|
| 1 | +# SlackMQ |
| 2 | + |
| 3 | +Enables a common Message Queue mechanism for "message locking" within Slack. |
| 4 | +When a message is sent to a channel, a Slack bot can acknowledge the message |
| 5 | +and “lock” it for processing. This keeps other worker bots from processing the |
| 6 | +message simultaneously. |
| 7 | + |
| 8 | +SlackMQ leverages the Slack API method for pinning, reactions and starring of |
| 9 | +messages, where only one is allowed per post per user (or bot) account. |
| 10 | + |
| 11 | +A Slack worker bot can use the same API token to connect to Slack up to 16 |
| 12 | +times. This is a restriction imposed by Slack. |
| 13 | + |
| 14 | +SlackMQ is not a replacement for dedicated Message Brokers and Queue systems. It |
| 15 | +only does simple message locking and is suitable for managing low bandwidth |
| 16 | +tasks or applications. For those use cases, it's one less moving part and makes |
| 17 | +Slack do the heavy lifting. |
| 18 | + |
| 19 | +To install: |
| 20 | +``` |
| 21 | +pip install SlackMQ |
| 22 | +``` |
| 23 | + |
| 24 | +## Message Locking Anatomy |
| 25 | + |
| 26 | +A star and pin action are both used to "lock" a message. |
| 27 | + |
| 28 | +The star is not seen in the Slack channel, as it is only visible by the user |
| 29 | +creating the star. |
| 30 | + |
| 31 | +Pins and reactions are visible to everyone in the channel. |
| 32 | + |
| 33 | +To avoid race conditions, where two or more bots simultaneously star the |
| 34 | +same message (the Slack API says it shouldn't but it can happen), a second |
| 35 | +action (the star) is invoked. An optional third action (a reaction emoji) for |
| 36 | +extra protection can also be invoked. |
| 37 | + |
| 38 | +## Example: The Slack Worker Bot |
| 39 | + |
| 40 | +For high availability and scalability reasons, multiple worker bots could be |
| 41 | +deployed through out a network. Using the Slackbot python library to listen and |
| 42 | +respond to certain Slack posts, all the bots will receive the message, but the |
| 43 | +first to acknowledge the message will be able to process it. |
| 44 | + |
| 45 | +``` |
| 46 | +from slackmq import slackmq |
| 47 | +from slackbot.bot import listen_to |
| 48 | +import socket |
| 49 | +
|
| 50 | +API_TOKEN = 'YOUR-BOT-API-TOKEN' |
| 51 | +
|
| 52 | +@listen_to('hostname') |
| 53 | +def myhostname(message): |
| 54 | + post = slackmq(API_TOKEN, |
| 55 | + message.body['channel'], |
| 56 | + message.body['ts']) |
| 57 | + if post.ack(): |
| 58 | + message.send('I am running on {}.'.format(socket.gethostname())) |
| 59 | + # Removes the visible pin (and hidden star) from the channel. |
| 60 | + post.unack() |
| 61 | +``` |
| 62 | + |
| 63 | +## Example: Continuous Delivery with SlackMQ |
| 64 | + |
| 65 | +Watch Continuous Delivery with SlackMQ in action, deploying a home automation |
| 66 | +application across several hosts. From Continuous Integration to an automated |
| 67 | +canary deployment. Once approved, a rolling deployment occurs with zero |
| 68 | +downtime. |
| 69 | + |
| 70 | +Video has no audio: |
| 71 | + |
| 72 | +[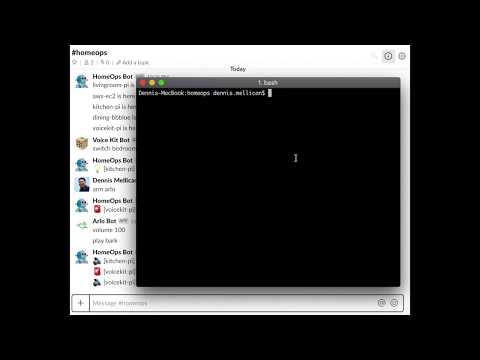](http://www.youtube.com/watch?v=YW6IdsvdxXI "Continuous Delivery with SlackMQ") |
0 commit comments