diff --git a/docs/Backpressure.md b/docs/Backpressure.md
index d9e7bfa65a..8feec0d487 100644
--- a/docs/Backpressure.md
+++ b/docs/Backpressure.md
@@ -20,7 +20,7 @@ Cold Observables are ideal for the reactive pull model of backpressure described
Your first line of defense against the problems of over-producing Observables is to use some of the ordinary set of Observable operators to reduce the number of emitted items to a more manageable number. The examples in this section will show how you might use such operators to handle a bursty Observable like the one illustrated in the following marble diagram:
-
+
By fine-tuning the parameters to these operators you can ensure that a slow-consuming observer is not overwhelmed by a fast-producing Observable.
@@ -33,7 +33,7 @@ The following diagrams show how you could use each of these operators on the bur
### sample (or throttleLast)
The `sample` operator periodically "dips" into the sequence and emits only the most recently emitted item during each dip:
-
+
````groovy
Observable burstySampled = bursty.sample(500, TimeUnit.MILLISECONDS);
````
@@ -41,7 +41,7 @@ Observable burstySampled = bursty.sample(500, TimeUnit.MILLISECONDS);
### throttleFirst
The `throttleFirst` operator is similar, but emits not the most recently emitted item, but the first item that was emitted after the previous "dip":
-
+
````groovy
Observable burstyThrottled = bursty.throttleFirst(500, TimeUnit.MILLISECONDS);
````
@@ -49,7 +49,7 @@ Observable burstyThrottled = bursty.throttleFirst(500, TimeUnit.MILLISE
### debounce (or throttleWithTimeout)
The `debounce` operator emits only those items from the source Observable that are not followed by another item within a specified duration:
-
+
````groovy
Observable burstyDebounced = bursty.debounce(10, TimeUnit.MILLISECONDS);
````
@@ -64,14 +64,14 @@ The following diagrams show how you could use each of these operators on the bur
