ r = g = b = l;
+ }else{
+ float q = l < 0.5 ? (l * (1.0 + s)) : (l + s - l * s);
+ float p = 2.0 * l - q;
+ r = hue2rgb(p, q, h + 1.0/3.0);
+ g = hue2rgb(p, q, h);
+ b = hue2rgb(p, q, h - 1.0/3.0);
+ }
+ return vec4(r,g,b,color.a);
+}
diff --git a/Pods/Vivid/Sources/YUCIHistogramEqualization.h b/Pods/Vivid/Sources/YUCIHistogramEqualization.h
new file mode 100644
index 0000000..fd935ef
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIHistogramEqualization.h
@@ -0,0 +1,15 @@
+//
+// YUCIHistogramEqualization.h
+// Pods
+//
+// Created by YuAo on 2/15/16.
+//
+//
+
+#import
+
+@interface YUCIHistogramEqualization : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIHistogramEqualization.m b/Pods/Vivid/Sources/YUCIHistogramEqualization.m
new file mode 100644
index 0000000..897feeb
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIHistogramEqualization.m
@@ -0,0 +1,69 @@
+//
+// YUCIHistogramEqualization.m
+// Pods
+//
+// Created by YuAo on 2/15/16.
+//
+//
+
+#import "YUCIHistogramEqualization.h"
+#import "YUCIFilterConstructor.h"
+#import
+
+@interface YUCIHistogramEqualization ()
+
+@property (nonatomic, strong) CIContext *context;
+
+@end
+
+@implementation YUCIHistogramEqualization
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIHistogramEqualization class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryColorAdjustment],
+ kCIAttributeFilterDisplayName: @"Histogram Equalization"}];
+ }
+ }
+ });
+}
+
+- (CIContext *)context {
+ if (!_context) {
+ _context = [CIContext contextWithOptions:@{kCIContextWorkingColorSpace: CFBridgingRelease(CGColorSpaceCreateWithName(kCGColorSpaceSRGB))}];
+ }
+ return _context;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ ptrdiff_t rowBytes = self.inputImage.extent.size.width * 4; // ARGB has 4 components
+ uint8_t *byteBuffer = calloc(rowBytes * self.inputImage.extent.size.height, sizeof(uint8_t)); // Buffer to render into
+ [self.context render:self.inputImage
+ toBitmap:byteBuffer
+ rowBytes:rowBytes
+ bounds:self.inputImage.extent
+ format:kCIFormatARGB8
+ colorSpace:self.context.workingColorSpace];
+
+ vImage_Buffer vImageBuffer;
+ vImageBuffer.data = byteBuffer;
+ vImageBuffer.width = self.inputImage.extent.size.width;
+ vImageBuffer.height = self.inputImage.extent.size.height;
+ vImageBuffer.rowBytes = rowBytes;
+
+ vImageEqualization_ARGB8888(&vImageBuffer, &vImageBuffer, kvImageNoFlags);
+
+ NSData *bitmapData = [NSData dataWithBytesNoCopy:vImageBuffer.data length:vImageBuffer.rowBytes * vImageBuffer.height freeWhenDone:YES];
+ CIImage *result = [[CIImage alloc] initWithBitmapData:bitmapData bytesPerRow:vImageBuffer.rowBytes size:CGSizeMake(vImageBuffer.width, vImageBuffer.height) format:kCIFormatARGB8 colorSpace:self.context.workingColorSpace];
+ return result;
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIRGBToHSL.cikernel b/Pods/Vivid/Sources/YUCIRGBToHSL.cikernel
new file mode 100644
index 0000000..361cbef
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIRGBToHSL.cikernel
@@ -0,0 +1,24 @@
+kernel vec4 rgb2hsl(__sample inputColor)
+{
+ vec4 color = clamp(inputColor,vec4(0.0),vec4(1.0));
+
+ //Compute min and max component values
+ float MAX = max(color.r, max(color.g, color.b));
+ float MIN = min(color.r, min(color.g, color.b));
+
+ //Make sure MAX > MIN to avoid division by zero later
+ MAX = max(MIN + 1e-6, MAX);
+
+ //Compute luminosity
+ float l = (MIN + MAX) / 2.0;
+
+ //Compute saturation
+ float s = (l < 0.5 ? (MAX - MIN) / (MIN + MAX) : (MAX - MIN) / (2.0 - MAX - MIN));
+
+ //Compute hue
+ float h = (MAX == color.r ? (color.g - color.b) / (MAX - MIN) : (MAX == color.g ? 2.0 + (color.b - color.r) / (MAX - MIN) : 4.0 + (color.r - color.g) / (MAX - MIN)));
+ h /= 6.0;
+ h = (h < 0.0 ? 1.0 + h : h);
+
+ return vec4(h, s, l, color.a);
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIRGBToneCurve.cikernel b/Pods/Vivid/Sources/YUCIRGBToneCurve.cikernel
new file mode 100644
index 0000000..864043f
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIRGBToneCurve.cikernel
@@ -0,0 +1,14 @@
+
+kernel vec4 filterKernel(sampler inputImage, sampler toneCurveTexture, float intensity) {
+ vec4 textureColor = sample(inputImage,samplerCoord(inputImage));
+ vec4 toneCurveTextureExtent = samplerExtent(toneCurveTexture);
+
+ vec2 redCoord = samplerTransform(toneCurveTexture,vec2(textureColor.r * 255.0 + 0.5 + toneCurveTextureExtent.x, toneCurveTextureExtent.y + 0.5));
+ vec2 greenCoord = samplerTransform(toneCurveTexture,vec2(textureColor.g * 255.0 + 0.5 + toneCurveTextureExtent.x, toneCurveTextureExtent.y + 0.5));
+ vec2 blueCoord = samplerTransform(toneCurveTexture,vec2(textureColor.b * 255.0 + 0.5 + toneCurveTextureExtent.x, toneCurveTextureExtent.y + 0.5));
+
+ float redCurveValue = sample(toneCurveTexture, redCoord).r;
+ float greenCurveValue = sample(toneCurveTexture, greenCoord).g;
+ float blueCurveValue = sample(toneCurveTexture, blueCoord).b;
+ return vec4(mix(textureColor.rgb,vec3(redCurveValue, greenCurveValue, blueCurveValue),intensity),textureColor.a);
+}
diff --git a/Pods/Vivid/Sources/YUCIRGBToneCurve.h b/Pods/Vivid/Sources/YUCIRGBToneCurve.h
new file mode 100644
index 0000000..aea1d91
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIRGBToneCurve.h
@@ -0,0 +1,22 @@
+//
+// YUCIRGBToneCurveFilter.h
+// Pods
+//
+// Created by YuAo on 1/21/16.
+//
+//
+
+#import
+
+@interface YUCIRGBToneCurve : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, copy, null_resettable) NSArray *inputRedControlPoints;
+@property (nonatomic, copy, null_resettable) NSArray *inputGreenControlPoints;
+@property (nonatomic, copy, null_resettable) NSArray *inputBlueControlPoints;
+@property (nonatomic, copy, null_resettable) NSArray *inputRGBCompositeControlPoints;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputIntensity; //default 1.0
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIRGBToneCurve.m b/Pods/Vivid/Sources/YUCIRGBToneCurve.m
new file mode 100644
index 0000000..331a2ad
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIRGBToneCurve.m
@@ -0,0 +1,371 @@
+//
+// YUCIRGBToneCurveFilter.m
+// Pods
+//
+// Created by YuAo on 1/21/16.
+//
+//
+
+#import "YUCIRGBToneCurve.h"
+#import "YUCIFilterConstructor.h"
+
+static NSCache * YUCIRGBToneCurveSplineCurveCache(void) {
+ static NSCache *cache;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ cache = [[NSCache alloc] init];
+ cache.name = @"YUCIRGBToneCurveSplineCurveCache";
+ cache.totalCostLimit = 40;
+ });
+ return cache;
+}
+
+@interface YUCIRGBToneCurve ()
+
+@property (nonatomic,copy) NSArray *redCurve, *greenCurve, *blueCurve, *rgbCompositeCurve;
+
+@property (nonatomic,strong) CIImage *toneCurveTexture;
+
+@end
+
+@implementation YUCIRGBToneCurve
+
+@synthesize inputRGBCompositeControlPoints = _inputRGBCompositeControlPoints;
+@synthesize inputRedControlPoints = _inputRedControlPoints;
+@synthesize inputGreenControlPoints = _inputGreenControlPoints;
+@synthesize inputBlueControlPoints = _inputBlueControlPoints;
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIRGBToneCurve class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo, kCICategoryColorAdjustment,kCICategoryInterlaced],
+ kCIAttributeFilterDisplayName: @"RGB Tone Curve"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIRGBToneCurve class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputIntensity {
+ if (!_inputIntensity) {
+ _inputIntensity = @(1.0);
+ }
+ return _inputIntensity;
+}
+
+- (NSArray *)defaultCurveControlPoints {
+ return @[[CIVector vectorWithX:0 Y:0],
+ [CIVector vectorWithX:0.5 Y:0.5],
+ [CIVector vectorWithX:1 Y:1]];
+}
+
+- (void)setDefaults {
+ self.inputIntensity = nil;
+ self.inputRedControlPoints = nil;
+ self.inputGreenControlPoints = nil;
+ self.inputBlueControlPoints = nil;
+ self.inputRGBCompositeControlPoints = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ if (!self.toneCurveTexture) {
+ [self updateToneCurveTexture];
+ }
+
+ return [[YUCIRGBToneCurve filterKernel] applyWithExtent:self.inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ if (index == 0) {
+ return destRect;
+ } else {
+ return self.toneCurveTexture.extent;
+ }
+ }
+ arguments:@[self.inputImage,
+ self.toneCurveTexture,
+ self.inputIntensity]];
+}
+
+- (void)updateToneCurveTexture {
+ if (self.rgbCompositeCurve.count != 256) {
+ self.rgbCompositeCurve = [self getPreparedSplineCurve:self.inputRGBCompositeControlPoints];
+ }
+
+ if (self.redCurve.count != 256) {
+ self.redCurve = [self getPreparedSplineCurve:self.inputRedControlPoints];
+ }
+
+ if (self.greenCurve.count != 256) {
+ self.greenCurve = [self getPreparedSplineCurve:self.inputGreenControlPoints];
+ }
+
+ if (self.blueCurve.count != 256) {
+ self.blueCurve = [self getPreparedSplineCurve:self.inputBlueControlPoints];
+ }
+
+ uint8_t *toneCurveByteArray = calloc(256 * 4, sizeof(uint8_t));
+ for (NSUInteger currentCurveIndex = 0; currentCurveIndex < 256; currentCurveIndex++)
+ {
+ // BGRA for upload to texture
+ uint8_t b = fmin(fmax(currentCurveIndex + self.blueCurve[currentCurveIndex].floatValue, 0), 255);
+ toneCurveByteArray[currentCurveIndex * 4] = fmin(fmax(b + self.rgbCompositeCurve[b].floatValue, 0), 255);
+ uint8_t g = fmin(fmax(currentCurveIndex + self.greenCurve[currentCurveIndex].floatValue, 0), 255);
+ toneCurveByteArray[currentCurveIndex * 4 + 1] = fmin(fmax(g + self.rgbCompositeCurve[g].floatValue, 0), 255);
+ uint8_t r = fmin(fmax(currentCurveIndex + self.redCurve[currentCurveIndex].floatValue, 0), 255);
+ toneCurveByteArray[currentCurveIndex * 4 + 2] = fmin(fmax(r + self.rgbCompositeCurve[r].floatValue, 0), 255);
+ toneCurveByteArray[currentCurveIndex * 4 + 3] = 255;
+ }
+ CIImage *toneCurveTexture = [CIImage imageWithBitmapData:[NSData dataWithBytesNoCopy:toneCurveByteArray length:256 * 4 * sizeof(uint8_t) freeWhenDone:YES] bytesPerRow:256 * 4 * sizeof(uint8_t) size:CGSizeMake(256, 1) format:kCIFormatBGRA8 colorSpace:nil];
+ self.toneCurveTexture = toneCurveTexture;
+}
+
+- (NSArray *)inputRGBCompositeControlPoints {
+ if (_inputRGBCompositeControlPoints.count == 0) {
+ _inputRGBCompositeControlPoints = self.defaultCurveControlPoints;
+ }
+ return _inputRGBCompositeControlPoints;
+}
+
+- (NSArray *)inputRedControlPoints {
+ if (_inputRedControlPoints.count == 0) {
+ _inputRedControlPoints = self.defaultCurveControlPoints;
+ }
+ return _inputRedControlPoints;
+}
+
+- (NSArray *)inputGreenControlPoints {
+ if (_inputGreenControlPoints.count == 0) {
+ _inputGreenControlPoints = self.defaultCurveControlPoints;
+ }
+ return _inputGreenControlPoints;
+}
+
+- (NSArray *)inputBlueControlPoints {
+ if (_inputBlueControlPoints.count == 0) {
+ _inputBlueControlPoints = self.defaultCurveControlPoints;
+ }
+ return _inputBlueControlPoints;
+}
+
+- (void)setInputRGBCompositeControlPoints:(NSArray *)inputRGBCompositeControlPoints {
+ _inputRGBCompositeControlPoints = inputRGBCompositeControlPoints.copy;
+ _rgbCompositeCurve = nil;
+ _toneCurveTexture = nil;
+}
+
+- (void)setInputRedControlPoints:(NSArray *)inputRedControlPoints {
+ _inputRedControlPoints = inputRedControlPoints.copy;
+ _redCurve = nil;
+ _toneCurveTexture = nil;
+}
+
+- (void)setInputGreenControlPoints:(NSArray *)inputGreenControlPoints {
+ _inputGreenControlPoints = inputGreenControlPoints.copy;
+ _greenCurve = nil;
+ _toneCurveTexture = nil;
+}
+
+- (void)setInputBlueControlPoints:(NSArray *)inputBlueControlPoints {
+ _inputBlueControlPoints = inputBlueControlPoints.copy;
+ _blueCurve = nil;
+ _toneCurveTexture = nil;
+}
+
+#pragma mark - Curve calculation
+
+- (NSArray *)getPreparedSplineCurve:(NSArray *)points
+{
+ NSArray *cachedCurve = [YUCIRGBToneCurveSplineCurveCache() objectForKey:points];
+ if (cachedCurve) {
+ return cachedCurve;
+ }
+
+ if (points && [points count] > 0)
+ {
+ // Sort the array.
+ NSArray *sortedPoints = [points sortedArrayUsingComparator:^NSComparisonResult(CIVector *a, CIVector *b) {
+ return a.X > b.X;
+ }];
+
+ // Convert from (0, 1) to (0, 255).
+ NSMutableArray *convertedPoints = [NSMutableArray arrayWithCapacity:[sortedPoints count]];
+ for (NSInteger i = 0; i < points.count; i++){
+ CIVector *point = [sortedPoints objectAtIndex:i];
+ [convertedPoints addObject:[CIVector vectorWithX:point.X * 255 Y:point.Y * 255]];
+ }
+
+
+ NSMutableArray *splinePoints = [self splineCurve:convertedPoints];
+
+ // If we have a first point like (0.3, 0) we'll be missing some points at the beginning
+ // that should be 0.
+ CIVector *firstSplinePoint = splinePoints.firstObject;
+
+ if (firstSplinePoint.X > 0) {
+ for (NSInteger i = firstSplinePoint.X; i >= 0; i--) {
+ [splinePoints insertObject:[CIVector vectorWithX:i Y:0] atIndex:0];
+ }
+ }
+
+ // Insert points similarly at the end, if necessary.
+ CIVector *lastSplinePoint = splinePoints.lastObject;
+ if (lastSplinePoint.X < 255) {
+ for (NSInteger i = lastSplinePoint.X + 1; i <= 255; i++) {
+ [splinePoints addObject:[CIVector vectorWithX:i Y:255]];
+ }
+ }
+
+ // Prepare the spline points.
+ NSMutableArray *preparedSplinePoints = [NSMutableArray arrayWithCapacity:[splinePoints count]];
+ for (NSInteger i=0; i<[splinePoints count]; i++)
+ {
+ CIVector *newPoint = splinePoints[i];
+ CIVector *origPoint = [CIVector vectorWithX:newPoint.X Y:newPoint.X];
+ float distance = sqrt(pow((origPoint.X - newPoint.X), 2.0) + pow((origPoint.Y - newPoint.Y), 2.0));
+ if (origPoint.Y > newPoint.Y)
+ {
+ distance = -distance;
+ }
+ [preparedSplinePoints addObject:@(distance)];
+ }
+
+ [YUCIRGBToneCurveSplineCurveCache() setObject:preparedSplinePoints forKey:points cost:1];
+
+ return preparedSplinePoints;
+ }
+
+ return nil;
+}
+
+
+- (NSMutableArray *)splineCurve:(NSArray *)points
+{
+ NSMutableArray *sdA = [self secondDerivative:points];
+
+ // [points count] is equal to [sdA count]
+ NSInteger n = [sdA count];
+ if (n < 1)
+ {
+ return nil;
+ }
+ double sd[n];
+
+ // From NSMutableArray to sd[n];
+ for (NSInteger i=0; i 255.0) {
+ y = 255.0;
+ } else if (y < 0.0) {
+ y = 0.0;
+ }
+ [output addObject:[CIVector vectorWithX:x Y:y]];
+ }
+ }
+
+ // The above always misses the last point because the last point is the last next, so we approach but don't equal it.
+ [output addObject:points.lastObject];
+ return output;
+}
+
+- (NSMutableArray *)secondDerivative:(NSArray *)points
+{
+ const NSInteger n = [points count];
+ if ((n <= 0) || (n == 1))
+ {
+ return nil;
+ }
+
+ double matrix[n][3];
+ double result[n];
+ matrix[0][1]=1;
+ // What about matrix[0][1] and matrix[0][0]? Assuming 0 for now (Brad L.)
+ matrix[0][0]=0;
+ matrix[0][2]=0;
+
+ for(NSInteger i=1; idown)
+ for(NSInteger i=1;iup)
+ for(NSInteger i=n-2;i>=0;i--) {
+ double k = matrix[i][2]/matrix[i+1][1];
+ matrix[i][1] -= k*matrix[i+1][0];
+ matrix[i][2] = 0;
+ result[i] -= k*result[i+1];
+ }
+
+ double y2[n];
+ for(NSInteger i=0; i= inputExtent.x &&
+ coord.x < inputExtent.x + inputExtent.z &&
+ coord.y >= inputExtent.y &&
+ coord.y < inputExtent.y + inputExtent.w
+ ) {
+ return coord;
+ } else {
+ int mode = int(floor(inputMode + 0.5));
+ float w = inputExtent.z - (mode == 0 ? 1.0: 0.0);
+ float h = inputExtent.w - (mode == 0 ? 1.0: 0.0);
+
+ float x = coord.x - inputExtent.x - inputExtent.z;
+ float nx = floor(x/w);
+ float dx = x - nx * w;
+
+ float y = coord.y - inputExtent.y - inputExtent.w;
+ float ny = floor(y/h);
+ float dy = y - ny * h;
+
+ if (int(mod(nx,2.0)) == 1) {
+ coord.x = inputExtent.x + inputExtent.z - (w - dx);
+ } else {
+ coord.x = inputExtent.x + (w - dx);
+ }
+
+ if (int(mod(ny,2.0)) == 1) {
+ coord.y = inputExtent.y + inputExtent.w - (h - dy);
+ } else {
+ coord.y = inputExtent.y + (h - dy);
+ }
+ return coord;
+ }
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIReflectedTile.h b/Pods/Vivid/Sources/YUCIReflectedTile.h
new file mode 100644
index 0000000..ef62a67
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIReflectedTile.h
@@ -0,0 +1,22 @@
+//
+// YUCIReflectTile.h
+// Pods
+//
+// Created by YuAo on 2/16/16.
+//
+//
+
+#import
+
+typedef NS_ENUM(NSInteger, YUCIReflectedTileMode) {
+ YUCIReflectedTileModeReflectWithoutBorder = 0,
+ YUCIReflectedTileModeReflectWithBorder = 1,
+};
+
+@interface YUCIReflectedTile : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputMode; //default: YUCIReflectedTileModeReflectWithoutBorder
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIReflectedTile.m b/Pods/Vivid/Sources/YUCIReflectedTile.m
new file mode 100644
index 0000000..7758ace
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIReflectedTile.m
@@ -0,0 +1,64 @@
+//
+// YUCIReflectTile.m
+// Pods
+//
+// Created by YuAo on 2/16/16.
+//
+//
+
+#import "YUCIReflectedTile.h"
+#import "YUCIFilterConstructor.h"
+#import "YUCIReflectedTileROICalculator.h"
+
+@implementation YUCIReflectedTile
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIReflectedTile class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryTileEffect],
+ kCIAttributeFilterDisplayName: @"Reflected Tile"}];
+ }
+ }
+ });
+}
+
++ (CIWarpKernel *)filterKernel {
+ static CIWarpKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIReflectedTile class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIWarpKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputMode {
+ if (!_inputMode) {
+ _inputMode = @(YUCIReflectedTileModeReflectWithoutBorder);
+ }
+ return _inputMode;
+}
+
+- (void)setDefaults {
+ self.inputMode = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ CGRect inputExtent = self.inputImage.extent;
+ return [[YUCIReflectedTile filterKernel] applyWithExtent:CGRectInfinite
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return [YUCIReflectedTileROICalculator ROIForDestinationRect:destRect inputImageExtent:inputExtent mode:self.inputMode.integerValue];
+ }
+ inputImage:self.inputImage
+ arguments:@[self.inputMode,[CIVector vectorWithCGRect:self.inputImage.extent]]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIReflectedTileROICalculator.h b/Pods/Vivid/Sources/YUCIReflectedTileROICalculator.h
new file mode 100644
index 0000000..bb1e03c
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIReflectedTileROICalculator.h
@@ -0,0 +1,16 @@
+//
+// YUCIReflectedTileROICalculator.h
+// Pods
+//
+// Created by YuAo on 2/19/16.
+//
+//
+
+#import
+#import "YUCIReflectedTile.h"
+
+@interface YUCIReflectedTileROICalculator : NSObject
+
++ (CGRect)ROIForDestinationRect:(CGRect)destRect inputImageExtent:(CGRect)inputExtent mode:(YUCIReflectedTileMode)mode;
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIReflectedTileROICalculator.m b/Pods/Vivid/Sources/YUCIReflectedTileROICalculator.m
new file mode 100644
index 0000000..e255b2d
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIReflectedTileROICalculator.m
@@ -0,0 +1,167 @@
+//
+// YUCIReflectedTileROICalculator.m
+// Pods
+//
+// Created by YuAo on 2/19/16.