You could, for example, close and emit a buffer of items from the bursty Observable periodically, at a regular interval of time:
-
+
````groovy
Observable> burstyBuffered = bursty.buffer(500, TimeUnit.MILLISECONDS);
````
Or you could get fancy, and collect items in buffers during the bursty periods and emit them at the end of each burst, by using the `debounce` operator to emit a buffer closing indicator to the `buffer` operator:
-
+
````groovy
// we have to multicast the original bursty Observable so we can use it
// both as our source and as the source for our buffer closing selector:
@@ -86,14 +86,14 @@ Observable> burstyBuffered = burstyMulticast.buffer(burstyDebounce
`window` is similar to `buffer`. One variant of `window` allows you to periodically emit Observable windows of items at a regular interval of time:
-
+
````groovy
Observable> burstyWindowed = bursty.window(500, TimeUnit.MILLISECONDS);
````
You could also choose to emit a new window each time you have collected a particular number of items from the source Observable:
-
+
````groovy
Observable> burstyWindowed = bursty.window(5);
````
@@ -158,11 +158,11 @@ For this to work, though, Observables _A_ and _B_ must respond correctly to the
- onBackpressureBuffer
- - maintains a buffer of all emissions from the source Observable and emits them to downstream Subscribers according to the requests they generate
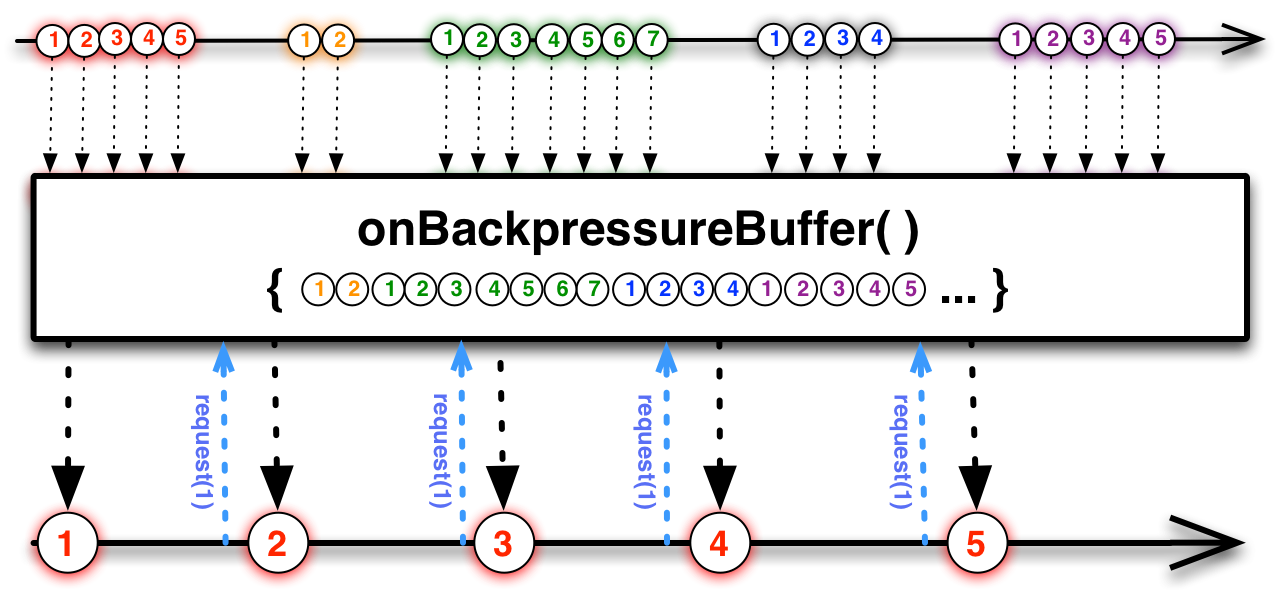
an experimental version of this operator (not available in RxJava 1.0) allows you to set the capacity of the buffer; applying this operator will cause the resulting Observable to terminate with an error if this buffer is overrun
+ - maintains a buffer of all emissions from the source Observable and emits them to downstream Subscribers according to the requests they generate
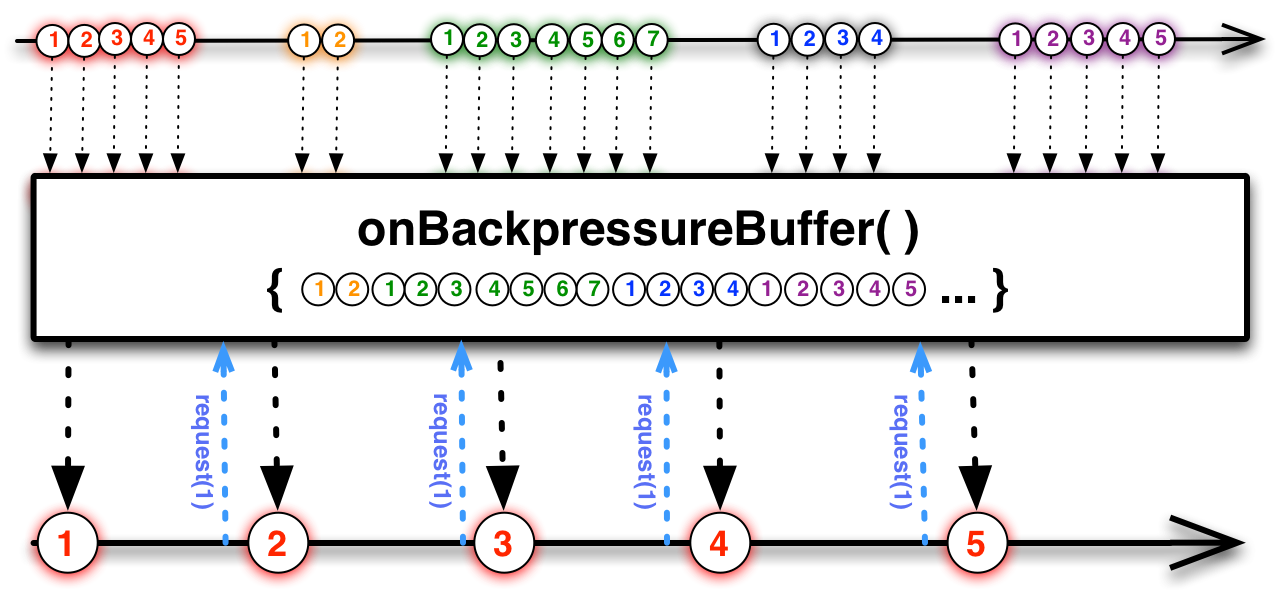