+//
+//
+
+#import "YUCIReflectedTileROICalculator.h"
+
+static void YUCIReflectedTileROICalculatorSplitRectWithBorderOfRect(CGRect rect,
+ CGRect split,
+ CGRect *insider,
+ CGRect *verticalRemining,
+ CGRect *horizonalRemining,
+ CGRect *diagonalRemining)
+{
+ CGRect insideRect = CGRectIntersection(split, rect);
+
+ CGRect unused;
+ CGRect verticalRect;
+ CGRectDivide(rect, &unused, &verticalRect, insideRect.size.height, CGRectMinYEdge);
+ CGRect horizonalRect;
+ CGRectDivide(rect, &unused, &horizonalRect, insideRect.size.width, CGRectMinXEdge);
+ CGRect diagonalRect = CGRectIntersection(verticalRect, horizonalRect);
+ verticalRect.size.width -= diagonalRect.size.width;
+ horizonalRect.size.height -= diagonalRect.size.height;
+
+ *insider = insideRect;
+ *verticalRemining = verticalRect;
+ *horizonalRemining = horizonalRect;
+ *diagonalRemining = diagonalRect;
+}
+
+@implementation YUCIReflectedTileROICalculator
+
++ (NSArray *)map:(NSArray *)array usingBlock:(id (^)(id))mapper {
+ NSMutableArray *result = [NSMutableArray new];
+ for (id element in array) {
+ [result addObject:mapper(element)];
+ }
+ return result.copy;
+}
+
++ (id)reduce:(NSArray *)array seed:(id)seed combiner:(id (^)(id, id))combiner {
+ id current = seed;
+ for (id element in array) {
+ current = combiner(current, element);
+ }
+ return current;
+}
+
++ (NSArray *)filter:(NSArray *)array usingFilter:(BOOL (^)(id))filter {
+ NSMutableArray *filteredArray = [NSMutableArray array];
+ for (id element in array) {
+ if (filter(element)) {
+ [filteredArray addObject:element];
+ }
+ }
+ return filteredArray.copy;
+}
+
++ (CGPoint)locationForPoint:(CGPoint)point inOrigin4GridRect:(CGRect)origin4GridRect {
+ CGPoint result = CGPointZero;
+ result.x = ((NSInteger)(point.x - origin4GridRect.origin.x) % (NSInteger)origin4GridRect.size.width);
+ result.y = ((NSInteger)(point.y - origin4GridRect.origin.y) % (NSInteger)origin4GridRect.size.height);
+ return result;
+}
+
++ (CGRect)rectByHorizonallyFoldingRect:(CGRect)rect insideRect:(CGRect)boundingRect {
+ CGFloat distance = rect.origin.x - (boundingRect.origin.x + boundingRect.size.width);
+ if (distance >= 0) {
+ CGRect result = rect;
+ result.origin.x = boundingRect.size.width - distance - result.size.width + boundingRect.origin.x;
+ return result;
+ } else {
+ CGRect inside, vertical, horizonal, diagonal;
+ YUCIReflectedTileROICalculatorSplitRectWithBorderOfRect(rect, boundingRect, &inside, &vertical, &horizonal, &diagonal);
+ if (CGRectIsEmpty(horizonal)) {
+ return inside;
+ }
+ horizonal.origin.x = horizonal.origin.x - horizonal.size.width;
+ return CGRectUnion(inside, horizonal);
+ }
+}
+
++ (CGRect)rectByVerticallyFoldingRect:(CGRect)rect insideRect:(CGRect)boundingRect {
+ CGFloat distance = rect.origin.y - (boundingRect.origin.y + boundingRect.size.height);
+ if (distance >= 0) {
+ CGRect result = rect;
+ result.origin.y = boundingRect.size.height - distance - result.size.height + boundingRect.origin.y;
+ return result;
+ } else {
+ CGRect inside, vertical, horizonal, diagonal;
+ YUCIReflectedTileROICalculatorSplitRectWithBorderOfRect(rect, boundingRect, &inside, &vertical, &horizonal, &diagonal);
+ if (CGRectIsEmpty(vertical)) {
+ return inside;
+ }
+ vertical.origin.y = vertical.origin.y - horizonal.size.height;
+ return CGRectUnion(inside, vertical);
+ }
+}
+
++ (CGRect)ROIForDestinationRect:(CGRect)destRect inputImageExtent:(CGRect)inputExtent mode:(YUCIReflectedTileMode)mode {
+ if (CGRectContainsRect(inputExtent, destRect)) {
+ return destRect;
+ } else if(CGRectContainsRect(destRect, inputExtent)) {
+ return inputExtent;
+ }
+
+ CGFloat modeDiff = (mode == YUCIReflectedTileModeReflectWithoutBorder ? 1 : 0);
+ CGRect grid = CGRectMake(inputExtent.origin.x, inputExtent.origin.y, inputExtent.size.width * 2 - modeDiff, inputExtent.size.height * 2 - modeDiff);
+
+ destRect.origin = [self locationForPoint:destRect.origin inOrigin4GridRect:grid];
+
+ CGRect inside, vertical, horizonal, diagonal;
+ YUCIReflectedTileROICalculatorSplitRectWithBorderOfRect(destRect, grid, &inside, &vertical, &horizonal, &diagonal);
+ if (CGRectIsEmpty(inside)) {
+ return CGRectZero;
+ }
+
+ //align rects
+ vertical.origin.y -= grid.size.height;
+ horizonal.origin.x -= grid.size.width;
+ diagonal.origin.y -= grid.size.height;
+ diagonal.origin.x -= grid.size.width;
+
+ vertical = CGRectIntersection(grid, vertical);
+ horizonal = CGRectIntersection(grid, horizonal);
+ diagonal = CGRectIntersection(grid, diagonal);
+
+ NSArray *rects = ({
+ NSMutableArray *rectsM = [NSMutableArray array];
+ [rectsM addObject:[CIVector vectorWithCGRect:inside]];
+ [rectsM addObject:[CIVector vectorWithCGRect:vertical]];
+ [rectsM addObject:[CIVector vectorWithCGRect:horizonal]];
+ [rectsM addObject:[CIVector vectorWithCGRect:diagonal]];
+ rectsM.copy;
+ });
+
+ rects = [self filter:rects usingFilter:^BOOL(CIVector *rectValue) {
+ if (CGRectIsEmpty(rectValue.CGRectValue)) {
+ return NO;
+ }
+ return YES;
+ }];
+
+ rects = [self map:rects usingBlock:^id(CIVector *rectValue) {
+ CGRect rect = [rectValue CGRectValue];
+ rect = [self rectByHorizonallyFoldingRect:rect insideRect:CGRectMake(inputExtent.origin.x, inputExtent.origin.y, inputExtent.size.width, grid.size.height)];
+ rect = [self rectByVerticallyFoldingRect:rect insideRect:inputExtent];
+ rect = CGRectInset(rect, -1, -1);
+ return [CIVector vectorWithCGRect:rect];
+ }];
+
+ CIVector *result = [self reduce:rects seed:nil combiner:^id(CIVector *pre, CIVector *current) {
+ if (pre) {
+ return [CIVector vectorWithCGRect:CGRectUnion(pre.CGRectValue, current.CGRectValue)];
+ }
+ return current;
+ }];
+
+ CGRect ROI = CGRectIntersection(inputExtent, result.CGRectValue);
+ return ROI;
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCISkyGenerator.cikernel b/Pods/Vivid/Sources/YUCISkyGenerator.cikernel
new file mode 100644
index 0000000..a75ec98
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCISkyGenerator.cikernel
@@ -0,0 +1,124 @@
+//https://github.com/wwwtyro/glsl-atmosphere
+
+#define PI 3.141592
+#define iSteps 16
+#define jSteps 8
+
+float rsi(vec3 r0, vec3 rd, float sr) {
+ // Simplified ray-sphere intersection that assumes
+ // the ray starts inside the sphere and that the
+ // sphere is centered at the origin. Always intersects.
+ float a = dot(rd, rd);
+ float b = 2.0 * dot(rd, r0);
+ float c = dot(r0, r0) - (sr * sr);
+ return (-b + sqrt((b*b) - 4.0*a*c))/(2.0*a);
+}
+
+vec3 atmosphere(vec3 r, vec3 r0, vec3 pSun, float iSun, float rPlanet, float rAtmos, vec3 kRlh, float kMie, float shRlh, float shMie, float g) {
+ // Normalize the sun and view directions.
+ pSun = normalize(pSun);
+ r = normalize(r);
+
+ // Calculate the step size of the primary ray.
+ float iStepSize = rsi(r0, r, rAtmos) / float(iSteps);
+
+ // Initialize the primary ray time.
+ float iTime = 0.0;
+
+ // Initialize accumulators for Rayleigh and Mie scattering.
+ vec3 totalRlh = vec3(0.0);
+ vec3 totalMie = vec3(0.0);
+
+ // Initialize optical depth accumulators for the primary ray.
+ float iOdRlh = 0.0;
+ float iOdMie = 0.0;
+
+ // Calculate the Rayleigh and Mie phases.
+ float mu = dot(r, pSun);
+ float mumu = mu * mu;
+ float gg = g * g;
+ float pRlh = 3.0 / (16.0 * PI) * (1.0 + mumu);
+ float pMie = 3.0 / (8.0 * PI) * ((1.0 - gg) * (mumu + 1.0)) / (pow(1.0 + gg - 2.0 * mu * g, 1.5) * (2.0 + gg));
+
+ // Sample the primary ray.
+ for (int i = 0; i < iSteps; i++) {
+
+ // Calculate the primary ray sample position.
+ vec3 iPos = r0 + r * (iTime + iStepSize * 0.5);
+
+ // Calculate the height of the sample.
+ float iHeight = length(iPos) - rPlanet;
+
+ // Calculate the optical depth of the Rayleigh and Mie scattering for this step.
+ float odStepRlh = exp(-iHeight / shRlh) * iStepSize;
+ float odStepMie = exp(-iHeight / shMie) * iStepSize;
+
+ // Accumulate optical depth.
+ iOdRlh += odStepRlh;
+ iOdMie += odStepMie;
+
+ // Calculate the step size of the secondary ray.
+ float jStepSize = rsi(iPos, pSun, rAtmos) / float(jSteps);
+
+ // Initialize the secondary ray time.
+ float jTime = 0.0;
+
+ // Initialize optical depth accumulators for the secondary ray.
+ float jOdRlh = 0.0;
+ float jOdMie = 0.0;
+
+ // Sample the secondary ray.
+ for (int j = 0; j < jSteps; j++) {
+
+ // Calculate the secondary ray sample position.
+ vec3 jPos = iPos + pSun * (jTime + jStepSize * 0.5);
+
+ // Calculate the height of the sample.
+ float jHeight = length(jPos) - rPlanet;
+
+ // Accumulate the optical depth.
+ jOdRlh += exp(-jHeight / shRlh) * jStepSize;
+ jOdMie += exp(-jHeight / shMie) * jStepSize;
+
+ // Increment the secondary ray time.
+ jTime += jStepSize;
+ }
+
+ // Calculate attenuation.
+ vec3 attn = exp(-(kMie * (iOdMie + jOdMie) + kRlh * (iOdRlh + jOdRlh)));
+
+ // Accumulate scattering.
+ totalRlh += odStepRlh * attn;
+ totalMie += odStepMie * attn;
+
+ // Increment the primary ray time.
+ iTime += iStepSize;
+
+ }
+
+ // Calculate and return the final color.
+ return iSun * (pRlh * kRlh * totalRlh + pMie * kMie * totalMie);
+}
+
+kernel vec4 coreImageKernel(vec4 inputExtent, vec2 viewPoint, vec3 sunPosition, float sunIntensity) {
+ vec2 fragCoord = destCoord();
+ vec2 iResolution = inputExtent.zw;
+ vec3 color = atmosphere(
+ normalize(vec3((vec2(0.5) - fragCoord/iResolution) * vec2(-2.0) + viewPoint,-1.0)), // normalized ray direction
+ vec3(0,6372e3,0), // ray origin
+ sunPosition, // position of the sun
+ sunIntensity, // intensity of the sun
+ 6371e3, // radius of the planet in meters
+ 6471e3, // radius of the atmosphere in meters
+ vec3(5.5e-6, 13.0e-6, 22.4e-6), // Rayleigh scattering coefficient
+ 21e-6, // Mie scattering coefficient
+ 8e3, // Rayleigh scale height
+ 1.2e3, // Mie scale height
+ 0.758 // Mie preferred scattering direction
+ );
+
+ // Apply exposure.
+ color = 1.0 - exp(-1.0 * color);
+
+ return vec4(color, 1.0);
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCISkyGenerator.h b/Pods/Vivid/Sources/YUCISkyGenerator.h
new file mode 100644
index 0000000..57af4fa
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCISkyGenerator.h
@@ -0,0 +1,21 @@
+//
+// YUCISkyGenerator.h
+// Pods
+//
+// Created by YuAo on 3/15/16.
+//
+//
+
+#import
+
+@interface YUCISkyGenerator : CIFilter
+
+@property (nonatomic, copy, null_resettable) CIVector *inputExtent;
+
+@property (nonatomic, copy, null_resettable) CIVector *inputSunPosition;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputSunIntensity;
+
+@property (nonatomic, copy, null_resettable) CIVector *inputViewPointOffset;
+
+@end
diff --git a/Pods/Vivid/Sources/YUCISkyGenerator.m b/Pods/Vivid/Sources/YUCISkyGenerator.m
new file mode 100644
index 0000000..a874031
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCISkyGenerator.m
@@ -0,0 +1,78 @@
+//
+// YUCISkyGenerator.m
+// Pods
+//
+// Created by YuAo on 3/15/16.
+//
+//
+
+#import "YUCISkyGenerator.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCISkyGenerator
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCISkyGenerator class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryGenerator],
+ kCIAttributeFilterDisplayName: @"Sky Generator"}];
+ }
+ }
+ });
+}
+
++ (CIColorKernel *)filterKernel {
+ static CIColorKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCISkyGenerator class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIColorKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (CIVector *)inputExtent {
+ if (!_inputExtent) {
+ _inputExtent = [CIVector vectorWithCGRect:CGRectMake(0, 0, 640, 480)];
+ }
+ return _inputExtent;
+}
+
+- (CIVector *)inputViewPointOffset {
+ if (!_inputViewPointOffset) {
+ _inputViewPointOffset = [CIVector vectorWithX:0 Y:0.9];
+ }
+ return _inputViewPointOffset;
+}
+
+- (CIVector *)inputSunPosition {
+ if (!_inputSunPosition) {
+ _inputSunPosition = [CIVector vectorWithX:0 Y:0.1 Z:-1.0];
+ }
+ return _inputSunPosition;
+}
+
+- (NSNumber *)inputSunIntensity {
+ if (!_inputSunIntensity) {
+ _inputSunIntensity = @30;
+ }
+ return _inputSunIntensity;
+}
+
+- (void)setDefaults {
+ self.inputExtent = nil;
+ self.inputViewPointOffset = nil;
+ self.inputSunPosition = nil;
+ self.inputSunIntensity = nil;
+}
+
+- (CIImage *)outputImage {
+ return [[YUCISkyGenerator filterKernel] applyWithExtent:self.inputExtent.CGRectValue
+ arguments:@[self.inputExtent,self.inputViewPointOffset,self.inputSunPosition,self.inputSunIntensity]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIStarfieldGenerator.cikernel b/Pods/Vivid/Sources/YUCIStarfieldGenerator.cikernel
new file mode 100644
index 0000000..8787085
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIStarfieldGenerator.cikernel
@@ -0,0 +1,72 @@
+// Star Nest by Pablo Román Andrioli
+
+// This content is under the MIT License.
+
+//mat2 is not supported by core image, use vec4. https://developer.apple.com/library/mac/documentation/GraphicsImaging/Reference/CIKernelLangRef/ci_gslang_ext.html
+vec2 vec2MultiplyMat2(vec2 v, vec4 m) {
+ return vec2(m.x * v.x + m.y * v.y, m.z * v.x + m.w * v.y);
+}
+
+kernel vec4 coreImageKernel(vec4 inputExtent, float inputTime, vec2 inputRotation)
+{
+ const int iterations = 17;
+ const float formuparam = 0.53;
+
+ const int volsteps = 20;
+ const float stepsize = 0.1;
+
+ const float zoom = 0.800;
+ const float tile = 0.850;
+ const float speed = 0.010;
+
+ const float brightness = 0.0015;
+ const float darkmatter = 0.300;
+ const float distfading = 0.730;
+ const float saturation = 0.850;
+
+ vec2 fragCoord = destCoord();
+ vec2 iResolution = inputExtent.zw;
+
+ //get coords and direction
+ vec2 uv=fragCoord.xy/iResolution.xy-.5;
+ uv.y*=iResolution.y/iResolution.x;
+ vec3 dir=vec3(uv*zoom,1.);
+ float time=inputTime*speed+.25;
+
+ //mouse rotation
+ vec2 iMouse = inputRotation;
+ float a1=.5+iMouse.x/iResolution.x*2.;
+ float a2=.8+iMouse.y/iResolution.y*2.;
+ vec4 rot1=vec4(cos(a1),sin(a1),-sin(a1),cos(a1));
+ vec4 rot2=vec4(cos(a2),sin(a2),-sin(a2),cos(a2));
+ dir.xz = vec2MultiplyMat2(dir.xz,rot1);
+ dir.xy = vec2MultiplyMat2(dir.xy,rot2);
+ vec3 from=vec3(1.,.5,0.5);
+ from+=vec3(time*2.,time,-2.);
+ from.xz = vec2MultiplyMat2(from.xz,rot1);
+ from.xy = vec2MultiplyMat2(from.xy,rot2);
+
+ //volumetric rendering
+ float s=0.1,fade=1.;
+ vec3 v=vec3(0.);
+ for (int r=0; r6) { fade*=1.-dm; } // dark matter, don't render near
+
+ v+=fade;
+ v+=vec3(s,s*s,s*s*s*s)*a*brightness*fade; // coloring based on distance
+ fade*=distfading; // distance fading
+ s+=stepsize;
+ }
+ v=mix(vec3(length(v)),v,saturation); //color adjust
+ return vec4(v*.01,1.);
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCIStarfieldGenerator.h b/Pods/Vivid/Sources/YUCIStarfieldGenerator.h
new file mode 100644
index 0000000..03702a1
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIStarfieldGenerator.h
@@ -0,0 +1,19 @@
+//
+// YUCIStarNestGenerator.h
+// Pods
+//
+// Created by YuAo on 2/4/16.
+//
+//
+
+#import
+
+@interface YUCIStarfieldGenerator : CIFilter
+
+@property (nonatomic, copy, null_resettable) CIVector *inputExtent;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputTime;
+
+@property (nonatomic, copy, null_resettable) CIVector *inputRotation;
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIStarfieldGenerator.m b/Pods/Vivid/Sources/YUCIStarfieldGenerator.m
new file mode 100644
index 0000000..0b53163
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIStarfieldGenerator.m
@@ -0,0 +1,70 @@
+//
+// YUCIStarNestGenerator.m
+// Pods
+//
+// Created by YuAo on 2/4/16.
+//
+//
+
+#import "YUCIStarfieldGenerator.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCIStarfieldGenerator
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCIStarfieldGenerator class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryGenerator],
+ kCIAttributeFilterDisplayName: @"Starfield Generator"}];
+ }
+ }
+ });
+}
+
++ (CIColorKernel *)filterKernel {
+ static CIColorKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCIStarfieldGenerator class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIColorKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (CIVector *)inputExtent {
+ if (!_inputExtent) {
+ _inputExtent = [CIVector vectorWithCGRect:CGRectMake(0, 0, 640, 480)];
+ }
+ return _inputExtent;
+}
+
+- (NSNumber *)inputTime {
+ if (!_inputTime) {
+ _inputTime = @(0);
+ }
+ return _inputTime;
+}
+
+- (CIVector *)inputRotation {
+ if (!_inputRotation) {
+ _inputRotation = [CIVector vectorWithX:0 Y:0];
+ }
+ return _inputRotation;
+}
+
+- (void)setDefaults {
+ self.inputExtent = nil;
+ self.inputTime = nil;
+ self.inputRotation = nil;
+}
+
+- (CIImage *)outputImage {
+ return [[YUCIStarfieldGenerator filterKernel] applyWithExtent:self.inputExtent.CGRectValue
+ arguments:@[self.inputExtent,self.inputTime,self.inputRotation]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCISurfaceBlur.cikernel b/Pods/Vivid/Sources/YUCISurfaceBlur.cikernel
new file mode 100644
index 0000000..d24c7ee
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCISurfaceBlur.cikernel
@@ -0,0 +1,47 @@
+float normpdf(in float x, in float sigma)
+{
+ return 0.39894*exp(-0.5*x*x/(sigma*sigma))/sigma;
+}
+
+float normpdf3(in vec3 v, in float sigma)
+{
+ return 0.39894*exp(-0.5*dot(v,v)/(sigma*sigma))/sigma;
+}
+
+kernel vec4 filterKernel(sampler inputImage, float bsigma) {
+ const int SAMPLES_COUNT = MACRO_SAMPLES_COUNT;
+
+ float GAUSSIAN_WEIGHTS[SAMPLES_COUNT];
+ MACRO_SETUP_GAUSSIAN_WEIGHTS
+ /*
+ float sigma = float(kSize);
+ for (int j = 0; j <= kSize; ++j)
+ {
+ GAUSSIAN_WEIGHTS[kSize+j] = GAUSSIAN_WEIGHTS[kSize-j] = normpdf(float(j), sigma);
+ }
+ */
+
+ const int kSize = (SAMPLES_COUNT-1)/2;
+ vec3 finalColor = vec3(0.0);
+
+ vec3 c = sample(inputImage,samplerCoord(inputImage)).rgb;
+
+ float Z = 0.0;
+
+ vec3 cc;
+ float factor;
+ float bZ = 1.0/normpdf(0.0, bsigma);
+
+ for (int i=-kSize; i <= kSize; ++i)
+ {
+ for (int j=-kSize; j <= kSize; ++j)
+ {
+ cc = sample(inputImage,samplerTransform(inputImage, destCoord().xy + vec2(float(i),float(j)))).rgb;
+ factor = normpdf3(cc-c, bsigma)*bZ*GAUSSIAN_WEIGHTS[kSize+j]*GAUSSIAN_WEIGHTS[kSize+i];
+ Z += factor;
+ finalColor += factor*cc;
+ }
+ }
+
+ return vec4(finalColor/Z, 1.0);
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCISurfaceBlur.h b/Pods/Vivid/Sources/YUCISurfaceBlur.h
new file mode 100644
index 0000000..09edd72
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCISurfaceBlur.h
@@ -0,0 +1,19 @@
+//
+// YUCISurfaceBlur.h
+// Pods
+//
+// Created by YuAo on 2/3/16.
+//
+//
+
+#import
+
+@interface YUCISurfaceBlur : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputRadius; //default 10
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputThreshold; //0.0-255.0; //default 10
+
+@end
diff --git a/Pods/Vivid/Sources/YUCISurfaceBlur.m b/Pods/Vivid/Sources/YUCISurfaceBlur.m
new file mode 100644
index 0000000..6ebbfbf
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCISurfaceBlur.m
@@ -0,0 +1,97 @@
+//
+// YUCISurfaceBlur.m
+// Pods
+//
+// Created by YuAo on 2/3/16.
+//
+//
+
+#import "YUCISurfaceBlur.h"
+#import "YUCIFilterUtilities.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCISurfaceBlur
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCISurfaceBlur class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryBlur],
+ kCIAttributeFilterDisplayName: @"Surface Blur"}];
+ }
+ }
+ });
+}
+
+static NSDictionary *YUCISurfaceBlurKernels;
+
++ (CIKernel *)filterKernelForRadius:(NSInteger)radius {
+ CIKernel *kernel = YUCISurfaceBlurKernels[@(radius)];
+
+ if (kernel) {
+ return kernel;
+ } else {
+ NSMutableArray *wights = [NSMutableArray array];
+ for (NSInteger i = 0; i < radius; ++i) {
+ double wight = YUCIGaussianDistributionPDF(i, radius);
+ [wights addObject:@(wight)];
+ }
+
+ NSString *gaussianWeightsSetupProgram = @"";
+ for (NSInteger i = 0; i < wights.count; ++i) {
+ gaussianWeightsSetupProgram = [gaussianWeightsSetupProgram stringByAppendingFormat:@"GAUSSIAN_WEIGHTS[%@] = GAUSSIAN_WEIGHTS[%@] = %@; \n",@(radius - 1 - i),@(radius - 1 + i),wights[i]];
+ }
+
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCISurfaceBlur class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernelString = [kernelString stringByReplacingOccurrencesOfString:@"MACRO_SAMPLES_COUNT" withString:@(radius * 2).stringValue];
+ kernelString = [kernelString stringByReplacingOccurrencesOfString:@"MACRO_SETUP_GAUSSIAN_WEIGHTS" withString:gaussianWeightsSetupProgram];
+ kernel = [CIKernel kernelWithString:kernelString];
+
+ NSMutableDictionary *newKernels = [NSMutableDictionary dictionaryWithDictionary:YUCISurfaceBlurKernels];
+ [newKernels setObject:kernel forKey:@(radius)];
+ YUCISurfaceBlurKernels = newKernels.copy;
+
+ return kernel;
+ }
+}
+
+- (NSNumber *)inputRadius {
+ if (!_inputRadius) {
+ _inputRadius = @(10);
+ }
+ return _inputRadius;
+}
+
+- (NSNumber *)inputThreshold {
+ if (!_inputThreshold) {
+ _inputThreshold = @(10);
+ }
+ return _inputThreshold;
+}
+
+- (void)setDefaults {
+ self.inputRadius = nil;
+ self.inputThreshold = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ if (self.inputRadius.integerValue <= 0 || self.inputThreshold.doubleValue < 0) {
+ return self.inputImage;
+ }
+
+ CIKernel *kernel = [YUCISurfaceBlur filterKernelForRadius:[self.inputRadius integerValue]];
+ return [kernel applyWithExtent:self.inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return CGRectInset(destRect, -self.inputRadius.integerValue, -self.inputRadius.integerValue);
+ } arguments:@[self.inputImage.imageByClampingToExtent,@(self.inputThreshold.doubleValue/255.0 * 2.0)]];
+}
+
+
+@end
diff --git a/Pods/Vivid/Sources/YUCITriangularPixellate.cikernel b/Pods/Vivid/Sources/YUCITriangularPixellate.cikernel
new file mode 100644
index 0000000..4695d42
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCITriangularPixellate.cikernel
@@ -0,0 +1,31 @@
+
+kernel vec4 filterKernel(sampler inputImage, vec2 center, float scale, float tan_halfInputAngle) {
+ const int MSAA_SAMPLE_COUNT = 4; //4xMSAA RGSS
+ vec2 MSAASampleCoords[MSAA_SAMPLE_COUNT];
+ MSAASampleCoords[0] = destCoord() + vec2(-0.375,0.125);
+ MSAASampleCoords[1] = destCoord() + vec2(0.125,0.375);
+ MSAASampleCoords[2] = destCoord() + vec2(0.375,-0.125);
+ MSAASampleCoords[3] = destCoord() + vec2(-0.125,-0.375);
+
+ float scaleY = scale/2.0 / tan_halfInputAngle;
+
+ vec4 color = vec4(0.0);
+ for (int i = 0; i < MSAA_SAMPLE_COUNT; ++i) {
+ vec2 sampleCoord = MSAASampleCoords[i] - center;
+ float nx = floor(sampleCoord.x/scale);
+ float ny = floor(sampleCoord.y/scaleY);
+ float ly = sampleCoord.y - ny * scaleY;
+ float lx = sampleCoord.x - nx * scale;
+ float dx = (scaleY - ly) * tan_halfInputAngle;
+ vec2 coord;
+ if (lx > scale/2.0 + dx) {
+ coord = vec2((nx + 1.0) * scale, (ny + 0.5) * scaleY);
+ } else if (lx < scale/2.0 - dx) {
+ coord = vec2(nx * scale, (ny + 0.5) * scaleY);
+ } else {
+ coord = vec2((nx + 0.5) * scale, (ny + 0.5) * scaleY);
+ }
+ color += sample(inputImage,samplerTransform(inputImage,coord + center));
+ }
+ return color/float(MSAA_SAMPLE_COUNT);
+}
\ No newline at end of file
diff --git a/Pods/Vivid/Sources/YUCITriangularPixellate.h b/Pods/Vivid/Sources/YUCITriangularPixellate.h
new file mode 100644
index 0000000..830e710
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCITriangularPixellate.h
@@ -0,0 +1,21 @@
+//
+// YUCITriangularPixellate.h
+// Pods
+//
+// Created by YuAo on 2/9/16.
+//
+//
+
+#import
+
+@interface YUCITriangularPixellate : CIFilter
+
+@property (nonatomic, strong, nullable) CIImage *inputImage;
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputVertexAngle; //default: M_PI/2.0
+
+@property (nonatomic, copy, null_resettable) NSNumber *inputScale; //default: 20.0
+
+@property (nonatomic, copy, null_resettable) CIVector *inputCenter; //default: (0,0)
+
+@end
diff --git a/Pods/Vivid/Sources/YUCITriangularPixellate.m b/Pods/Vivid/Sources/YUCITriangularPixellate.m
new file mode 100644
index 0000000..eaa7762
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCITriangularPixellate.m
@@ -0,0 +1,77 @@
+//
+// YUCITriangularPixellate.m
+// Pods
+//
+// Created by YuAo on 2/9/16.
+//
+//
+
+#import "YUCITriangularPixellate.h"
+#import "YUCIFilterConstructor.h"
+
+@implementation YUCITriangularPixellate
+
++ (void)load {
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ @autoreleasepool {
+ if ([CIFilter respondsToSelector:@selector(registerFilterName:constructor:classAttributes:)]) {
+ [CIFilter registerFilterName:NSStringFromClass([YUCITriangularPixellate class])
+ constructor:[YUCIFilterConstructor constructor]
+ classAttributes:@{kCIAttributeFilterCategories: @[kCICategoryStillImage,kCICategoryVideo,kCICategoryStylize],
+ kCIAttributeFilterDisplayName: @"Triangular Pixellate"}];
+ }
+ }
+ });
+}
+
++ (CIKernel *)filterKernel {
+ static CIKernel *kernel;
+ static dispatch_once_t onceToken;
+ dispatch_once(&onceToken, ^{
+ NSString *kernelString = [[NSString alloc] initWithContentsOfURL:[[NSBundle bundleForClass:self] URLForResource:NSStringFromClass([YUCITriangularPixellate class]) withExtension:@"cikernel"] encoding:NSUTF8StringEncoding error:nil];
+ kernel = [CIKernel kernelWithString:kernelString];
+ });
+ return kernel;
+}
+
+- (NSNumber *)inputScale {
+ if (!_inputScale) {
+ _inputScale = @(20);
+ }
+ return _inputScale;
+}
+
+- (NSNumber *)inputVertexAngle {
+ if (!_inputVertexAngle) {
+ _inputVertexAngle = @(M_PI/2.0);
+ }
+ return _inputVertexAngle;
+}
+
+- (CIVector *)inputCenter {
+ if (!_inputCenter) {
+ _inputCenter = [CIVector vectorWithX:0 Y:0];
+ }
+ return _inputCenter;
+}
+
+- (void)setDefaults {
+ self.inputScale = nil;
+ self.inputVertexAngle = nil;
+ self.inputCenter = nil;
+}
+
+- (CIImage *)outputImage {
+ if (!self.inputImage) {
+ return nil;
+ }
+
+ CGFloat tanHalfInputAngle = tan(self.inputVertexAngle.floatValue/2.0);
+ return [[YUCITriangularPixellate filterKernel] applyWithExtent:self.inputImage.extent
+ roiCallback:^CGRect(int index, CGRect destRect) {
+ return CGRectInset(destRect, -self.inputScale.floatValue, -self.inputScale.floatValue/2.0 * tanHalfInputAngle);
+ } arguments:@[self.inputImage.imageByClampingToExtent,self.inputCenter,self.inputScale,@(tanHalfInputAngle)]];
+}
+
+@end
diff --git a/Pods/Vivid/Sources/YUCIUtilities.h b/Pods/Vivid/Sources/YUCIUtilities.h
new file mode 100644
index 0000000..f971373
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIUtilities.h
@@ -0,0 +1,22 @@
+//
+// YUCIUtilities.h
+// Pods
+//
+// Created by YuAo on 2/17/16.
+//
+//
+
+#import
+
+#define YUCI_METAMACRO_CONCAT(A, B) \
+YUCI_METAMACRO_CONCAT_(A, B)
+
+#define YUCI_METAMACRO_CONCAT_(A, B) A ## B
+
+#define YUCIDefer \
+ try {} @finally {} \
+ __strong YUCIDeferCleanupBlock YUCI_METAMACRO_CONCAT(YUCIDeferBlock_, __LINE__) __attribute__((cleanup(YUCIDeferExecuteCleanupBlock), unused)) = ^
+
+typedef void (^YUCIDeferCleanupBlock)();
+
+void YUCIDeferExecuteCleanupBlock (__strong YUCIDeferCleanupBlock *block);
diff --git a/Pods/Vivid/Sources/YUCIUtilities.m b/Pods/Vivid/Sources/YUCIUtilities.m
new file mode 100644
index 0000000..2376590
--- /dev/null
+++ b/Pods/Vivid/Sources/YUCIUtilities.m
@@ -0,0 +1,13 @@
+//
+// YUCIUtilities.m
+// Pods
+//
+// Created by YuAo on 2/17/16.
+//
+//
+
+#import "YUCIUtilities.h"
+
+void YUCIDeferExecuteCleanupBlock (__strong YUCIDeferCleanupBlock *block) {
+ (*block)();
+}
diff --git a/iOS-Depth-Sampler.xcodeproj/project.pbxproj b/iOS-Depth-Sampler.xcodeproj/project.pbxproj
new file mode 100644
index 0000000..a3c0268
--- /dev/null
+++ b/iOS-Depth-Sampler.xcodeproj/project.pbxproj
@@ -0,0 +1,598 @@
+// !$*UTF8*$!
+{
+ archiveVersion = 1;
+ classes = {
+ };
+ objectVersion = 50;
+ objects = {
+
+/* Begin PBXBuildFile section */
+ 1B4D7FEC5A6D1DAFCE88D110 /* Pods_iOS_Depth_Sampler.framework in Frameworks */ = {isa = PBXBuildFile; fileRef = FDB82C9008285D580EA95E40 /* Pods_iOS_Depth_Sampler.framework */; };
+ 8A171F0B2148FE4100121E80 /* RealtimeDepthViewController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A171F062148FE4100121E80 /* RealtimeDepthViewController.swift */; };
+ 8A171F0C2148FE4100121E80 /* RealtimeDepth.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8A171F072148FE4100121E80 /* RealtimeDepth.storyboard */; };
+ 8A171F13214905D400121E80 /* CVPixelBuffer+CIImage.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A171F12214905D400121E80 /* CVPixelBuffer+CIImage.swift */; };
+ 8A171F15214928BB00121E80 /* AVDepthData+Utils.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A171F14214928BB00121E80 /* AVDepthData+Utils.swift */; };
+ 8A171F1A214930D100121E80 /* RealtimeDepthMaskViewController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A171F17214930D100121E80 /* RealtimeDepthMaskViewController.swift */; };
+ 8A171F1B214930D100121E80 /* RealtimeDepthMask.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8A171F18214930D100121E80 /* RealtimeDepthMask.storyboard */; };
+ 8A603D87214945A900F848A4 /* PortraitMatteViewController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A603D85214945A900F848A4 /* PortraitMatteViewController.swift */; };
+ 8A603D88214945A900F848A4 /* PortraitMatte.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8A603D86214945A900F848A4 /* PortraitMatte.storyboard */; };
+ 8A603D8A2149460E00F848A4 /* PhotosUtils.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A603D892149460E00F848A4 /* PhotosUtils.swift */; };
+ 8A603D8C2149590F00F848A4 /* UIAlertController+Utils.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A603D8B2149590F00F848A4 /* UIAlertController+Utils.swift */; };
+ 8A702F98212A3BD3004039F7 /* AppDelegate.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A702F97212A3BD3004039F7 /* AppDelegate.swift */; };
+ 8A702F9F212A3BD5004039F7 /* Assets.xcassets in Resources */ = {isa = PBXBuildFile; fileRef = 8A702F9E212A3BD5004039F7 /* Assets.xcassets */; };
+ 8A702FA2212A3BD5004039F7 /* LaunchScreen.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8A702FA0212A3BD5004039F7 /* LaunchScreen.storyboard */; };
+ 8A702FAD212A3CDE004039F7 /* VideoCameraType.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A702FAA212A3CDE004039F7 /* VideoCameraType.swift */; };
+ 8A702FAE212A3CDE004039F7 /* VideoCapture.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A702FAB212A3CDE004039F7 /* VideoCapture.swift */; };
+ 8A702FAF212A3CDE004039F7 /* AVCaptureDevice+Extension.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A702FAC212A3CDE004039F7 /* AVCaptureDevice+Extension.swift */; };
+ 8A7F88042148199000170436 /* ARKitDepth.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8A7F88022148199000170436 /* ARKitDepth.storyboard */; };
+ 8A7F88052148199000170436 /* ARKitDepthViewController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A7F88032148199000170436 /* ARKitDepthViewController.swift */; };
+ 8A7F8807214819FF00170436 /* TrackingState+Description.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8A7F8806214819FF00170436 /* TrackingState+Description.swift */; };
+ 8AAF370F21480CAC006A551B /* Main.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8AAF370E21480CAC006A551B /* Main.storyboard */; };
+ 8AAF371321480D32006A551B /* RootViewCell.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AAF371021480D31006A551B /* RootViewCell.swift */; };
+ 8AAF371421480D32006A551B /* RootViewController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AAF371121480D32006A551B /* RootViewController.swift */; };
+ 8AAF371521480D32006A551B /* SampleDataSource.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AAF371221480D32006A551B /* SampleDataSource.swift */; };
+ 8AAF371B21481369006A551B /* UIImage+CVPixelBuffer.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AAF371821481369006A551B /* UIImage+CVPixelBuffer.swift */; };
+ 8AAF371C21481369006A551B /* DepthFromCameraRollViewController.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AAF371921481369006A551B /* DepthFromCameraRollViewController.swift */; };
+ 8AAF371D2148136A006A551B /* CGImageSource+Depth.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AAF371A21481369006A551B /* CGImageSource+Depth.swift */; };
+ 8AAF371F214814BE006A551B /* DepthFromCameraRoll.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 8AAF371E214814BE006A551B /* DepthFromCameraRoll.storyboard */; };
+ 8AB76029213628440051C3D1 /* MetalRenderer.swift in Sources */ = {isa = PBXBuildFile; fileRef = 8AB76028213628440051C3D1 /* MetalRenderer.swift */; };
+ 8AB7602B213631AD0051C3D1 /* PassThrough.metal in Sources */ = {isa = PBXBuildFile; fileRef = 8AB7602A213631AD0051C3D1 /* PassThrough.metal */; };
+/* End PBXBuildFile section */
+
+/* Begin PBXFileReference section */
+ 8A171F062148FE4100121E80 /* RealtimeDepthViewController.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = RealtimeDepthViewController.swift; sourceTree = ""; };
+ 8A171F082148FE4100121E80 /* Base */ = {isa = PBXFileReference; lastKnownFileType = file.storyboard; name = Base; path = Base.lproj/RealtimeDepth.storyboard; sourceTree = ""; };
+ 8A171F12214905D400121E80 /* CVPixelBuffer+CIImage.swift */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.swift; path = "CVPixelBuffer+CIImage.swift"; sourceTree = ""; };
+ 8A171F14214928BB00121E80 /* AVDepthData+Utils.swift */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.swift; path = "AVDepthData+Utils.swift"; sourceTree = ""; };
+ 8A171F17214930D100121E80 /* RealtimeDepthMaskViewController.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = RealtimeDepthMaskViewController.swift; sourceTree = ""; };
+ 8A171F19214930D100121E80 /* Base */ = {isa = PBXFileReference; lastKnownFileType = file.storyboard; name = Base; path = Base.lproj/RealtimeDepthMask.storyboard; sourceTree = ""; };
+ 8A603D85214945A900F848A4 /* PortraitMatteViewController.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = PortraitMatteViewController.swift; sourceTree = ""; };
+ 8A603D86214945A900F848A4 /* PortraitMatte.storyboard */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = file.storyboard; path = PortraitMatte.storyboard; sourceTree = ""; };
+ 8A603D892149460E00F848A4 /* PhotosUtils.swift */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.swift; path = PhotosUtils.swift; sourceTree = ""; };
+ 8A603D8B2149590F00F848A4 /* UIAlertController+Utils.swift */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.swift; path = "UIAlertController+Utils.swift"; sourceTree = ""; };
+ 8A702F94212A3BD3004039F7 /* iOS-Depth-Sampler.app */ = {isa = PBXFileReference; explicitFileType = wrapper.application; includeInIndex = 0; path = "iOS-Depth-Sampler.app"; sourceTree = BUILT_PRODUCTS_DIR; };
+ 8A702F97212A3BD3004039F7 /* AppDelegate.swift */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.swift; path = AppDelegate.swift; sourceTree = ""; };
+ 8A702F9E212A3BD5004039F7 /* Assets.xcassets */ = {isa = PBXFileReference; lastKnownFileType = folder.assetcatalog; path = Assets.xcassets; sourceTree = ""; };
+ 8A702FA1212A3BD5004039F7 /* Base */ = {isa = PBXFileReference; lastKnownFileType = file.storyboard; name = Base; path = Base.lproj/LaunchScreen.storyboard; sourceTree = ""; };
+ 8A702FA3212A3BD5004039F7 /* Info.plist */ = {isa = PBXFileReference; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = ""; };
+ 8A702FAA212A3CDE004039F7 /* VideoCameraType.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = VideoCameraType.swift; sourceTree = ""; };
+ 8A702FAB212A3CDE004039F7 /* VideoCapture.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = VideoCapture.swift; sourceTree = ""; };
+ 8A702FAC212A3CDE004039F7 /* AVCaptureDevice+Extension.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = "AVCaptureDevice+Extension.swift"; sourceTree = ""; };
+ 8A7F88022148199000170436 /* ARKitDepth.storyboard */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = file.storyboard; path = ARKitDepth.storyboard; sourceTree = ""; };
+ 8A7F88032148199000170436 /* ARKitDepthViewController.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = ARKitDepthViewController.swift; sourceTree = ""; };
+ 8A7F8806214819FF00170436 /* TrackingState+Description.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = "TrackingState+Description.swift"; sourceTree = ""; };
+ 8AABBCCC2136463900C938EE /* iOS-Depth-Sampler-Bridging-Header.h */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.h; path = "iOS-Depth-Sampler-Bridging-Header.h"; sourceTree = ""; };
+ 8AAF370E21480CAC006A551B /* Main.storyboard */ = {isa = PBXFileReference; lastKnownFileType = file.storyboard; path = Main.storyboard; sourceTree = ""; };
+ 8AAF371021480D31006A551B /* RootViewCell.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = RootViewCell.swift; sourceTree = ""; };
+ 8AAF371121480D32006A551B /* RootViewController.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = RootViewController.swift; sourceTree = ""; };
+ 8AAF371221480D32006A551B /* SampleDataSource.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = SampleDataSource.swift; sourceTree = ""; };
+ 8AAF371821481369006A551B /* UIImage+CVPixelBuffer.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = "UIImage+CVPixelBuffer.swift"; sourceTree = ""; };
+ 8AAF371921481369006A551B /* DepthFromCameraRollViewController.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = DepthFromCameraRollViewController.swift; sourceTree = ""; };
+ 8AAF371A21481369006A551B /* CGImageSource+Depth.swift */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.swift; path = "CGImageSource+Depth.swift"; sourceTree = ""; };
+ 8AAF371E214814BE006A551B /* DepthFromCameraRoll.storyboard */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = file.storyboard; path = DepthFromCameraRoll.storyboard; sourceTree = ""; };
+ 8AB76028213628440051C3D1 /* MetalRenderer.swift */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.swift; path = MetalRenderer.swift; sourceTree = ""; };
+ 8AB7602A213631AD0051C3D1 /* PassThrough.metal */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.metal; path = PassThrough.metal; sourceTree = ""; };
+ 8FA83CA5E0F8F1F5F2E5DF63 /* Pods-iOS-Depth-Sampler.release.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-iOS-Depth-Sampler.release.xcconfig"; path = "Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.release.xcconfig"; sourceTree = ""; };
+ DDC3399A5A9E8877FB64BCD8 /* Pods-iOS-Depth-Sampler.debug.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-iOS-Depth-Sampler.debug.xcconfig"; path = "Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler.debug.xcconfig"; sourceTree = ""; };
+ FDB82C9008285D580EA95E40 /* Pods_iOS_Depth_Sampler.framework */ = {isa = PBXFileReference; explicitFileType = wrapper.framework; includeInIndex = 0; path = Pods_iOS_Depth_Sampler.framework; sourceTree = BUILT_PRODUCTS_DIR; };
+/* End PBXFileReference section */
+
+/* Begin PBXFrameworksBuildPhase section */
+ 8A702F91212A3BD3004039F7 /* Frameworks */ = {