an experimental version of this operator (not available in RxJava 1.0) allows you to set the capacity of the buffer; applying this operator will cause the resulting Observable to terminate with an error if this buffer is overrun
- onBackpressureDrop
- - drops emissions from the source Observable unless there is a pending request from a downstream Subscriber, in which case it will emit enough items to fulfill the request
+ - drops emissions from the source Observable unless there is a pending request from a downstream Subscriber, in which case it will emit enough items to fulfill the request
- onBackpressureBlock (experimental, not in RxJava 1.0)
- - blocks the thread on which the source Observable is operating until such time as a Subscriber issues a request for items, and then unblocks the thread only so long as there are pending requests
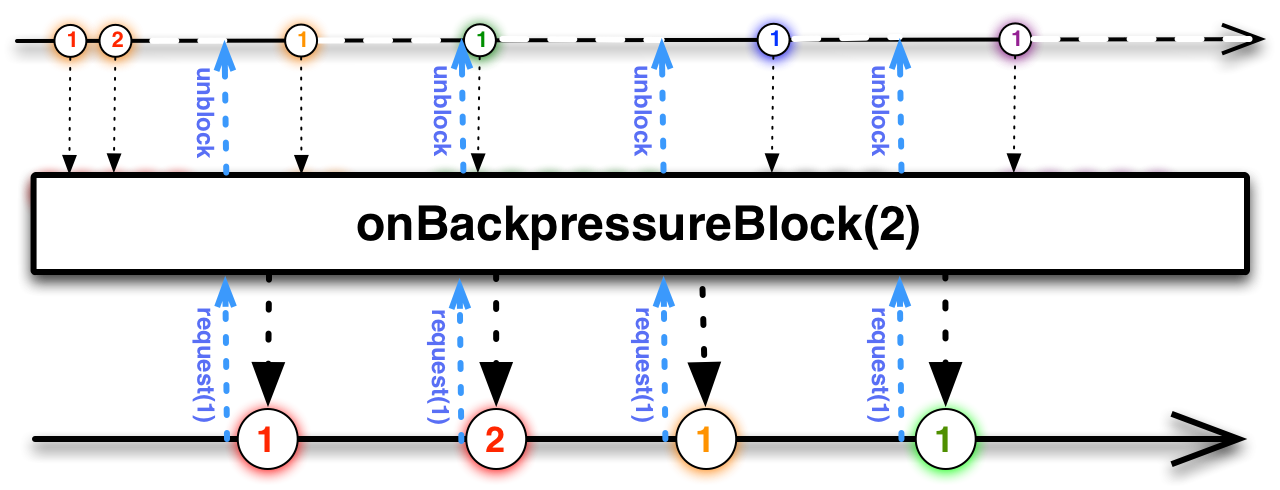
+ - blocks the thread on which the source Observable is operating until such time as a Subscriber issues a request for items, and then unblocks the thread only so long as there are pending requests
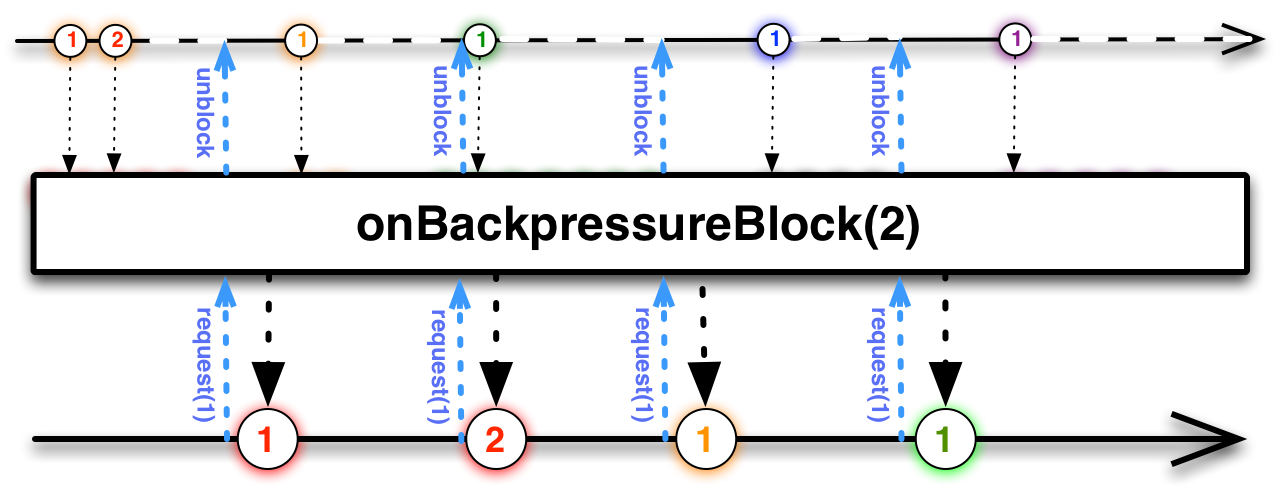
If you do not apply any of these operators to an Observable that does not support backpressure, _and_ if either you as the Subscriber or some operator between you and the Observable attempts to apply reactive pull backpressure, you will encounter a `MissingBackpressureException` which you will be notified of via your `onError()` callback.
@@ -172,4 +172,4 @@ If you do not apply any of these operators to an Observable that does not suppor
If the standard operators are providing the expected behavior, [one can write custom operators in RxJava](https://github.com/ReactiveX/RxJava/wiki/Implementing-custom-operators-(draft)).
# See also
-* [RxJava 0.20.0-RC1 release notes](https://github.com/ReactiveX/RxJava/releases/tag/0.20.0-RC1)
\ No newline at end of file
+* [RxJava 0.20.0-RC1 release notes](https://github.com/ReactiveX/RxJava/releases/tag/0.20.0-RC1)