+ isa = PBXFrameworksBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 1B4D7FEC5A6D1DAFCE88D110 /* Pods_iOS_Depth_Sampler.framework in Frameworks */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXFrameworksBuildPhase section */
+
+/* Begin PBXGroup section */
+ 2824CEA4531F10AD95165322 /* Pods */ = {
+ isa = PBXGroup;
+ children = (
+ DDC3399A5A9E8877FB64BCD8 /* Pods-iOS-Depth-Sampler.debug.xcconfig */,
+ 8FA83CA5E0F8F1F5F2E5DF63 /* Pods-iOS-Depth-Sampler.release.xcconfig */,
+ );
+ name = Pods;
+ sourceTree = "";
+ };
+ 6ECE07E18B1808EB59C8741A /* Frameworks */ = {
+ isa = PBXGroup;
+ children = (
+ FDB82C9008285D580EA95E40 /* Pods_iOS_Depth_Sampler.framework */,
+ );
+ name = Frameworks;
+ sourceTree = "";
+ };
+ 8A171F052148FE4100121E80 /* Realtime-Depth */ = {
+ isa = PBXGroup;
+ children = (
+ 8A171F062148FE4100121E80 /* RealtimeDepthViewController.swift */,
+ 8A171F072148FE4100121E80 /* RealtimeDepth.storyboard */,
+ );
+ path = "Realtime-Depth";
+ sourceTree = "";
+ };
+ 8A171F11214905A400121E80 /* Utils */ = {
+ isa = PBXGroup;
+ children = (
+ 8A171F12214905D400121E80 /* CVPixelBuffer+CIImage.swift */,
+ 8AAF371A21481369006A551B /* CGImageSource+Depth.swift */,
+ 8AAF371821481369006A551B /* UIImage+CVPixelBuffer.swift */,
+ 8A171F14214928BB00121E80 /* AVDepthData+Utils.swift */,
+ 8A603D892149460E00F848A4 /* PhotosUtils.swift */,
+ 8A603D8B2149590F00F848A4 /* UIAlertController+Utils.swift */,
+ );
+ path = Utils;
+ sourceTree = "";
+ };
+ 8A171F16214930D100121E80 /* Realtime-Mask */ = {
+ isa = PBXGroup;
+ children = (
+ 8A171F17214930D100121E80 /* RealtimeDepthMaskViewController.swift */,
+ 8A171F18214930D100121E80 /* RealtimeDepthMask.storyboard */,
+ );
+ path = "Realtime-Mask";
+ sourceTree = "";
+ };
+ 8A3D1A74213E5F2F0061470F /* Renderer */ = {
+ isa = PBXGroup;
+ children = (
+ 8AB7602A213631AD0051C3D1 /* PassThrough.metal */,
+ 8AB76028213628440051C3D1 /* MetalRenderer.swift */,
+ );
+ path = Renderer;
+ sourceTree = "";
+ };
+ 8A603D84214945A900F848A4 /* Portrait Matte */ = {
+ isa = PBXGroup;
+ children = (
+ 8A603D85214945A900F848A4 /* PortraitMatteViewController.swift */,
+ 8A603D86214945A900F848A4 /* PortraitMatte.storyboard */,
+ );
+ path = "Portrait Matte";
+ sourceTree = "";
+ };
+ 8A603D8D21495B8A00F848A4 /* Samples */ = {
+ isa = PBXGroup;
+ children = (
+ 8A171F052148FE4100121E80 /* Realtime-Depth */,
+ 8A171F16214930D100121E80 /* Realtime-Mask */,
+ 8AAF371721481369006A551B /* Depth-from-Camera-Roll */,
+ 8A603D84214945A900F848A4 /* Portrait Matte */,
+ 8A7F88012148199000170436 /* ARKit */,
+ );
+ path = Samples;
+ sourceTree = "";
+ };
+ 8A702F8B212A3BD3004039F7 = {
+ isa = PBXGroup;
+ children = (
+ 8A702F96212A3BD3004039F7 /* iOS-Depth-Sampler */,
+ 8A702F95212A3BD3004039F7 /* Products */,
+ 2824CEA4531F10AD95165322 /* Pods */,
+ 6ECE07E18B1808EB59C8741A /* Frameworks */,
+ );
+ sourceTree = "";
+ };
+ 8A702F95212A3BD3004039F7 /* Products */ = {
+ isa = PBXGroup;
+ children = (
+ 8A702F94212A3BD3004039F7 /* iOS-Depth-Sampler.app */,
+ );
+ name = Products;
+ sourceTree = "";
+ };
+ 8A702F96212A3BD3004039F7 /* iOS-Depth-Sampler */ = {
+ isa = PBXGroup;
+ children = (
+ 8AABBCCC2136463900C938EE /* iOS-Depth-Sampler-Bridging-Header.h */,
+ 8A702FA3212A3BD5004039F7 /* Info.plist */,
+ 8A702F9E212A3BD5004039F7 /* Assets.xcassets */,
+ 8A702FA0212A3BD5004039F7 /* LaunchScreen.storyboard */,
+ 8AAF370E21480CAC006A551B /* Main.storyboard */,
+ 8A702F97212A3BD3004039F7 /* AppDelegate.swift */,
+ 8AAF371021480D31006A551B /* RootViewCell.swift */,
+ 8AAF371121480D32006A551B /* RootViewController.swift */,
+ 8AAF371221480D32006A551B /* SampleDataSource.swift */,
+ 8A171F11214905A400121E80 /* Utils */,
+ 8A702FA9212A3CDE004039F7 /* VideoCapture */,
+ 8A3D1A74213E5F2F0061470F /* Renderer */,
+ 8A603D8D21495B8A00F848A4 /* Samples */,
+ );
+ path = "iOS-Depth-Sampler";
+ sourceTree = "";
+ };
+ 8A702FA9212A3CDE004039F7 /* VideoCapture */ = {
+ isa = PBXGroup;
+ children = (
+ 8A702FAA212A3CDE004039F7 /* VideoCameraType.swift */,
+ 8A702FAB212A3CDE004039F7 /* VideoCapture.swift */,
+ 8A702FAC212A3CDE004039F7 /* AVCaptureDevice+Extension.swift */,
+ );
+ path = VideoCapture;
+ sourceTree = "";
+ };
+ 8A7F88012148199000170436 /* ARKit */ = {
+ isa = PBXGroup;
+ children = (
+ 8A7F8806214819FF00170436 /* TrackingState+Description.swift */,
+ 8A7F88022148199000170436 /* ARKitDepth.storyboard */,
+ 8A7F88032148199000170436 /* ARKitDepthViewController.swift */,
+ );
+ path = ARKit;
+ sourceTree = "";
+ };
+ 8AAF371721481369006A551B /* Depth-from-Camera-Roll */ = {
+ isa = PBXGroup;
+ children = (
+ 8AAF371921481369006A551B /* DepthFromCameraRollViewController.swift */,
+ 8AAF371E214814BE006A551B /* DepthFromCameraRoll.storyboard */,
+ );
+ path = "Depth-from-Camera-Roll";
+ sourceTree = "";
+ };
+/* End PBXGroup section */
+
+/* Begin PBXNativeTarget section */
+ 8A702F93212A3BD3004039F7 /* iOS-Depth-Sampler */ = {
+ isa = PBXNativeTarget;
+ buildConfigurationList = 8A702FA6212A3BD5004039F7 /* Build configuration list for PBXNativeTarget "iOS-Depth-Sampler" */;
+ buildPhases = (
+ 04E184A9F0C6982EBCC0B6C1 /* [CP] Check Pods Manifest.lock */,
+ 8A702F90212A3BD3004039F7 /* Sources */,
+ 8A702F91212A3BD3004039F7 /* Frameworks */,
+ 8A702F92212A3BD3004039F7 /* Resources */,
+ 8D362FBD9C4404F36E8734DF /* [CP] Embed Pods Frameworks */,
+ );
+ buildRules = (
+ );
+ dependencies = (
+ );
+ name = "iOS-Depth-Sampler";
+ productName = "iOS-Depth-Sampler";
+ productReference = 8A702F94212A3BD3004039F7 /* iOS-Depth-Sampler.app */;
+ productType = "com.apple.product-type.application";
+ };
+/* End PBXNativeTarget section */
+
+/* Begin PBXProject section */
+ 8A702F8C212A3BD3004039F7 /* Project object */ = {
+ isa = PBXProject;
+ attributes = {
+ LastSwiftUpdateCheck = 1000;
+ LastUpgradeCheck = 1000;
+ ORGANIZATIONNAME = "Shuichi Tsutsumi";
+ TargetAttributes = {
+ 8A702F93212A3BD3004039F7 = {
+ CreatedOnToolsVersion = 10.0;
+ LastSwiftMigration = 1000;
+ };
+ };
+ };
+ buildConfigurationList = 8A702F8F212A3BD3004039F7 /* Build configuration list for PBXProject "iOS-Depth-Sampler" */;
+ compatibilityVersion = "Xcode 9.3";
+ developmentRegion = en;
+ hasScannedForEncodings = 0;
+ knownRegions = (
+ en,
+ Base,
+ );
+ mainGroup = 8A702F8B212A3BD3004039F7;
+ productRefGroup = 8A702F95212A3BD3004039F7 /* Products */;
+ projectDirPath = "";
+ projectRoot = "";
+ targets = (
+ 8A702F93212A3BD3004039F7 /* iOS-Depth-Sampler */,
+ );
+ };
+/* End PBXProject section */
+
+/* Begin PBXResourcesBuildPhase section */
+ 8A702F92212A3BD3004039F7 /* Resources */ = {
+ isa = PBXResourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 8A603D88214945A900F848A4 /* PortraitMatte.storyboard in Resources */,
+ 8A702FA2212A3BD5004039F7 /* LaunchScreen.storyboard in Resources */,
+ 8A171F0C2148FE4100121E80 /* RealtimeDepth.storyboard in Resources */,
+ 8A171F1B214930D100121E80 /* RealtimeDepthMask.storyboard in Resources */,
+ 8A702F9F212A3BD5004039F7 /* Assets.xcassets in Resources */,
+ 8AAF370F21480CAC006A551B /* Main.storyboard in Resources */,
+ 8A7F88042148199000170436 /* ARKitDepth.storyboard in Resources */,
+ 8AAF371F214814BE006A551B /* DepthFromCameraRoll.storyboard in Resources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXResourcesBuildPhase section */
+
+/* Begin PBXShellScriptBuildPhase section */
+ 04E184A9F0C6982EBCC0B6C1 /* [CP] Check Pods Manifest.lock */ = {
+ isa = PBXShellScriptBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ );
+ inputPaths = (
+ "${PODS_PODFILE_DIR_PATH}/Podfile.lock",
+ "${PODS_ROOT}/Manifest.lock",
+ );
+ name = "[CP] Check Pods Manifest.lock";
+ outputPaths = (
+ "$(DERIVED_FILE_DIR)/Pods-iOS-Depth-Sampler-checkManifestLockResult.txt",
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ shellPath = /bin/sh;
+ shellScript = "diff \"${PODS_PODFILE_DIR_PATH}/Podfile.lock\" \"${PODS_ROOT}/Manifest.lock\" > /dev/null\nif [ $? != 0 ] ; then\n # print error to STDERR\n echo \"error: The sandbox is not in sync with the Podfile.lock. Run 'pod install' or update your CocoaPods installation.\" >&2\n exit 1\nfi\n# This output is used by Xcode 'outputs' to avoid re-running this script phase.\necho \"SUCCESS\" > \"${SCRIPT_OUTPUT_FILE_0}\"\n";
+ showEnvVarsInLog = 0;
+ };
+ 8D362FBD9C4404F36E8734DF /* [CP] Embed Pods Frameworks */ = {
+ isa = PBXShellScriptBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ );
+ inputPaths = (
+ "${SRCROOT}/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-frameworks.sh",
+ "${BUILT_PRODUCTS_DIR}/CheckMarkView/CheckMarkView.framework",
+ "${BUILT_PRODUCTS_DIR}/SwiftAssetsPickerController/SwiftAssetsPickerController.framework",
+ "${BUILT_PRODUCTS_DIR}/Vivid/Vivid.framework",
+ );
+ name = "[CP] Embed Pods Frameworks";
+ outputPaths = (
+ "${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}/CheckMarkView.framework",
+ "${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}/SwiftAssetsPickerController.framework",
+ "${TARGET_BUILD_DIR}/${FRAMEWORKS_FOLDER_PATH}/Vivid.framework",
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ shellPath = /bin/sh;
+ shellScript = "\"${SRCROOT}/Pods/Target Support Files/Pods-iOS-Depth-Sampler/Pods-iOS-Depth-Sampler-frameworks.sh\"\n";
+ showEnvVarsInLog = 0;
+ };
+/* End PBXShellScriptBuildPhase section */
+
+/* Begin PBXSourcesBuildPhase section */
+ 8A702F90212A3BD3004039F7 /* Sources */ = {
+ isa = PBXSourcesBuildPhase;
+ buildActionMask = 2147483647;
+ files = (
+ 8A702FAE212A3CDE004039F7 /* VideoCapture.swift in Sources */,
+ 8AB7602B213631AD0051C3D1 /* PassThrough.metal in Sources */,
+ 8A7F88052148199000170436 /* ARKitDepthViewController.swift in Sources */,
+ 8AAF371421480D32006A551B /* RootViewController.swift in Sources */,
+ 8AAF371C21481369006A551B /* DepthFromCameraRollViewController.swift in Sources */,
+ 8A171F13214905D400121E80 /* CVPixelBuffer+CIImage.swift in Sources */,
+ 8AAF371B21481369006A551B /* UIImage+CVPixelBuffer.swift in Sources */,
+ 8AAF371521480D32006A551B /* SampleDataSource.swift in Sources */,
+ 8AB76029213628440051C3D1 /* MetalRenderer.swift in Sources */,
+ 8A7F8807214819FF00170436 /* TrackingState+Description.swift in Sources */,
+ 8A603D8A2149460E00F848A4 /* PhotosUtils.swift in Sources */,
+ 8A171F0B2148FE4100121E80 /* RealtimeDepthViewController.swift in Sources */,
+ 8AAF371321480D32006A551B /* RootViewCell.swift in Sources */,
+ 8A603D8C2149590F00F848A4 /* UIAlertController+Utils.swift in Sources */,
+ 8AAF371D2148136A006A551B /* CGImageSource+Depth.swift in Sources */,
+ 8A171F15214928BB00121E80 /* AVDepthData+Utils.swift in Sources */,
+ 8A702F98212A3BD3004039F7 /* AppDelegate.swift in Sources */,
+ 8A171F1A214930D100121E80 /* RealtimeDepthMaskViewController.swift in Sources */,
+ 8A603D87214945A900F848A4 /* PortraitMatteViewController.swift in Sources */,
+ 8A702FAF212A3CDE004039F7 /* AVCaptureDevice+Extension.swift in Sources */,
+ 8A702FAD212A3CDE004039F7 /* VideoCameraType.swift in Sources */,
+ );
+ runOnlyForDeploymentPostprocessing = 0;
+ };
+/* End PBXSourcesBuildPhase section */
+
+/* Begin PBXVariantGroup section */
+ 8A171F072148FE4100121E80 /* RealtimeDepth.storyboard */ = {
+ isa = PBXVariantGroup;
+ children = (
+ 8A171F082148FE4100121E80 /* Base */,
+ );
+ name = RealtimeDepth.storyboard;
+ sourceTree = "";
+ };
+ 8A171F18214930D100121E80 /* RealtimeDepthMask.storyboard */ = {
+ isa = PBXVariantGroup;
+ children = (
+ 8A171F19214930D100121E80 /* Base */,
+ );
+ name = RealtimeDepthMask.storyboard;
+ sourceTree = "";
+ };
+ 8A702FA0212A3BD5004039F7 /* LaunchScreen.storyboard */ = {
+ isa = PBXVariantGroup;
+ children = (
+ 8A702FA1212A3BD5004039F7 /* Base */,
+ );
+ name = LaunchScreen.storyboard;
+ sourceTree = "";
+ };
+/* End PBXVariantGroup section */
+
+/* Begin XCBuildConfiguration section */
+ 8A702FA4212A3BD5004039F7 /* Debug */ = {
+ isa = XCBuildConfiguration;
+ buildSettings = {
+ ALWAYS_SEARCH_USER_PATHS = NO;
+ CLANG_ANALYZER_NONNULL = YES;
+ CLANG_ANALYZER_NUMBER_OBJECT_CONVERSION = YES_AGGRESSIVE;
+ CLANG_CXX_LANGUAGE_STANDARD = "gnu++14";
+ CLANG_CXX_LIBRARY = "libc++";
+ CLANG_ENABLE_MODULES = YES;
+ CLANG_ENABLE_OBJC_ARC = YES;
+ CLANG_ENABLE_OBJC_WEAK = YES;
+ CLANG_WARN_BLOCK_CAPTURE_AUTORELEASING = YES;
+ CLANG_WARN_BOOL_CONVERSION = YES;
+ CLANG_WARN_COMMA = YES;
+ CLANG_WARN_CONSTANT_CONVERSION = YES;
+ CLANG_WARN_DEPRECATED_OBJC_IMPLEMENTATIONS = YES;
+ CLANG_WARN_DIRECT_OBJC_ISA_USAGE = YES_ERROR;
+ CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
+ CLANG_WARN_EMPTY_BODY = YES;
+ CLANG_WARN_ENUM_CONVERSION = YES;
+ CLANG_WARN_INFINITE_RECURSION = YES;
+ CLANG_WARN_INT_CONVERSION = YES;
+ CLANG_WARN_NON_LITERAL_NULL_CONVERSION = YES;
+ CLANG_WARN_OBJC_IMPLICIT_RETAIN_SELF = YES;
+ CLANG_WARN_OBJC_LITERAL_CONVERSION = YES;
+ CLANG_WARN_OBJC_ROOT_CLASS = YES_ERROR;
+ CLANG_WARN_RANGE_LOOP_ANALYSIS = YES;
+ CLANG_WARN_STRICT_PROTOTYPES = YES;
+ CLANG_WARN_SUSPICIOUS_MOVE = YES;
+ CLANG_WARN_UNGUARDED_AVAILABILITY = YES_AGGRESSIVE;
+ CLANG_WARN_UNREACHABLE_CODE = YES;
+ CLANG_WARN__DUPLICATE_METHOD_MATCH = YES;
+ CODE_SIGN_IDENTITY = "iPhone Developer";
+ COPY_PHASE_STRIP = NO;
+ DEBUG_INFORMATION_FORMAT = dwarf;
+ ENABLE_STRICT_OBJC_MSGSEND = YES;
+ ENABLE_TESTABILITY = YES;
+ GCC_C_LANGUAGE_STANDARD = gnu11;
+ GCC_DYNAMIC_NO_PIC = NO;
+ GCC_NO_COMMON_BLOCKS = YES;
+ GCC_OPTIMIZATION_LEVEL = 0;
+ GCC_PREPROCESSOR_DEFINITIONS = (
+ "DEBUG=1",
+ "$(inherited)",
+ );
+ GCC_WARN_64_TO_32_BIT_CONVERSION = YES;
+ GCC_WARN_ABOUT_RETURN_TYPE = YES_ERROR;
+ GCC_WARN_UNDECLARED_SELECTOR = YES;
+ GCC_WARN_UNINITIALIZED_AUTOS = YES_AGGRESSIVE;
+ GCC_WARN_UNUSED_FUNCTION = YES;
+ GCC_WARN_UNUSED_VARIABLE = YES;
+ IPHONEOS_DEPLOYMENT_TARGET = 12.0;
+ MTL_ENABLE_DEBUG_INFO = INCLUDE_SOURCE;
+ MTL_FAST_MATH = YES;
+ ONLY_ACTIVE_ARCH = YES;
+ SDKROOT = iphoneos;
+ SWIFT_ACTIVE_COMPILATION_CONDITIONS = DEBUG;
+ SWIFT_OPTIMIZATION_LEVEL = "-Onone";
+ };
+ name = Debug;
+ };
+ 8A702FA5212A3BD5004039F7 /* Release */ = {
+ isa = XCBuildConfiguration;
+ buildSettings = {
+ ALWAYS_SEARCH_USER_PATHS = NO;
+ CLANG_ANALYZER_NONNULL = YES;
+ CLANG_ANALYZER_NUMBER_OBJECT_CONVERSION = YES_AGGRESSIVE;
+ CLANG_CXX_LANGUAGE_STANDARD = "gnu++14";
+ CLANG_CXX_LIBRARY = "libc++";
+ CLANG_ENABLE_MODULES = YES;
+ CLANG_ENABLE_OBJC_ARC = YES;
+ CLANG_ENABLE_OBJC_WEAK = YES;
+ CLANG_WARN_BLOCK_CAPTURE_AUTORELEASING = YES;
+ CLANG_WARN_BOOL_CONVERSION = YES;
+ CLANG_WARN_COMMA = YES;
+ CLANG_WARN_CONSTANT_CONVERSION = YES;
+ CLANG_WARN_DEPRECATED_OBJC_IMPLEMENTATIONS = YES;
+ CLANG_WARN_DIRECT_OBJC_ISA_USAGE = YES_ERROR;
+ CLANG_WARN_DOCUMENTATION_COMMENTS = YES;
+ CLANG_WARN_EMPTY_BODY = YES;
+ CLANG_WARN_ENUM_CONVERSION = YES;
+ CLANG_WARN_INFINITE_RECURSION = YES;
+ CLANG_WARN_INT_CONVERSION = YES;
+ CLANG_WARN_NON_LITERAL_NULL_CONVERSION = YES;
+ CLANG_WARN_OBJC_IMPLICIT_RETAIN_SELF = YES;
+ CLANG_WARN_OBJC_LITERAL_CONVERSION = YES;
+ CLANG_WARN_OBJC_ROOT_CLASS = YES_ERROR;
+ CLANG_WARN_RANGE_LOOP_ANALYSIS = YES;
+ CLANG_WARN_STRICT_PROTOTYPES = YES;
+ CLANG_WARN_SUSPICIOUS_MOVE = YES;
+ CLANG_WARN_UNGUARDED_AVAILABILITY = YES_AGGRESSIVE;
+ CLANG_WARN_UNREACHABLE_CODE = YES;
+ CLANG_WARN__DUPLICATE_METHOD_MATCH = YES;
+ CODE_SIGN_IDENTITY = "iPhone Developer";
+ COPY_PHASE_STRIP = NO;
+ DEBUG_INFORMATION_FORMAT = "dwarf-with-dsym";
+ ENABLE_NS_ASSERTIONS = NO;
+ ENABLE_STRICT_OBJC_MSGSEND = YES;
+ GCC_C_LANGUAGE_STANDARD = gnu11;
+ GCC_NO_COMMON_BLOCKS = YES;
+ GCC_WARN_64_TO_32_BIT_CONVERSION = YES;
+ GCC_WARN_ABOUT_RETURN_TYPE = YES_ERROR;
+ GCC_WARN_UNDECLARED_SELECTOR = YES;
+ GCC_WARN_UNINITIALIZED_AUTOS = YES_AGGRESSIVE;
+ GCC_WARN_UNUSED_FUNCTION = YES;
+ GCC_WARN_UNUSED_VARIABLE = YES;
+ IPHONEOS_DEPLOYMENT_TARGET = 12.0;
+ MTL_ENABLE_DEBUG_INFO = NO;
+ MTL_FAST_MATH = YES;
+ SDKROOT = iphoneos;
+ SWIFT_COMPILATION_MODE = wholemodule;
+ SWIFT_OPTIMIZATION_LEVEL = "-O";
+ VALIDATE_PRODUCT = YES;
+ };
+ name = Release;
+ };
+ 8A702FA7212A3BD5004039F7 /* Debug */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = DDC3399A5A9E8877FB64BCD8 /* Pods-iOS-Depth-Sampler.debug.xcconfig */;
+ buildSettings = {
+ ASSETCATALOG_COMPILER_APPICON_NAME = AppIcon;
+ CLANG_ENABLE_MODULES = YES;
+ CODE_SIGN_STYLE = Automatic;
+ DEVELOPMENT_TEAM = 9Z86A4AWDE;
+ INFOPLIST_FILE = "iOS-Depth-Sampler/Info.plist";
+ IPHONEOS_DEPLOYMENT_TARGET = 12.0;
+ LD_RUNPATH_SEARCH_PATHS = (
+ "$(inherited)",
+ "@executable_path/Frameworks",
+ );
+ PRODUCT_BUNDLE_IDENTIFIER = "com.shu223.iOS-Depth-Sampler";
+ PRODUCT_NAME = "$(TARGET_NAME)";
+ SWIFT_OBJC_BRIDGING_HEADER = "iOS-Depth-Sampler/iOS-Depth-Sampler-Bridging-Header.h";
+ SWIFT_OPTIMIZATION_LEVEL = "-Onone";
+ SWIFT_VERSION = 4.2;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ };
+ name = Debug;
+ };
+ 8A702FA8212A3BD5004039F7 /* Release */ = {
+ isa = XCBuildConfiguration;
+ baseConfigurationReference = 8FA83CA5E0F8F1F5F2E5DF63 /* Pods-iOS-Depth-Sampler.release.xcconfig */;
+ buildSettings = {
+ ASSETCATALOG_COMPILER_APPICON_NAME = AppIcon;
+ CLANG_ENABLE_MODULES = YES;
+ CODE_SIGN_STYLE = Automatic;
+ DEVELOPMENT_TEAM = 9Z86A4AWDE;
+ INFOPLIST_FILE = "iOS-Depth-Sampler/Info.plist";
+ IPHONEOS_DEPLOYMENT_TARGET = 12.0;
+ LD_RUNPATH_SEARCH_PATHS = (
+ "$(inherited)",
+ "@executable_path/Frameworks",
+ );
+ PRODUCT_BUNDLE_IDENTIFIER = "com.shu223.iOS-Depth-Sampler";
+ PRODUCT_NAME = "$(TARGET_NAME)";
+ SWIFT_OBJC_BRIDGING_HEADER = "iOS-Depth-Sampler/iOS-Depth-Sampler-Bridging-Header.h";
+ SWIFT_VERSION = 4.2;
+ TARGETED_DEVICE_FAMILY = "1,2";
+ };
+ name = Release;
+ };
+/* End XCBuildConfiguration section */
+
+/* Begin XCConfigurationList section */
+ 8A702F8F212A3BD3004039F7 /* Build configuration list for PBXProject "iOS-Depth-Sampler" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ 8A702FA4212A3BD5004039F7 /* Debug */,
+ 8A702FA5212A3BD5004039F7 /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+ 8A702FA6212A3BD5004039F7 /* Build configuration list for PBXNativeTarget "iOS-Depth-Sampler" */ = {
+ isa = XCConfigurationList;
+ buildConfigurations = (
+ 8A702FA7212A3BD5004039F7 /* Debug */,
+ 8A702FA8212A3BD5004039F7 /* Release */,
+ );
+ defaultConfigurationIsVisible = 0;
+ defaultConfigurationName = Release;
+ };
+/* End XCConfigurationList section */
+ };
+ rootObject = 8A702F8C212A3BD3004039F7 /* Project object */;
+}
diff --git a/iOS-Depth-Sampler.xcodeproj/project.xcworkspace/contents.xcworkspacedata b/iOS-Depth-Sampler.xcodeproj/project.xcworkspace/contents.xcworkspacedata
new file mode 100644
index 0000000..91ad967
--- /dev/null
+++ b/iOS-Depth-Sampler.xcodeproj/project.xcworkspace/contents.xcworkspacedata
@@ -0,0 +1,7 @@
+
+
+
+
+
diff --git a/iOS-Depth-Sampler.xcodeproj/project.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist b/iOS-Depth-Sampler.xcodeproj/project.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
new file mode 100644
index 0000000..18d9810
--- /dev/null
+++ b/iOS-Depth-Sampler.xcodeproj/project.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
@@ -0,0 +1,8 @@
+
+
+
+
+ IDEDidComputeMac32BitWarning
+
+
+
diff --git a/iOS-Depth-Sampler.xcworkspace/contents.xcworkspacedata b/iOS-Depth-Sampler.xcworkspace/contents.xcworkspacedata
new file mode 100644
index 0000000..d4164e0
--- /dev/null
+++ b/iOS-Depth-Sampler.xcworkspace/contents.xcworkspacedata
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist b/iOS-Depth-Sampler.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
new file mode 100644
index 0000000..18d9810
--- /dev/null
+++ b/iOS-Depth-Sampler.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
@@ -0,0 +1,8 @@
+
+
+
+
+ IDEDidComputeMac32BitWarning
+
+
+
diff --git a/iOS-Depth-Sampler/AppDelegate.swift b/iOS-Depth-Sampler/AppDelegate.swift
new file mode 100644
index 0000000..615efaa
--- /dev/null
+++ b/iOS-Depth-Sampler/AppDelegate.swift
@@ -0,0 +1,21 @@
+//
+// AppDelegate.swift
+// AvailableCameraDevices
+//
+// Created by Shuichi Tsutsumi on 2018/08/20.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+
+@UIApplicationMain
+class AppDelegate: UIResponder, UIApplicationDelegate {
+
+ var window: UIWindow?
+
+ func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
+ return true
+ }
+
+}
+
diff --git a/iOS-Depth-Sampler/Assets.xcassets/AppIcon.appiconset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/AppIcon.appiconset/Contents.json
new file mode 100644
index 0000000..d8db8d6
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/AppIcon.appiconset/Contents.json
@@ -0,0 +1,98 @@
+{
+ "images" : [
+ {
+ "idiom" : "iphone",
+ "size" : "20x20",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "20x20",
+ "scale" : "3x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "29x29",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "29x29",
+ "scale" : "3x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "40x40",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "40x40",
+ "scale" : "3x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "60x60",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "iphone",
+ "size" : "60x60",
+ "scale" : "3x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "20x20",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "20x20",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "29x29",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "29x29",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "40x40",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "40x40",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "76x76",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "76x76",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "ipad",
+ "size" : "83.5x83.5",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "ios-marketing",
+ "size" : "1024x1024",
+ "scale" : "1x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/BigBang.imageset/BigBang-184895179-web-2.jpg b/iOS-Depth-Sampler/Assets.xcassets/BigBang.imageset/BigBang-184895179-web-2.jpg
new file mode 100644
index 0000000..6b8d270
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/BigBang.imageset/BigBang-184895179-web-2.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/BigBang.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/BigBang.imageset/Contents.json
new file mode 100644
index 0000000..1a04e82
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/BigBang.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "BigBang-184895179-web-2.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/Contents.json
new file mode 100644
index 0000000..da4a164
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/Contents.json
@@ -0,0 +1,6 @@
+{
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/Contents.json
new file mode 100644
index 0000000..da4a164
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/Contents.json
@@ -0,0 +1,6 @@
+{
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000001.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000001.imageset/Contents.json
new file mode 100644
index 0000000..c866159
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000001.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000001.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000001.imageset/burn000001.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000001.imageset/burn000001.jpg
new file mode 100644
index 0000000..2f3264a
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000001.imageset/burn000001.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000002.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000002.imageset/Contents.json
new file mode 100644
index 0000000..8f2c4b7
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000002.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000002.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000002.imageset/burn000002.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000002.imageset/burn000002.jpg
new file mode 100644
index 0000000..f19ce33
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000002.imageset/burn000002.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000003.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000003.imageset/Contents.json
new file mode 100644
index 0000000..166dc2d
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000003.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000003.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000003.imageset/burn000003.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000003.imageset/burn000003.jpg
new file mode 100644
index 0000000..0b001bd
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000003.imageset/burn000003.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000004.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000004.imageset/Contents.json
new file mode 100644
index 0000000..212fb38
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000004.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000004.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000004.imageset/burn000004.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000004.imageset/burn000004.jpg
new file mode 100644
index 0000000..2578e90
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000004.imageset/burn000004.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000005.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000005.imageset/Contents.json
new file mode 100644
index 0000000..23fa156
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000005.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000005.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000005.imageset/burn000005.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000005.imageset/burn000005.jpg
new file mode 100644
index 0000000..4faefda
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000005.imageset/burn000005.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000006.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000006.imageset/Contents.json
new file mode 100644
index 0000000..a5f404c
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000006.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000006.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000006.imageset/burn000006.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000006.imageset/burn000006.jpg
new file mode 100644
index 0000000..b413a55
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000006.imageset/burn000006.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000007.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000007.imageset/Contents.json
new file mode 100644
index 0000000..f9a0fe0
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000007.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000007.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000007.imageset/burn000007.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000007.imageset/burn000007.jpg
new file mode 100644
index 0000000..6a1482f
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000007.imageset/burn000007.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000008.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000008.imageset/Contents.json
new file mode 100644
index 0000000..176e525
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000008.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000008.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000008.imageset/burn000008.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000008.imageset/burn000008.jpg
new file mode 100644
index 0000000..74de81b
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000008.imageset/burn000008.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000009.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000009.imageset/Contents.json
new file mode 100644
index 0000000..9f94da0
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000009.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000009.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000009.imageset/burn000009.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000009.imageset/burn000009.jpg
new file mode 100644
index 0000000..5d144c0
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000009.imageset/burn000009.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000010.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000010.imageset/Contents.json
new file mode 100644
index 0000000..55a8769
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000010.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000010.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000010.imageset/burn000010.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000010.imageset/burn000010.jpg
new file mode 100644
index 0000000..c25e69b
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000010.imageset/burn000010.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000011.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000011.imageset/Contents.json
new file mode 100644
index 0000000..921c01b
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000011.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000011.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000011.imageset/burn000011.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000011.imageset/burn000011.jpg
new file mode 100644
index 0000000..062abe7
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000011.imageset/burn000011.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000012.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000012.imageset/Contents.json
new file mode 100644
index 0000000..4d6ba51
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000012.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000012.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000012.imageset/burn000012.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000012.imageset/burn000012.jpg
new file mode 100644
index 0000000..f80c4b9
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000012.imageset/burn000012.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000013.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000013.imageset/Contents.json
new file mode 100644
index 0000000..14a6026
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000013.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000013.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000013.imageset/burn000013.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000013.imageset/burn000013.jpg
new file mode 100644
index 0000000..7201fd2
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000013.imageset/burn000013.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000014.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000014.imageset/Contents.json
new file mode 100644
index 0000000..a261392
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000014.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000014.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000014.imageset/burn000014.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000014.imageset/burn000014.jpg
new file mode 100644
index 0000000..e1e43a2
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000014.imageset/burn000014.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000015.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000015.imageset/Contents.json
new file mode 100644
index 0000000..44d64d2
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000015.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000015.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000015.imageset/burn000015.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000015.imageset/burn000015.jpg
new file mode 100644
index 0000000..38371e0
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000015.imageset/burn000015.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000016.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000016.imageset/Contents.json
new file mode 100644
index 0000000..d9c067b
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000016.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000016.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000016.imageset/burn000016.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000016.imageset/burn000016.jpg
new file mode 100644
index 0000000..e7c327c
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000016.imageset/burn000016.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000017.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000017.imageset/Contents.json
new file mode 100644
index 0000000..3540bff
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000017.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000017.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000017.imageset/burn000017.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000017.imageset/burn000017.jpg
new file mode 100644
index 0000000..b114514
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000017.imageset/burn000017.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000018.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000018.imageset/Contents.json
new file mode 100644
index 0000000..88ee8fd
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000018.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000018.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000018.imageset/burn000018.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000018.imageset/burn000018.jpg
new file mode 100644
index 0000000..94d2dd8
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000018.imageset/burn000018.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000019.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000019.imageset/Contents.json
new file mode 100644
index 0000000..857e701
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000019.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000019.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000019.imageset/burn000019.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000019.imageset/burn000019.jpg
new file mode 100644
index 0000000..a5b7d51
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000019.imageset/burn000019.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000020.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000020.imageset/Contents.json
new file mode 100644
index 0000000..e8765db
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000020.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000020.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000020.imageset/burn000020.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000020.imageset/burn000020.jpg
new file mode 100644
index 0000000..c57d6a5
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000020.imageset/burn000020.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000021.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000021.imageset/Contents.json
new file mode 100644
index 0000000..359645c
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000021.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000021.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000021.imageset/burn000021.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000021.imageset/burn000021.jpg
new file mode 100644
index 0000000..ee9f84f
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000021.imageset/burn000021.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000022.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000022.imageset/Contents.json
new file mode 100644
index 0000000..1b895d6
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000022.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000022.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000022.imageset/burn000022.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000022.imageset/burn000022.jpg
new file mode 100644
index 0000000..f4eb6e1
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000022.imageset/burn000022.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000023.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000023.imageset/Contents.json
new file mode 100644
index 0000000..66e6b1e
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000023.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000023.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000023.imageset/burn000023.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000023.imageset/burn000023.jpg
new file mode 100644
index 0000000..0b3bbc5
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000023.imageset/burn000023.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000024.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000024.imageset/Contents.json
new file mode 100644
index 0000000..6a289f2
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000024.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "burn000024.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/burn/burn000024.imageset/burn000024.jpg b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000024.imageset/burn000024.jpg
new file mode 100644
index 0000000..736fba8
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/burn/burn000024.imageset/burn000024.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/earth.imageset/10207-1.jpg b/iOS-Depth-Sampler/Assets.xcassets/earth.imageset/10207-1.jpg
new file mode 100644
index 0000000..5265d76
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/earth.imageset/10207-1.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/earth.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/earth.imageset/Contents.json
new file mode 100644
index 0000000..2579141
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/earth.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "10207-1.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/something.imageset/7341d720.jpg b/iOS-Depth-Sampler/Assets.xcassets/something.imageset/7341d720.jpg
new file mode 100644
index 0000000..e8c0ba0
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/something.imageset/7341d720.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/something.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/something.imageset/Contents.json
new file mode 100644
index 0000000..1b8b680
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/something.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "7341d720.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000001.imageset/000001.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000001.imageset/000001.jpg
new file mode 100644
index 0000000..a77ce61
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000001.imageset/000001.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000001.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000001.imageset/Contents.json
new file mode 100644
index 0000000..9940157
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000001.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000001.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000002.imageset/000002.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000002.imageset/000002.jpg
new file mode 100644
index 0000000..0caa46c
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000002.imageset/000002.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000002.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000002.imageset/Contents.json
new file mode 100644
index 0000000..9c776b1
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000002.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000002.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000003.imageset/000003.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000003.imageset/000003.jpg
new file mode 100644
index 0000000..c605afc
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000003.imageset/000003.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000003.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000003.imageset/Contents.json
new file mode 100644
index 0000000..26eba97
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000003.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000003.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000004.imageset/000004.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000004.imageset/000004.jpg
new file mode 100644
index 0000000..ddaf3fd
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000004.imageset/000004.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000004.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000004.imageset/Contents.json
new file mode 100644
index 0000000..c6f1b29
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000004.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000004.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000005.imageset/000005.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000005.imageset/000005.jpg
new file mode 100644
index 0000000..ae0299e
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000005.imageset/000005.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000005.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000005.imageset/Contents.json
new file mode 100644
index 0000000..7b687ba
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000005.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000005.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000006.imageset/000006.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000006.imageset/000006.jpg
new file mode 100644
index 0000000..9e30bdc
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000006.imageset/000006.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000006.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000006.imageset/Contents.json
new file mode 100644
index 0000000..f40cfcf
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000006.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000006.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000007.imageset/000007.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000007.imageset/000007.jpg
new file mode 100644
index 0000000..441486c
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000007.imageset/000007.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000007.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000007.imageset/Contents.json
new file mode 100644
index 0000000..800bccb
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000007.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000007.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000008.imageset/000008.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000008.imageset/000008.jpg
new file mode 100644
index 0000000..cb3a65a
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000008.imageset/000008.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000008.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000008.imageset/Contents.json
new file mode 100644
index 0000000..95fa882
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000008.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000008.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000009.imageset/000009.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000009.imageset/000009.jpg
new file mode 100644
index 0000000..2456a64
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000009.imageset/000009.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000009.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000009.imageset/Contents.json
new file mode 100644
index 0000000..a0631c0
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000009.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000009.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000010.imageset/000010.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000010.imageset/000010.jpg
new file mode 100644
index 0000000..af78e39
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000010.imageset/000010.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000010.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000010.imageset/Contents.json
new file mode 100644
index 0000000..a79088f
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000010.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000010.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000011.imageset/000011.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000011.imageset/000011.jpg
new file mode 100644
index 0000000..4309ed2
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000011.imageset/000011.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000011.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000011.imageset/Contents.json
new file mode 100644
index 0000000..21258b5
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000011.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000011.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000012.imageset/000012.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000012.imageset/000012.jpg
new file mode 100644
index 0000000..cc09112
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000012.imageset/000012.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000012.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000012.imageset/Contents.json
new file mode 100644
index 0000000..eb031b1
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000012.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000012.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000013.imageset/000013.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000013.imageset/000013.jpg
new file mode 100644
index 0000000..caaac4e
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000013.imageset/000013.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000013.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000013.imageset/Contents.json
new file mode 100644
index 0000000..e4023ff
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000013.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000013.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000014.imageset/000014.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000014.imageset/000014.jpg
new file mode 100644
index 0000000..e2777d4
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000014.imageset/000014.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000014.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000014.imageset/Contents.json
new file mode 100644
index 0000000..28236aa
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000014.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000014.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000015.imageset/000015.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000015.imageset/000015.jpg
new file mode 100644
index 0000000..ac5cdc8
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000015.imageset/000015.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000015.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000015.imageset/Contents.json
new file mode 100644
index 0000000..154fe89
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000015.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000015.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000016.imageset/000016.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000016.imageset/000016.jpg
new file mode 100644
index 0000000..988b14f
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000016.imageset/000016.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000016.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000016.imageset/Contents.json
new file mode 100644
index 0000000..8e5f6b0
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000016.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000016.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000017.imageset/000017.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000017.imageset/000017.jpg
new file mode 100644
index 0000000..420aa10
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000017.imageset/000017.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000017.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000017.imageset/Contents.json
new file mode 100644
index 0000000..2fa5224
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000017.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000017.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000018.imageset/000018.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000018.imageset/000018.jpg
new file mode 100644
index 0000000..4bacd3f
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000018.imageset/000018.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000018.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000018.imageset/Contents.json
new file mode 100644
index 0000000..e7aba7e
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000018.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000018.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000019.imageset/000019.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000019.imageset/000019.jpg
new file mode 100644
index 0000000..58c82b8
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000019.imageset/000019.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000019.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000019.imageset/Contents.json
new file mode 100644
index 0000000..4574dba
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000019.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000019.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000020.imageset/000020.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000020.imageset/000020.jpg
new file mode 100644
index 0000000..baccc37
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000020.imageset/000020.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000020.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000020.imageset/Contents.json
new file mode 100644
index 0000000..7986970
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000020.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000020.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000021.imageset/000021.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000021.imageset/000021.jpg
new file mode 100644
index 0000000..6636672
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000021.imageset/000021.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000021.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000021.imageset/Contents.json
new file mode 100644
index 0000000..9f303de
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000021.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000021.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000022.imageset/000022.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000022.imageset/000022.jpg
new file mode 100644
index 0000000..4424c69
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000022.imageset/000022.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000022.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000022.imageset/Contents.json
new file mode 100644
index 0000000..5194dd0
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000022.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000022.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000023.imageset/000023.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000023.imageset/000023.jpg
new file mode 100644
index 0000000..78f5ec9
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000023.imageset/000023.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000023.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000023.imageset/Contents.json
new file mode 100644
index 0000000..5654320
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000023.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000023.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000024.imageset/000024.jpg b/iOS-Depth-Sampler/Assets.xcassets/warp/000024.imageset/000024.jpg
new file mode 100644
index 0000000..2923ef1
Binary files /dev/null and b/iOS-Depth-Sampler/Assets.xcassets/warp/000024.imageset/000024.jpg differ
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/000024.imageset/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/000024.imageset/Contents.json
new file mode 100644
index 0000000..96eb3d1
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/000024.imageset/Contents.json
@@ -0,0 +1,21 @@
+{
+ "images" : [
+ {
+ "idiom" : "universal",
+ "filename" : "000024.jpg",
+ "scale" : "1x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "2x"
+ },
+ {
+ "idiom" : "universal",
+ "scale" : "3x"
+ }
+ ],
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Assets.xcassets/warp/Contents.json b/iOS-Depth-Sampler/Assets.xcassets/warp/Contents.json
new file mode 100644
index 0000000..da4a164
--- /dev/null
+++ b/iOS-Depth-Sampler/Assets.xcassets/warp/Contents.json
@@ -0,0 +1,6 @@
+{
+ "info" : {
+ "version" : 1,
+ "author" : "xcode"
+ }
+}
\ No newline at end of file
diff --git a/iOS-Depth-Sampler/Base.lproj/LaunchScreen.storyboard b/iOS-Depth-Sampler/Base.lproj/LaunchScreen.storyboard
new file mode 100644
index 0000000..bfa3612
--- /dev/null
+++ b/iOS-Depth-Sampler/Base.lproj/LaunchScreen.storyboard
@@ -0,0 +1,25 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Info.plist b/iOS-Depth-Sampler/Info.plist
new file mode 100644
index 0000000..10e709f
--- /dev/null
+++ b/iOS-Depth-Sampler/Info.plist
@@ -0,0 +1,47 @@
+
+
+
+
+ CFBundleDevelopmentRegion
+ $(DEVELOPMENT_LANGUAGE)
+ CFBundleExecutable
+ $(EXECUTABLE_NAME)
+ CFBundleIdentifier
+ $(PRODUCT_BUNDLE_IDENTIFIER)
+ CFBundleInfoDictionaryVersion
+ 6.0
+ CFBundleName
+ $(PRODUCT_NAME)
+ CFBundlePackageType
+ APPL
+ CFBundleShortVersionString
+ 1.0
+ CFBundleVersion
+ 1
+ LSRequiresIPhoneOS
+
+ NSCameraUsageDescription
+ Needs to use camera
+ NSPhotoLibraryUsageDescription
+ Needs to use pictures in the Photo Library
+ UILaunchStoryboardName
+ LaunchScreen
+ UIMainStoryboardFile
+ Main
+ UIRequiredDeviceCapabilities
+
+ armv7
+
+ UISupportedInterfaceOrientations
+
+ UIInterfaceOrientationPortrait
+
+ UISupportedInterfaceOrientations~ipad
+
+ UIInterfaceOrientationPortrait
+ UIInterfaceOrientationPortraitUpsideDown
+ UIInterfaceOrientationLandscapeLeft
+ UIInterfaceOrientationLandscapeRight
+
+
+
diff --git a/iOS-Depth-Sampler/Main.storyboard b/iOS-Depth-Sampler/Main.storyboard
new file mode 100644
index 0000000..f29180f
--- /dev/null
+++ b/iOS-Depth-Sampler/Main.storyboard
@@ -0,0 +1,85 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Renderer/MetalRenderer.swift b/iOS-Depth-Sampler/Renderer/MetalRenderer.swift
new file mode 100644
index 0000000..453c877
--- /dev/null
+++ b/iOS-Depth-Sampler/Renderer/MetalRenderer.swift
@@ -0,0 +1,142 @@
+//
+// MetalRenderer.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/29.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import Metal
+import MetalKit
+
+// The max number of command buffers in flight
+let kMaxBuffersInFlight: Int = 1
+
+// Vertex data for an image plane
+let kImagePlaneVertexData: [Float] = [
+ -1.0, -1.0, 0.0, 1.0,
+ 1.0, -1.0, 1.0, 1.0,
+ -1.0, 1.0, 0.0, 0.0,
+ 1.0, 1.0, 1.0, 0.0,
+]
+
+class MetalRenderer {
+ private let device: MTLDevice
+ private let inFlightSemaphore = DispatchSemaphore(value: kMaxBuffersInFlight)
+ private var renderDestination: MTKView
+
+ private var commandQueue: MTLCommandQueue!
+ private var vertexBuffer: MTLBuffer!
+ private var passThroughPipeline: MTLRenderPipelineState!
+
+ init(metalDevice device: MTLDevice, renderDestination: MTKView) {
+ self.device = device
+ self.renderDestination = renderDestination
+
+ // Set the default formats needed to render
+ self.renderDestination.colorPixelFormat = .bgra8Unorm
+ self.renderDestination.sampleCount = 1
+
+ commandQueue = device.makeCommandQueue()
+
+ prepareRenderPipelines(library: device.makeDefaultLibrary()!)
+
+ // prepare vertex buffer(s)
+ let imagePlaneVertexDataCount = kImagePlaneVertexData.count * MemoryLayout.size
+ vertexBuffer = device.makeBuffer(bytes: kImagePlaneVertexData, length: imagePlaneVertexDataCount, options: [])
+ vertexBuffer.label = "vertexBuffer"
+ }
+
+ private let ciContext = CIContext()
+ private let colorSpace = CGColorSpaceCreateDeviceRGB()
+
+ func update(with ciImage: CIImage) {
+ // Wait to ensure only kMaxBuffersInFlight are getting proccessed by any stage in the Metal
+ // pipeline (App, Metal, Drivers, GPU, etc)
+ let _ = inFlightSemaphore.wait(timeout: .distantFuture)
+
+ guard
+ let commandBuffer = commandQueue.makeCommandBuffer(),
+ let currentDrawable = renderDestination.currentDrawable
+ else {
+ inFlightSemaphore.signal()
+ return
+ }
+ commandBuffer.label = "MyCommand"
+
+ commandBuffer.addCompletedHandler{ [weak self] commandBuffer in
+ if let strongSelf = self {
+ strongSelf.inFlightSemaphore.signal()
+ }
+ }
+ ciContext.render(ciImage, to: currentDrawable.texture, commandBuffer: commandBuffer, bounds: ciImage.extent, colorSpace: colorSpace)
+
+ commandBuffer.present(currentDrawable)
+ commandBuffer.commit()
+ }
+
+ // MARK: - Private
+
+ // Create render pipeline states
+ private func prepareRenderPipelines(library: MTLLibrary) {
+ let passThroughVertexFunction = library.passThroughVertexFunction
+ let passThroughFragmentFunction = library.passThroughFragmentFunction
+
+ // Create a vertex descriptor for our image plane vertex buffer
+ let imagePlaneVertexDescriptor = MTLVertexDescriptor()
+
+ // Positions.
+ imagePlaneVertexDescriptor.attributes[0].format = .float2
+ imagePlaneVertexDescriptor.attributes[0].offset = 0
+ imagePlaneVertexDescriptor.attributes[0].bufferIndex = 0
+
+ // Texture coordinates.
+ imagePlaneVertexDescriptor.attributes[1].format = .float2
+ imagePlaneVertexDescriptor.attributes[1].offset = 8
+ imagePlaneVertexDescriptor.attributes[1].bufferIndex = 0
+
+ // Buffer Layout
+ imagePlaneVertexDescriptor.layouts[0].stride = 16
+ imagePlaneVertexDescriptor.layouts[0].stepRate = 1
+ imagePlaneVertexDescriptor.layouts[0].stepFunction = .perVertex
+
+ let createPipeline = { (fragmentFunction: MTLFunction, sampleCount: Int?, pixelFormat: MTLPixelFormat) -> MTLRenderPipelineState in
+ let pipelineDescriptor = MTLRenderPipelineDescriptor()
+ if let sampleCount = sampleCount {
+ pipelineDescriptor.sampleCount = sampleCount
+ }
+ pipelineDescriptor.vertexFunction = passThroughVertexFunction
+ pipelineDescriptor.fragmentFunction = fragmentFunction
+ pipelineDescriptor.vertexDescriptor = imagePlaneVertexDescriptor
+ pipelineDescriptor.colorAttachments[0].pixelFormat = pixelFormat
+// pipelineDescriptor.depthAttachmentPixelFormat = MTLPixelFormat.depth32Float
+ return try! self.device.makeRenderPipelineState(descriptor: pipelineDescriptor)
+ }
+
+ passThroughPipeline = createPipeline(passThroughFragmentFunction, renderDestination.sampleCount, renderDestination.colorPixelFormat)
+ }
+}
+
+extension MTLLibrary {
+
+ var passThroughVertexFunction: MTLFunction {
+ return makeFunction(name: "passThroughVertex")!
+ }
+
+ var passThroughFragmentFunction: MTLFunction {
+ return makeFunction(name: "passThroughFragment")!
+ }
+}
+
+extension MTLRenderCommandEncoder {
+
+ func encode(renderPipeline: MTLRenderPipelineState, vertexBuffer: MTLBuffer, textures: [MTLTexture]) {
+ setRenderPipelineState(renderPipeline)
+ setVertexBuffer(vertexBuffer, offset: 0, index: 0)
+ var index: Int = 0
+ textures.forEach { (texture) in
+ setFragmentTexture(texture, index: index)
+ index += 1
+ }
+ drawPrimitives(type: .triangleStrip, vertexStart: 0, vertexCount: 4)
+ }
+}
diff --git a/iOS-Depth-Sampler/Renderer/PassThrough.metal b/iOS-Depth-Sampler/Renderer/PassThrough.metal
new file mode 100644
index 0000000..0249b53
--- /dev/null
+++ b/iOS-Depth-Sampler/Renderer/PassThrough.metal
@@ -0,0 +1,36 @@
+//
+// PassThrough.metal
+//
+// Created by Shuichi Tsutsumi on 2018/08/29.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+#include
+using namespace metal;
+
+typedef struct {
+ float2 position [[ attribute(0) ]];
+ float2 texCoord [[ attribute(1) ]];
+} Vertex;
+
+typedef struct {
+ float4 position [[ position ]];
+ float2 texCoord;
+} ColorInOut;
+
+vertex ColorInOut passThroughVertex(Vertex in [[ stage_in ]])
+{
+ ColorInOut out;
+ out.position = float4(in.position, 0.0, 1.0);
+ out.texCoord = in.texCoord;
+ return out;
+}
+
+fragment float4 passThroughFragment(ColorInOut in [[ stage_in ]],
+ texture2d texture [[ texture(0) ]])
+{
+ constexpr sampler colorSampler;
+ float4 color = texture.sample(colorSampler, in.texCoord);
+ return color;
+}
+
diff --git a/iOS-Depth-Sampler/RootViewCell.swift b/iOS-Depth-Sampler/RootViewCell.swift
new file mode 100644
index 0000000..a47806d
--- /dev/null
+++ b/iOS-Depth-Sampler/RootViewCell.swift
@@ -0,0 +1,28 @@
+//
+// RootViewCell.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/11.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+
+class RootViewCell: UITableViewCell {
+
+ @IBOutlet private weak var titleLabel: UILabel!
+ @IBOutlet private weak var detailLabel: UILabel!
+
+ override func awakeFromNib() {
+ super.awakeFromNib()
+ }
+
+ override func setSelected(_ selected: Bool, animated: Bool) {
+ super.setSelected(selected, animated: animated)
+ }
+
+ func showSample(_ sample: Sample) {
+ titleLabel.text = sample.title
+ detailLabel.text = sample.detail
+ }
+}
diff --git a/iOS-Depth-Sampler/RootViewController.swift b/iOS-Depth-Sampler/RootViewController.swift
new file mode 100644
index 0000000..7ed5bb8
--- /dev/null
+++ b/iOS-Depth-Sampler/RootViewController.swift
@@ -0,0 +1,45 @@
+//
+// RootViewController.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/11.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+
+class RootViewController: UITableViewController {
+
+ private let dataSource = SampleDataSource()
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+ }
+
+ override func didReceiveMemoryWarning() {
+ super.didReceiveMemoryWarning()
+ }
+
+ // MARK: UITableViewDataSource
+
+ override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
+ return dataSource.samples.count
+ }
+
+ override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
+ guard let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as? RootViewCell else {fatalError()}
+
+ let sample = dataSource.samples[(indexPath as NSIndexPath).row]
+ cell.showSample(sample)
+
+ return cell
+ }
+
+ override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
+ let sample = dataSource.samples[(indexPath as NSIndexPath).row]
+
+ navigationController?.pushViewController(sample.controller(), animated: true)
+
+ tableView.deselectRow(at: indexPath, animated: true)
+ }
+}
diff --git a/iOS-Depth-Sampler/SampleDataSource.swift b/iOS-Depth-Sampler/SampleDataSource.swift
new file mode 100644
index 0000000..bead8da
--- /dev/null
+++ b/iOS-Depth-Sampler/SampleDataSource.swift
@@ -0,0 +1,52 @@
+//
+// SampleDataSource.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/11.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+
+struct Sample {
+ let title: String
+ let detail: String
+ let classPrefix: String
+
+ func controller() -> UIViewController {
+ let storyboard = UIStoryboard(name: classPrefix, bundle: nil)
+ guard let controller = storyboard.instantiateInitialViewController() else {fatalError()}
+ controller.title = title
+ return controller
+ }
+}
+
+struct SampleDataSource {
+ let samples = [
+ Sample(
+ title: "Real-time Depth",
+ detail: "Depth visualization in real time using AV Foundation",
+ classPrefix: "RealtimeDepth"
+ ),
+ Sample(
+ title: "Real-time Dpeth Mask",
+ detail: "Blending a background image with a mask created from depth",
+ classPrefix: "RealtimeDepthMask"
+ ),
+ Sample(
+ title: "Depth from Camera Roll",
+ detail: "Depth visualization from pictures in the camera roll",
+ classPrefix: "DepthFromCameraRoll"
+ ),
+ Sample(
+ title: "Portrait Matte",
+ detail: "Depth visualization from pictures in the camera roll",
+ classPrefix: "PortraitMatte"
+ ),
+ Sample(
+ title: "ARKit Depth",
+ detail: "Depth on ARKit",
+ classPrefix: "ARKitDepth"
+ ),
+ ]
+}
diff --git a/iOS-Depth-Sampler/Samples/ARKit/ARKitDepth.storyboard b/iOS-Depth-Sampler/Samples/ARKit/ARKitDepth.storyboard
new file mode 100644
index 0000000..5c0fedd
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/ARKit/ARKitDepth.storyboard
@@ -0,0 +1,64 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Samples/ARKit/ARKitDepthViewController.swift b/iOS-Depth-Sampler/Samples/ARKit/ARKitDepthViewController.swift
new file mode 100644
index 0000000..fb0256f
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/ARKit/ARKitDepthViewController.swift
@@ -0,0 +1,106 @@
+//
+// ARKitDepthViewController.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/08.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+import ARKit
+import MetalKit
+
+class ARKitDepthViewController: UIViewController {
+
+ @IBOutlet weak var mtkView: MTKView!
+ @IBOutlet weak var sceneView: ARSCNView!
+ @IBOutlet weak var trackingStateLabel: UILabel!
+
+ private var faceGeometry: ARSCNFaceGeometry!
+ private let faceNode = SCNNode()
+
+ private var renderer: MetalRenderer!
+ private var depthImage: CIImage?
+ private var currentDrawableSize: CGSize!
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+
+ guard ARFaceTrackingConfiguration.isSupported else { fatalError() }
+
+ sceneView.delegate = self
+ sceneView.automaticallyUpdatesLighting = true
+ sceneView.scene = SCNScene()
+
+ guard let device = sceneView.device else { fatalError("This device doesn't support Metal.") }
+ mtkView.device = device
+ mtkView.backgroundColor = UIColor.clear
+ mtkView.delegate = self
+ renderer = MetalRenderer(metalDevice: device, renderDestination: mtkView)
+ currentDrawableSize = mtkView.currentDrawable!.layer.drawableSize
+
+ faceGeometry = ARSCNFaceGeometry(device: device, fillMesh: true)
+ if let material = faceGeometry.firstMaterial {
+ material.diffuse.contents = UIColor.green
+ material.lightingModel = .physicallyBased
+ }
+ faceNode.geometry = faceGeometry
+
+ let configuration = ARFaceTrackingConfiguration()
+ configuration.isLightEstimationEnabled = true
+ sceneView.session.run(configuration)
+ }
+}
+
+extension ARKitDepthViewController: ARSCNViewDelegate {
+ func renderer(_ renderer: SCNSceneRenderer, updateAtTime time: TimeInterval) {
+ DispatchQueue.global(qos: .default).async {
+ guard let frame = self.sceneView.session.currentFrame else { return }
+ if let depthImage = frame.transformedDepthImage(targetSize: self.currentDrawableSize) {
+ self.depthImage = depthImage
+ }
+ }
+ }
+
+ func session(_ session: ARSession, cameraDidChangeTrackingState camera: ARCamera) {
+ print("trackingState: \(camera.trackingState)")
+ trackingStateLabel.text = camera.trackingState.description
+ }
+
+ func renderer(_ renderer: SCNSceneRenderer, didAdd node: SCNNode, for anchor: ARAnchor) {
+ print("anchor:\(anchor), node: \(node), node geometry: \(String(describing: node.geometry))")
+ guard let faceAnchor = anchor as? ARFaceAnchor else { return }
+
+ faceGeometry.update(from: faceAnchor.geometry)
+
+ node.addChildNode(faceNode)
+ }
+
+ func renderer(_ renderer: SCNSceneRenderer, didUpdate node: SCNNode, for anchor: ARAnchor) {
+ guard let faceAnchor = anchor as? ARFaceAnchor else { return }
+
+ faceGeometry.update(from: faceAnchor.geometry)
+ }
+
+ func renderer(_ renderer: SCNSceneRenderer, didRemove node: SCNNode, for anchor: ARAnchor) {
+ print("\(self.classForCoder)/" + #function)
+ }
+}
+
+extension ARKitDepthViewController: MTKViewDelegate {
+ func mtkView(_ view: MTKView, drawableSizeWillChange size: CGSize) {
+ currentDrawableSize = size
+ }
+
+ func draw(in view: MTKView) {
+ if let image = depthImage {
+ renderer.update(with: image)
+ }
+ }
+}
+
+extension ARFrame {
+ func transformedDepthImage(targetSize: CGSize) -> CIImage? {
+ guard let depthData = capturedDepthData else { return nil }
+ return depthData.depthDataMap.transformedImage(targetSize: CGSize(width: targetSize.height, height: targetSize.width), rotationAngle: -CGFloat.pi/2)
+ }
+}
diff --git a/iOS-Depth-Sampler/Samples/ARKit/TrackingState+Description.swift b/iOS-Depth-Sampler/Samples/ARKit/TrackingState+Description.swift
new file mode 100644
index 0000000..db94cde
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/ARKit/TrackingState+Description.swift
@@ -0,0 +1,30 @@
+//
+// TrackingState+Description.swift
+//
+// Created by Shuichi Tsutsumi on 2017/08/25.
+// Copyright © 2017 Shuichi Tsutsumi. All rights reserved.
+//
+
+import ARKit
+
+extension ARCamera.TrackingState {
+ public var description: String {
+ switch self {
+ case .notAvailable:
+ return "TRACKING UNAVAILABLE"
+ case .normal:
+ return "TRACKING NORMAL"
+ case .limited(let reason):
+ switch reason {
+ case .excessiveMotion:
+ return "TRACKING LIMITED\nToo much camera movement"
+ case .insufficientFeatures:
+ return "TRACKING LIMITED\nNot enough surface detail"
+ case .initializing:
+ return "Tracking LIMITED\nInitialization in progress."
+ case .relocalizing:
+ return "Tracking LIMITED\nRelocalizing."
+ }
+ }
+ }
+}
diff --git a/iOS-Depth-Sampler/Samples/Depth-from-Camera-Roll/DepthFromCameraRoll.storyboard b/iOS-Depth-Sampler/Samples/Depth-from-Camera-Roll/DepthFromCameraRoll.storyboard
new file mode 100644
index 0000000..2938d63
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Depth-from-Camera-Roll/DepthFromCameraRoll.storyboard
@@ -0,0 +1,67 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Samples/Depth-from-Camera-Roll/DepthFromCameraRollViewController.swift b/iOS-Depth-Sampler/Samples/Depth-from-Camera-Roll/DepthFromCameraRollViewController.swift
new file mode 100644
index 0000000..55f065f
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Depth-from-Camera-Roll/DepthFromCameraRollViewController.swift
@@ -0,0 +1,148 @@
+//
+// DepthFromCameraRollViewController.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/22.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+import Photos
+import SwiftAssetsPickerController
+
+class DepthFromCameraRollViewController: UIViewController {
+
+ @IBOutlet weak var imageView: UIImageView!
+ @IBOutlet weak var typeSegmentedCtl: UISegmentedControl!
+
+ private var asset: PHAsset? {
+ didSet {
+ if let asset = asset {
+ asset.requestColorImage { image in
+ self.image = image
+ self.drawImage(image)
+ }
+ asset.requestContentEditingInput(with: nil) { contentEditingInput, info in
+ self.imageSource = contentEditingInput?.createImageSource()
+ self.getDisparity()
+ self.getDepth()
+ }
+ } else {
+ resetControls()
+ }
+ }
+ }
+ private var image: UIImage?
+ private var imageSource: CGImageSource? {
+ didSet {
+ let isEnabled = imageSource != nil
+ typeSegmentedCtl.isEnabled = isEnabled
+ }
+ }
+ private var disparityPixelBuffer: CVPixelBuffer?
+ private var depthPixelBuffer: CVPixelBuffer?
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+
+ resetControls()
+
+ PHPhotoLibrary.requestAuthorization({ status in
+ switch status {
+ case .authorized:
+ self.openPickerIfNeeded()
+ default:
+ fatalError()
+ }
+ })
+ }
+
+ private func openPickerIfNeeded() {
+ // Are there pictures with depth?
+ let assets = PHAsset.fetchAssetsWithDepth()
+ if assets.isEmpty {
+ let alert = UIAlertController(title: "No pictures with depth",
+ message: "Plaease take a picture with the Camera app using the PORTRAIT mode.",
+ preferredStyle: .alert)
+ let okAction = UIAlertAction(title: "OK", style: .cancel, handler: nil)
+ alert.addAction(okAction)
+ present(alert, animated: true, completion: nil)
+ } else {
+ pickerBtnTapped()
+ }
+ }
+
+ private func resetControls() {
+ typeSegmentedCtl.isEnabled = false
+ }
+
+ private func drawImage(_ image: UIImage?) {
+ DispatchQueue.main.async {
+ self.imageView.image = image
+ }
+ }
+
+ private func draw(pixelBuffer: CVPixelBuffer?) {
+ var image: UIImage? = nil
+ if let pixelBuffer = pixelBuffer {
+ if let depthMapImage = UIImage(pixelBuffer: pixelBuffer) {
+ image = depthMapImage
+ }
+ }
+ drawImage(image)
+ }
+
+ private func getDisparity() {
+ var depthDataMap: CVPixelBuffer? = nil
+ if let disparityData = imageSource?.getDisparityData() {
+ depthDataMap = disparityData.depthDataMap
+ } else if let depthData = imageSource?.getDepthData() {
+ // Depthの場合はDisparityに変換
+ depthDataMap = depthData.convertToDisparity().depthDataMap
+ }
+ disparityPixelBuffer = depthDataMap
+ }
+
+ private func getDepth() {
+ var depthDataMap: CVPixelBuffer? = nil
+ if let depthData = imageSource?.getDepthData() {
+ depthDataMap = depthData.depthDataMap
+ } else if let depthData = imageSource?.getDisparityData() {
+ // Disparityの場合はDepthに変換
+ depthDataMap = depthData.convertToDepth().depthDataMap
+ }
+ depthPixelBuffer = depthDataMap
+ }
+
+ private func update() {
+ switch typeSegmentedCtl.selectedSegmentIndex {
+ case 0:
+ drawImage(image)
+ case 1:
+ draw(pixelBuffer: disparityPixelBuffer)
+ case 2:
+ draw(pixelBuffer: depthPixelBuffer)
+ default:
+ fatalError()
+ }
+ }
+
+ // MARK: - Actions
+
+ @IBAction func typeSegmentChanged(_ sender: UISegmentedControl) {
+ update()
+ }
+
+ @IBAction func pickerBtnTapped() {
+ let rootListAssets = AssetsPickerController()
+ rootListAssets.didSelectAssets = {(assets: Array) -> () in
+ if let asset = assets.first {
+ self.asset = asset
+
+ // カラー画像に戻す
+ self.typeSegmentedCtl.selectedSegmentIndex = 0
+ }
+ }
+ let navigationController = UINavigationController(rootViewController: rootListAssets)
+ present(navigationController, animated: true, completion: nil)
+ }
+}
diff --git a/iOS-Depth-Sampler/Samples/Portrait Matte/PortraitMatte.storyboard b/iOS-Depth-Sampler/Samples/Portrait Matte/PortraitMatte.storyboard
new file mode 100644
index 0000000..67be398
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Portrait Matte/PortraitMatte.storyboard
@@ -0,0 +1,67 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Samples/Portrait Matte/PortraitMatteViewController.swift b/iOS-Depth-Sampler/Samples/Portrait Matte/PortraitMatteViewController.swift
new file mode 100644
index 0000000..ebaaa17
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Portrait Matte/PortraitMatteViewController.swift
@@ -0,0 +1,154 @@
+//
+// DepthFromCameraRollViewController.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/22.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+import Photos
+import SwiftAssetsPickerController
+
+class PortraitMatteViewController: UIViewController {
+
+ @IBOutlet weak var imageView: UIImageView!
+ @IBOutlet weak var typeSegmentedCtl: UISegmentedControl!
+
+ private var asset: PHAsset? {
+ didSet {
+ if let asset = asset {
+ asset.requestColorImage { image in
+ self.image = image
+ self.drawImage(image)
+ }
+ asset.requestContentEditingInput(with: nil) { contentEditingInput, info in
+ self.imageSource = contentEditingInput?.createImageSource()
+ self.getPortraitMatte()
+ }
+ } else {
+ resetControls()
+ }
+ }
+ }
+ private var image: UIImage?
+ private var imageSource: CGImageSource? {
+ didSet {
+ let isEnabled = imageSource != nil
+ typeSegmentedCtl.isEnabled = isEnabled
+ }
+ }
+ private var mattePixelBuffer: CVPixelBuffer?
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+
+ resetControls()
+
+ PHPhotoLibrary.requestAuthorization({ status in
+ switch status {
+ case .authorized:
+ self.openPickerIfNeeded()
+ default:
+ fatalError()
+ }
+ })
+ }
+
+ private func openPickerIfNeeded() {
+ // Are there pictures with depth?
+ let assets = PHAsset.fetchAssetsWithDepth()
+ if assets.isEmpty {
+ UIAlertController.showAlert(title: "No pictures with portrait matte", message: "Plaease take pictures of a HUMAN with 'Camera' app using the PORTRAIT mode.", on: self)
+ } else {
+ pickerBtnTapped()
+ }
+ }
+
+ private func showNoPortraitMatteAlert() {
+ UIAlertController.showAlert(title: "No Portrait Matte", message: "This picture doesn't have portrait matte info. Plaease take a picture of a HUMAN with PORTRAIT mode.", on: self)
+ }
+
+ private func resetControls() {
+ typeSegmentedCtl.isEnabled = false
+ }
+
+ private func drawImage(_ image: UIImage?) {
+ DispatchQueue.main.async {
+ self.imageView.image = image
+ }
+ }
+
+ private func draw(pixelBuffer: CVPixelBuffer?) {
+ var image: UIImage? = nil
+ if let pixelBuffer = pixelBuffer {
+ if let depthMapImage = UIImage(pixelBuffer: pixelBuffer) {
+ image = depthMapImage
+ }
+ }
+ drawImage(image)
+ }
+
+ private func getPortraitMatte() {
+ var depthDataMap: CVPixelBuffer? = nil
+ if let matteData = imageSource?.getMatteData() {
+ depthDataMap = matteData.mattingImage
+ }
+ mattePixelBuffer = depthDataMap
+ }
+
+ private func update() {
+ switch typeSegmentedCtl.selectedSegmentIndex {
+ case 0:
+ drawImage(image)
+ case 1:
+ guard let matte = mattePixelBuffer else {
+ showNoPortraitMatteAlert()
+ return
+ }
+ draw(pixelBuffer: matte)
+ case 2:
+ guard let cgOriginalImage = image?.cgImage else { return }
+ guard let matte = mattePixelBuffer else {
+ showNoPortraitMatteAlert()
+ return
+ }
+ let orgImage = CIImage(cgImage: cgOriginalImage)
+ let maskImage = CIImage(cvPixelBuffer: matte).resizeToSameSize(as: orgImage)
+ let filter = CIFilter(name: "CIBlendWithMask", parameters: [
+ kCIInputImageKey: orgImage,
+ kCIInputMaskImageKey: maskImage])!
+ let outputImage = filter.outputImage!
+ drawImage(UIImage(ciImage: outputImage))
+ default:
+ fatalError()
+ }
+ }
+
+ // MARK: - Actions
+
+ @IBAction func typeSegmentChanged(_ sender: UISegmentedControl) {
+ update()
+ }
+
+ @IBAction func pickerBtnTapped() {
+ let picker = AssetsPickerController()
+ picker.didSelectAssets = {(assets: Array) -> () in
+ if let asset = assets.first {
+ self.asset = asset
+
+ self.typeSegmentedCtl.selectedSegmentIndex = 0
+ }
+ }
+ let navigationController = UINavigationController(rootViewController: picker)
+ present(navigationController, animated: true, completion: nil)
+ }
+}
+
+extension CIImage {
+ func resizeToSameSize(as anotherImage: CIImage) -> CIImage {
+ let size1 = extent.size
+ let size2 = anotherImage.extent.size
+ let transform = CGAffineTransform(scaleX: size2.width / size1.width, y: size2.height / size1.height)
+ return transformed(by: transform)
+ }
+}
diff --git a/iOS-Depth-Sampler/Samples/Realtime-Depth/Base.lproj/RealtimeDepth.storyboard b/iOS-Depth-Sampler/Samples/Realtime-Depth/Base.lproj/RealtimeDepth.storyboard
new file mode 100644
index 0000000..b27cef8
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Realtime-Depth/Base.lproj/RealtimeDepth.storyboard
@@ -0,0 +1,135 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Samples/Realtime-Depth/RealtimeDepthViewController.swift b/iOS-Depth-Sampler/Samples/Realtime-Depth/RealtimeDepthViewController.swift
new file mode 100644
index 0000000..a498e50
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Realtime-Depth/RealtimeDepthViewController.swift
@@ -0,0 +1,113 @@
+//
+// RealtimeDepthViewController.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/20.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+import MetalKit
+import AVFoundation
+
+class RealtimeDepthViewController: UIViewController {
+
+ @IBOutlet weak var previewView: UIView!
+ @IBOutlet weak var mtkView: MTKView!
+ @IBOutlet weak var filterSwitch: UISwitch!
+ @IBOutlet weak var disparitySwitch: UISwitch!
+ @IBOutlet weak var equalizeSwitch: UISwitch!
+
+ private var videoCapture: VideoCapture!
+ var currentCameraType: CameraType = .back(true)
+ private let serialQueue = DispatchQueue(label: "com.shu223.iOS-Depth-Sampler.queue")
+
+ private var renderer: MetalRenderer!
+ private var depthImage: CIImage?
+ private var currentDrawableSize: CGSize!
+
+ private var videoImage: CIImage?
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+
+ let device = MTLCreateSystemDefaultDevice()!
+ mtkView.device = device
+ mtkView.backgroundColor = UIColor.clear
+ mtkView.delegate = self
+ renderer = MetalRenderer(metalDevice: device, renderDestination: mtkView)
+ currentDrawableSize = mtkView.currentDrawable!.layer.drawableSize
+
+ videoCapture = VideoCapture(cameraType: currentCameraType,
+ preferredSpec: nil,
+ previewContainer: previewView.layer)
+
+ videoCapture.syncedDataBufferHandler = { [weak self] videoPixelBuffer, depthData, face in
+ guard let self = self else { return }
+
+ self.videoImage = CIImage(cvPixelBuffer: videoPixelBuffer)
+
+ var useDisparity: Bool = false
+ var applyHistoEq: Bool = false
+ DispatchQueue.main.sync(execute: {
+ useDisparity = self.disparitySwitch.isOn
+ applyHistoEq = self.equalizeSwitch.isOn
+ })
+
+ self.serialQueue.async {
+ guard let depthData = useDisparity ? depthData?.convertToDisparity() : depthData else { return }
+
+ guard let ciImage = depthData.depthDataMap.transformedImage(targetSize: self.currentDrawableSize, rotationAngle: 0) else { return }
+ self.depthImage = applyHistoEq ? ciImage.applyingFilter("YUCIHistogramEqualization") : ciImage
+ }
+ }
+ videoCapture.setDepthFilterEnabled(filterSwitch.isOn)
+ }
+
+ override func viewWillAppear(_ animated: Bool) {
+ super.viewWillAppear(animated)
+ guard let videoCapture = videoCapture else {return}
+ videoCapture.startCapture()
+ }
+
+ override func viewDidLayoutSubviews() {
+ super.viewDidLayoutSubviews()
+ guard let videoCapture = videoCapture else {return}
+ videoCapture.resizePreview()
+ }
+
+ override func viewWillDisappear(_ animated: Bool) {
+ guard let videoCapture = videoCapture else {return}
+ videoCapture.imageBufferHandler = nil
+ videoCapture.stopCapture()
+ mtkView.delegate = nil
+ super.viewWillDisappear(animated)
+ }
+
+ // MARK: - Actions
+
+ @IBAction func cameraSwitchBtnTapped(_ sender: UIButton) {
+ switch currentCameraType {
+ case .back:
+ currentCameraType = .front(true)
+ case .front:
+ currentCameraType = .back(true)
+ }
+ videoCapture.changeCamera(with: currentCameraType)
+ }
+
+ @IBAction func filterSwitched(_ sender: UISwitch) {
+ videoCapture.setDepthFilterEnabled(sender.isOn)
+ }
+}
+
+extension RealtimeDepthViewController: MTKViewDelegate {
+ func mtkView(_ view: MTKView, drawableSizeWillChange size: CGSize) {
+ currentDrawableSize = size
+ }
+
+ func draw(in view: MTKView) {
+ if let image = depthImage {
+ renderer.update(with: image)
+ }
+ }
+}
diff --git a/iOS-Depth-Sampler/Samples/Realtime-Mask/Base.lproj/RealtimeDepthMask.storyboard b/iOS-Depth-Sampler/Samples/Realtime-Mask/Base.lproj/RealtimeDepthMask.storyboard
new file mode 100644
index 0000000..da2df94
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Realtime-Mask/Base.lproj/RealtimeDepthMask.storyboard
@@ -0,0 +1,138 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/iOS-Depth-Sampler/Samples/Realtime-Mask/RealtimeDepthMaskViewController.swift b/iOS-Depth-Sampler/Samples/Realtime-Mask/RealtimeDepthMaskViewController.swift
new file mode 100644
index 0000000..a302702
--- /dev/null
+++ b/iOS-Depth-Sampler/Samples/Realtime-Mask/RealtimeDepthMaskViewController.swift
@@ -0,0 +1,276 @@
+//
+// RealtimeDepthViewController.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/20.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+import MetalKit
+import AVFoundation
+
+class RealtimeDepthMaskViewController: UIViewController {
+
+ @IBOutlet weak var mtkView: MTKView!
+ @IBOutlet weak var segmentedCtl: UISegmentedControl!
+ @IBOutlet weak var binarizeSwitch: UISwitch!
+ @IBOutlet weak var filterSwitch: UISwitch!
+ @IBOutlet weak var gammaSwitch: UISwitch!
+
+ private var videoCapture: VideoCapture!
+ private var currentCameraType: CameraType = .front(true)
+ private let serialQueue = DispatchQueue(label: "com.shu223.iOS-Depth-Sampler.queue")
+ private var currentCaptureSize: CGSize = CGSize.zero
+
+ private var renderer: MetalRenderer!
+
+ private var bgUIImages: [UIImage] = []
+ private var bgImages: [CIImage] = []
+ private var bgImageIndex: Int = 0
+ private var videoImage: CIImage?
+ private var maskImage: CIImage?
+
+ override func viewDidLoad() {
+ super.viewDidLoad()
+
+ for index in 1...24 {
+ let filename = String(format: "burn%06d", index)
+ let image = UIImage(named: filename)!
+ bgUIImages.append(image)
+ }
+
+ let device = MTLCreateSystemDefaultDevice()!
+ mtkView.device = device
+ mtkView.backgroundColor = UIColor.clear
+ mtkView.delegate = self
+
+ renderer = MetalRenderer(metalDevice: device, renderDestination: mtkView)
+
+ videoCapture = VideoCapture(cameraType: currentCameraType,
+ preferredSpec: nil,
+ previewContainer: nil)
+
+ videoCapture.syncedDataBufferHandler = { [weak self] videoPixelBuffer, depthData, face in
+ guard let self = self else { return }
+
+ self.videoImage = CIImage(cvPixelBuffer: videoPixelBuffer)
+
+ let videoWidth = CVPixelBufferGetWidth(videoPixelBuffer)
+ let videoHeight = CVPixelBufferGetHeight(videoPixelBuffer)
+
+ let captureSize = CGSize(width: videoWidth, height: videoHeight)
+ guard self.currentCaptureSize == captureSize else {
+ // Update the images' size
+ self.bgImages.removeAll()
+ self.bgImages = self.bgUIImages.map {
+ return $0.adjustedCIImage(targetSize: captureSize)!
+ }
+ self.currentCaptureSize = captureSize
+ return
+ }
+
+ DispatchQueue.main.async(execute: {
+ let binarize = self.binarizeSwitch.isOn
+ let gamma = self.gammaSwitch.isOn
+ self.serialQueue.async {
+ guard let depthPixelBuffer = depthData?.depthDataMap else { return }
+ self.processBuffer(videoPixelBuffer: videoPixelBuffer, depthPixelBuffer: depthPixelBuffer, face: face, shouldBinarize: binarize, shouldGamma: gamma)
+ }
+ })
+ }
+
+ videoCapture.setDepthFilterEnabled(filterSwitch.isOn)
+ }
+
+ override func viewWillAppear(_ animated: Bool) {
+ super.viewWillAppear(animated)
+ guard let videoCapture = videoCapture else {return}
+ videoCapture.startCapture()
+ }
+
+ override func viewDidLayoutSubviews() {
+ super.viewDidLayoutSubviews()
+ guard let videoCapture = videoCapture else {return}
+ videoCapture.resizePreview()
+ }
+
+ override func viewWillDisappear(_ animated: Bool) {
+ guard let videoCapture = videoCapture else {return}
+ videoCapture.imageBufferHandler = nil
+ videoCapture.stopCapture()
+ mtkView.delegate = nil
+ super.viewWillDisappear(animated)
+ }
+
+ @IBAction func cameraSwitchBtnTapped(_ sender: UIButton) {
+ switch currentCameraType {
+ case .back:
+ currentCameraType = .front(true)
+ case .front:
+ currentCameraType = .back(true)
+ }
+ bgImageIndex = 0
+ videoCapture.changeCamera(with: currentCameraType)
+ }
+
+ @IBAction func filterSwitched(_ sender: UISwitch) {
+ videoCapture.setDepthFilterEnabled(sender.isOn)
+ }
+}
+
+extension RealtimeDepthMaskViewController {
+ private func readDepth(from depthPixelBuffer: CVPixelBuffer, at position: CGPoint, scaleFactor: CGFloat) -> Float {
+ let pixelX = Int((position.x * scaleFactor).rounded())
+ let pixelY = Int((position.y * scaleFactor).rounded())
+
+ CVPixelBufferLockBaseAddress(depthPixelBuffer, .readOnly)
+
+ let rowData = CVPixelBufferGetBaseAddress(depthPixelBuffer)! + pixelY * CVPixelBufferGetBytesPerRow(depthPixelBuffer)
+ let faceCenterDepth = rowData.assumingMemoryBound(to: Float32.self)[pixelX]
+ CVPixelBufferUnlockBaseAddress(depthPixelBuffer, .readOnly)
+
+ return faceCenterDepth
+ }
+
+ func processBuffer(videoPixelBuffer: CVPixelBuffer, depthPixelBuffer: CVPixelBuffer, face: AVMetadataObject?, shouldBinarize: Bool, shouldGamma: Bool) {
+ let videoWidth = CVPixelBufferGetWidth(videoPixelBuffer)
+ let depthWidth = CVPixelBufferGetWidth(depthPixelBuffer)
+
+ var depthCutOff: Float = 1.0
+ if let face = face {
+ let faceCenter = CGPoint(x: face.bounds.midX, y: face.bounds.midY)
+ let scaleFactor = CGFloat(depthWidth) / CGFloat(videoWidth)
+ let faceCenterDepth = readDepth(from: depthPixelBuffer, at: faceCenter, scaleFactor: scaleFactor)
+ depthCutOff = faceCenterDepth + 0.25
+ }
+
+ // 二値化
+ // Convert depth map in-place: every pixel above cutoff is converted to 1. otherwise it's 0
+ if shouldBinarize {
+ depthPixelBuffer.binarize(cutOff: depthCutOff)
+ }
+
+ // Create the mask from that pixel buffer.
+ let depthImage = CIImage(cvPixelBuffer: depthPixelBuffer, options: [:])
+
+ // Smooth edges to create an alpha matte, then upscale it to the RGB resolution.
+ let alphaUpscaleFactor = Float(CVPixelBufferGetWidth(videoPixelBuffer)) / Float(depthWidth)
+ let processedDepth: CIImage
+ processedDepth = shouldGamma ? depthImage.applyBlurAndGamma() : depthImage
+
+ self.maskImage = processedDepth.applyingFilter("CIBicubicScaleTransform", parameters: ["inputScale": alphaUpscaleFactor])
+ }
+}
+
+extension CVPixelBuffer {
+
+ func binarize(cutOff: Float) {
+ CVPixelBufferLockBaseAddress(self, CVPixelBufferLockFlags(rawValue: 0))
+ let width = CVPixelBufferGetWidth(self)
+ let height = CVPixelBufferGetHeight(self)
+ for yMap in 0 ..< height {
+ let rowData = CVPixelBufferGetBaseAddress(self)! + yMap * CVPixelBufferGetBytesPerRow(self)
+ let data = UnsafeMutableBufferPointer(start: rowData.assumingMemoryBound(to: Float32.self), count: width)
+ for index in 0 ..< width {
+ if data[index] > 0 && data[index] <= cutOff {
+ data[index] = 1.0
+ } else {
+ data[index] = 0.0
+ }
+ }
+ }
+ CVPixelBufferUnlockBaseAddress(self, CVPixelBufferLockFlags(rawValue: 0))
+ }
+}
+
+extension CIImage {
+ func applyBlurAndGamma() -> CIImage {
+ return clampedToExtent()
+ .applyingFilter("CIGaussianBlur", parameters: ["inputRadius": 3.0])
+ .applyingFilter("CIGammaAdjust", parameters: ["inputPower": 0.5])
+ .cropped(to: extent)
+ }
+}
+
+extension RealtimeDepthMaskViewController: MTKViewDelegate {
+ func mtkView(_ view: MTKView, drawableSizeWillChange size: CGSize) {
+ }
+
+ func draw(in view: MTKView) {
+ switch segmentedCtl.selectedSegmentIndex {
+ case 0:
+ // original
+ if let image = videoImage {
+ renderer.update(with: image)
+ }
+ case 1:
+ // depth
+ if let image = maskImage {
+ renderer.update(with: image)
+ }
+ case 2:
+ // blended
+ guard let image = videoImage, let maskImage = maskImage else { return }
+
+ var parameters = ["inputMaskImage": maskImage]
+
+ let index = self.bgImageIndex
+ let bgImage = self.bgImages[index]
+ parameters["inputBackgroundImage"] = bgImage
+ self.bgImageIndex = index == self.bgImages.count - 1 ? 0 : index + 1
+
+ let outputImage = image.applyingFilter("CIBlendWithMask", parameters: parameters)
+ renderer.update(with: outputImage)
+ default:
+ return
+ }
+ }
+}
+
+extension UIImage {
+ func adjustedCIImage(targetSize: CGSize) -> CIImage? {
+ guard let cgImage = cgImage else { fatalError() }
+
+ let imageWidth = cgImage.width
+ let imageHeight = cgImage.height
+
+ // Video preview is running at 1280x720. Downscale background to same resolution
+ let videoWidth = Int(targetSize.width)
+ let videoHeight = Int(targetSize.height)
+
+ let scaleX = CGFloat(imageWidth) / CGFloat(videoWidth)
+ let scaleY = CGFloat(imageHeight) / CGFloat(videoHeight)
+
+ let scale = min(scaleX, scaleY)
+
+ // crop the image to have the right aspect ratio
+ let cropSize = CGSize(width: CGFloat(videoWidth) * scale, height: CGFloat(videoHeight) * scale)
+ let croppedImage = cgImage.cropping(to: CGRect(origin: CGPoint(
+ x: (imageWidth - Int(cropSize.width)) / 2,
+ y: (imageHeight - Int(cropSize.height)) / 2), size: cropSize))
+
+ let colorSpace = CGColorSpaceCreateDeviceRGB()
+ guard let context = CGContext(data: nil,
+ width: videoWidth,
+ height: videoHeight,
+ bitsPerComponent: 8,
+ bytesPerRow: 0,
+ space: colorSpace,
+ bitmapInfo: CGImageAlphaInfo.premultipliedLast.rawValue) else {
+ print("error")
+ return nil
+ }
+
+ let bounds = CGRect(origin: CGPoint(x: 0, y: 0), size: CGSize(width: videoWidth, height: videoHeight))
+ context.clear(bounds)
+
+ context.draw(croppedImage!, in: bounds)
+
+ guard let scaledImage = context.makeImage() else {
+ print("failed")
+ return nil
+ }
+
+ return CIImage(cgImage: scaledImage)
+ }
+}
diff --git a/iOS-Depth-Sampler/Utils/AVDepthData+Utils.swift b/iOS-Depth-Sampler/Utils/AVDepthData+Utils.swift
new file mode 100644
index 0000000..589aa4d
--- /dev/null
+++ b/iOS-Depth-Sampler/Utils/AVDepthData+Utils.swift
@@ -0,0 +1,34 @@
+//
+// AVDepthData+Utils.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/12.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import AVFoundation
+
+extension AVDepthData {
+
+ func convertToDepth() -> AVDepthData {
+ switch depthDataType {
+ case kCVPixelFormatType_DisparityFloat16:
+ return converting(toDepthDataType: kCVPixelFormatType_DepthFloat16)
+ case kCVPixelFormatType_DisparityFloat32:
+ return converting(toDepthDataType: kCVPixelFormatType_DepthFloat32)
+ default:
+ return self
+ }
+ }
+
+ func convertToDisparity() -> AVDepthData {
+ switch depthDataType {
+ case kCVPixelFormatType_DepthFloat16:
+ return converting(toDepthDataType: kCVPixelFormatType_DisparityFloat16)
+ case kCVPixelFormatType_DepthFloat32:
+ return converting(toDepthDataType: kCVPixelFormatType_DisparityFloat32)
+ default:
+ return self
+ }
+ }
+}
diff --git a/iOS-Depth-Sampler/Utils/CGImageSource+Depth.swift b/iOS-Depth-Sampler/Utils/CGImageSource+Depth.swift
new file mode 100644
index 0000000..245da2a
--- /dev/null
+++ b/iOS-Depth-Sampler/Utils/CGImageSource+Depth.swift
@@ -0,0 +1,65 @@
+//
+// CGImageSource+Depth.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/30.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import ImageIO
+import AVFoundation
+
+extension CGImageSource {
+
+ var auxiliaryDataProperties: [[String : AnyObject]]? {
+ guard let sourceProperties = CGImageSourceCopyProperties(self, nil) as? [String: AnyObject] else { fatalError() }
+ guard let fileContentsProperties = sourceProperties[String(kCGImagePropertyFileContentsDictionary)] as? [String : AnyObject] else { fatalError() }
+ guard let images = fileContentsProperties[String(kCGImagePropertyImages)] as? [AnyObject] else { return nil }
+ for imageProperties in images {
+ guard let auxiliaryDataProperties = imageProperties[String(kCGImagePropertyAuxiliaryData)] as? [[String : AnyObject]] else { continue }
+ return auxiliaryDataProperties
+ }
+ return nil
+ }
+
+ /* Depth data support for JPEG, HEIF, and DNG images.
+ * The returned CFDictionary contains:
+ * - the depth data (CFDataRef) - (kCGImageAuxiliaryDataInfoData),
+ * - the depth data description (CFDictionary) - (kCGImageAuxiliaryDataInfoDataDescription)
+ * - metadata (CGImageMetadataRef) - (kCGImageAuxiliaryDataInfoMetadata)
+ * CGImageSourceCopyAuxiliaryDataInfoAtIndex returns nil if the image did not contain ‘auxiliaryImageDataType’ data.
+ */
+ private var disparityDataInfo: [String : AnyObject]? {
+ return CGImageSourceCopyAuxiliaryDataInfoAtIndex(self, 0, kCGImageAuxiliaryDataTypeDisparity) as? [String : AnyObject]
+ }
+
+ private var depthDataInfo: [String : AnyObject]? {
+ return CGImageSourceCopyAuxiliaryDataInfoAtIndex(self, 0, kCGImageAuxiliaryDataTypeDepth) as? [String : AnyObject]
+ }
+
+ @available(iOS 12.0, *)
+ private var portraitEffectsMatteDataInfo: [String : AnyObject]? {
+ return CGImageSourceCopyAuxiliaryDataInfoAtIndex(self, 0, kCGImageAuxiliaryDataTypePortraitEffectsMatte) as? [String : AnyObject]
+ }
+
+ func getDisparityData() -> AVDepthData? {
+ if let disparityDataInfo = disparityDataInfo {
+ return try! AVDepthData(fromDictionaryRepresentation: disparityDataInfo)
+ }
+ return nil
+ }
+
+ func getDepthData() -> AVDepthData? {
+ if let depthDataInfo = depthDataInfo {
+ return try! AVDepthData(fromDictionaryRepresentation: depthDataInfo)
+ }
+ return nil
+ }
+
+ @available(iOS 12.0, *)
+ func getMatteData() -> AVPortraitEffectsMatte? {
+ if let info = portraitEffectsMatteDataInfo {
+ return try? AVPortraitEffectsMatte(fromDictionaryRepresentation: info)
+ }
+ return nil
+ }
+}
diff --git a/iOS-Depth-Sampler/Utils/CVPixelBuffer+CIImage.swift b/iOS-Depth-Sampler/Utils/CVPixelBuffer+CIImage.swift
new file mode 100644
index 0000000..77b81c8
--- /dev/null
+++ b/iOS-Depth-Sampler/Utils/CVPixelBuffer+CIImage.swift
@@ -0,0 +1,18 @@
+//
+// CVPixelBuffer+CIImage.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/12.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import CoreVideo
+import CoreImage
+
+extension CVPixelBuffer {
+ func transformedImage(targetSize: CGSize, rotationAngle: CGFloat) -> CIImage? {
+ let image = CIImage(cvPixelBuffer: self, options: [:])
+ let scaleFactor = Float(targetSize.width) / Float(image.extent.width)
+ return image.transformed(by: CGAffineTransform(rotationAngle: rotationAngle)).applyingFilter("CIBicubicScaleTransform", parameters: ["inputScale": scaleFactor])
+ }
+}
diff --git a/iOS-Depth-Sampler/Utils/PhotosUtils.swift b/iOS-Depth-Sampler/Utils/PhotosUtils.swift
new file mode 100644
index 0000000..1c65441
--- /dev/null
+++ b/iOS-Depth-Sampler/Utils/PhotosUtils.swift
@@ -0,0 +1,56 @@
+//
+// PhotosUtils.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/12.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import Photos
+
+extension PHAsset {
+ class func fetchAssetsWithDepth() -> [PHAsset] {
+ let resultCollections = PHAssetCollection.fetchAssetCollections(
+ with: .smartAlbum,
+ subtype: .smartAlbumDepthEffect,
+ options: nil)
+ var assets: [PHAsset] = []
+ resultCollections.enumerateObjects({ collection, index, stop in
+ let result = PHAsset.fetchAssets(in: collection, options: nil)
+ result.enumerateObjects({ asset, index, stop in
+ assets.append(asset)
+ })
+ })
+ return assets
+ }
+
+ func requestColorImage(handler: @escaping (UIImage?) -> Void) {
+ PHImageManager.default().requestImage(for: self, targetSize: PHImageManagerMaximumSize, contentMode: PHImageContentMode.aspectFit, options: nil) { (image, info) in
+ handler(image)
+ }
+ }
+
+ func hasPortraitMatte() -> Bool {
+ var result: Bool = false
+ let semaphore = DispatchSemaphore(value: 0)
+ requestContentEditingInput(with: nil) { contentEditingInput, info in
+ let imageSource = contentEditingInput?.createImageSource()
+ result = imageSource?.getMatteData() != nil
+ semaphore.signal()
+ }
+ semaphore.wait()
+ return result
+ }
+}
+
+extension PHContentEditingInput {
+ func createDepthImage() -> CIImage {
+ guard let url = fullSizeImageURL else { fatalError() }
+ return CIImage(contentsOf: url, options: [CIImageOption.auxiliaryDisparity : true])!
+ }
+
+ func createImageSource() -> CGImageSource {
+ guard let url = fullSizeImageURL else { fatalError() }
+ return CGImageSourceCreateWithURL(url as CFURL, nil)!
+ }
+}
diff --git a/iOS-Depth-Sampler/Utils/UIAlertController+Utils.swift b/iOS-Depth-Sampler/Utils/UIAlertController+Utils.swift
new file mode 100644
index 0000000..7bf336d
--- /dev/null
+++ b/iOS-Depth-Sampler/Utils/UIAlertController+Utils.swift
@@ -0,0 +1,20 @@
+//
+// UIAlertController+Utils.swift
+// iOS-Depth-Sampler
+//
+// Created by Shuichi Tsutsumi on 2018/09/12.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+
+extension UIAlertController {
+
+ class func showAlert(title: String, message: String, on viewController: UIViewController) {
+ let alert = UIAlertController(title: title, message: message,
+ preferredStyle: .alert)
+ let okAction = UIAlertAction(title: "OK", style: .cancel, handler: nil)
+ alert.addAction(okAction)
+ viewController.present(alert, animated: true, completion: nil)
+ }
+}
diff --git a/iOS-Depth-Sampler/Utils/UIImage+CVPixelBuffer.swift b/iOS-Depth-Sampler/Utils/UIImage+CVPixelBuffer.swift
new file mode 100644
index 0000000..76933db
--- /dev/null
+++ b/iOS-Depth-Sampler/Utils/UIImage+CVPixelBuffer.swift
@@ -0,0 +1,50 @@
+//
+// UIImage+CVPixelBuffer.swift
+//
+// Created by Shuichi Tsutsumi on 2018/08/28.
+// Copyright © 2018 Shuichi Tsutsumi. All rights reserved.
+//
+
+import UIKit
+//import VideoToolbox
+
+extension UIImage {
+ // https://github.com/hollance/CoreMLHelpers
+// NOTE: This only works for RGB pixel buffers, not for grayscale.
+// public convenience init?(pixelBuffer: CVPixelBuffer) {
+// var cgImage: CGImage?
+// VTCreateCGImageFromCVPixelBuffer(pixelBuffer, options: nil, imageOut: &cgImage)
+//
+// if let cgImage = cgImage {
+// self.init(cgImage: cgImage)
+// } else {
+// return nil
+// }
+// }
+//
+// /**
+// Creates a new UIImage from a CVPixelBuffer, using Core Image.
+// */
+// public convenience init?(pixelBuffer: CVPixelBuffer, context: CIContext) {
+// let ciImage = CIImage(cvPixelBuffer: pixelBuffer)
+// let rect = CGRect(x: 0, y: 0, width: CVPixelBufferGetWidth(pixelBuffer),
+// height: CVPixelBufferGetHeight(pixelBuffer))
+// if let cgImage = context.createCGImage(ciImage, from: rect) {
+// self.init(cgImage: cgImage)
+// } else {
+// return nil
+// }
+// }
+
+ public convenience init?(pixelBuffer: CVPixelBuffer) {
+ let ciImage = CIImage(cvPixelBuffer: pixelBuffer)
+ let pixelBufferWidth = CGFloat(CVPixelBufferGetWidth(pixelBuffer))
+ let pixelBufferHeight = CGFloat(CVPixelBufferGetHeight(pixelBuffer))
+ let imageRect:CGRect = CGRect(x: 0, y: 0, width: pixelBufferWidth, height: pixelBufferHeight)
+ let ciContext = CIContext.init()
+ guard let cgImage = ciContext.createCGImage(ciImage, from: imageRect) else {
+ return nil
+ }
+ self.init(cgImage: cgImage)
+ }
+}
diff --git a/iOS-Depth-Sampler/VideoCapture/AVCaptureDevice+Extension.swift b/iOS-Depth-Sampler/VideoCapture/AVCaptureDevice+Extension.swift
new file mode 100644
index 0000000..556df36
--- /dev/null
+++ b/iOS-Depth-Sampler/VideoCapture/AVCaptureDevice+Extension.swift
@@ -0,0 +1,50 @@
+//
+// AVCaptureDevice+Extension.swift
+//
+// Created by Shuichi Tsutsumi on 4/3/16.
+// Copyright © 2016 Shuichi Tsutsumi. All rights reserved.
+//
+
+import AVFoundation
+
+extension AVCaptureDevice {
+ private func formatWithHighestResolution(_ availableFormats: [AVCaptureDevice.Format]) -> AVCaptureDevice.Format?
+ {
+ var maxWidth: Int32 = 0
+ var selectedFormat: AVCaptureDevice.Format?
+ for format in availableFormats {
+ let dimensions = CMVideoFormatDescriptionGetDimensions(format.formatDescription)
+ let width = dimensions.width
+ if width >= maxWidth {
+ maxWidth = width
+ selectedFormat = format
+ }
+ }
+ return selectedFormat
+ }
+
+ func selectDepthFormat() {
+ let availableFormats = formats.filter { format -> Bool in
+ let validDepthFormats = format.supportedDepthDataFormats.filter{ depthFormat in
+ return CMFormatDescriptionGetMediaSubType(depthFormat.formatDescription) == kCVPixelFormatType_DepthFloat32
+ }
+ return validDepthFormats.count > 0
+ }
+ guard let selectedFormat = formatWithHighestResolution(availableFormats) else { fatalError() }
+
+ let depthFormats = selectedFormat.supportedDepthDataFormats
+ let depth32formats = depthFormats.filter({
+ CMFormatDescriptionGetMediaSubType($0.formatDescription) == kCVPixelFormatType_DepthFloat32
+ })
+ guard !depth32formats.isEmpty else { fatalError() }
+ let selectedDepthFormat = depth32formats.max(by: { first, second in
+ CMVideoFormatDescriptionGetDimensions(first.formatDescription).width <
+ CMVideoFormatDescriptionGetDimensions(second.formatDescription).width })!
+
+ print("selected format: \(selectedFormat), depth format: \(selectedDepthFormat)")
+ try! lockForConfiguration()
+ activeFormat = selectedFormat
+ activeDepthDataFormat = selectedDepthFormat
+ unlockForConfiguration()
+ }
+}
diff --git a/iOS-Depth-Sampler/VideoCapture/VideoCameraType.swift b/iOS-Depth-Sampler/VideoCapture/VideoCameraType.swift
new file mode 100644
index 0000000..e547679
--- /dev/null
+++ b/iOS-Depth-Sampler/VideoCapture/VideoCameraType.swift
@@ -0,0 +1,35 @@
+//
+// VideoCameraType.swift
+//
+// Created by Shuichi Tsutsumi on 4/3/16.
+// Copyright © 2016 Shuichi Tsutsumi. All rights reserved.
+//
+
+import AVFoundation
+
+enum CameraType {
+ case back(Bool)
+ case front(Bool)
+
+ func captureDevice() -> AVCaptureDevice {
+ let devices: [AVCaptureDevice]
+ switch self {
+ case .front(let requireDepth):
+ var deviceTypes: [AVCaptureDevice.DeviceType] = [.builtInTrueDepthCamera]
+ if !requireDepth {
+ deviceTypes.append(.builtInWideAngleCamera)
+ }
+ devices = AVCaptureDevice.DiscoverySession(deviceTypes: deviceTypes, mediaType: .video, position: .front).devices
+ case .back(let requireDepth):
+ var deviceTypes: [AVCaptureDevice.DeviceType] = [.builtInDualCamera]
+ if !requireDepth {
+ deviceTypes.append(contentsOf: [.builtInWideAngleCamera, .builtInTelephotoCamera])
+ }
+ devices = AVCaptureDevice.DiscoverySession(deviceTypes: deviceTypes, mediaType: .video, position: .back).devices
+ }
+ guard let device = devices.first else {
+ return AVCaptureDevice.default(for: .video)!
+ }
+ return device
+ }
+}
diff --git a/iOS-Depth-Sampler/VideoCapture/VideoCapture.swift b/iOS-Depth-Sampler/VideoCapture/VideoCapture.swift
new file mode 100644
index 0000000..a05be8b
--- /dev/null
+++ b/iOS-Depth-Sampler/VideoCapture/VideoCapture.swift
@@ -0,0 +1,243 @@
+//
+// VideoCapture.swift
+//
+// Created by Shuichi Tsutsumi on 4/3/16.
+// Copyright © 2016 Shuichi Tsutsumi. All rights reserved.
+//
+
+import AVFoundation
+import Foundation
+
+
+struct VideoSpec {
+ var fps: Int32?
+ var size: CGSize?
+}
+
+typealias ImageBufferHandler = (CVPixelBuffer, CMTime, CVPixelBuffer?) -> Void
+typealias SynchronizedDataBufferHandler = (CVPixelBuffer, AVDepthData?, AVMetadataObject?) -> Void
+
+extension AVCaptureDevice {
+ func printDepthFormats() {
+ formats.forEach { (format) in
+ let depthFormats = format.supportedDepthDataFormats
+ if depthFormats.count > 0 {
+ print("format: \(format), supported depth formats: \(depthFormats)")
+ }
+ }
+ }
+}
+
+class VideoCapture: NSObject {
+
+ private let captureSession = AVCaptureSession()
+ private var videoDevice: AVCaptureDevice!
+ private var videoConnection: AVCaptureConnection!
+ private var previewLayer: AVCaptureVideoPreviewLayer?
+
+ private let dataOutputQueue = DispatchQueue(label: "com.shu223.dataOutputQueue")
+
+ var imageBufferHandler: ImageBufferHandler?
+ var syncedDataBufferHandler: SynchronizedDataBufferHandler?
+
+ // プロパティで保持しておかないとdelegate呼ばれない
+ // AVCaptureDepthDataOutputはプロパティで保持しなくても大丈夫(CaptureSessionにaddOutputするからだと思う)
+ private var dataOutputSynchronizer: AVCaptureDataOutputSynchronizer!
+
+ // プロパティに保持しておかなくてもOKだが、AVCaptureSynchronizedDataCollectionからデータを取り出す際にあると便利
+ private let videoDataOutput = AVCaptureVideoDataOutput()
+ private let depthDataOutput = AVCaptureDepthDataOutput()
+ private let metadataOutput = AVCaptureMetadataOutput()
+
+ init(cameraType: CameraType, preferredSpec: VideoSpec?, previewContainer: CALayer?)
+ {
+ super.init()
+
+ captureSession.beginConfiguration()
+
+ // inputPriorityだと深度とれない
+ captureSession.sessionPreset = AVCaptureSession.Preset.photo
+
+ setupCaptureVideoDevice(with: cameraType)
+
+ // setup preview
+ if let previewContainer = previewContainer {
+ let previewLayer = AVCaptureVideoPreviewLayer(session: captureSession)
+ previewLayer.frame = previewContainer.bounds
+ previewLayer.contentsGravity = CALayerContentsGravity.resizeAspectFill
+ previewLayer.videoGravity = .resizeAspectFill
+ previewContainer.insertSublayer(previewLayer, at: 0)
+ self.previewLayer = previewLayer
+ }
+
+ // setup outputs
+ do {
+ // video output
+ videoDataOutput.videoSettings = [kCVPixelBufferPixelFormatTypeKey as String: Int(kCVPixelFormatType_32BGRA)]
+ videoDataOutput.alwaysDiscardsLateVideoFrames = true
+ videoDataOutput.setSampleBufferDelegate(self, queue: dataOutputQueue)
+ guard captureSession.canAddOutput(videoDataOutput) else { fatalError() }
+ captureSession.addOutput(videoDataOutput)
+ videoConnection = videoDataOutput.connection(with: .video)
+
+ // depth output
+ guard captureSession.canAddOutput(depthDataOutput) else { fatalError() }
+ captureSession.addOutput(depthDataOutput)
+ depthDataOutput.setDelegate(self, callbackQueue: dataOutputQueue)
+ depthDataOutput.isFilteringEnabled = false
+ guard let connection = depthDataOutput.connection(with: .depthData) else { fatalError() }
+ connection.isEnabled = true
+
+ // metadata output
+ guard captureSession.canAddOutput(metadataOutput) else { fatalError() }
+ captureSession.addOutput(metadataOutput)
+ if metadataOutput.availableMetadataObjectTypes.contains(.face) {
+ metadataOutput.metadataObjectTypes = [.face]
+ }
+
+
+ // synchronize outputs
+ dataOutputSynchronizer = AVCaptureDataOutputSynchronizer(dataOutputs: [videoDataOutput, depthDataOutput, metadataOutput])
+ dataOutputSynchronizer.setDelegate(self, queue: dataOutputQueue)
+ }
+
+ setupConnections(with: cameraType)
+
+ captureSession.commitConfiguration()
+ }
+
+ private func setupCaptureVideoDevice(with cameraType: CameraType) {
+
+ videoDevice = cameraType.captureDevice()
+ print("selected video device: \(String(describing: videoDevice))")
+
+ videoDevice.selectDepthFormat()
+
+ captureSession.inputs.forEach { (captureInput) in
+ captureSession.removeInput(captureInput)
+ }
+ let videoDeviceInput = try! AVCaptureDeviceInput(device: videoDevice)
+ guard captureSession.canAddInput(videoDeviceInput) else { fatalError() }
+ captureSession.addInput(videoDeviceInput)
+ }
+
+ private func setupConnections(with cameraType: CameraType) {
+ videoConnection = videoDataOutput.connection(with: .video)!
+ let depthConnection = depthDataOutput.connection(with: .depthData)
+ switch cameraType {
+ case .front:
+ videoConnection.isVideoMirrored = true
+ depthConnection?.isVideoMirrored = true
+ default:
+ break
+ }
+ videoConnection.videoOrientation = .portrait
+ depthConnection?.videoOrientation = .portrait
+ }
+
+ func startCapture() {
+ print("\(self.classForCoder)/" + #function)
+ if captureSession.isRunning {
+ print("already running")
+ return
+ }
+ captureSession.startRunning()
+ }
+
+ func stopCapture() {
+ print("\(self.classForCoder)/" + #function)
+ if !captureSession.isRunning {
+ print("already stopped")
+ return
+ }
+ captureSession.stopRunning()
+ }
+
+ func resizePreview() {
+ if let previewLayer = previewLayer {
+ guard let superlayer = previewLayer.superlayer else {return}
+ previewLayer.frame = superlayer.bounds
+ }
+ }
+
+ func changeCamera(with cameraType: CameraType) {
+ let wasRunning = captureSession.isRunning
+ if wasRunning {
+ captureSession.stopRunning()
+ }
+ captureSession.beginConfiguration()
+
+ setupCaptureVideoDevice(with: cameraType)
+ setupConnections(with: cameraType)
+
+ captureSession.commitConfiguration()
+
+ if wasRunning {
+ captureSession.startRunning()
+ }
+ }
+
+ func setDepthFilterEnabled(_ enabled: Bool) {
+ depthDataOutput.isFilteringEnabled = enabled
+ }
+}
+
+extension VideoCapture: AVCaptureVideoDataOutputSampleBufferDelegate {
+
+ func captureOutput(_ output: AVCaptureOutput, didDrop sampleBuffer: CMSampleBuffer, from connection: AVCaptureConnection) {
+// print("\(self.classForCoder)/" + #function)
+ }
+
+ // synchronizer使ってる場合は呼ばれない
+ func captureOutput(_ output: AVCaptureOutput, didOutput sampleBuffer: CMSampleBuffer, from connection: AVCaptureConnection) {
+ if let imageBufferHandler = imageBufferHandler, connection == videoConnection
+ {
+ guard let imageBuffer = CMSampleBufferGetImageBuffer(sampleBuffer) else { fatalError() }
+
+ let timestamp = CMSampleBufferGetPresentationTimeStamp(sampleBuffer)
+ imageBufferHandler(imageBuffer, timestamp, nil)
+ }
+ }
+}
+
+extension VideoCapture: AVCaptureDepthDataOutputDelegate {
+
+ func depthDataOutput(_ output: AVCaptureDepthDataOutput, didDrop depthData: AVDepthData, timestamp: CMTime, connection: AVCaptureConnection, reason: AVCaptureOutput.DataDroppedReason) {
+ print("\(self.classForCoder)/\(#function)")
+ }
+
+ // synchronizer使ってる場合は呼ばれない
+ func depthDataOutput(_ output: AVCaptureDepthDataOutput, didOutput depthData: AVDepthData, timestamp: CMTime, connection: AVCaptureConnection) {
+ print("\(self.classForCoder)/\(#function)")
+ }
+}
+
+extension VideoCapture: AVCaptureDataOutputSynchronizerDelegate {
+
+ func dataOutputSynchronizer(_ synchronizer: AVCaptureDataOutputSynchronizer, didOutput synchronizedDataCollection: AVCaptureSynchronizedDataCollection) {
+
+ guard let syncedVideoData = synchronizedDataCollection.synchronizedData(for: videoDataOutput) as? AVCaptureSynchronizedSampleBufferData else { return }
+ guard !syncedVideoData.sampleBufferWasDropped else {
+ print("dropped video:\(syncedVideoData)")
+ return
+ }
+ let videoSampleBuffer = syncedVideoData.sampleBuffer
+
+ let syncedDepthData = synchronizedDataCollection.synchronizedData(for: depthDataOutput) as? AVCaptureSynchronizedDepthData
+ var depthData = syncedDepthData?.depthData
+ if let syncedDepthData = syncedDepthData, syncedDepthData.depthDataWasDropped {
+ print("dropped depth:\(syncedDepthData)")
+ depthData = nil
+ }
+
+ // 顔のある位置のしきい値を求める
+ let syncedMetaData = synchronizedDataCollection.synchronizedData(for: metadataOutput) as? AVCaptureSynchronizedMetadataObjectData
+ var face: AVMetadataObject? = nil
+ if let firstFace = syncedMetaData?.metadataObjects.first {
+ face = videoDataOutput.transformedMetadataObject(for: firstFace, connection: videoConnection)
+ }
+ guard let imagePixelBuffer = CMSampleBufferGetImageBuffer(videoSampleBuffer) else { fatalError() }
+
+ syncedDataBufferHandler?(imagePixelBuffer, depthData, face)
+ }
+}
diff --git a/iOS-Depth-Sampler/iOS-Depth-Sampler-Bridging-Header.h b/iOS-Depth-Sampler/iOS-Depth-Sampler-Bridging-Header.h
new file mode 100644
index 0000000..e11d920
--- /dev/null
+++ b/iOS-Depth-Sampler/iOS-Depth-Sampler-Bridging-Header.h
@@ -0,0 +1,3 @@
+//
+// Use this file to import your target's public headers that you would like to expose to Swift.
+//